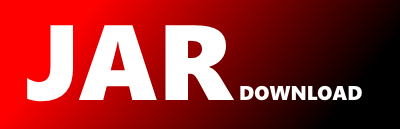
com.github.lsqlebai.annotation.ReflectHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of server-common Show documentation
Show all versions of server-common Show documentation
An kotlin server common jar
package com.github.lsqlebai.annotation;
import java.lang.reflect.*;
import java.util.HashMap;
import java.util.List;
public class ReflectHelper {
static HashMap> sfiledCacheHashMap = new HashMap>();
static HashMap> sfiledStaticCacheHashMap = new HashMap>();
public static String getStaticIntFieldName(Class cls, int value) {
HashMap fieldHashMap = ReflectHelper.getStaticFields(cls);
for (String key : fieldHashMap.keySet()) {
Field field = fieldHashMap.get(key);
try {
int fieldValue = field.getInt(cls);
if (fieldValue == value) {
return key;
}
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (IllegalArgumentException e) {
}
}
return "can't find" + value;
}
static class CacheKey {
Class> cls;
public CacheKey(Class> cls) {
this.cls = cls;
}
@Override
public int hashCode() {
return (cls.getClass().getName()).hashCode();
}
@Override
public boolean equals(Object o) {
if (o instanceof CacheKey) {
CacheKey that = (CacheKey) o;
if (this == o) {
return true;
}
if (that.cls == null || this.cls == null) {
return false;
}
if (this.cls.getName().equals(that.cls.getName())) {
return true;
}
}
return false;
}
}
public static HashMap getFields(Object object) {
Class> cls = object.getClass();
CacheKey cacheKey = new CacheKey(cls);
if (sfiledCacheHashMap.containsKey(cacheKey)) {
return sfiledCacheHashMap.get(cacheKey);
}
HashMap retFields = new HashMap();
loopGetFields(cls, retFields);
sfiledCacheHashMap.put(cacheKey, retFields);
return retFields;
}
private static void loopGetFields(Class> cls, HashMap retFields) {
while (cls != Object.class) {
Field[] fields = cls.getDeclaredFields();
for (Field field : fields) {
try {
if ((field.getModifiers() & Modifier.STATIC) != Modifier.STATIC) {
field.setAccessible(true);
retFields.put(field.getName(), field);
}
} catch (IllegalArgumentException e) {
e.printStackTrace();
}
}
cls = cls.getSuperclass();
}
}
public static HashMap getStaticFields(Class> cls) {
CacheKey cacheKey = new CacheKey(cls);
if (sfiledStaticCacheHashMap.containsKey(cacheKey)) {
return sfiledStaticCacheHashMap.get(cacheKey);
}
HashMap retFields = new HashMap();
while (cls != Object.class) {
Field[] fields = cls.getDeclaredFields();
for (Field field : fields) {
try {
if ((field.getModifiers() & Modifier.STATIC) == Modifier.STATIC) {
field.setAccessible(true);
retFields.put(field.getName(), field);
}
} catch (IllegalArgumentException e) {
e.printStackTrace();
}
}
cls = cls.getSuperclass();
}
sfiledStaticCacheHashMap.put(cacheKey, retFields);
return retFields;
}
public static HashMap getFields(Class> cls) {
CacheKey cacheKey = new CacheKey(cls);
if (sfiledCacheHashMap.containsKey(cacheKey)) {
return sfiledCacheHashMap.get(cacheKey);
}
HashMap retFields = new HashMap();
loopGetFields(cls, retFields);
sfiledCacheHashMap.put(cacheKey, retFields);
return retFields;
}
public static T newInstance(Class cls) {
Constructor cons[] = cls.getDeclaredConstructors();
if (cons.length > 0) {
cons[0].setAccessible(true);
try {
return (T) cons[0].newInstance();
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (InvocationTargetException e) {
e.printStackTrace();
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
return null;
}
public static T newInstance(Class cls, Object... args) {
Constructor cons[] = cls.getDeclaredConstructors();
if (cons.length > 0) {
cons[0].setAccessible(true);
try {
return (T) cons[0].newInstance(args);
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (InvocationTargetException e) {
e.printStackTrace();
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
return null;
}
public static Object invoke(Class> cls, String methodName) {
try {
Method method = cls.getMethod(methodName);
return method.invoke(cls);
} catch (NoSuchMethodException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IllegalAccessException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IllegalArgumentException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (InvocationTargetException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
public static Object invoke(Object instance, String methodName) {
try {
Method method = instance.getClass().getMethod(methodName);
return method.invoke(instance);
} catch (NoSuchMethodException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IllegalAccessException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IllegalArgumentException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (InvocationTargetException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
public static Object invoke(Object instance, Method method) {
try {
return method.invoke(instance);
} catch (IllegalAccessException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IllegalArgumentException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (InvocationTargetException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
public static Object invokeByArg(Object instance, String methodName, Object... arg) {
try {
Class[] argCls = new Class[arg.length];
for (int i = 0; i < arg.length; i++) {
argCls[i] = arg[i].getClass();
}
Method method = instance.getClass().getMethod(methodName, argCls);
//如果是private修饰符的,则把可访问性设置为true
if (!method.isAccessible()) {
method.setAccessible(true);
}
return method.invoke(instance, arg);
} catch (NoSuchMethodException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IllegalArgumentException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (InvocationTargetException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
public static Object invokeByType(Object instance, String methodName, Object... args) {
try {
Class[] cls = new Class[args.length];
for (int i = 0; i < args.length; i++) {
cls[i] = args[i].getClass();
}
Method method = instance.getClass().getMethod(methodName, cls);
if (!method.isAccessible()) {
method.setAccessible(true);
}
return method.invoke(instance, args);
} catch (NoSuchMethodException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IllegalArgumentException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (InvocationTargetException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
public static Object invoke(Object instance, String methodName, Object... arg) {
try {
Method[] obj = instance.getClass().getMethods();
for (Method method : obj) {
if (methodName != null && methodName.equals(method.getName())) {
if (!method.isAccessible()) {
method.setAccessible(true);
}
Class>[] parameterClazz = method.getParameterTypes();
return method.invoke(instance, arg);
}
}
} catch (IllegalAccessException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IllegalArgumentException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (InvocationTargetException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
public static Method[] getMethods(Class> cls) {
return cls.getMethods();
}
private static void fillList(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy