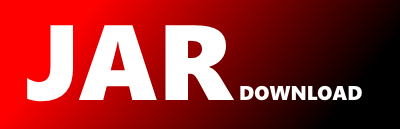
com.github.lontime.extkafka.configuration.OptionResolver Maven / Gradle / Ivy
package com.github.lontime.extkafka.configuration;
import java.time.Duration;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import java.util.function.Supplier;
import com.github.lontime.base.commonj.utils.SupplierHelper;
import com.github.lontime.extconfig.ConfigHelper;
import com.github.lontime.extconfig.WrapperConfig;
import com.github.lontime.extkafka.common.CommitKind;
import com.github.lontime.shaded.io.helidon.config.Config;
/**
* Redisson Option Resolver.
*
* @author lontime
* @since 1.0
*/
public class OptionResolver {
private static final String NAME = "kafka";
//------------------singleton------------------
/**
* supplier.
*/
private static Supplier supplier = SupplierHelper.memoize(OptionResolver::new);
public static OptionResolver getInstance() {
return supplier.get();
}
//------------------singleton------------------
private List producerOptions;
private List consumerOptions;
public OptionResolver() {
final Config config = ConfigHelper.resolve(NAME);
final List specs = config.get("specs").asList(new SpecMapper()).orElse(Collections.emptyList());
this.producerOptions = config.get("producers").asList(new ProducerMapper(specs)).orElse(Collections.emptyList());
this.consumerOptions = config.get("consumers").asList(new ConsumerMapper(specs)).orElse(Collections.emptyList());
}
/**
* getProducers.
*
* @return Map
*/
public List getProducers() {
return this.producerOptions;
}
/**
* getProducers.
*
* @return Map
*/
public List getConsumers() {
return this.consumerOptions;
}
static class SpecMapper implements Function {
@Override
public SpecOption apply(Config config) {
final WrapperConfig wrapperConfig = new WrapperConfig(config);
final SpecOption option = new SpecOption();
option.setName(config.name());
wrapperConfig.getAsMap().ifPresent(option::setSpecs);
return option;
}
}
static class ConsumerMapper extends SpecResolver implements Function {
public ConsumerMapper(List specs) {
super(specs);
}
@Override
public ConsumerOption apply(Config config) {
final WrapperConfig wrapperConfig = new WrapperConfig(config);
final ConsumerOption option = new ConsumerOption();
final List defaultTopics = Collections.singletonList(config.name());
final String defaultGroupId = config.name();
option.setName(config.name());
option.setTopics(config.get("topics").asList(String.class).orElse(defaultTopics));
option.setGroupId(config.get("groupId").asString().orElse(defaultGroupId));
wrapperConfig.getAsDuration("pullTimeout").ifPresent(option::setPullTimeout);
config.get("commitKind").asString().as(String::toUpperCase).map(CommitKind::valueOf).ifPresent(option::setCommitKind);
config.get("syncCommitTimeout").asString().map(Duration::parse).ifPresent(option::setSyncCommitTimeout);
config.get("interval").asString().map(Duration::parse).ifPresent(option::setInterval);
config.get("intervalMax").asString().map(Duration::parse).ifPresent(option::setIntervalMax);
config.get("warmup").asString().map(Duration::parse).ifPresent(option::setWarmup);
config.get("enableAutoCommit").asBoolean().ifPresent(option::setEnableAutoCommit);
config.get("autoCommitInterval").asString().map(Duration::parse).ifPresent(option::setIntervalMax);
option.setSpecs(resolve(config));
return option;
}
}
static class ProducerMapper extends SpecResolver implements Function {
public ProducerMapper(List specs) {
super(specs);
}
@Override
public ProducerOption apply(Config config) {
final ProducerOption option = new ProducerOption();
final String topic = config.get("topic").asString().orElse(config.name());
option.setName(config.name());
option.setTopic(topic);
config.get("batchSize").asInt().ifPresent(option::setBatchSize);
config.get("partition").asInt().ifPresent(option::setPartition);
option.setSpecs(resolve(config));
return option;
}
}
static class SpecResolver {
private List specs;
public SpecResolver(List specs) {
this.specs = specs;
}
protected Map resolve(Config config) {
final Map mapNew = new HashMap<>();
final WrapperConfig wrapperConfig = new WrapperConfig(config);
wrapperConfig.getAsString("specName")
.flatMap(value -> specs.stream()
.filter(s -> value.equals(s.getName()) && s.getSpecs() != null)
.findFirst()
.map(SpecOption::getSpecs))
.ifPresent(mapNew::putAll);
wrapperConfig.getAsMap("specs").ifPresent(mapNew::putAll);
return mapNew;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy