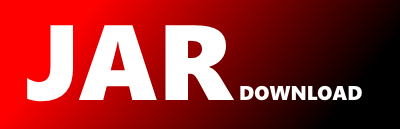
com.github.magrifle.data.searchapi.specification.SpecificationsBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of data-search-api Show documentation
Show all versions of data-search-api Show documentation
A library that helps you instantly turn your Spring powered endpoints into a query engine. It makes use
of AOP to intercept the calls to your Controller or RestController endpoints and then builds a Specification
from the provided query parameters
package com.github.magrifle.data.searchapi.specification;
import com.github.magrifle.data.searchapi.SearchKeyConfigurerService;
import com.github.magrifle.data.searchapi.SearchOperation;
import com.github.magrifle.data.searchapi.data.SearchCriteria;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import org.springframework.data.jpa.domain.Specification;
public class SpecificationsBuilder {
private final SearchKeyConfigurerService searchKeyConfigurerService;
private final boolean caseSensitive;
private List params = new ArrayList<>();
public SpecificationsBuilder(SearchKeyConfigurerService searchKeyConfigurerService, boolean caseSensitive) {
this.searchKeyConfigurerService = searchKeyConfigurerService;
this.caseSensitive = caseSensitive;
}
public SpecificationsBuilder with(
String key, String operation, Object value, String prefix, String suffix) {
SearchOperation op = SearchOperation.SIMPLE_OPERATION_SET.get(operation.charAt(0));
if (op != null) {
if (op == SearchOperation.EQUALITY) {
boolean startWithAsterisk = prefix.equals("*");
boolean endWithAsterisk = suffix.equals("*");
if (startWithAsterisk && endWithAsterisk) {
op = SearchOperation.CONTAINS;
} else if (startWithAsterisk)
{
op = SearchOperation.ENDS_WITH;
}
else if (endWithAsterisk)
{
op = SearchOperation.STARTS_WITH;
}
else if (prefix.equals("[") && suffix.equals("]"))
{
op = SearchOperation.IN;
}
}
SearchCriteria searchCriteria = new SearchCriteria(key, op, value);
searchCriteria.setCaseSensitive(caseSensitive);
params.add(searchCriteria);
}
return this;
}
public Specification build() {
if (params.size() == 0) {
return null;
}
this.searchKeyConfigurerService.prepareSearchData(params);
List> specs = new ArrayList<>();
for (SearchCriteria param : params) {
specs.add(new EntitySpecification<>(param));
}
Specification result = specs.get(0);
for (int i = 1; i < specs.size(); i++) {
result = Objects.requireNonNull(Specification.where(result)).and(specs.get(i));
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy