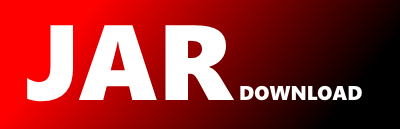
com.luues.security.core.sqlite.SqliteTemplate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-boot-starter-security Show documentation
Show all versions of spring-boot-starter-security Show documentation
身份凭证的生成及验证封装(jwt、token、session共享三种方式)
/*
package com.luues.security.core.sqlite;
import com.luues.security.core.interfaces.Security;
import com.luues.security.enumeration.SecurityConsts;
import lombok.SneakyThrows;
import org.springframework.boot.jdbc.DataSourceBuilder;
import org.springframework.stereotype.Repository;
import org.sqlite.SQLiteDataSource;
import javax.annotation.PostConstruct;
import javax.sql.DataSource;
import java.io.File;
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
*/
/**
* @author wg
* @date 2021/6/15 9:36
*//*
@Repository
public class SqliteTemplate {
private DataSource dataSource;
private final String url = "jdbc:sqlite:".concat(System.getProperty("java.io.tmpdir").concat("luues/security/black/black.db"));
@PostConstruct
@SneakyThrows
public void init() {
File file = new File(url.split("/black.db")[0].split("jdbc:sqlite:")[1]);
if (!file.exists()) {
file.mkdirs();
}
file = new File(file, "black.db");
if (!file.exists()) {
file.createNewFile();
}
dataSource = DataSourceBuilder.create()
.type(SQLiteDataSource.class)
.driverClassName("org.sqlite.SQLiteDataSource")
.url(url)
.build();
this.insert("CREATE TABLE IF NOT EXISTS security_black(" +
"id bigint(20) PRIMARY KEY NOT NULL, " +
"ip varchar(19) NOT NULL, " +
"createTime varchar(23) NOT NULL, " +
"expireTime varchar(23) NOT NULL, " +
"type varchar(10) NOT NULL, " +
"remove int(1) NOT NULL " +
")");
}
@SneakyThrows
public List list(String sql) {
Connection connection = null;
Statement statement = null;
ResultSet resultSet = null;
List list = new ArrayList<>();
try {
connection = dataSource.getConnection();
statement = connection.createStatement();
resultSet = statement.executeQuery(sql);
if (null != resultSet) {
while (resultSet.next()) {
list.add(new BlackVo(
resultSet.getLong(1),
resultSet.getString(2),
resultSet.getString(3),
resultSet.getString(4),
SecurityConsts.GENERATE.getByName(resultSet.getString(5)),
resultSet.getInt(6)));
}
return list;
}
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
if (null != resultSet)
resultSet.close();
if (null != statement)
statement.close();
if (null != connection)
connection.close();
}
return null;
}
@SneakyThrows
public BlackVo getOne(String sql) {
Connection connection = null;
Statement statement = null;
ResultSet resultSet = null;
try {
connection = dataSource.getConnection();
statement = connection.createStatement();
resultSet = statement.executeQuery(sql);
if (null != resultSet) {
while (resultSet.next()) {
return new BlackVo(
resultSet.getLong(1),
resultSet.getString(2),
resultSet.getString(3),
resultSet.getString(4),
SecurityConsts.GENERATE.valueOf(resultSet.getString(5)),
resultSet.getInt(6));
}
}
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
if (null != resultSet)
resultSet.close();
if (null != statement)
statement.close();
if (null != connection)
connection.close();
}
return null;
}
@SneakyThrows
public boolean insert(String sql) {
Connection connection = null;
Statement statement = null;
try {
connection = dataSource.getConnection();
statement = connection.createStatement();
int k = statement.executeUpdate(sql);
if (k > 0)
return true;
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
if (null != statement)
statement.close();
if (null != connection)
connection.close();
}
return false;
}
@SneakyThrows
public boolean update(String sql) {
Connection connection = null;
Statement statement = null;
try {
connection = dataSource.getConnection();
statement = connection.createStatement();
int k = statement.executeUpdate(sql);
if (k > 0)
return true;
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
if (null != statement)
statement.close();
if (null != connection)
connection.close();
}
return false;
}
}
*/
© 2015 - 2025 Weber Informatics LLC | Privacy Policy