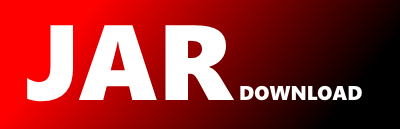
com.github.lontime.extjobrunr.beans.BackgroundJobServerStatusBean Maven / Gradle / Ivy
package com.github.lontime.extjobrunr.beans;
import java.time.Duration;
import java.time.Instant;
import java.util.Map;
import org.jobrunr.storage.BackgroundJobServerStatus;
import org.joda.beans.Bean;
import org.joda.beans.BeanBuilder;
import org.joda.beans.JodaBeanUtils;
import org.joda.beans.MetaBean;
import org.joda.beans.MetaProperty;
import org.joda.beans.Property;
import org.joda.beans.gen.BeanDefinition;
import org.joda.beans.gen.PropertyDefinition;
import org.joda.beans.impl.direct.DirectBeanBuilder;
import org.joda.beans.impl.direct.DirectMetaBean;
import org.joda.beans.impl.direct.DirectMetaProperty;
import org.joda.beans.impl.direct.DirectMetaPropertyMap;
@BeanDefinition
public class BackgroundJobServerStatusBean implements Bean {
@PropertyDefinition
private String id;
@PropertyDefinition
private Integer workerPoolSize;
@PropertyDefinition
private Integer pollIntervalInSeconds;
@PropertyDefinition
private Duration deleteSucceededJobsAfter;
@PropertyDefinition
private Duration permanentlyDeleteDeletedJobsAfter;
@PropertyDefinition
private Instant firstHeartbeat;
@PropertyDefinition
private Instant lastHeartbeat;
@PropertyDefinition
private Boolean running;
@PropertyDefinition
private Long systemTotalMemory;
@PropertyDefinition
private Long systemFreeMemory;
@PropertyDefinition
private Double systemCpuLoad;
@PropertyDefinition
private Long processMaxMemory;
@PropertyDefinition
private Long processFreeMemory;
@PropertyDefinition
private Long processAllocatedMemory;
@PropertyDefinition
private Double processCpuLoad;
public BackgroundJobServerStatusBean() {
}
public BackgroundJobServerStatusBean(BackgroundJobServerStatus serverStatus) {
this.id = serverStatus.getId().toString();
this.workerPoolSize = serverStatus.getWorkerPoolSize();
this.pollIntervalInSeconds = serverStatus.getPollIntervalInSeconds();
this.deleteSucceededJobsAfter = serverStatus.getDeleteSucceededJobsAfter();
// private final UUID id;
// private final int workerPoolSize;
// private final int pollIntervalInSeconds;
// private final Duration deleteSucceededJobsAfter;
// private final Duration permanentlyDeleteDeletedJobsAfter;
// private final Instant firstHeartbeat;
// private final Instant lastHeartbeat;
// private final Boolean running;
// private final Long systemTotalMemory;
// private final Long systemFreeMemory;
// private final Double systemCpuLoad;
// private final Long processMaxMemory;
// private final Long processFreeMemory;
// private final Long processAllocatedMemory;
// private final Double processCpuLoad;
this.permanentlyDeleteDeletedJobsAfter = serverStatus.getPermanentlyDeleteDeletedJobsAfter();
this.firstHeartbeat = serverStatus.getFirstHeartbeat();
this.lastHeartbeat = serverStatus.getLastHeartbeat();
this.running = serverStatus.isRunning();
this.systemTotalMemory = serverStatus.getSystemTotalMemory();
this.systemFreeMemory = serverStatus.getSystemFreeMemory();
this.systemCpuLoad = serverStatus.getSystemCpuLoad();
this.processMaxMemory = serverStatus.getProcessMaxMemory();
this.processFreeMemory = serverStatus.getProcessFreeMemory();
this.processAllocatedMemory = serverStatus.getProcessAllocatedMemory();
this.processCpuLoad = serverStatus.getProcessCpuLoad();
}
//------------------------- AUTOGENERATED START -------------------------
/**
* The meta-bean for {@code BackgroundJobServerStatusBean}.
* @return the meta-bean, not null
*/
public static BackgroundJobServerStatusBean.Meta meta() {
return BackgroundJobServerStatusBean.Meta.INSTANCE;
}
static {
MetaBean.register(BackgroundJobServerStatusBean.Meta.INSTANCE);
}
@Override
public BackgroundJobServerStatusBean.Meta metaBean() {
return BackgroundJobServerStatusBean.Meta.INSTANCE;
}
//-----------------------------------------------------------------------
/**
* Gets the id.
* @return the value of the property
*/
public String getId() {
return id;
}
/**
* Sets the id.
* @param id the new value of the property
*/
public void setId(String id) {
this.id = id;
}
/**
* Gets the the {@code id} property.
* @return the property, not null
*/
public final Property id() {
return metaBean().id().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the workerPoolSize.
* @return the value of the property
*/
public Integer getWorkerPoolSize() {
return workerPoolSize;
}
/**
* Sets the workerPoolSize.
* @param workerPoolSize the new value of the property
*/
public void setWorkerPoolSize(Integer workerPoolSize) {
this.workerPoolSize = workerPoolSize;
}
/**
* Gets the the {@code workerPoolSize} property.
* @return the property, not null
*/
public final Property workerPoolSize() {
return metaBean().workerPoolSize().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the pollIntervalInSeconds.
* @return the value of the property
*/
public Integer getPollIntervalInSeconds() {
return pollIntervalInSeconds;
}
/**
* Sets the pollIntervalInSeconds.
* @param pollIntervalInSeconds the new value of the property
*/
public void setPollIntervalInSeconds(Integer pollIntervalInSeconds) {
this.pollIntervalInSeconds = pollIntervalInSeconds;
}
/**
* Gets the the {@code pollIntervalInSeconds} property.
* @return the property, not null
*/
public final Property pollIntervalInSeconds() {
return metaBean().pollIntervalInSeconds().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the deleteSucceededJobsAfter.
* @return the value of the property
*/
public Duration getDeleteSucceededJobsAfter() {
return deleteSucceededJobsAfter;
}
/**
* Sets the deleteSucceededJobsAfter.
* @param deleteSucceededJobsAfter the new value of the property
*/
public void setDeleteSucceededJobsAfter(Duration deleteSucceededJobsAfter) {
this.deleteSucceededJobsAfter = deleteSucceededJobsAfter;
}
/**
* Gets the the {@code deleteSucceededJobsAfter} property.
* @return the property, not null
*/
public final Property deleteSucceededJobsAfter() {
return metaBean().deleteSucceededJobsAfter().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the permanentlyDeleteDeletedJobsAfter.
* @return the value of the property
*/
public Duration getPermanentlyDeleteDeletedJobsAfter() {
return permanentlyDeleteDeletedJobsAfter;
}
/**
* Sets the permanentlyDeleteDeletedJobsAfter.
* @param permanentlyDeleteDeletedJobsAfter the new value of the property
*/
public void setPermanentlyDeleteDeletedJobsAfter(Duration permanentlyDeleteDeletedJobsAfter) {
this.permanentlyDeleteDeletedJobsAfter = permanentlyDeleteDeletedJobsAfter;
}
/**
* Gets the the {@code permanentlyDeleteDeletedJobsAfter} property.
* @return the property, not null
*/
public final Property permanentlyDeleteDeletedJobsAfter() {
return metaBean().permanentlyDeleteDeletedJobsAfter().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the firstHeartbeat.
* @return the value of the property
*/
public Instant getFirstHeartbeat() {
return firstHeartbeat;
}
/**
* Sets the firstHeartbeat.
* @param firstHeartbeat the new value of the property
*/
public void setFirstHeartbeat(Instant firstHeartbeat) {
this.firstHeartbeat = firstHeartbeat;
}
/**
* Gets the the {@code firstHeartbeat} property.
* @return the property, not null
*/
public final Property firstHeartbeat() {
return metaBean().firstHeartbeat().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the lastHeartbeat.
* @return the value of the property
*/
public Instant getLastHeartbeat() {
return lastHeartbeat;
}
/**
* Sets the lastHeartbeat.
* @param lastHeartbeat the new value of the property
*/
public void setLastHeartbeat(Instant lastHeartbeat) {
this.lastHeartbeat = lastHeartbeat;
}
/**
* Gets the the {@code lastHeartbeat} property.
* @return the property, not null
*/
public final Property lastHeartbeat() {
return metaBean().lastHeartbeat().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the running.
* @return the value of the property
*/
public Boolean getRunning() {
return running;
}
/**
* Sets the running.
* @param running the new value of the property
*/
public void setRunning(Boolean running) {
this.running = running;
}
/**
* Gets the the {@code running} property.
* @return the property, not null
*/
public final Property running() {
return metaBean().running().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the systemTotalMemory.
* @return the value of the property
*/
public Long getSystemTotalMemory() {
return systemTotalMemory;
}
/**
* Sets the systemTotalMemory.
* @param systemTotalMemory the new value of the property
*/
public void setSystemTotalMemory(Long systemTotalMemory) {
this.systemTotalMemory = systemTotalMemory;
}
/**
* Gets the the {@code systemTotalMemory} property.
* @return the property, not null
*/
public final Property systemTotalMemory() {
return metaBean().systemTotalMemory().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the systemFreeMemory.
* @return the value of the property
*/
public Long getSystemFreeMemory() {
return systemFreeMemory;
}
/**
* Sets the systemFreeMemory.
* @param systemFreeMemory the new value of the property
*/
public void setSystemFreeMemory(Long systemFreeMemory) {
this.systemFreeMemory = systemFreeMemory;
}
/**
* Gets the the {@code systemFreeMemory} property.
* @return the property, not null
*/
public final Property systemFreeMemory() {
return metaBean().systemFreeMemory().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the systemCpuLoad.
* @return the value of the property
*/
public Double getSystemCpuLoad() {
return systemCpuLoad;
}
/**
* Sets the systemCpuLoad.
* @param systemCpuLoad the new value of the property
*/
public void setSystemCpuLoad(Double systemCpuLoad) {
this.systemCpuLoad = systemCpuLoad;
}
/**
* Gets the the {@code systemCpuLoad} property.
* @return the property, not null
*/
public final Property systemCpuLoad() {
return metaBean().systemCpuLoad().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the processMaxMemory.
* @return the value of the property
*/
public Long getProcessMaxMemory() {
return processMaxMemory;
}
/**
* Sets the processMaxMemory.
* @param processMaxMemory the new value of the property
*/
public void setProcessMaxMemory(Long processMaxMemory) {
this.processMaxMemory = processMaxMemory;
}
/**
* Gets the the {@code processMaxMemory} property.
* @return the property, not null
*/
public final Property processMaxMemory() {
return metaBean().processMaxMemory().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the processFreeMemory.
* @return the value of the property
*/
public Long getProcessFreeMemory() {
return processFreeMemory;
}
/**
* Sets the processFreeMemory.
* @param processFreeMemory the new value of the property
*/
public void setProcessFreeMemory(Long processFreeMemory) {
this.processFreeMemory = processFreeMemory;
}
/**
* Gets the the {@code processFreeMemory} property.
* @return the property, not null
*/
public final Property processFreeMemory() {
return metaBean().processFreeMemory().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the processAllocatedMemory.
* @return the value of the property
*/
public Long getProcessAllocatedMemory() {
return processAllocatedMemory;
}
/**
* Sets the processAllocatedMemory.
* @param processAllocatedMemory the new value of the property
*/
public void setProcessAllocatedMemory(Long processAllocatedMemory) {
this.processAllocatedMemory = processAllocatedMemory;
}
/**
* Gets the the {@code processAllocatedMemory} property.
* @return the property, not null
*/
public final Property processAllocatedMemory() {
return metaBean().processAllocatedMemory().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the processCpuLoad.
* @return the value of the property
*/
public Double getProcessCpuLoad() {
return processCpuLoad;
}
/**
* Sets the processCpuLoad.
* @param processCpuLoad the new value of the property
*/
public void setProcessCpuLoad(Double processCpuLoad) {
this.processCpuLoad = processCpuLoad;
}
/**
* Gets the the {@code processCpuLoad} property.
* @return the property, not null
*/
public final Property processCpuLoad() {
return metaBean().processCpuLoad().createProperty(this);
}
//-----------------------------------------------------------------------
@Override
public BackgroundJobServerStatusBean clone() {
return JodaBeanUtils.cloneAlways(this);
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj != null && obj.getClass() == this.getClass()) {
BackgroundJobServerStatusBean other = (BackgroundJobServerStatusBean) obj;
return JodaBeanUtils.equal(getId(), other.getId()) &&
JodaBeanUtils.equal(getWorkerPoolSize(), other.getWorkerPoolSize()) &&
JodaBeanUtils.equal(getPollIntervalInSeconds(), other.getPollIntervalInSeconds()) &&
JodaBeanUtils.equal(getDeleteSucceededJobsAfter(), other.getDeleteSucceededJobsAfter()) &&
JodaBeanUtils.equal(getPermanentlyDeleteDeletedJobsAfter(), other.getPermanentlyDeleteDeletedJobsAfter()) &&
JodaBeanUtils.equal(getFirstHeartbeat(), other.getFirstHeartbeat()) &&
JodaBeanUtils.equal(getLastHeartbeat(), other.getLastHeartbeat()) &&
JodaBeanUtils.equal(getRunning(), other.getRunning()) &&
JodaBeanUtils.equal(getSystemTotalMemory(), other.getSystemTotalMemory()) &&
JodaBeanUtils.equal(getSystemFreeMemory(), other.getSystemFreeMemory()) &&
JodaBeanUtils.equal(getSystemCpuLoad(), other.getSystemCpuLoad()) &&
JodaBeanUtils.equal(getProcessMaxMemory(), other.getProcessMaxMemory()) &&
JodaBeanUtils.equal(getProcessFreeMemory(), other.getProcessFreeMemory()) &&
JodaBeanUtils.equal(getProcessAllocatedMemory(), other.getProcessAllocatedMemory()) &&
JodaBeanUtils.equal(getProcessCpuLoad(), other.getProcessCpuLoad());
}
return false;
}
@Override
public int hashCode() {
int hash = getClass().hashCode();
hash = hash * 31 + JodaBeanUtils.hashCode(getId());
hash = hash * 31 + JodaBeanUtils.hashCode(getWorkerPoolSize());
hash = hash * 31 + JodaBeanUtils.hashCode(getPollIntervalInSeconds());
hash = hash * 31 + JodaBeanUtils.hashCode(getDeleteSucceededJobsAfter());
hash = hash * 31 + JodaBeanUtils.hashCode(getPermanentlyDeleteDeletedJobsAfter());
hash = hash * 31 + JodaBeanUtils.hashCode(getFirstHeartbeat());
hash = hash * 31 + JodaBeanUtils.hashCode(getLastHeartbeat());
hash = hash * 31 + JodaBeanUtils.hashCode(getRunning());
hash = hash * 31 + JodaBeanUtils.hashCode(getSystemTotalMemory());
hash = hash * 31 + JodaBeanUtils.hashCode(getSystemFreeMemory());
hash = hash * 31 + JodaBeanUtils.hashCode(getSystemCpuLoad());
hash = hash * 31 + JodaBeanUtils.hashCode(getProcessMaxMemory());
hash = hash * 31 + JodaBeanUtils.hashCode(getProcessFreeMemory());
hash = hash * 31 + JodaBeanUtils.hashCode(getProcessAllocatedMemory());
hash = hash * 31 + JodaBeanUtils.hashCode(getProcessCpuLoad());
return hash;
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder(512);
buf.append("BackgroundJobServerStatusBean{");
int len = buf.length();
toString(buf);
if (buf.length() > len) {
buf.setLength(buf.length() - 2);
}
buf.append('}');
return buf.toString();
}
protected void toString(StringBuilder buf) {
buf.append("id").append('=').append(JodaBeanUtils.toString(getId())).append(',').append(' ');
buf.append("workerPoolSize").append('=').append(JodaBeanUtils.toString(getWorkerPoolSize())).append(',').append(' ');
buf.append("pollIntervalInSeconds").append('=').append(JodaBeanUtils.toString(getPollIntervalInSeconds())).append(',').append(' ');
buf.append("deleteSucceededJobsAfter").append('=').append(JodaBeanUtils.toString(getDeleteSucceededJobsAfter())).append(',').append(' ');
buf.append("permanentlyDeleteDeletedJobsAfter").append('=').append(JodaBeanUtils.toString(getPermanentlyDeleteDeletedJobsAfter())).append(',').append(' ');
buf.append("firstHeartbeat").append('=').append(JodaBeanUtils.toString(getFirstHeartbeat())).append(',').append(' ');
buf.append("lastHeartbeat").append('=').append(JodaBeanUtils.toString(getLastHeartbeat())).append(',').append(' ');
buf.append("running").append('=').append(JodaBeanUtils.toString(getRunning())).append(',').append(' ');
buf.append("systemTotalMemory").append('=').append(JodaBeanUtils.toString(getSystemTotalMemory())).append(',').append(' ');
buf.append("systemFreeMemory").append('=').append(JodaBeanUtils.toString(getSystemFreeMemory())).append(',').append(' ');
buf.append("systemCpuLoad").append('=').append(JodaBeanUtils.toString(getSystemCpuLoad())).append(',').append(' ');
buf.append("processMaxMemory").append('=').append(JodaBeanUtils.toString(getProcessMaxMemory())).append(',').append(' ');
buf.append("processFreeMemory").append('=').append(JodaBeanUtils.toString(getProcessFreeMemory())).append(',').append(' ');
buf.append("processAllocatedMemory").append('=').append(JodaBeanUtils.toString(getProcessAllocatedMemory())).append(',').append(' ');
buf.append("processCpuLoad").append('=').append(JodaBeanUtils.toString(getProcessCpuLoad())).append(',').append(' ');
}
//-----------------------------------------------------------------------
/**
* The meta-bean for {@code BackgroundJobServerStatusBean}.
*/
public static class Meta extends DirectMetaBean {
/**
* The singleton instance of the meta-bean.
*/
static final Meta INSTANCE = new Meta();
/**
* The meta-property for the {@code id} property.
*/
private final MetaProperty id = DirectMetaProperty.ofReadWrite(
this, "id", BackgroundJobServerStatusBean.class, String.class);
/**
* The meta-property for the {@code workerPoolSize} property.
*/
private final MetaProperty workerPoolSize = DirectMetaProperty.ofReadWrite(
this, "workerPoolSize", BackgroundJobServerStatusBean.class, Integer.class);
/**
* The meta-property for the {@code pollIntervalInSeconds} property.
*/
private final MetaProperty pollIntervalInSeconds = DirectMetaProperty.ofReadWrite(
this, "pollIntervalInSeconds", BackgroundJobServerStatusBean.class, Integer.class);
/**
* The meta-property for the {@code deleteSucceededJobsAfter} property.
*/
private final MetaProperty deleteSucceededJobsAfter = DirectMetaProperty.ofReadWrite(
this, "deleteSucceededJobsAfter", BackgroundJobServerStatusBean.class, Duration.class);
/**
* The meta-property for the {@code permanentlyDeleteDeletedJobsAfter} property.
*/
private final MetaProperty permanentlyDeleteDeletedJobsAfter = DirectMetaProperty.ofReadWrite(
this, "permanentlyDeleteDeletedJobsAfter", BackgroundJobServerStatusBean.class, Duration.class);
/**
* The meta-property for the {@code firstHeartbeat} property.
*/
private final MetaProperty firstHeartbeat = DirectMetaProperty.ofReadWrite(
this, "firstHeartbeat", BackgroundJobServerStatusBean.class, Instant.class);
/**
* The meta-property for the {@code lastHeartbeat} property.
*/
private final MetaProperty lastHeartbeat = DirectMetaProperty.ofReadWrite(
this, "lastHeartbeat", BackgroundJobServerStatusBean.class, Instant.class);
/**
* The meta-property for the {@code running} property.
*/
private final MetaProperty running = DirectMetaProperty.ofReadWrite(
this, "running", BackgroundJobServerStatusBean.class, Boolean.class);
/**
* The meta-property for the {@code systemTotalMemory} property.
*/
private final MetaProperty systemTotalMemory = DirectMetaProperty.ofReadWrite(
this, "systemTotalMemory", BackgroundJobServerStatusBean.class, Long.class);
/**
* The meta-property for the {@code systemFreeMemory} property.
*/
private final MetaProperty systemFreeMemory = DirectMetaProperty.ofReadWrite(
this, "systemFreeMemory", BackgroundJobServerStatusBean.class, Long.class);
/**
* The meta-property for the {@code systemCpuLoad} property.
*/
private final MetaProperty systemCpuLoad = DirectMetaProperty.ofReadWrite(
this, "systemCpuLoad", BackgroundJobServerStatusBean.class, Double.class);
/**
* The meta-property for the {@code processMaxMemory} property.
*/
private final MetaProperty processMaxMemory = DirectMetaProperty.ofReadWrite(
this, "processMaxMemory", BackgroundJobServerStatusBean.class, Long.class);
/**
* The meta-property for the {@code processFreeMemory} property.
*/
private final MetaProperty processFreeMemory = DirectMetaProperty.ofReadWrite(
this, "processFreeMemory", BackgroundJobServerStatusBean.class, Long.class);
/**
* The meta-property for the {@code processAllocatedMemory} property.
*/
private final MetaProperty processAllocatedMemory = DirectMetaProperty.ofReadWrite(
this, "processAllocatedMemory", BackgroundJobServerStatusBean.class, Long.class);
/**
* The meta-property for the {@code processCpuLoad} property.
*/
private final MetaProperty processCpuLoad = DirectMetaProperty.ofReadWrite(
this, "processCpuLoad", BackgroundJobServerStatusBean.class, Double.class);
/**
* The meta-properties.
*/
private final Map> metaPropertyMap$ = new DirectMetaPropertyMap(
this, null,
"id",
"workerPoolSize",
"pollIntervalInSeconds",
"deleteSucceededJobsAfter",
"permanentlyDeleteDeletedJobsAfter",
"firstHeartbeat",
"lastHeartbeat",
"running",
"systemTotalMemory",
"systemFreeMemory",
"systemCpuLoad",
"processMaxMemory",
"processFreeMemory",
"processAllocatedMemory",
"processCpuLoad");
/**
* Restricted constructor.
*/
protected Meta() {
}
@Override
protected MetaProperty> metaPropertyGet(String propertyName) {
switch (propertyName.hashCode()) {
case 3355: // id
return id;
case -1564289061: // workerPoolSize
return workerPoolSize;
case 1666693206: // pollIntervalInSeconds
return pollIntervalInSeconds;
case 1841317584: // deleteSucceededJobsAfter
return deleteSucceededJobsAfter;
case 17931123: // permanentlyDeleteDeletedJobsAfter
return permanentlyDeleteDeletedJobsAfter;
case 1453769772: // firstHeartbeat
return firstHeartbeat;
case -1593473274: // lastHeartbeat
return lastHeartbeat;
case 1550783935: // running
return running;
case 488985526: // systemTotalMemory
return systemTotalMemory;
case -65741284: // systemFreeMemory
return systemFreeMemory;
case -1280587393: // systemCpuLoad
return systemCpuLoad;
case -769162794: // processMaxMemory
return processMaxMemory;
case -1278526884: // processFreeMemory
return processFreeMemory;
case 517709369: // processAllocatedMemory
return processAllocatedMemory;
case 900519231: // processCpuLoad
return processCpuLoad;
}
return super.metaPropertyGet(propertyName);
}
@Override
public BeanBuilder extends BackgroundJobServerStatusBean> builder() {
return new DirectBeanBuilder<>(new BackgroundJobServerStatusBean());
}
@Override
public Class extends BackgroundJobServerStatusBean> beanType() {
return BackgroundJobServerStatusBean.class;
}
@Override
public Map> metaPropertyMap() {
return metaPropertyMap$;
}
//-----------------------------------------------------------------------
/**
* The meta-property for the {@code id} property.
* @return the meta-property, not null
*/
public final MetaProperty id() {
return id;
}
/**
* The meta-property for the {@code workerPoolSize} property.
* @return the meta-property, not null
*/
public final MetaProperty workerPoolSize() {
return workerPoolSize;
}
/**
* The meta-property for the {@code pollIntervalInSeconds} property.
* @return the meta-property, not null
*/
public final MetaProperty pollIntervalInSeconds() {
return pollIntervalInSeconds;
}
/**
* The meta-property for the {@code deleteSucceededJobsAfter} property.
* @return the meta-property, not null
*/
public final MetaProperty deleteSucceededJobsAfter() {
return deleteSucceededJobsAfter;
}
/**
* The meta-property for the {@code permanentlyDeleteDeletedJobsAfter} property.
* @return the meta-property, not null
*/
public final MetaProperty permanentlyDeleteDeletedJobsAfter() {
return permanentlyDeleteDeletedJobsAfter;
}
/**
* The meta-property for the {@code firstHeartbeat} property.
* @return the meta-property, not null
*/
public final MetaProperty firstHeartbeat() {
return firstHeartbeat;
}
/**
* The meta-property for the {@code lastHeartbeat} property.
* @return the meta-property, not null
*/
public final MetaProperty lastHeartbeat() {
return lastHeartbeat;
}
/**
* The meta-property for the {@code running} property.
* @return the meta-property, not null
*/
public final MetaProperty running() {
return running;
}
/**
* The meta-property for the {@code systemTotalMemory} property.
* @return the meta-property, not null
*/
public final MetaProperty systemTotalMemory() {
return systemTotalMemory;
}
/**
* The meta-property for the {@code systemFreeMemory} property.
* @return the meta-property, not null
*/
public final MetaProperty systemFreeMemory() {
return systemFreeMemory;
}
/**
* The meta-property for the {@code systemCpuLoad} property.
* @return the meta-property, not null
*/
public final MetaProperty systemCpuLoad() {
return systemCpuLoad;
}
/**
* The meta-property for the {@code processMaxMemory} property.
* @return the meta-property, not null
*/
public final MetaProperty processMaxMemory() {
return processMaxMemory;
}
/**
* The meta-property for the {@code processFreeMemory} property.
* @return the meta-property, not null
*/
public final MetaProperty processFreeMemory() {
return processFreeMemory;
}
/**
* The meta-property for the {@code processAllocatedMemory} property.
* @return the meta-property, not null
*/
public final MetaProperty processAllocatedMemory() {
return processAllocatedMemory;
}
/**
* The meta-property for the {@code processCpuLoad} property.
* @return the meta-property, not null
*/
public final MetaProperty processCpuLoad() {
return processCpuLoad;
}
//-----------------------------------------------------------------------
@Override
protected Object propertyGet(Bean bean, String propertyName, boolean quiet) {
switch (propertyName.hashCode()) {
case 3355: // id
return ((BackgroundJobServerStatusBean) bean).getId();
case -1564289061: // workerPoolSize
return ((BackgroundJobServerStatusBean) bean).getWorkerPoolSize();
case 1666693206: // pollIntervalInSeconds
return ((BackgroundJobServerStatusBean) bean).getPollIntervalInSeconds();
case 1841317584: // deleteSucceededJobsAfter
return ((BackgroundJobServerStatusBean) bean).getDeleteSucceededJobsAfter();
case 17931123: // permanentlyDeleteDeletedJobsAfter
return ((BackgroundJobServerStatusBean) bean).getPermanentlyDeleteDeletedJobsAfter();
case 1453769772: // firstHeartbeat
return ((BackgroundJobServerStatusBean) bean).getFirstHeartbeat();
case -1593473274: // lastHeartbeat
return ((BackgroundJobServerStatusBean) bean).getLastHeartbeat();
case 1550783935: // running
return ((BackgroundJobServerStatusBean) bean).getRunning();
case 488985526: // systemTotalMemory
return ((BackgroundJobServerStatusBean) bean).getSystemTotalMemory();
case -65741284: // systemFreeMemory
return ((BackgroundJobServerStatusBean) bean).getSystemFreeMemory();
case -1280587393: // systemCpuLoad
return ((BackgroundJobServerStatusBean) bean).getSystemCpuLoad();
case -769162794: // processMaxMemory
return ((BackgroundJobServerStatusBean) bean).getProcessMaxMemory();
case -1278526884: // processFreeMemory
return ((BackgroundJobServerStatusBean) bean).getProcessFreeMemory();
case 517709369: // processAllocatedMemory
return ((BackgroundJobServerStatusBean) bean).getProcessAllocatedMemory();
case 900519231: // processCpuLoad
return ((BackgroundJobServerStatusBean) bean).getProcessCpuLoad();
}
return super.propertyGet(bean, propertyName, quiet);
}
@Override
protected void propertySet(Bean bean, String propertyName, Object newValue, boolean quiet) {
switch (propertyName.hashCode()) {
case 3355: // id
((BackgroundJobServerStatusBean) bean).setId((String) newValue);
return;
case -1564289061: // workerPoolSize
((BackgroundJobServerStatusBean) bean).setWorkerPoolSize((Integer) newValue);
return;
case 1666693206: // pollIntervalInSeconds
((BackgroundJobServerStatusBean) bean).setPollIntervalInSeconds((Integer) newValue);
return;
case 1841317584: // deleteSucceededJobsAfter
((BackgroundJobServerStatusBean) bean).setDeleteSucceededJobsAfter((Duration) newValue);
return;
case 17931123: // permanentlyDeleteDeletedJobsAfter
((BackgroundJobServerStatusBean) bean).setPermanentlyDeleteDeletedJobsAfter((Duration) newValue);
return;
case 1453769772: // firstHeartbeat
((BackgroundJobServerStatusBean) bean).setFirstHeartbeat((Instant) newValue);
return;
case -1593473274: // lastHeartbeat
((BackgroundJobServerStatusBean) bean).setLastHeartbeat((Instant) newValue);
return;
case 1550783935: // running
((BackgroundJobServerStatusBean) bean).setRunning((Boolean) newValue);
return;
case 488985526: // systemTotalMemory
((BackgroundJobServerStatusBean) bean).setSystemTotalMemory((Long) newValue);
return;
case -65741284: // systemFreeMemory
((BackgroundJobServerStatusBean) bean).setSystemFreeMemory((Long) newValue);
return;
case -1280587393: // systemCpuLoad
((BackgroundJobServerStatusBean) bean).setSystemCpuLoad((Double) newValue);
return;
case -769162794: // processMaxMemory
((BackgroundJobServerStatusBean) bean).setProcessMaxMemory((Long) newValue);
return;
case -1278526884: // processFreeMemory
((BackgroundJobServerStatusBean) bean).setProcessFreeMemory((Long) newValue);
return;
case 517709369: // processAllocatedMemory
((BackgroundJobServerStatusBean) bean).setProcessAllocatedMemory((Long) newValue);
return;
case 900519231: // processCpuLoad
((BackgroundJobServerStatusBean) bean).setProcessCpuLoad((Double) newValue);
return;
}
super.propertySet(bean, propertyName, newValue, quiet);
}
}
//-------------------------- AUTOGENERATED END --------------------------
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy