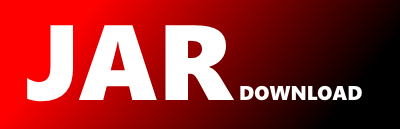
com.github.lontime.extjobrunr.beans.JobStatsExtendedBean Maven / Gradle / Ivy
package com.github.lontime.extjobrunr.beans;
import java.time.Instant;
import java.util.Map;
import org.jobrunr.storage.JobStats;
import org.jobrunr.storage.JobStatsExtended;
import org.joda.beans.Bean;
import org.joda.beans.BeanBuilder;
import org.joda.beans.JodaBeanUtils;
import org.joda.beans.MetaBean;
import org.joda.beans.MetaProperty;
import org.joda.beans.Property;
import org.joda.beans.gen.BeanDefinition;
import org.joda.beans.gen.PropertyDefinition;
import org.joda.beans.impl.direct.DirectBeanBuilder;
import org.joda.beans.impl.direct.DirectMetaBean;
import org.joda.beans.impl.direct.DirectMetaProperty;
import org.joda.beans.impl.direct.DirectMetaPropertyMap;
@BeanDefinition
public class JobStatsExtendedBean implements Bean {
@PropertyDefinition
private Long amountSucceeded;
@PropertyDefinition
private Long amountFailed;
@PropertyDefinition
private EstimationBean estimation;
@PropertyDefinition
private Instant timeStamp;
@PropertyDefinition
private Long total;
@PropertyDefinition
private Long scheduled;
@PropertyDefinition
private Long enqueued;
@PropertyDefinition
private Long processing;
@PropertyDefinition
private Long failed;
@PropertyDefinition
private Long succeeded;
@PropertyDefinition
private Long allTimeSucceeded;
@PropertyDefinition
private Long deleted;
@PropertyDefinition
private Integer recurringJobs;
@PropertyDefinition
private Integer backgroundJobServers;
public JobStatsExtendedBean() {
}
public JobStatsExtendedBean(JobStatsExtended jobStatsExtended) {
this.amountSucceeded = jobStatsExtended.getAmountSucceeded();
this.amountFailed = jobStatsExtended.getAmountFailed();
this.estimation = new EstimationBean(jobStatsExtended.getEstimation());
this.timeStamp = jobStatsExtended.getTimeStamp();
this.total = jobStatsExtended.getTotal();
this.scheduled = jobStatsExtended.getScheduled();
this.enqueued = jobStatsExtended.getEnqueued();
this.processing = jobStatsExtended.getProcessing();
this.failed = jobStatsExtended.getFailed();
this.succeeded = jobStatsExtended.getSucceeded();
this.allTimeSucceeded = jobStatsExtended.getAllTimeSucceeded();
this.deleted = jobStatsExtended.getDeleted();
this.recurringJobs = jobStatsExtended.getRecurringJobs();
this.backgroundJobServers = jobStatsExtended.getBackgroundJobServers();
}
public JobStatsExtended toJobStatsExtended() {
//Long amountSucceeded, Long amountFailed, Instant estimatedProcessingFinishedInstant
//Instant timeStamp, Long total, Long scheduled, Long enqueued, Long processing, Long failed, Long succeeded, Long allTimeSucceeded, Long deleted, int recurringJobs, int backgroundJobServers
return new JobStatsExtended(new JobStats(getTimeStamp(), getTotal(),
getScheduled(), getEnqueued(), getProcessing(),
getFailed(), getSucceeded(), getAllTimeSucceeded(),
getDeleted(), getRecurringJobs(), getBackgroundJobServers()),
getAmountSucceeded(), getAmountFailed(), getEstimation().getEstimatedProcessingFinishedAt());
}
//------------------------- AUTOGENERATED START -------------------------
/**
* The meta-bean for {@code JobStatsExtendedBean}.
* @return the meta-bean, not null
*/
public static JobStatsExtendedBean.Meta meta() {
return JobStatsExtendedBean.Meta.INSTANCE;
}
static {
MetaBean.register(JobStatsExtendedBean.Meta.INSTANCE);
}
@Override
public JobStatsExtendedBean.Meta metaBean() {
return JobStatsExtendedBean.Meta.INSTANCE;
}
//-----------------------------------------------------------------------
/**
* Gets the amountSucceeded.
* @return the value of the property
*/
public Long getAmountSucceeded() {
return amountSucceeded;
}
/**
* Sets the amountSucceeded.
* @param amountSucceeded the new value of the property
*/
public void setAmountSucceeded(Long amountSucceeded) {
this.amountSucceeded = amountSucceeded;
}
/**
* Gets the the {@code amountSucceeded} property.
* @return the property, not null
*/
public final Property amountSucceeded() {
return metaBean().amountSucceeded().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the amountFailed.
* @return the value of the property
*/
public Long getAmountFailed() {
return amountFailed;
}
/**
* Sets the amountFailed.
* @param amountFailed the new value of the property
*/
public void setAmountFailed(Long amountFailed) {
this.amountFailed = amountFailed;
}
/**
* Gets the the {@code amountFailed} property.
* @return the property, not null
*/
public final Property amountFailed() {
return metaBean().amountFailed().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the estimation.
* @return the value of the property
*/
public EstimationBean getEstimation() {
return estimation;
}
/**
* Sets the estimation.
* @param estimation the new value of the property
*/
public void setEstimation(EstimationBean estimation) {
this.estimation = estimation;
}
/**
* Gets the the {@code estimation} property.
* @return the property, not null
*/
public final Property estimation() {
return metaBean().estimation().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the timeStamp.
* @return the value of the property
*/
public Instant getTimeStamp() {
return timeStamp;
}
/**
* Sets the timeStamp.
* @param timeStamp the new value of the property
*/
public void setTimeStamp(Instant timeStamp) {
this.timeStamp = timeStamp;
}
/**
* Gets the the {@code timeStamp} property.
* @return the property, not null
*/
public final Property timeStamp() {
return metaBean().timeStamp().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the total.
* @return the value of the property
*/
public Long getTotal() {
return total;
}
/**
* Sets the total.
* @param total the new value of the property
*/
public void setTotal(Long total) {
this.total = total;
}
/**
* Gets the the {@code total} property.
* @return the property, not null
*/
public final Property total() {
return metaBean().total().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the scheduled.
* @return the value of the property
*/
public Long getScheduled() {
return scheduled;
}
/**
* Sets the scheduled.
* @param scheduled the new value of the property
*/
public void setScheduled(Long scheduled) {
this.scheduled = scheduled;
}
/**
* Gets the the {@code scheduled} property.
* @return the property, not null
*/
public final Property scheduled() {
return metaBean().scheduled().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the enqueued.
* @return the value of the property
*/
public Long getEnqueued() {
return enqueued;
}
/**
* Sets the enqueued.
* @param enqueued the new value of the property
*/
public void setEnqueued(Long enqueued) {
this.enqueued = enqueued;
}
/**
* Gets the the {@code enqueued} property.
* @return the property, not null
*/
public final Property enqueued() {
return metaBean().enqueued().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the processing.
* @return the value of the property
*/
public Long getProcessing() {
return processing;
}
/**
* Sets the processing.
* @param processing the new value of the property
*/
public void setProcessing(Long processing) {
this.processing = processing;
}
/**
* Gets the the {@code processing} property.
* @return the property, not null
*/
public final Property processing() {
return metaBean().processing().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the failed.
* @return the value of the property
*/
public Long getFailed() {
return failed;
}
/**
* Sets the failed.
* @param failed the new value of the property
*/
public void setFailed(Long failed) {
this.failed = failed;
}
/**
* Gets the the {@code failed} property.
* @return the property, not null
*/
public final Property failed() {
return metaBean().failed().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the succeeded.
* @return the value of the property
*/
public Long getSucceeded() {
return succeeded;
}
/**
* Sets the succeeded.
* @param succeeded the new value of the property
*/
public void setSucceeded(Long succeeded) {
this.succeeded = succeeded;
}
/**
* Gets the the {@code succeeded} property.
* @return the property, not null
*/
public final Property succeeded() {
return metaBean().succeeded().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the allTimeSucceeded.
* @return the value of the property
*/
public Long getAllTimeSucceeded() {
return allTimeSucceeded;
}
/**
* Sets the allTimeSucceeded.
* @param allTimeSucceeded the new value of the property
*/
public void setAllTimeSucceeded(Long allTimeSucceeded) {
this.allTimeSucceeded = allTimeSucceeded;
}
/**
* Gets the the {@code allTimeSucceeded} property.
* @return the property, not null
*/
public final Property allTimeSucceeded() {
return metaBean().allTimeSucceeded().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the deleted.
* @return the value of the property
*/
public Long getDeleted() {
return deleted;
}
/**
* Sets the deleted.
* @param deleted the new value of the property
*/
public void setDeleted(Long deleted) {
this.deleted = deleted;
}
/**
* Gets the the {@code deleted} property.
* @return the property, not null
*/
public final Property deleted() {
return metaBean().deleted().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the recurringJobs.
* @return the value of the property
*/
public Integer getRecurringJobs() {
return recurringJobs;
}
/**
* Sets the recurringJobs.
* @param recurringJobs the new value of the property
*/
public void setRecurringJobs(Integer recurringJobs) {
this.recurringJobs = recurringJobs;
}
/**
* Gets the the {@code recurringJobs} property.
* @return the property, not null
*/
public final Property recurringJobs() {
return metaBean().recurringJobs().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the backgroundJobServers.
* @return the value of the property
*/
public Integer getBackgroundJobServers() {
return backgroundJobServers;
}
/**
* Sets the backgroundJobServers.
* @param backgroundJobServers the new value of the property
*/
public void setBackgroundJobServers(Integer backgroundJobServers) {
this.backgroundJobServers = backgroundJobServers;
}
/**
* Gets the the {@code backgroundJobServers} property.
* @return the property, not null
*/
public final Property backgroundJobServers() {
return metaBean().backgroundJobServers().createProperty(this);
}
//-----------------------------------------------------------------------
@Override
public JobStatsExtendedBean clone() {
return JodaBeanUtils.cloneAlways(this);
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj != null && obj.getClass() == this.getClass()) {
JobStatsExtendedBean other = (JobStatsExtendedBean) obj;
return JodaBeanUtils.equal(getAmountSucceeded(), other.getAmountSucceeded()) &&
JodaBeanUtils.equal(getAmountFailed(), other.getAmountFailed()) &&
JodaBeanUtils.equal(getEstimation(), other.getEstimation()) &&
JodaBeanUtils.equal(getTimeStamp(), other.getTimeStamp()) &&
JodaBeanUtils.equal(getTotal(), other.getTotal()) &&
JodaBeanUtils.equal(getScheduled(), other.getScheduled()) &&
JodaBeanUtils.equal(getEnqueued(), other.getEnqueued()) &&
JodaBeanUtils.equal(getProcessing(), other.getProcessing()) &&
JodaBeanUtils.equal(getFailed(), other.getFailed()) &&
JodaBeanUtils.equal(getSucceeded(), other.getSucceeded()) &&
JodaBeanUtils.equal(getAllTimeSucceeded(), other.getAllTimeSucceeded()) &&
JodaBeanUtils.equal(getDeleted(), other.getDeleted()) &&
JodaBeanUtils.equal(getRecurringJobs(), other.getRecurringJobs()) &&
JodaBeanUtils.equal(getBackgroundJobServers(), other.getBackgroundJobServers());
}
return false;
}
@Override
public int hashCode() {
int hash = getClass().hashCode();
hash = hash * 31 + JodaBeanUtils.hashCode(getAmountSucceeded());
hash = hash * 31 + JodaBeanUtils.hashCode(getAmountFailed());
hash = hash * 31 + JodaBeanUtils.hashCode(getEstimation());
hash = hash * 31 + JodaBeanUtils.hashCode(getTimeStamp());
hash = hash * 31 + JodaBeanUtils.hashCode(getTotal());
hash = hash * 31 + JodaBeanUtils.hashCode(getScheduled());
hash = hash * 31 + JodaBeanUtils.hashCode(getEnqueued());
hash = hash * 31 + JodaBeanUtils.hashCode(getProcessing());
hash = hash * 31 + JodaBeanUtils.hashCode(getFailed());
hash = hash * 31 + JodaBeanUtils.hashCode(getSucceeded());
hash = hash * 31 + JodaBeanUtils.hashCode(getAllTimeSucceeded());
hash = hash * 31 + JodaBeanUtils.hashCode(getDeleted());
hash = hash * 31 + JodaBeanUtils.hashCode(getRecurringJobs());
hash = hash * 31 + JodaBeanUtils.hashCode(getBackgroundJobServers());
return hash;
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder(480);
buf.append("JobStatsExtendedBean{");
int len = buf.length();
toString(buf);
if (buf.length() > len) {
buf.setLength(buf.length() - 2);
}
buf.append('}');
return buf.toString();
}
protected void toString(StringBuilder buf) {
buf.append("amountSucceeded").append('=').append(JodaBeanUtils.toString(getAmountSucceeded())).append(',').append(' ');
buf.append("amountFailed").append('=').append(JodaBeanUtils.toString(getAmountFailed())).append(',').append(' ');
buf.append("estimation").append('=').append(JodaBeanUtils.toString(getEstimation())).append(',').append(' ');
buf.append("timeStamp").append('=').append(JodaBeanUtils.toString(getTimeStamp())).append(',').append(' ');
buf.append("total").append('=').append(JodaBeanUtils.toString(getTotal())).append(',').append(' ');
buf.append("scheduled").append('=').append(JodaBeanUtils.toString(getScheduled())).append(',').append(' ');
buf.append("enqueued").append('=').append(JodaBeanUtils.toString(getEnqueued())).append(',').append(' ');
buf.append("processing").append('=').append(JodaBeanUtils.toString(getProcessing())).append(',').append(' ');
buf.append("failed").append('=').append(JodaBeanUtils.toString(getFailed())).append(',').append(' ');
buf.append("succeeded").append('=').append(JodaBeanUtils.toString(getSucceeded())).append(',').append(' ');
buf.append("allTimeSucceeded").append('=').append(JodaBeanUtils.toString(getAllTimeSucceeded())).append(',').append(' ');
buf.append("deleted").append('=').append(JodaBeanUtils.toString(getDeleted())).append(',').append(' ');
buf.append("recurringJobs").append('=').append(JodaBeanUtils.toString(getRecurringJobs())).append(',').append(' ');
buf.append("backgroundJobServers").append('=').append(JodaBeanUtils.toString(getBackgroundJobServers())).append(',').append(' ');
}
//-----------------------------------------------------------------------
/**
* The meta-bean for {@code JobStatsExtendedBean}.
*/
public static class Meta extends DirectMetaBean {
/**
* The singleton instance of the meta-bean.
*/
static final Meta INSTANCE = new Meta();
/**
* The meta-property for the {@code amountSucceeded} property.
*/
private final MetaProperty amountSucceeded = DirectMetaProperty.ofReadWrite(
this, "amountSucceeded", JobStatsExtendedBean.class, Long.class);
/**
* The meta-property for the {@code amountFailed} property.
*/
private final MetaProperty amountFailed = DirectMetaProperty.ofReadWrite(
this, "amountFailed", JobStatsExtendedBean.class, Long.class);
/**
* The meta-property for the {@code estimation} property.
*/
private final MetaProperty estimation = DirectMetaProperty.ofReadWrite(
this, "estimation", JobStatsExtendedBean.class, EstimationBean.class);
/**
* The meta-property for the {@code timeStamp} property.
*/
private final MetaProperty timeStamp = DirectMetaProperty.ofReadWrite(
this, "timeStamp", JobStatsExtendedBean.class, Instant.class);
/**
* The meta-property for the {@code total} property.
*/
private final MetaProperty total = DirectMetaProperty.ofReadWrite(
this, "total", JobStatsExtendedBean.class, Long.class);
/**
* The meta-property for the {@code scheduled} property.
*/
private final MetaProperty scheduled = DirectMetaProperty.ofReadWrite(
this, "scheduled", JobStatsExtendedBean.class, Long.class);
/**
* The meta-property for the {@code enqueued} property.
*/
private final MetaProperty enqueued = DirectMetaProperty.ofReadWrite(
this, "enqueued", JobStatsExtendedBean.class, Long.class);
/**
* The meta-property for the {@code processing} property.
*/
private final MetaProperty processing = DirectMetaProperty.ofReadWrite(
this, "processing", JobStatsExtendedBean.class, Long.class);
/**
* The meta-property for the {@code failed} property.
*/
private final MetaProperty failed = DirectMetaProperty.ofReadWrite(
this, "failed", JobStatsExtendedBean.class, Long.class);
/**
* The meta-property for the {@code succeeded} property.
*/
private final MetaProperty succeeded = DirectMetaProperty.ofReadWrite(
this, "succeeded", JobStatsExtendedBean.class, Long.class);
/**
* The meta-property for the {@code allTimeSucceeded} property.
*/
private final MetaProperty allTimeSucceeded = DirectMetaProperty.ofReadWrite(
this, "allTimeSucceeded", JobStatsExtendedBean.class, Long.class);
/**
* The meta-property for the {@code deleted} property.
*/
private final MetaProperty deleted = DirectMetaProperty.ofReadWrite(
this, "deleted", JobStatsExtendedBean.class, Long.class);
/**
* The meta-property for the {@code recurringJobs} property.
*/
private final MetaProperty recurringJobs = DirectMetaProperty.ofReadWrite(
this, "recurringJobs", JobStatsExtendedBean.class, Integer.class);
/**
* The meta-property for the {@code backgroundJobServers} property.
*/
private final MetaProperty backgroundJobServers = DirectMetaProperty.ofReadWrite(
this, "backgroundJobServers", JobStatsExtendedBean.class, Integer.class);
/**
* The meta-properties.
*/
private final Map> metaPropertyMap$ = new DirectMetaPropertyMap(
this, null,
"amountSucceeded",
"amountFailed",
"estimation",
"timeStamp",
"total",
"scheduled",
"enqueued",
"processing",
"failed",
"succeeded",
"allTimeSucceeded",
"deleted",
"recurringJobs",
"backgroundJobServers");
/**
* Restricted constructor.
*/
protected Meta() {
}
@Override
protected MetaProperty> metaPropertyGet(String propertyName) {
switch (propertyName.hashCode()) {
case -2115013399: // amountSucceeded
return amountSucceeded;
case 383659701: // amountFailed
return amountFailed;
case 2143000587: // estimation
return estimation;
case 25573622: // timeStamp
return timeStamp;
case 110549828: // total
return total;
case -160710483: // scheduled
return scheduled;
case 2117612380: // enqueued
return enqueued;
case 422194963: // processing
return processing;
case -1281977283: // failed
return failed;
case 945734241: // succeeded
return succeeded;
case -1955821069: // allTimeSucceeded
return allTimeSucceeded;
case 1550463001: // deleted
return deleted;
case 908232915: // recurringJobs
return recurringJobs;
case 127960801: // backgroundJobServers
return backgroundJobServers;
}
return super.metaPropertyGet(propertyName);
}
@Override
public BeanBuilder extends JobStatsExtendedBean> builder() {
return new DirectBeanBuilder<>(new JobStatsExtendedBean());
}
@Override
public Class extends JobStatsExtendedBean> beanType() {
return JobStatsExtendedBean.class;
}
@Override
public Map> metaPropertyMap() {
return metaPropertyMap$;
}
//-----------------------------------------------------------------------
/**
* The meta-property for the {@code amountSucceeded} property.
* @return the meta-property, not null
*/
public final MetaProperty amountSucceeded() {
return amountSucceeded;
}
/**
* The meta-property for the {@code amountFailed} property.
* @return the meta-property, not null
*/
public final MetaProperty amountFailed() {
return amountFailed;
}
/**
* The meta-property for the {@code estimation} property.
* @return the meta-property, not null
*/
public final MetaProperty estimation() {
return estimation;
}
/**
* The meta-property for the {@code timeStamp} property.
* @return the meta-property, not null
*/
public final MetaProperty timeStamp() {
return timeStamp;
}
/**
* The meta-property for the {@code total} property.
* @return the meta-property, not null
*/
public final MetaProperty total() {
return total;
}
/**
* The meta-property for the {@code scheduled} property.
* @return the meta-property, not null
*/
public final MetaProperty scheduled() {
return scheduled;
}
/**
* The meta-property for the {@code enqueued} property.
* @return the meta-property, not null
*/
public final MetaProperty enqueued() {
return enqueued;
}
/**
* The meta-property for the {@code processing} property.
* @return the meta-property, not null
*/
public final MetaProperty processing() {
return processing;
}
/**
* The meta-property for the {@code failed} property.
* @return the meta-property, not null
*/
public final MetaProperty failed() {
return failed;
}
/**
* The meta-property for the {@code succeeded} property.
* @return the meta-property, not null
*/
public final MetaProperty succeeded() {
return succeeded;
}
/**
* The meta-property for the {@code allTimeSucceeded} property.
* @return the meta-property, not null
*/
public final MetaProperty allTimeSucceeded() {
return allTimeSucceeded;
}
/**
* The meta-property for the {@code deleted} property.
* @return the meta-property, not null
*/
public final MetaProperty deleted() {
return deleted;
}
/**
* The meta-property for the {@code recurringJobs} property.
* @return the meta-property, not null
*/
public final MetaProperty recurringJobs() {
return recurringJobs;
}
/**
* The meta-property for the {@code backgroundJobServers} property.
* @return the meta-property, not null
*/
public final MetaProperty backgroundJobServers() {
return backgroundJobServers;
}
//-----------------------------------------------------------------------
@Override
protected Object propertyGet(Bean bean, String propertyName, boolean quiet) {
switch (propertyName.hashCode()) {
case -2115013399: // amountSucceeded
return ((JobStatsExtendedBean) bean).getAmountSucceeded();
case 383659701: // amountFailed
return ((JobStatsExtendedBean) bean).getAmountFailed();
case 2143000587: // estimation
return ((JobStatsExtendedBean) bean).getEstimation();
case 25573622: // timeStamp
return ((JobStatsExtendedBean) bean).getTimeStamp();
case 110549828: // total
return ((JobStatsExtendedBean) bean).getTotal();
case -160710483: // scheduled
return ((JobStatsExtendedBean) bean).getScheduled();
case 2117612380: // enqueued
return ((JobStatsExtendedBean) bean).getEnqueued();
case 422194963: // processing
return ((JobStatsExtendedBean) bean).getProcessing();
case -1281977283: // failed
return ((JobStatsExtendedBean) bean).getFailed();
case 945734241: // succeeded
return ((JobStatsExtendedBean) bean).getSucceeded();
case -1955821069: // allTimeSucceeded
return ((JobStatsExtendedBean) bean).getAllTimeSucceeded();
case 1550463001: // deleted
return ((JobStatsExtendedBean) bean).getDeleted();
case 908232915: // recurringJobs
return ((JobStatsExtendedBean) bean).getRecurringJobs();
case 127960801: // backgroundJobServers
return ((JobStatsExtendedBean) bean).getBackgroundJobServers();
}
return super.propertyGet(bean, propertyName, quiet);
}
@Override
protected void propertySet(Bean bean, String propertyName, Object newValue, boolean quiet) {
switch (propertyName.hashCode()) {
case -2115013399: // amountSucceeded
((JobStatsExtendedBean) bean).setAmountSucceeded((Long) newValue);
return;
case 383659701: // amountFailed
((JobStatsExtendedBean) bean).setAmountFailed((Long) newValue);
return;
case 2143000587: // estimation
((JobStatsExtendedBean) bean).setEstimation((EstimationBean) newValue);
return;
case 25573622: // timeStamp
((JobStatsExtendedBean) bean).setTimeStamp((Instant) newValue);
return;
case 110549828: // total
((JobStatsExtendedBean) bean).setTotal((Long) newValue);
return;
case -160710483: // scheduled
((JobStatsExtendedBean) bean).setScheduled((Long) newValue);
return;
case 2117612380: // enqueued
((JobStatsExtendedBean) bean).setEnqueued((Long) newValue);
return;
case 422194963: // processing
((JobStatsExtendedBean) bean).setProcessing((Long) newValue);
return;
case -1281977283: // failed
((JobStatsExtendedBean) bean).setFailed((Long) newValue);
return;
case 945734241: // succeeded
((JobStatsExtendedBean) bean).setSucceeded((Long) newValue);
return;
case -1955821069: // allTimeSucceeded
((JobStatsExtendedBean) bean).setAllTimeSucceeded((Long) newValue);
return;
case 1550463001: // deleted
((JobStatsExtendedBean) bean).setDeleted((Long) newValue);
return;
case 908232915: // recurringJobs
((JobStatsExtendedBean) bean).setRecurringJobs((Integer) newValue);
return;
case 127960801: // backgroundJobServers
((JobStatsExtendedBean) bean).setBackgroundJobServers((Integer) newValue);
return;
}
super.propertySet(bean, propertyName, newValue, quiet);
}
}
//-------------------------- AUTOGENERATED END --------------------------
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy