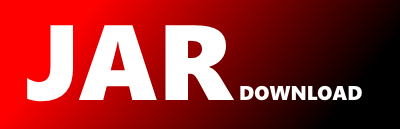
com.github.ltsopensource.cmd.HttpCmdExecutor Maven / Gradle / Ivy
package com.github.ltsopensource.cmd;
import com.github.ltsopensource.core.commons.utils.Assert;
import com.github.ltsopensource.core.commons.utils.DateUtils;
import com.github.ltsopensource.core.json.JSON;
import com.github.ltsopensource.core.logger.Logger;
import com.github.ltsopensource.core.logger.LoggerFactory;
import java.io.*;
import java.net.Socket;
import java.net.URLDecoder;
import java.util.*;
/**
* @author Robert HG ([email protected]) on 2/17/16.
*/
public class HttpCmdExecutor implements Runnable {
private static final Logger LOGGER = LoggerFactory.getLogger(HttpCmdExecutor.class);
private HttpCmdContext context;
private Socket socket;
public HttpCmdExecutor(HttpCmdContext context, Socket socket) {
this.context = context;
this.socket = socket;
}
@Override
public void run() {
try {
// 解析请求
HttpCmdRequest request = parseRequest();
Assert.notNull(request, "Request Error");
Assert.hasText(request.getCommand(), "Command is blank");
Assert.hasText(request.getNodeIdentity(), "nodeIdentity is blank");
HttpCmdProc httpCmdProc = context.getCmdProcessor(request.getNodeIdentity(), request.getCommand());
Assert.notNull(httpCmdProc, "Can not find the command:[" + request.getCommand() + "]");
sendResponse(HTTP_OK, JSON.toJSONString(httpCmdProc.execute(request)));
} catch (HttpCMDErrorException ignored) {
// 忽略
} catch (IllegalArgumentException e) {
sendError(HTTP_BADREQUEST, JSON.toJSONString(HttpCmdResponse.newResponse(false, e.getMessage())), false);
} catch (Throwable t) {
LOGGER.error("Error When Execute Command", t);
sendError(HTTP_INTERNALERROR, JSON.toJSONString(HttpCmdResponse.newResponse(false, "Error:" + t.getMessage())), false);
}
}
private HttpCmdRequest parseRequest() throws Exception {
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream(), "UTF-8"));
StringTokenizer st = new StringTokenizer(in.readLine());
if (!st.hasMoreTokens())
sendError(HTTP_BADREQUEST, "BAD REQUEST: Syntax error");
String method = st.nextToken();
if (!st.hasMoreTokens())
sendError(HTTP_BADREQUEST, "BAD REQUEST: Missing URI");
String uri = st.nextToken();
Properties params = new Properties();
assert uri != null;
int qmi = uri.indexOf('?');
if (qmi >= 0) {
decodeParams(uri.substring(qmi + 1), params);
uri = uri.substring(0, qmi);
}
Properties header = new Properties();
if (st.hasMoreTokens()) {
String line = in.readLine();
while (line.trim().length() > 0) {
int p = line.indexOf(':');
header.put(line.substring(0, p).trim().toLowerCase(), line.substring(p + 1).trim());
line = in.readLine();
}
}
if (method.equalsIgnoreCase("POST")) {
long size = 0x7FFFFFFFFFFFFFFFL;
String contentLength = header.getProperty("Content-Length");
if (contentLength == null) {
contentLength = header.getProperty("content-length");
}
if (contentLength != null) {
size = Integer.parseInt(contentLength);
}
String postLine = "";
char buf[] = new char[512];
int read = in.read(buf);
while (read >= 0 && size > 0 && !postLine.endsWith("\r\n")) {
size -= read;
postLine += String.valueOf(buf, 0, read);
if (size > 0)
read = in.read(buf);
}
postLine = postLine.trim();
decodeParams(postLine, params);
}
return resolveRequest(uri, params);
}
protected static HttpCmdRequest resolveRequest(String uri, Properties params) {
HttpCmdRequest request = new HttpCmdRequest();
String[] pathNode = uri.substring(1, uri.length()).split("/");
String nodeIdentity = pathNode[0];
String command = pathNode[1];
;
request.setCommand(command);
request.setNodeIdentity(nodeIdentity);
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy