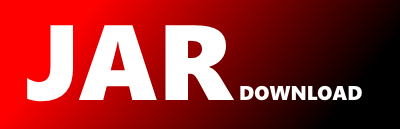
com.github.lontime.extjobrunr.beans.VersionUIModelBean Maven / Gradle / Ivy
package com.github.lontime.extjobrunr.beans;
import java.util.Map;
import org.jobrunr.dashboard.ui.model.VersionUIModel;
import org.joda.beans.Bean;
import org.joda.beans.BeanBuilder;
import org.joda.beans.JodaBeanUtils;
import org.joda.beans.MetaBean;
import org.joda.beans.MetaProperty;
import org.joda.beans.Property;
import org.joda.beans.gen.BeanDefinition;
import org.joda.beans.gen.PropertyDefinition;
import org.joda.beans.impl.direct.DirectBeanBuilder;
import org.joda.beans.impl.direct.DirectMetaBean;
import org.joda.beans.impl.direct.DirectMetaProperty;
import org.joda.beans.impl.direct.DirectMetaPropertyMap;
@BeanDefinition
public class VersionUIModelBean implements Bean {
@PropertyDefinition
private String version;
public VersionUIModelBean() {
}
public VersionUIModelBean(VersionUIModel versionUIModel) {
this.version = versionUIModel.getVersion();
}
//------------------------- AUTOGENERATED START -------------------------
/**
* The meta-bean for {@code VersionUIModelBean}.
* @return the meta-bean, not null
*/
public static VersionUIModelBean.Meta meta() {
return VersionUIModelBean.Meta.INSTANCE;
}
static {
MetaBean.register(VersionUIModelBean.Meta.INSTANCE);
}
@Override
public VersionUIModelBean.Meta metaBean() {
return VersionUIModelBean.Meta.INSTANCE;
}
//-----------------------------------------------------------------------
/**
* Gets the version.
* @return the value of the property
*/
public String getVersion() {
return version;
}
/**
* Sets the version.
* @param version the new value of the property
*/
public void setVersion(String version) {
this.version = version;
}
/**
* Gets the the {@code version} property.
* @return the property, not null
*/
public final Property version() {
return metaBean().version().createProperty(this);
}
//-----------------------------------------------------------------------
@Override
public VersionUIModelBean clone() {
return JodaBeanUtils.cloneAlways(this);
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj != null && obj.getClass() == this.getClass()) {
VersionUIModelBean other = (VersionUIModelBean) obj;
return JodaBeanUtils.equal(getVersion(), other.getVersion());
}
return false;
}
@Override
public int hashCode() {
int hash = getClass().hashCode();
hash = hash * 31 + JodaBeanUtils.hashCode(getVersion());
return hash;
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder(64);
buf.append("VersionUIModelBean{");
int len = buf.length();
toString(buf);
if (buf.length() > len) {
buf.setLength(buf.length() - 2);
}
buf.append('}');
return buf.toString();
}
protected void toString(StringBuilder buf) {
buf.append("version").append('=').append(JodaBeanUtils.toString(getVersion())).append(',').append(' ');
}
//-----------------------------------------------------------------------
/**
* The meta-bean for {@code VersionUIModelBean}.
*/
public static class Meta extends DirectMetaBean {
/**
* The singleton instance of the meta-bean.
*/
static final Meta INSTANCE = new Meta();
/**
* The meta-property for the {@code version} property.
*/
private final MetaProperty version = DirectMetaProperty.ofReadWrite(
this, "version", VersionUIModelBean.class, String.class);
/**
* The meta-properties.
*/
private final Map> metaPropertyMap$ = new DirectMetaPropertyMap(
this, null,
"version");
/**
* Restricted constructor.
*/
protected Meta() {
}
@Override
protected MetaProperty> metaPropertyGet(String propertyName) {
switch (propertyName.hashCode()) {
case 351608024: // version
return version;
}
return super.metaPropertyGet(propertyName);
}
@Override
public BeanBuilder extends VersionUIModelBean> builder() {
return new DirectBeanBuilder<>(new VersionUIModelBean());
}
@Override
public Class extends VersionUIModelBean> beanType() {
return VersionUIModelBean.class;
}
@Override
public Map> metaPropertyMap() {
return metaPropertyMap$;
}
//-----------------------------------------------------------------------
/**
* The meta-property for the {@code version} property.
* @return the meta-property, not null
*/
public final MetaProperty version() {
return version;
}
//-----------------------------------------------------------------------
@Override
protected Object propertyGet(Bean bean, String propertyName, boolean quiet) {
switch (propertyName.hashCode()) {
case 351608024: // version
return ((VersionUIModelBean) bean).getVersion();
}
return super.propertyGet(bean, propertyName, quiet);
}
@Override
protected void propertySet(Bean bean, String propertyName, Object newValue, boolean quiet) {
switch (propertyName.hashCode()) {
case 351608024: // version
((VersionUIModelBean) bean).setVersion((String) newValue);
return;
}
super.propertySet(bean, propertyName, newValue, quiet);
}
}
//-------------------------- AUTOGENERATED END --------------------------
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy