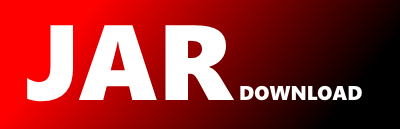
com.github.lontime.extjobrunr.beans.RecurringJobUIModelBean Maven / Gradle / Ivy
package com.github.lontime.extjobrunr.beans;
import java.time.Instant;
import java.util.Map;
import org.jobrunr.dashboard.ui.model.RecurringJobUIModel;
import org.jobrunr.jobs.RecurringJob;
import org.joda.beans.Bean;
import org.joda.beans.BeanBuilder;
import org.joda.beans.JodaBeanUtils;
import org.joda.beans.MetaBean;
import org.joda.beans.MetaProperty;
import org.joda.beans.Property;
import org.joda.beans.gen.BeanDefinition;
import org.joda.beans.gen.PropertyDefinition;
import org.joda.beans.impl.direct.DirectBeanBuilder;
import org.joda.beans.impl.direct.DirectMetaBean;
import org.joda.beans.impl.direct.DirectMetaProperty;
import org.joda.beans.impl.direct.DirectMetaPropertyMap;
@BeanDefinition
public class RecurringJobUIModelBean implements Bean {
@PropertyDefinition
private Instant nextRun;
@PropertyDefinition
private String id;
@PropertyDefinition
private String cronExpression;
@PropertyDefinition
private String zoneId;
@PropertyDefinition
private Integer version;
@PropertyDefinition
private String jobSignature;
@PropertyDefinition
private String jobName;
@PropertyDefinition
private JobDetailsBean jobDetails;
public RecurringJobUIModelBean() {
}
public RecurringJobUIModelBean(RecurringJobUIModel recurringJobUIModel) {
this.id = recurringJobUIModel.getId();
this.cronExpression = recurringJobUIModel.getScheduleExpression();
this.zoneId = recurringJobUIModel.getZoneId();
this.version = recurringJobUIModel.getVersion();
this.jobSignature = recurringJobUIModel.getJobSignature();
this.jobName = recurringJobUIModel.getJobName();
this.jobDetails = new JobDetailsBean(recurringJobUIModel.getJobDetails());
this.nextRun = recurringJobUIModel.getNextRun();
}
public RecurringJobUIModel toRecurringJobUIModel() {
final RecurringJob job = new RecurringJob(getId(),
getJobDetails().toJobDetails(),
getCronExpression(),
getZoneId());
job.setJobName(getJobName());
return new RecurringJobUIModel(job);
}
//------------------------- AUTOGENERATED START -------------------------
/**
* The meta-bean for {@code RecurringJobUIModelBean}.
* @return the meta-bean, not null
*/
public static RecurringJobUIModelBean.Meta meta() {
return RecurringJobUIModelBean.Meta.INSTANCE;
}
static {
MetaBean.register(RecurringJobUIModelBean.Meta.INSTANCE);
}
@Override
public RecurringJobUIModelBean.Meta metaBean() {
return RecurringJobUIModelBean.Meta.INSTANCE;
}
//-----------------------------------------------------------------------
/**
* Gets the nextRun.
* @return the value of the property
*/
public Instant getNextRun() {
return nextRun;
}
/**
* Sets the nextRun.
* @param nextRun the new value of the property
*/
public void setNextRun(Instant nextRun) {
this.nextRun = nextRun;
}
/**
* Gets the the {@code nextRun} property.
* @return the property, not null
*/
public final Property nextRun() {
return metaBean().nextRun().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the id.
* @return the value of the property
*/
public String getId() {
return id;
}
/**
* Sets the id.
* @param id the new value of the property
*/
public void setId(String id) {
this.id = id;
}
/**
* Gets the the {@code id} property.
* @return the property, not null
*/
public final Property id() {
return metaBean().id().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the cronExpression.
* @return the value of the property
*/
public String getCronExpression() {
return cronExpression;
}
/**
* Sets the cronExpression.
* @param cronExpression the new value of the property
*/
public void setCronExpression(String cronExpression) {
this.cronExpression = cronExpression;
}
/**
* Gets the the {@code cronExpression} property.
* @return the property, not null
*/
public final Property cronExpression() {
return metaBean().cronExpression().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the zoneId.
* @return the value of the property
*/
public String getZoneId() {
return zoneId;
}
/**
* Sets the zoneId.
* @param zoneId the new value of the property
*/
public void setZoneId(String zoneId) {
this.zoneId = zoneId;
}
/**
* Gets the the {@code zoneId} property.
* @return the property, not null
*/
public final Property zoneId() {
return metaBean().zoneId().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the version.
* @return the value of the property
*/
public Integer getVersion() {
return version;
}
/**
* Sets the version.
* @param version the new value of the property
*/
public void setVersion(Integer version) {
this.version = version;
}
/**
* Gets the the {@code version} property.
* @return the property, not null
*/
public final Property version() {
return metaBean().version().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the jobSignature.
* @return the value of the property
*/
public String getJobSignature() {
return jobSignature;
}
/**
* Sets the jobSignature.
* @param jobSignature the new value of the property
*/
public void setJobSignature(String jobSignature) {
this.jobSignature = jobSignature;
}
/**
* Gets the the {@code jobSignature} property.
* @return the property, not null
*/
public final Property jobSignature() {
return metaBean().jobSignature().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the jobName.
* @return the value of the property
*/
public String getJobName() {
return jobName;
}
/**
* Sets the jobName.
* @param jobName the new value of the property
*/
public void setJobName(String jobName) {
this.jobName = jobName;
}
/**
* Gets the the {@code jobName} property.
* @return the property, not null
*/
public final Property jobName() {
return metaBean().jobName().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the jobDetails.
* @return the value of the property
*/
public JobDetailsBean getJobDetails() {
return jobDetails;
}
/**
* Sets the jobDetails.
* @param jobDetails the new value of the property
*/
public void setJobDetails(JobDetailsBean jobDetails) {
this.jobDetails = jobDetails;
}
/**
* Gets the the {@code jobDetails} property.
* @return the property, not null
*/
public final Property jobDetails() {
return metaBean().jobDetails().createProperty(this);
}
//-----------------------------------------------------------------------
@Override
public RecurringJobUIModelBean clone() {
return JodaBeanUtils.cloneAlways(this);
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj != null && obj.getClass() == this.getClass()) {
RecurringJobUIModelBean other = (RecurringJobUIModelBean) obj;
return JodaBeanUtils.equal(getNextRun(), other.getNextRun()) &&
JodaBeanUtils.equal(getId(), other.getId()) &&
JodaBeanUtils.equal(getCronExpression(), other.getCronExpression()) &&
JodaBeanUtils.equal(getZoneId(), other.getZoneId()) &&
JodaBeanUtils.equal(getVersion(), other.getVersion()) &&
JodaBeanUtils.equal(getJobSignature(), other.getJobSignature()) &&
JodaBeanUtils.equal(getJobName(), other.getJobName()) &&
JodaBeanUtils.equal(getJobDetails(), other.getJobDetails());
}
return false;
}
@Override
public int hashCode() {
int hash = getClass().hashCode();
hash = hash * 31 + JodaBeanUtils.hashCode(getNextRun());
hash = hash * 31 + JodaBeanUtils.hashCode(getId());
hash = hash * 31 + JodaBeanUtils.hashCode(getCronExpression());
hash = hash * 31 + JodaBeanUtils.hashCode(getZoneId());
hash = hash * 31 + JodaBeanUtils.hashCode(getVersion());
hash = hash * 31 + JodaBeanUtils.hashCode(getJobSignature());
hash = hash * 31 + JodaBeanUtils.hashCode(getJobName());
hash = hash * 31 + JodaBeanUtils.hashCode(getJobDetails());
return hash;
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder(288);
buf.append("RecurringJobUIModelBean{");
int len = buf.length();
toString(buf);
if (buf.length() > len) {
buf.setLength(buf.length() - 2);
}
buf.append('}');
return buf.toString();
}
protected void toString(StringBuilder buf) {
buf.append("nextRun").append('=').append(JodaBeanUtils.toString(getNextRun())).append(',').append(' ');
buf.append("id").append('=').append(JodaBeanUtils.toString(getId())).append(',').append(' ');
buf.append("cronExpression").append('=').append(JodaBeanUtils.toString(getCronExpression())).append(',').append(' ');
buf.append("zoneId").append('=').append(JodaBeanUtils.toString(getZoneId())).append(',').append(' ');
buf.append("version").append('=').append(JodaBeanUtils.toString(getVersion())).append(',').append(' ');
buf.append("jobSignature").append('=').append(JodaBeanUtils.toString(getJobSignature())).append(',').append(' ');
buf.append("jobName").append('=').append(JodaBeanUtils.toString(getJobName())).append(',').append(' ');
buf.append("jobDetails").append('=').append(JodaBeanUtils.toString(getJobDetails())).append(',').append(' ');
}
//-----------------------------------------------------------------------
/**
* The meta-bean for {@code RecurringJobUIModelBean}.
*/
public static class Meta extends DirectMetaBean {
/**
* The singleton instance of the meta-bean.
*/
static final Meta INSTANCE = new Meta();
/**
* The meta-property for the {@code nextRun} property.
*/
private final MetaProperty nextRun = DirectMetaProperty.ofReadWrite(
this, "nextRun", RecurringJobUIModelBean.class, Instant.class);
/**
* The meta-property for the {@code id} property.
*/
private final MetaProperty id = DirectMetaProperty.ofReadWrite(
this, "id", RecurringJobUIModelBean.class, String.class);
/**
* The meta-property for the {@code cronExpression} property.
*/
private final MetaProperty cronExpression = DirectMetaProperty.ofReadWrite(
this, "cronExpression", RecurringJobUIModelBean.class, String.class);
/**
* The meta-property for the {@code zoneId} property.
*/
private final MetaProperty zoneId = DirectMetaProperty.ofReadWrite(
this, "zoneId", RecurringJobUIModelBean.class, String.class);
/**
* The meta-property for the {@code version} property.
*/
private final MetaProperty version = DirectMetaProperty.ofReadWrite(
this, "version", RecurringJobUIModelBean.class, Integer.class);
/**
* The meta-property for the {@code jobSignature} property.
*/
private final MetaProperty jobSignature = DirectMetaProperty.ofReadWrite(
this, "jobSignature", RecurringJobUIModelBean.class, String.class);
/**
* The meta-property for the {@code jobName} property.
*/
private final MetaProperty jobName = DirectMetaProperty.ofReadWrite(
this, "jobName", RecurringJobUIModelBean.class, String.class);
/**
* The meta-property for the {@code jobDetails} property.
*/
private final MetaProperty jobDetails = DirectMetaProperty.ofReadWrite(
this, "jobDetails", RecurringJobUIModelBean.class, JobDetailsBean.class);
/**
* The meta-properties.
*/
private final Map> metaPropertyMap$ = new DirectMetaPropertyMap(
this, null,
"nextRun",
"id",
"cronExpression",
"zoneId",
"version",
"jobSignature",
"jobName",
"jobDetails");
/**
* Restricted constructor.
*/
protected Meta() {
}
@Override
protected MetaProperty> metaPropertyGet(String propertyName) {
switch (propertyName.hashCode()) {
case 1847062168: // nextRun
return nextRun;
case 3355: // id
return id;
case -348729402: // cronExpression
return cronExpression;
case -696323609: // zoneId
return zoneId;
case 351608024: // version
return version;
case -96140933: // jobSignature
return jobSignature;
case -1438096408: // jobName
return jobName;
case -195448891: // jobDetails
return jobDetails;
}
return super.metaPropertyGet(propertyName);
}
@Override
public BeanBuilder extends RecurringJobUIModelBean> builder() {
return new DirectBeanBuilder<>(new RecurringJobUIModelBean());
}
@Override
public Class extends RecurringJobUIModelBean> beanType() {
return RecurringJobUIModelBean.class;
}
@Override
public Map> metaPropertyMap() {
return metaPropertyMap$;
}
//-----------------------------------------------------------------------
/**
* The meta-property for the {@code nextRun} property.
* @return the meta-property, not null
*/
public final MetaProperty nextRun() {
return nextRun;
}
/**
* The meta-property for the {@code id} property.
* @return the meta-property, not null
*/
public final MetaProperty id() {
return id;
}
/**
* The meta-property for the {@code cronExpression} property.
* @return the meta-property, not null
*/
public final MetaProperty cronExpression() {
return cronExpression;
}
/**
* The meta-property for the {@code zoneId} property.
* @return the meta-property, not null
*/
public final MetaProperty zoneId() {
return zoneId;
}
/**
* The meta-property for the {@code version} property.
* @return the meta-property, not null
*/
public final MetaProperty version() {
return version;
}
/**
* The meta-property for the {@code jobSignature} property.
* @return the meta-property, not null
*/
public final MetaProperty jobSignature() {
return jobSignature;
}
/**
* The meta-property for the {@code jobName} property.
* @return the meta-property, not null
*/
public final MetaProperty jobName() {
return jobName;
}
/**
* The meta-property for the {@code jobDetails} property.
* @return the meta-property, not null
*/
public final MetaProperty jobDetails() {
return jobDetails;
}
//-----------------------------------------------------------------------
@Override
protected Object propertyGet(Bean bean, String propertyName, boolean quiet) {
switch (propertyName.hashCode()) {
case 1847062168: // nextRun
return ((RecurringJobUIModelBean) bean).getNextRun();
case 3355: // id
return ((RecurringJobUIModelBean) bean).getId();
case -348729402: // cronExpression
return ((RecurringJobUIModelBean) bean).getCronExpression();
case -696323609: // zoneId
return ((RecurringJobUIModelBean) bean).getZoneId();
case 351608024: // version
return ((RecurringJobUIModelBean) bean).getVersion();
case -96140933: // jobSignature
return ((RecurringJobUIModelBean) bean).getJobSignature();
case -1438096408: // jobName
return ((RecurringJobUIModelBean) bean).getJobName();
case -195448891: // jobDetails
return ((RecurringJobUIModelBean) bean).getJobDetails();
}
return super.propertyGet(bean, propertyName, quiet);
}
@Override
protected void propertySet(Bean bean, String propertyName, Object newValue, boolean quiet) {
switch (propertyName.hashCode()) {
case 1847062168: // nextRun
((RecurringJobUIModelBean) bean).setNextRun((Instant) newValue);
return;
case 3355: // id
((RecurringJobUIModelBean) bean).setId((String) newValue);
return;
case -348729402: // cronExpression
((RecurringJobUIModelBean) bean).setCronExpression((String) newValue);
return;
case -696323609: // zoneId
((RecurringJobUIModelBean) bean).setZoneId((String) newValue);
return;
case 351608024: // version
((RecurringJobUIModelBean) bean).setVersion((Integer) newValue);
return;
case -96140933: // jobSignature
((RecurringJobUIModelBean) bean).setJobSignature((String) newValue);
return;
case -1438096408: // jobName
((RecurringJobUIModelBean) bean).setJobName((String) newValue);
return;
case -195448891: // jobDetails
((RecurringJobUIModelBean) bean).setJobDetails((JobDetailsBean) newValue);
return;
}
super.propertySet(bean, propertyName, newValue, quiet);
}
}
//-------------------------- AUTOGENERATED END --------------------------
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy