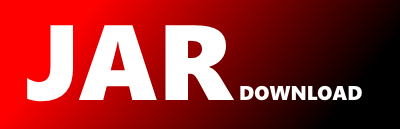
CookerCucumberMavenPlugin.FeatureFactory.ScenarioOutlineUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cooker-maven-plugin Show documentation
Show all versions of cooker-maven-plugin Show documentation
Derives smallest Feature File, Allows Data from Excel(xls and xlsx) and Also provides a clear and
concise reporting
package CookerCucumberMavenPlugin.FeatureFactory;
import gherkin.ast.Examples;
import gherkin.ast.ScenarioOutline;
import gherkin.ast.Step;
import gherkin.ast.Tag;
import java.util.ArrayList;
import java.util.List;
public class ScenarioOutlineUtils implements ScenarioOutlineI {
private ScenarioOutline scenarioOutline = null;
private StringBuilder result = new StringBuilder();
private String sSoKeyword;
private String sSoName;
private String sSoDescription;
private List sSoTags;
private List sSoStep;
public String getsSoKeyword() {
return sSoKeyword;
}
public String getsSoName() {
return sSoName;
}
public String getsSoDescription() {
return sSoDescription;
}
List sSoExamples;
public ScenarioOutlineUtils(ScenarioOutline pScenarioOutline) {
this.scenarioOutline = pScenarioOutline;
sSoKeyword = this.scenarioOutline.getKeyword();
sSoName = this.scenarioOutline.getName();
sSoDescription = this.scenarioOutline.getDescription();
sSoTags = this.scenarioOutline.getTags();
sSoStep = scenarioOutline.getSteps();
sSoExamples = scenarioOutline.getExamples();
}
public String getScenarioOutlineData() {
try {
for (Tag tag : sSoTags) {
TagUtils tu = new TagUtils(tag);
String soTagsData = tu.getTagsData();
this.result.append(soTagsData);
}
this.result.append(scenarioOutline.getKeyword()).append(": ").append(scenarioOutline.getName());
this.result.append(System.getProperty("line.separator"));
this.result.append(scenarioOutline.getDescription() == null ? "" : scenarioOutline.getDescription());
this.result.append(System.getProperty("line.separator"));
for (Step step : sSoStep) {
StepsUtils stepsUtils = new StepsUtils(step);
String soSteps = stepsUtils.getStepsData();
this.result.append(soSteps);
}
for (Examples examples : sSoExamples) {
ExamplesUtils examplesUtils = new ExamplesUtils(examples);
String soExampleData = examplesUtils.getExamplesData();
this.result.append(soExampleData);
}
} catch (Exception e) {
e.printStackTrace();
}
return String.valueOf(this.result);
}
// public String getScenarioOutlineTags() {
// StringBuilder ScenarioOutlineTags = new StringBuilder();
//
// for (Tag tag : sSoTags) {
// TagUtils tagUtils = new TagUtils(tag);
// ScenarioOutlineTags.append(tagUtils.getTags());
// }
// return String.valueOf(ScenarioOutlineTags);
// }
public List getScenarioOutlineTagsList() {
List res = new ArrayList();
try {
for (Tag tag : sSoTags) {
TagUtils tagUtils = new TagUtils(tag);
res.add(tagUtils.getTagsData().trim());
}
} catch (Exception e) {
e.printStackTrace();
}
return res;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy