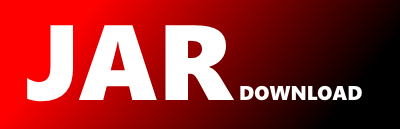
com.luoshu.open.id.JdbcIdFactory Maven / Gradle / Ivy
package com.luoshu.open.id;
import com.luoshu.open.id.exception.IdException;
import lombok.extern.slf4j.Slf4j;
import javax.sql.DataSource;
import java.sql.ResultSet;
import java.sql.SQLException;
/**
* 基于 jdbc 的 id 工厂
*/
@Slf4j
public class JdbcIdFactory implements IdFactory{
private final DataSource dataSource;
/**
* 是否自动创建表,只支持 mysql
*/
private final boolean autoCreateTable;
private final String name;
public JdbcIdFactory(DataSource dataSource , boolean autoCreateTable) {
this(IdContext.DEFAULT , dataSource , autoCreateTable);
}
public JdbcIdFactory(String name , DataSource dataSource , boolean autoCreateTable) {
this.dataSource = dataSource;
this.autoCreateTable = autoCreateTable;
this.name = name;
// 根据需要,初始化表
initTable();
}
@Override
public String getName() {
return this.name;
}
@Override
public IdGenerate create(String category, JdbcIdConfig config) {
if(category == null || "".equals(category.trim())){
throw new IdException("category is empty");
}
if(config == null){
throw new IdException("config is null");
}
// category 最大长度
int maxCategoryLength = 40;
if(category.length() > maxCategoryLength){
throw new IdException("category max length " + maxCategoryLength);
}
return new JdbcIdGenerate(category , dataSource , config);
}
@Override
public IdGenerate create(String category) {
return create(category , new JdbcIdConfig());
}
/**
* 根据需求,初始化数据库表
*/
protected void initTable(){
if(!autoCreateTable){
return;
}
Boolean existTable = null;
// 查询数据库是否已存在该表
String checkSql = "select * from t_id limit 1;";
try {
SqlUtil.executeQuery(dataSource , checkSql);
existTable = true;
} catch (SQLException e) {
// 查询失败,代表着需要去初始化创建表
// log.error(e.getMessage() , e);
existTable = false;
}
if(existTable){
// 已经存在表,不需要创建
return;
}
String createTableSql = "CREATE TABLE `t_id` (\n" +
"\t`id` int NOT NULL AUTO_INCREMENT,\n" +
"\t`category` varchar(50) NOT NULL COMMENT '类别,定义不同的类型,每个类别有一个自己的序列',\n" +
"\t`num` bigint NOT NULL DEFAULT 1 COMMENT '序列的当前值 , 当前值可用',\n" +
"\t`max_num` bigint COMMENT '序列的最大值,超过将会无法获取',\n" +
"\t`version` varchar(20) NOT NULL COMMENT '版本号,用于乐观锁',\n" +
"\t`remark` varchar(128) NOT NULL DEFAULT '' COMMENT '备注信息',\n" +
"\t`create_time` datetime NOT NULL COMMENT '创建时间',\n" +
"\t`update_time` datetime NOT NULL COMMENT '修改时间',\n" +
"\tPRIMARY KEY (`id`),\n" +
"\tUnique KEY `idx_uni_category`(`category`)\n" +
") \n" +
"COMMENT='分布式id';";
log.info("luoshu-id init table\n" + createTableSql);
try {
SqlUtil.execute(dataSource , createTableSql);
} catch (Exception e) {
String errMsg = "luoshu-id init table fail";
System.err.println(errMsg);
log.error(errMsg + "," + e.getMessage() , e);
try {
Thread.sleep(5000);
} catch (InterruptedException interruptedException) {
interruptedException.printStackTrace();
}
System.exit(3);
// 这里不会执行到,为了防止 ide 报黄
throw new IdException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy