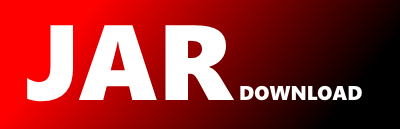
com.web.spring.datatable.TableQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-jquery-datatable Show documentation
Show all versions of spring-jquery-datatable Show documentation
Spring extension to work with the great jQuery plugin DataTables
package com.web.spring.datatable;
import com.library.common.ReflectHelper;
import com.library.common.StringHelper;
import com.web.spring.datatable.annotations.SqlCondition;
import com.web.spring.datatable.annotations.SqlIndex;
import com.web.spring.datatable.annotations.SqlIndexOperator;
import com.web.spring.datatable.util.Validate;
import javax.persistence.*;
import java.lang.reflect.Field;
import java.math.BigInteger;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* The type Table query.
*/
public class TableQuery {
private static HashMap innodbMap = new HashMap<>();
private static HashMap entityManagerInitMap = new HashMap<>();
private EntityManager entityManager;
private Class entiteClass;
private DatatablesCriterias criterias;
private int displayRecordsLength = 0;
private String customSQL = "";
private List selectColumnList = new ArrayList<>();
private HashMap fieldTypeMap = new HashMap<>();
private String entiteTableName = "";
private Long totalCount = 0L;
private Long filteredCount = 0L;
/**
* Instantiates a new Table query.
*
* @param entityManager the entity manager
* @param entiteClass the entite class
* @param criterias the criterias
*/
public TableQuery(EntityManager entityManager, Class entiteClass, DatatablesCriterias criterias) {
this.entityManager = entityManager;
this.entiteClass = entiteClass;
this.criterias = criterias;
init();
}
/**
* Instantiates a new Table query.
*
* @param entityManager the entity manager
* @param entiteClass the entite class
* @param criterias the criterias
* @param customSQL the custom sql
*/
public TableQuery(EntityManager entityManager, Class entiteClass, DatatablesCriterias criterias, String customSQL) {
this.entityManager = entityManager;
this.entiteClass = entiteClass;
this.criterias = criterias;
this.customSQL = customSQL;
List columnList;
int from_min = 10000;
String from_str = "";
int from_1 = this.customSQL.indexOf(" from ");
if (from_1 > 0 && from_min > from_1) {
from_min = from_1;
from_str = " from ";
}
int from_2 = this.customSQL.indexOf("\nfrom ");
if (from_2 > 0 && from_min > from_2) {
from_min = from_2;
from_str = "\nfrom ";
}
int from_3 = this.customSQL.indexOf(" from\n");
if (from_3 > 0 && from_min > from_3) {
from_str = " from\n";
}
String columnString = StringHelper.getBetweenString(this.customSQL.toLowerCase(), "select", from_str);
String regex = "\\(.*?\\) *?as";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(columnString);
while (matcher.find()) {
System.out.println("matcher: " + matcher.group(0));
columnString = columnString.replace(matcher.group(0).substring(0, matcher.group(0).length() - 3), "");
}
columnList = Arrays.asList(columnString.split(","));
for (String columnName : columnList) {
if (columnName.toLowerCase().contains(" as ")) {
selectColumnList.add(columnName.substring(columnName.lastIndexOf(" as ") + 4).trim());
} else {
if (columnName.contains("(") || columnName.contains(")")) {
continue;
}
if (columnName.contains(".")) {
selectColumnList.add(columnName.substring(columnName.lastIndexOf(".") + 1).trim());
} else {
selectColumnList.add(columnName.trim());
}
}
}
init();
}
/**
* Gets filtered count.
*
* @return the filtered count
*/
public Long getFilteredCount() {
return filteredCount;
}
/**
* Gets total count.
*
* @return the total count
*/
public Long getTotalCount() {
return totalCount;
}
/**
* Init.
*/
@SuppressWarnings("unchecked")
public void init() {
if (this.entiteClass.isAnnotationPresent(Table.class)) {
Table table = (Table) this.entiteClass.getAnnotation(Table.class);
this.entiteTableName = table.name();
} else {
this.entiteTableName = this.entiteClass.getSimpleName();
}
if (entityManagerInitMap.get(this.entityManager) == null) {
Query query = this.entityManager.createNativeQuery("SELECT table_name FROM INFORMATION_SCHEMA.TABLES WHERE engine = 'InnoDB' and TABLE_SCHEMA != 'mysql'");
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy