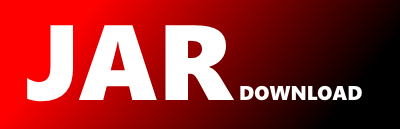
com.github.lsqlebai.utils.DateUtils.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of server-common Show documentation
Show all versions of server-common Show documentation
An kotlin server common jar
package com.github.lsqlebai.utils
import java.text.SimpleDateFormat
import java.util.*
import kotlin.concurrent.getOrSet
object DateUtils {
var shortFormat: ThreadLocal = ThreadLocal()
var normalFormat: ThreadLocal = ThreadLocal()
fun getCurrentZeroDay(): Date {
val calendar = Calendar.getInstance()
calendar.time = Date()
calendar.set(Calendar.HOUR_OF_DAY, 0)
calendar.set(Calendar.MINUTE, 0)
calendar.set(Calendar.SECOND, 0)
calendar.set(Calendar.MILLISECOND, 0)
return calendar.time
}
fun getYesterdayZero(): Date {
val calendar = Calendar.getInstance()
calendar.time = Date()
calendar.set(Calendar.HOUR_OF_DAY, 0)
calendar.set(Calendar.MINUTE, 0)
calendar.set(Calendar.SECOND, 0)
calendar.set(Calendar.MILLISECOND, 0)
calendar.add(Calendar.DATE, -1)
return calendar.time
}
fun getYesterday(): Date {
val calendar = Calendar.getInstance()
calendar.add(Calendar.DATE, -1)
return calendar.time
}
fun getYesterdayStr(): String {
return shortFormat.getOrSet { SimpleDateFormat("yyyyMMdd") }.format(getYesterday())
}
fun getTodayStr(): String {
return normalFormat.getOrSet { SimpleDateFormat("yyyy-MM-dd") }.format(Date())
}
fun getDateStr(date: Date): String {
return normalFormat.getOrSet { SimpleDateFormat("yyyy-MM-dd") }.format(date)
}
fun getSimpleDateStr(date: Date): String {
return shortFormat.getOrSet { SimpleDateFormat("yyyyMMdd") }.format(date)
}
fun createBySimpleDate(date: String): Date {
return normalFormat.getOrSet { SimpleDateFormat("yyyy-MM-dd") }.parse(date)
}
fun createByShortDate(date: String): Date {
return shortFormat.getOrSet { SimpleDateFormat("yyyyMMdd") }.parse(date)
}
}
fun main(args: Array) {
DateUtils.getTodayStr()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy