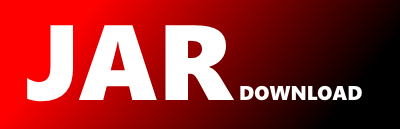
com.github.lontime.extjobrunr.beans.states.EnqueuedStateBean Maven / Gradle / Ivy
package com.github.lontime.extjobrunr.beans.states;
import java.time.Instant;
import java.util.Map;
import org.jobrunr.jobs.states.DeletedState;
import org.jobrunr.jobs.states.EnqueuedState;
import org.jobrunr.jobs.states.JobState;
import org.joda.beans.Bean;
import org.joda.beans.BeanBuilder;
import org.joda.beans.JodaBeanUtils;
import org.joda.beans.MetaBean;
import org.joda.beans.MetaProperty;
import org.joda.beans.Property;
import org.joda.beans.gen.BeanDefinition;
import org.joda.beans.gen.PropertyDefinition;
import org.joda.beans.impl.direct.DirectBeanBuilder;
import org.joda.beans.impl.direct.DirectMetaBean;
import org.joda.beans.impl.direct.DirectMetaProperty;
import org.joda.beans.impl.direct.DirectMetaPropertyMap;
@BeanDefinition
public class EnqueuedStateBean implements Bean, JobStateBean {
@PropertyDefinition
private Instant enqueuedAt;
@PropertyDefinition
private String state;
@PropertyDefinition
private Instant createdAt;
@PropertyDefinition
private Instant updatedAt;
public EnqueuedStateBean() {
}
public EnqueuedStateBean(EnqueuedState enqueuedState) {
this.enqueuedAt = enqueuedState.getEnqueuedAt();
this.state = enqueuedState.getName().name();
this.createdAt = enqueuedState.getCreatedAt();
this.updatedAt = enqueuedState.getCreatedAt();
}
@Override
public JobState toState() {
return new EnqueuedState();
}
//------------------------- AUTOGENERATED START -------------------------
/**
* The meta-bean for {@code EnqueuedStateBean}.
* @return the meta-bean, not null
*/
public static EnqueuedStateBean.Meta meta() {
return EnqueuedStateBean.Meta.INSTANCE;
}
static {
MetaBean.register(EnqueuedStateBean.Meta.INSTANCE);
}
@Override
public EnqueuedStateBean.Meta metaBean() {
return EnqueuedStateBean.Meta.INSTANCE;
}
//-----------------------------------------------------------------------
/**
* Gets the enqueuedAt.
* @return the value of the property
*/
public Instant getEnqueuedAt() {
return enqueuedAt;
}
/**
* Sets the enqueuedAt.
* @param enqueuedAt the new value of the property
*/
public void setEnqueuedAt(Instant enqueuedAt) {
this.enqueuedAt = enqueuedAt;
}
/**
* Gets the the {@code enqueuedAt} property.
* @return the property, not null
*/
public final Property enqueuedAt() {
return metaBean().enqueuedAt().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the state.
* @return the value of the property
*/
public String getState() {
return state;
}
/**
* Sets the state.
* @param state the new value of the property
*/
public void setState(String state) {
this.state = state;
}
/**
* Gets the the {@code state} property.
* @return the property, not null
*/
public final Property state() {
return metaBean().state().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the createdAt.
* @return the value of the property
*/
public Instant getCreatedAt() {
return createdAt;
}
/**
* Sets the createdAt.
* @param createdAt the new value of the property
*/
public void setCreatedAt(Instant createdAt) {
this.createdAt = createdAt;
}
/**
* Gets the the {@code createdAt} property.
* @return the property, not null
*/
public final Property createdAt() {
return metaBean().createdAt().createProperty(this);
}
//-----------------------------------------------------------------------
/**
* Gets the updatedAt.
* @return the value of the property
*/
public Instant getUpdatedAt() {
return updatedAt;
}
/**
* Sets the updatedAt.
* @param updatedAt the new value of the property
*/
public void setUpdatedAt(Instant updatedAt) {
this.updatedAt = updatedAt;
}
/**
* Gets the the {@code updatedAt} property.
* @return the property, not null
*/
public final Property updatedAt() {
return metaBean().updatedAt().createProperty(this);
}
//-----------------------------------------------------------------------
@Override
public EnqueuedStateBean clone() {
return JodaBeanUtils.cloneAlways(this);
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj != null && obj.getClass() == this.getClass()) {
EnqueuedStateBean other = (EnqueuedStateBean) obj;
return JodaBeanUtils.equal(getEnqueuedAt(), other.getEnqueuedAt()) &&
JodaBeanUtils.equal(getState(), other.getState()) &&
JodaBeanUtils.equal(getCreatedAt(), other.getCreatedAt()) &&
JodaBeanUtils.equal(getUpdatedAt(), other.getUpdatedAt());
}
return false;
}
@Override
public int hashCode() {
int hash = getClass().hashCode();
hash = hash * 31 + JodaBeanUtils.hashCode(getEnqueuedAt());
hash = hash * 31 + JodaBeanUtils.hashCode(getState());
hash = hash * 31 + JodaBeanUtils.hashCode(getCreatedAt());
hash = hash * 31 + JodaBeanUtils.hashCode(getUpdatedAt());
return hash;
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder(160);
buf.append("EnqueuedStateBean{");
int len = buf.length();
toString(buf);
if (buf.length() > len) {
buf.setLength(buf.length() - 2);
}
buf.append('}');
return buf.toString();
}
protected void toString(StringBuilder buf) {
buf.append("enqueuedAt").append('=').append(JodaBeanUtils.toString(getEnqueuedAt())).append(',').append(' ');
buf.append("state").append('=').append(JodaBeanUtils.toString(getState())).append(',').append(' ');
buf.append("createdAt").append('=').append(JodaBeanUtils.toString(getCreatedAt())).append(',').append(' ');
buf.append("updatedAt").append('=').append(JodaBeanUtils.toString(getUpdatedAt())).append(',').append(' ');
}
//-----------------------------------------------------------------------
/**
* The meta-bean for {@code EnqueuedStateBean}.
*/
public static class Meta extends DirectMetaBean {
/**
* The singleton instance of the meta-bean.
*/
static final Meta INSTANCE = new Meta();
/**
* The meta-property for the {@code enqueuedAt} property.
*/
private final MetaProperty enqueuedAt = DirectMetaProperty.ofReadWrite(
this, "enqueuedAt", EnqueuedStateBean.class, Instant.class);
/**
* The meta-property for the {@code state} property.
*/
private final MetaProperty state = DirectMetaProperty.ofReadWrite(
this, "state", EnqueuedStateBean.class, String.class);
/**
* The meta-property for the {@code createdAt} property.
*/
private final MetaProperty createdAt = DirectMetaProperty.ofReadWrite(
this, "createdAt", EnqueuedStateBean.class, Instant.class);
/**
* The meta-property for the {@code updatedAt} property.
*/
private final MetaProperty updatedAt = DirectMetaProperty.ofReadWrite(
this, "updatedAt", EnqueuedStateBean.class, Instant.class);
/**
* The meta-properties.
*/
private final Map> metaPropertyMap$ = new DirectMetaPropertyMap(
this, null,
"enqueuedAt",
"state",
"createdAt",
"updatedAt");
/**
* Restricted constructor.
*/
protected Meta() {
}
@Override
protected MetaProperty> metaPropertyGet(String propertyName) {
switch (propertyName.hashCode()) {
case -788998993: // enqueuedAt
return enqueuedAt;
case 109757585: // state
return state;
case 598371643: // createdAt
return createdAt;
case -1949194674: // updatedAt
return updatedAt;
}
return super.metaPropertyGet(propertyName);
}
@Override
public BeanBuilder extends EnqueuedStateBean> builder() {
return new DirectBeanBuilder<>(new EnqueuedStateBean());
}
@Override
public Class extends EnqueuedStateBean> beanType() {
return EnqueuedStateBean.class;
}
@Override
public Map> metaPropertyMap() {
return metaPropertyMap$;
}
//-----------------------------------------------------------------------
/**
* The meta-property for the {@code enqueuedAt} property.
* @return the meta-property, not null
*/
public final MetaProperty enqueuedAt() {
return enqueuedAt;
}
/**
* The meta-property for the {@code state} property.
* @return the meta-property, not null
*/
public final MetaProperty state() {
return state;
}
/**
* The meta-property for the {@code createdAt} property.
* @return the meta-property, not null
*/
public final MetaProperty createdAt() {
return createdAt;
}
/**
* The meta-property for the {@code updatedAt} property.
* @return the meta-property, not null
*/
public final MetaProperty updatedAt() {
return updatedAt;
}
//-----------------------------------------------------------------------
@Override
protected Object propertyGet(Bean bean, String propertyName, boolean quiet) {
switch (propertyName.hashCode()) {
case -788998993: // enqueuedAt
return ((EnqueuedStateBean) bean).getEnqueuedAt();
case 109757585: // state
return ((EnqueuedStateBean) bean).getState();
case 598371643: // createdAt
return ((EnqueuedStateBean) bean).getCreatedAt();
case -1949194674: // updatedAt
return ((EnqueuedStateBean) bean).getUpdatedAt();
}
return super.propertyGet(bean, propertyName, quiet);
}
@Override
protected void propertySet(Bean bean, String propertyName, Object newValue, boolean quiet) {
switch (propertyName.hashCode()) {
case -788998993: // enqueuedAt
((EnqueuedStateBean) bean).setEnqueuedAt((Instant) newValue);
return;
case 109757585: // state
((EnqueuedStateBean) bean).setState((String) newValue);
return;
case 598371643: // createdAt
((EnqueuedStateBean) bean).setCreatedAt((Instant) newValue);
return;
case -1949194674: // updatedAt
((EnqueuedStateBean) bean).setUpdatedAt((Instant) newValue);
return;
}
super.propertySet(bean, propertyName, newValue, quiet);
}
}
//-------------------------- AUTOGENERATED END --------------------------
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy