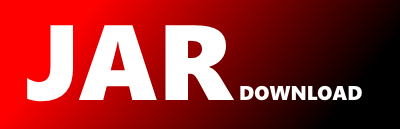
com.github.lpandzic.junit.bdd.Then Maven / Gradle / Ivy
package com.github.lpandzic.junit.bdd;
import java.util.Optional;
import java.util.function.Consumer;
/**
* Defines expected outcomes produced by {@link When}.
*
* @author Lovro Pandzic
*/
public final class Then {
/**
* Used to describe expected thrown exception.
*
* @param type of expected {@link Throwable}
*/
public static final class Throws {
private final Bdd bdd;
public Throws(Bdd bdd) {
this.bdd = bdd;
}
public void then(Consumer consumer) {
Optional throwable = bdd.takeThrownException();
consumer.accept(throwable.orElse(null));
}
@SuppressWarnings("unchecked")
public void thenChecked(Consumer consumer) {
Optional throwable = bdd.takeThrownException();
try {
consumer.accept((E) throwable.orElse(null));
} catch (ClassCastException e) {
bdd.throwUnexpectedException(throwable.get());
}
}
}
/**
* Used to describe expected returned value.
*
* @param type of returned value
*/
public static final class Returns {
private final Optional value;
public Returns(Optional value) {
this.value = value;
}
public void then(Consumer consumer) {
consumer.accept(value.orElse(null));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy