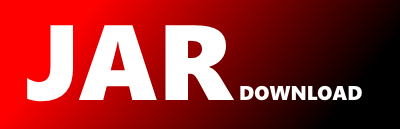
com.github.ltsopensource.autoconfigure.PropertiesConfigurationFactory Maven / Gradle / Ivy
package com.github.ltsopensource.autoconfigure;
import com.github.ltsopensource.autoconfigure.annotation.ConfigurationProperties;
import com.github.ltsopensource.autoconfigure.resolver.Resolver;
import com.github.ltsopensource.autoconfigure.resolver.ResolverUtils;
import com.github.ltsopensource.core.commons.utils.CollectionUtils;
import java.beans.PropertyDescriptor;
import java.io.IOException;
import java.util.*;
/**
* @author Robert HG ([email protected]) on 4/18/16.
*/
public class PropertiesConfigurationFactory {
public static T createPropertiesConfiguration(Class clazz, Map propMap) {
if (clazz == null) {
throw new IllegalArgumentException("clazz should not be null");
}
if (!clazz.isAnnotationPresent(ConfigurationProperties.class)) {
throw new IllegalArgumentException(clazz.getName() + " must annotation with @" + ConfigurationProperties.class.getName());
}
ConfigurationProperties annotation = clazz.getAnnotation(ConfigurationProperties.class);
String prefix = annotation.prefix();
return createPropertiesConfiguration(clazz, prefix, propMap);
}
public static T createPropertiesConfiguration(Class clazz, String prefix, Map propMap) {
T targetObj;
try {
targetObj = clazz.newInstance();
} catch (Exception e) {
throw new IllegalStateException(clazz.getName() + " instance error", e);
}
AutoConfigContext configContext = new AutoConfigContextBuilder()
.setPrefix(prefix)
.setPropMap(propMap)
.setTargetObj(targetObj)
.build();
Set includeNames = new HashSet();
if (CollectionUtils.isNotEmpty(configContext.getNameDescriptorMap())) {
for (String key : propMap.keySet()) {
for (String name : configContext.getNameDescriptorMap().keySet()) {
if (key.startsWith(name)) {
includeNames.add(name);
}
}
}
}
for (String includeName : includeNames) {
PropertyDescriptor descriptor = configContext.getNameDescriptorMap().get(includeName);
Class> propertyType = descriptor.getPropertyType();
Resolver resolver = ResolverUtils.getResolver(propertyType);
if (resolver != null) {
resolver.resolve(configContext, descriptor, propertyType);
} else {
throw new PropertiesConfigurationResolveException("Can not find Resolver for type:" + propertyType.getName());
}
}
return targetObj;
}
public static T createPropertiesConfiguration(Class clazz) {
if (clazz == null) {
throw new IllegalArgumentException("clazz should not be null");
}
if (!clazz.isAnnotationPresent(ConfigurationProperties.class)) {
throw new IllegalArgumentException(clazz.getName() + " must annotation with @" + ConfigurationProperties.class.getName());
}
ConfigurationProperties annotation = clazz.getAnnotation(ConfigurationProperties.class);
String[] locations = annotation.locations();
return createPropertiesConfiguration(clazz, locations);
}
public static T createPropertiesConfiguration(Class clazz, String[] locations) {
if (clazz == null) {
throw new IllegalArgumentException("clazz should not be null");
}
if (!clazz.isAnnotationPresent(ConfigurationProperties.class)) {
throw new IllegalArgumentException(clazz.getName() + " must annotation with @" + ConfigurationProperties.class.getName());
}
if (locations == null || locations.length == 0) {
throw new IllegalArgumentException(clazz.getName() + " must specified the properties locations");
}
Properties properties = new Properties();
for (String location : locations) {
try {
properties.load(PropertiesConfigurationFactory.class.getClassLoader().getResourceAsStream(location.trim()));
} catch (IOException e) {
throw new IllegalStateException("Load properties [" + location + "] error", e);
}
}
Map map = new HashMap(properties.size());
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy