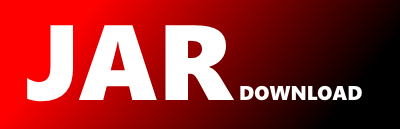
com.github.ltsopensource.biz.logger.mongo.MongoJobLogger Maven / Gradle / Ivy
package com.github.ltsopensource.biz.logger.mongo;
import com.github.ltsopensource.biz.logger.JobLogger;
import com.github.ltsopensource.biz.logger.domain.JobLogPo;
import com.github.ltsopensource.biz.logger.domain.JobLoggerRequest;
import com.github.ltsopensource.core.cluster.Config;
import com.github.ltsopensource.core.commons.utils.CollectionUtils;
import com.github.ltsopensource.core.commons.utils.StringUtils;
import com.github.ltsopensource.admin.response.PaginationRsp;
import com.github.ltsopensource.store.mongo.MongoRepository;
import com.mongodb.DBCollection;
import com.mongodb.DBObject;
import org.mongodb.morphia.query.Query;
import java.util.Date;
import java.util.List;
/**
* @author Robert HG ([email protected]) on 3/27/15.
*/
public class MongoJobLogger extends MongoRepository implements JobLogger {
public MongoJobLogger(Config config) {
super(config);
setTableName("lts_job_log_po");
// create table
DBCollection dbCollection = template.getCollection();
List indexInfo = dbCollection.getIndexInfo();
// create index if not exist
if (CollectionUtils.sizeOf(indexInfo) <= 1) {
template.ensureIndex("idx_logTime", "logTime");
template.ensureIndex("idx_taskId_taskTrackerNodeGroup", "taskId,taskTrackerNodeGroup");
template.ensureIndex("idx_realTaskId_taskTrackerNodeGroup", "realTaskId, taskTrackerNodeGroup");
}
}
@Override
public void log(JobLogPo jobLogPo) {
template.save(jobLogPo);
}
@Override
public void log(List jobLogPos) {
template.save(jobLogPos);
}
@Override
public PaginationRsp search(JobLoggerRequest request) {
Query query = template.createQuery(JobLogPo.class);
if(StringUtils.isNotEmpty(request.getTaskId())){
query.field("taskId").equal(request.getTaskId());
}
if(StringUtils.isNotEmpty(request.getTaskTrackerNodeGroup())){
query.field("taskTrackerNodeGroup").equal(request.getTaskTrackerNodeGroup());
}
if (request.getStartLogTime() != null) {
query.filter("logTime >= ", getTimestamp(request.getStartLogTime()));
}
if (request.getEndLogTime() != null) {
query.filter("logTime <= ", getTimestamp(request.getEndLogTime()));
}
PaginationRsp paginationRsp = new PaginationRsp();
Long results = template.getCount(query);
paginationRsp.setResults(results.intValue());
if (results == 0) {
return paginationRsp;
}
// 查询rows
query.order("-logTime").offset(request.getStart()).limit(request.getLimit());
paginationRsp.setRows(query.asList());
return paginationRsp;
}
private Long getTimestamp(Date timestamp) {
if (timestamp == null) {
return null;
}
return timestamp.getTime();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy