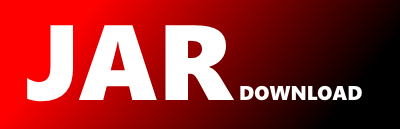
com.github.lontime.extpac4j.impl.HeaderSimpleSessionStore Maven / Gradle / Ivy
The newest version!
package com.github.lontime.extpac4j.impl;
import java.time.Duration;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.github.lontime.base.commonj.utils.UuidHelper;
import com.github.lontime.shaded.com.github.benmanes.caffeine.cache.Cache;
import com.github.lontime.shaded.com.github.benmanes.caffeine.cache.Caffeine;
import org.pac4j.core.context.JEEContext;
import org.pac4j.core.context.session.SessionStore;
/**
* LocalJEESessionStore.
* @author lontime
* @since 1.0
*/
public class HeaderSimpleSessionStore implements SessionStore {
public static final HeaderSimpleSessionStore INSTANCE = new HeaderSimpleSessionStore();
private static final int MAP_SIZE = 16;
public static final String DEFAULT_HEADER_NAME = "X-Token";
protected Cache> cache;
protected final Logger logger = LoggerFactory.getLogger(getClass());
protected String headerName;
public HeaderSimpleSessionStore(String headerName, Duration ttl) {
this.headerName = headerName == null ? DEFAULT_HEADER_NAME : headerName;
this.cache = Caffeine.newBuilder().expireAfterAccess(ttl).build();
}
public HeaderSimpleSessionStore(String headerName) {
this(headerName, Duration.ofMinutes(5));
}
public HeaderSimpleSessionStore() {
this(null);
}
protected boolean check(JEEContext context) {
return false;
}
@Override
public String getOrCreateSessionId(JEEContext context) {
final Optional sessionIdOptional = getSessionId(context);
if (sessionIdOptional.isPresent()) {
return sessionIdOptional.get();
}
final String sessionId = UuidHelper.fastUUID();
context.setRequestAttribute(headerName, sessionId);
return sessionId;
}
@Override
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy