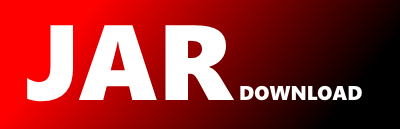
com.github.markusbernhardt.selenium2library.keywords.Logging Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of robotframework-selenium2library-java Show documentation
Show all versions of robotframework-selenium2library-java Show documentation
Java port of the Selenium 2 (WebDriver) Python library for Robot Framework
package com.github.markusbernhardt.selenium2library.keywords;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import org.python.core.PyString;
import org.python.util.PythonInterpreter;
import com.github.markusbernhardt.selenium2library.Selenium2LibraryNonFatalException;
import com.github.markusbernhardt.selenium2library.utils.Python;
public abstract class Logging extends JavaScript {
// ##############################
// Internal Methods
// ##############################
@Override
protected void trace(String msg) {
loggingPythonInterpreter.get().exec(
String.format("logger.trace('%s');", msg.replace("'", "\\'")
.replace("\n", "\\n")));
}
@Override
protected void debug(String msg) {
loggingPythonInterpreter.get().exec(
String.format("logger.debug('%s');", msg.replace("'", "\\'")
.replace("\n", "\\n")));
}
@Override
protected void info(String msg) {
loggingPythonInterpreter.get().exec(
String.format("logger.info('%s');", msg.replace("'", "\\'")
.replace("\n", "\\n")));
}
@Override
protected void html(String msg) {
loggingPythonInterpreter.get().exec(
String.format("logger.info('%s', True, False);",
msg.replace("'", "\\'").replace("\n", "\\n")));
}
@Override
protected void warn(String msg) {
loggingPythonInterpreter.get().exec(
String.format("logger.warn('%s');", msg.replace("'", "\\'")
.replace("\n", "\\n")));
}
@Override
protected void log(String msg, String logLevel) {
logLevel = logLevel.toLowerCase();
if (logLevel.equals("trace")) {
trace(msg);
} else if (logLevel.equals("debug")) {
debug(msg);
} else if (logLevel.equals("info")) {
info(msg);
} else if (logLevel.equals("html")) {
html(msg);
} else if (logLevel.equals("warn")) {
warn(msg);
} else {
throw new Selenium2LibraryNonFatalException(String.format(
"Given log level %s is invalid.", logLevel));
}
}
@Override
protected List logList(List items) {
return logList(items, "item");
}
@Override
protected List logList(List items, String what) {
List msg = new ArrayList();
msg.add(String.format("Altogether %d %s%s.\n", items.size(), what,
items.size() == 1 ? "" : "s"));
for (int index = 0; index < items.size(); index++) {
msg.add(String.format("%d: %s", index + 1, items.get(index)));
}
info(Python.join("\n", msg));
return items;
}
@Override
protected File getLogDir() {
PyString logDirName = (PyString) loggingPythonInterpreter.get().eval(
"GLOBAL_VARIABLES['${LOG FILE}']");
if (logDirName != null) {
return new File(logDirName.asString()).getParentFile();
}
logDirName = (PyString) loggingPythonInterpreter.get().eval(
"GLOBAL_VARIABLES['${OUTPUTDIR}']");
return new File(logDirName.asString()).getParentFile();
}
protected static ThreadLocal loggingPythonInterpreter = new ThreadLocal() {
@Override
protected PythonInterpreter initialValue() {
PythonInterpreter pythonInterpreter = new PythonInterpreter();
pythonInterpreter
.exec("from robot.variables import GLOBAL_VARIABLES; from robot.api import logger;");
return pythonInterpreter;
}
};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy