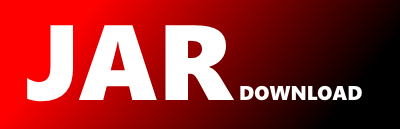
com.luues.rabbitmq.service.RabbitTemplate Maven / Gradle / Ivy
package com.luues.rabbitmq.service;
//import com.luues.util.logs.LogUtil;
//import com.luues.util.uuid.JUUID;
import org.springframework.amqp.core.*;
import org.springframework.amqp.rabbit.connection.CorrelationData;
import org.springframework.amqp.rabbit.core.RabbitAdmin;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Configuration;
import org.springframework.transaction.annotation.Transactional;
import java.util.UUID;
@Configuration
public class RabbitTemplate {
@Autowired
@Qualifier(value = "officialRabbitTemplate")
private org.springframework.amqp.rabbit.core.RabbitTemplate rabbitTemplate;
@Autowired(required = false)
private RabbitAdmin rabbitAdmin;
@Transactional
public void send(String routingKey, Object message){
//addQueue(new Queue(routingKey));
rabbitTemplate.convertAndSend(routingKey, message, new CorrelationData(UUID.randomUUID().toString()));
}
@Transactional
public void send(String routingKey, Object message, MessagePostProcessor messagePostProcessor){
//addQueue(new Queue(routingKey));
rabbitTemplate.convertAndSend(routingKey, message, messagePostProcessor, new CorrelationData(UUID.randomUUID().toString()));
}
/*@Transactional
public Message sendSync(String routingKey, Object message){
return rabbitTemplate.sendAndReceive(routingKey, new Message(message.toString().getBytes(), new MessageProperties()), new CorrelationData(UUID.randomUUID().toString()));
}*/
@Transactional
public void send(String exchange, String routingKey, Object message){
/*Queue queue = new Queue(routingKey);
addQueue(queue);
switch (type){
case ExchangeTypes.TOPIC:
TopicExchange topicExchange = new TopicExchange(exchange);
addExchange(topicExchange);
addBinding(queue, topicExchange, routingKey.contains(".#") ? routingKey.substring(0, routingKey.indexOf(".")) + ".#" : routingKey);
break;
case ExchangeTypes.FANOUT:
FanoutExchange fanoutExchange = new FanoutExchange(exchange);
addExchange(fanoutExchange);
addBinding(queue, fanoutExchange);
break;
case ExchangeTypes.DIRECT:
DirectExchange directExchange = new DirectExchange(exchange);
addExchange(directExchange);
addBinding(queue, directExchange, routingKey);
case ExchangeTypes.HEADERS:
*//*HeadersExchange headersExchange = new HeadersExchange(exchange);
addExchange(headersExchange);
addBinding(queue, headersExchange);*//*
break;
case ExchangeTypes.SYSTEM:
break;
default:
//LogUtil.error("error:{}", "未定义的类型!");
break;
}*/
rabbitTemplate.convertAndSend(exchange, routingKey, message, new CorrelationData(UUID.randomUUID().toString()));
}
@Transactional
public void send(String exchange, String routingKey, Object message, MessagePostProcessor messagePostProcessor){
rabbitTemplate.convertAndSend(exchange, routingKey, message, messagePostProcessor, new CorrelationData(UUID.randomUUID().toString()));
}
/*@Transactional
public Message sendSync(String exchange, String routingKey, Object message){
return rabbitTemplate.sendAndReceive(exchange, routingKey, new Message(message.toString().getBytes(), new MessageProperties()), new CorrelationData(UUID.randomUUID().toString()));
}*/
/*public void setConfirmCallback(org.springframework.amqp.rabbit.core.RabbitTemplate.ConfirmCallback confirmCallback){
rabbitTemplate.setConfirmCallback(confirmCallback);
}
public void setReturnCallback(org.springframework.amqp.rabbit.core.RabbitTemplate.ReturnCallback returnCallback){
rabbitTemplate.setReturnCallback(returnCallback);
}*/
/**
* 创建Exchange
*
* @param exchange
*/
private void addExchange(AbstractExchange exchange) {
rabbitAdmin.declareExchange(exchange);
}
/**
* 创建一个指定的Queue
*
* @param queue
* @return queueName
*/
private String addQueue(Queue queue) {
return rabbitAdmin.declareQueue(queue);
}
/**
* 绑定一个队列到一个匹配型交换器使用一个routingKey
*
* @param queue
* @param exchange
* @param routingKey
*/
private void addBinding(Queue queue, TopicExchange exchange, String routingKey) {
Binding binding = BindingBuilder.bind(queue).to(exchange).with(routingKey);
rabbitAdmin.declareBinding(binding);
}
private void addBinding(Queue queue, FanoutExchange exchange) {
Binding binding = BindingBuilder.bind(queue).to(exchange);
rabbitAdmin.declareBinding(binding);
}
private void addBinding(Queue queue, DirectExchange exchange, String routingKey) {
Binding binding = BindingBuilder.bind(queue).to(exchange).with(routingKey);
rabbitAdmin.declareBinding(binding);
}
/*private void addBinding(Queue queue, HeadersExchange exchange, String routingKey) {
Binding binding = BindingBuilder.bind(queue).to(exchange).where(routingKey).;
rabbitAdmin.declareBinding(binding);
}*/
/* private Queue createDelayQueue(String queueName, Integer delayMillis) {
*//**
* 队列名称 //死信时间 ,死信重新投递的交换机 ,路由到队列的routingKey
*//*
return QueueBuilder.durable(queueName)
.withArgument("x-message-ttl", delayMillis)
.withArgument("x-expires", delayMillis * DELAY_LIFE) //设置队列自动删除时间
.withArgument("x-dead-letter-exchange", rabbitConfig.getExchange())
.withArgument("x-dead-letter-routing-key", rabbitConfig.getQueue())
.build();
}
*//**
* 创建不删除的队列
* @param queueName
* @param delayMillis
* @return
*//*
private Queue createDelayQueueNoDelete(String queueName, Integer delayMillis) {
*//**
* 队列名称 //死信时间 ,死信重新投递的交换机 ,路由到队列的routingKey
*//*
return QueueBuilder.durable(queueName)
.withArgument("x-message-ttl", delayMillis)
.withArgument("x-dead-letter-exchange", rabbitConfig.getExchange())
.withArgument("x-dead-letter-routing-key", rabbitConfig.getQueue())
.build();
}*/
private DirectExchange createExchange(String exchangeName) {
return new DirectExchange(exchangeName, true, false);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy