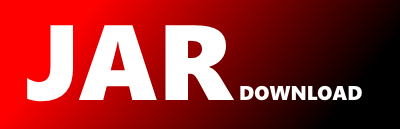
com.github.lucapino.confluence.macro.CodeBlockMacro Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of confluence-maven-plugin Show documentation
Show all versions of confluence-maven-plugin Show documentation
Maven plugin for accessing Atlassian Confluence
The newest version!
/*
* Copyright 2013 Luca Tagliani
* Copyright 2015 Jonathon Hope
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.lucapino.confluence.macro;
import com.github.lucapino.confluence.util.StringUtils;
import java.util.EnumMap;
import java.util.Map;
/**
* Represents a confluence Code Block Macro.
*
* @author Jonathon Hope
* @see
* Confluence Page describing the Macro
*/
public class CodeBlockMacro {
/**
* Stores the parameters of this code block macro.
*/
private final EnumMap parameters;
/**
* The code of this CodeBlockMacro.
*/
private final String body;
/**
* Parameters are options that you can set to control the content or format
* of
* the macro output.
*/
public enum Parameters {
/**
* Can be either {@literal true} or {@literal false}
* Default: false
*/
COLLAPSE,
/**
* When Show line numbers is selected, this value defines the number of
* the
* first line of code.
* Default: 1
*/
FIRSTlINE,
/**
* Specifies the language (or environment) for syntax highlighting. The
* default
* language is Java but you can choose from:
* {@link Languages}
*
* @see Languages
*/
LANGUAGE,
/**
* If selected, line numbers will be shown to the left of the lines of
* code.
* Default: false
*/
LINENUMBERS,
/**
* Specifies the colour scheme used for displaying your code block.
* Many of these themes are based on the default colour schemes of
* popular
* integrated development environments (IDEs). The default theme is
* Confluence (also known as Default), which is typically black and
* coloured
* text on a blank background.
* However, you can also choose from one of the following other
* popular themes:
*
* @see CodeBlockMacro.Themes
*/
THEME,
/**
* Adds a title to the code block. If specified, the title will be
* displayed in a header row at the top
* of the code block.
* Default: none
*/
TITLE;
@Override
public String toString() {
// replace the underscore with a slash and print as lower case
return name().replace("_", "/").toLowerCase();
}
}
/**
* The enumerated list of languages supported by the confluence code macro.
*/
public enum Languages {
ACTION_SCRIPT_3("actionscript3"),
BASH("bash"),
C_SHARP("c#"), // (C#)
COLD_FUSION("coldfusion"),
CPP("cpp"), //(C++)
CSS("css"),
DELPHI("delphi"),
DIFF("diff"),
ERLANG("erlang"),
GROOVY("groovy"),
HTML_XML("xml"), // html/xml
JAVA("java"),
JAVA_FX("javafx"),
JAVA_SCRIPT("js"), // JavaScript
NONE("none"), // (no syntax highlighting)
PERL("perl"),
PHP("php"),
POWER_SHELL("powershell"),
PYTHON("python"),
RUBY("ruby"),
SCALA("scala"),
SQL("sql"),
VB("vb");
public final String value;
Languages(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
}
/**
* The available values for the Theme parameter.
*
* @see CodeBlockMacro.Parameters#THEME
*/
public enum Themes {
D_JANGO,
EMACS,
FADE_TO_GREY,
MIDNIGHT,
R_DARK,
ECLIPSE,
CONFLUENCE;
@Override
public String toString() {
return StringUtils.convertToUpperCamel(super.toString());
}
}
/**
* Constructor.
*
* @param builder the builder instance to use as a factory.
*
* @see CodeBlockMacro.Builder
*/
protected CodeBlockMacro(final Builder builder) {
this.parameters = builder.parameters;
this.body = builder.code;
}
/**
* Converts this CodeBlockMacro into confluence markup form.
*
* @return a {@code String} containing the markup generated by this
* {@code CodeBlockMacro}.
*
* @see
*
* Confluence Page describing the Macro
*/
public String toMarkup() {
StringBuilder sb = new StringBuilder();
sb.append("");
for (Map.Entry entry : parameters.entrySet()) {
sb.append("");
sb.append(entry.getValue());
sb.append(" ");
}
sb.append("");
sb.append(body);
sb.append(" ");
sb.append(" ");
return sb.toString();
}
/**
* Builder factory method.
*
* @return a {@code Builder} instance for chain-building a CodeBlockMacro.
*/
public static Builder builder() {
return new Builder();
}
/**
* A class for implementing the Builder Pattern for {@code CodeBlockMacro}.
*/
public static class Builder {
private EnumMap parameters;
private String code;
/**
* Constructor.
*/
private Builder() {
parameters = new EnumMap<>(Parameters.class);
}
/**
* @return a new instance of {@code CodeBlockMacro}.
*/
public CodeBlockMacro build() {
return new CodeBlockMacro(this);
}
/**
*
* Example
*
{@literal
* xml
* }
*
* would be represented as:
*
{@code
* CodeBlockMacro.builder().language(Languages.HTML_XML)
* }
*
* @param l the Languages enum.
*
* @return {@code this}.
*
* @see CodeBlockMacro.Languages
*/
public Builder language(Languages l) {
parameters.putIfAbsent(Parameters.LANGUAGE, l.value);
return this;
}
/**
*
* Makes the macro collapsible:
*
{@literal
* true
* }
*
* @return {@code this}.
*/
public Builder collapse() {
parameters.putIfAbsent(Parameters.COLLAPSE, "true");
return this;
}
/**
* Enables linenumbers:
* {@literal
* true
* }
*
* @return {@code this}.
*/
public Builder showLineNumbers() {
parameters.putIfAbsent(Parameters.LINENUMBERS, "true");
return this;
}
/**
* If {@code Parameters.LINENUMBERS} is set, sets the first line number
* to start at {@code first}:
* {@literal
* true 1
* }
*
* @param first the first line number.
*
* @return {@code this}.
*/
public Builder firstline(int first) {
if (parameters.containsKey(Parameters.LINENUMBERS)) {
parameters.putIfAbsent(Parameters.FIRSTlINE, Integer.toString(first));
}
return this;
}
/**
*
* Adds a theme, for example; adding eclipse theme:
*
{@literal
* Eclipse
* }
*
* @param theme the {@code Theme} to use.
*
* @return {@code this}.
*/
public Builder theme(Themes theme) {
parameters.putIfAbsent(Parameters.THEME, theme.toString());
return this;
}
/**
*
* Sets the title for the code block, for example, here we call it
* {@literal "Request"}:
*
{@literal
* Request
* }
*
* @param title the title for this {@code CodeBlockMacto}.
*
* @return {@code this}
*/
public Builder title(String title) {
parameters.putIfAbsent(Parameters.TITLE, title);
return this;
}
/**
* The code to be displayed by this code body.
*
* @param code the code contents, as a String.
*
* @return {@code this}.
*/
public Builder code(String code) {
// escape code in wrapper
this.code = "";
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy