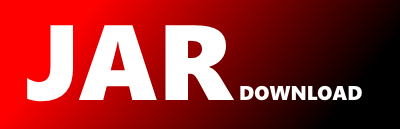
com.github.lontime.extpac4j.impl.BasicUserProfileReader Maven / Gradle / Ivy
The newest version!
package com.github.lontime.extpac4j.impl;
import java.io.IOException;
import java.util.List;
import java.util.Map;
import com.github.lontime.extjson.shaded.net.minidev.json.JSONValue;
import com.github.lontime.extjson.shaded.net.minidev.json.parser.ParseException;
import com.github.lontime.extjson.shaded.net.minidev.json.writer.JsonReaderI;
import org.pac4j.core.profile.BasicUserProfile;
/**
* BasicUserProfileReader.
* @author lontime
* @see BasicUserProfile
* @since 1.0
*/
public class BasicUserProfileReader extends JsonReaderI {
public BasicUserProfileReader() {
super(JSONValue.defaultReader);
}
@Override
public void setValue(Object current, String key, Object value) throws ParseException, IOException {
if ("id".equals(key)) {
((BasicUserProfile) current).setId((String) value);
return;
}
if ("attributes".equals(key)) {
final Map objectValue = (Map) value;
for (Map.Entry entry : objectValue.entrySet()) {
((BasicUserProfile) current).addAttribute(entry.getKey(), entry.getValue());
}
return;
}
if ("authenticationAttributes".equals(key)) {
final Map objectValue = (Map) value;
for (Map.Entry entry : objectValue.entrySet()) {
((BasicUserProfile) current).addAuthenticationAttribute(entry.getKey(), entry.getValue());
}
return;
}
if ("isRemembered".equals(key)) {
((BasicUserProfile) current).setRemembered((Boolean) value);
return;
}
if ("roles".equals(key)) {
for (String k : (List) value) {
((BasicUserProfile) current).addRole(k);
}
return;
}
if ("permissions".equals(key)) {
for (String k : (List) value) {
((BasicUserProfile) current).addPermission(k);
}
return;
}
if ("clientName".equals(key)) {
((BasicUserProfile) current).setClientName((String) value);
return;
}
if ("linkedId".equals(key)) {
((BasicUserProfile) current).setLinkedId((String) value);
return;
}
}
@Override
public JsonReaderI> startObject(String key) throws ParseException, IOException {
return JSONValue.defaultReader.getMapper(Map.class);
}
@Override
public JsonReaderI> startArray(String key) throws ParseException, IOException {
return JSONValue.defaultReader.getMapper(List.class);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy