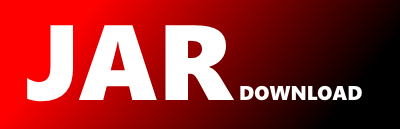
org.chronos.common.logging.ChronoLogger Maven / Gradle / Ivy
package org.chronos.common.logging;
import org.chronos.common.base.CCC;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.HashMap;
import java.util.Map;
/**
* This is a thin wrapper around a logging facility (org.slf4j
).
* It is recommended to import this class using a static
import as such:
*
*
*
* import static org.chronos.common.logging.ChronoLogger.*;
*
*
*
* Afterwards, you can access the static methods provided by this class without qualifying them. For example:
*
*
*
* log("This is an information");
* logWarning("This is a warning");
* logError("This is an error");
*
*
*
* Also, you can log exceptions directly as such:
*
*
*
* try {
* // do some unsafe work here...
* } catch (Exception e) {
* // log the exception directly
* logError(e);
* }
*
*
*
* @author [email protected] -- Initial Contribution and API
*/
public class ChronoLogger {
/**
* The map of all maintained loggers.
*/
private static final Map LOGGERS = new HashMap();
/**
* Returns a logger for the given name.
* The retrieved logger is a java.util.logging.
{@link Logger} that does not listen to its parent
* handlers, and has a custom {@link ConsoleLogHandler} attached that works based on a custom {@link LogFormatter}.
*
* @param name The name of the logger to retrieve
* @return The logger for this name.
*/
private static Logger getLogger(final String name) {
if (LOGGERS.containsKey(name)) {
return LOGGERS.get(name);
}
Logger newLogger = LoggerFactory.getLogger(name);
// newLogger.setUseParentHandlers(false);
// Handler logHandler = new ConsoleLogHandler();
// logHandler.setFormatter(LogFormatter.INSTANCE);
// newLogger.addHandler(logHandler);
LOGGERS.put(name, newLogger);
return newLogger;
}
/**
* Internal method for logging purposes. Must not be called by clients directly.
*
* @param level The log level to use
* @param message The message to log
* @param error Only valid for {@link LogLevel#ERROR} messages. Contains an optional throwable. May be
* null
.
*/
private static void logInternal(final LogLevel level, final String message, final Throwable error) {
StackTraceElement caller = Thread.currentThread().getStackTrace()[3];
String callerClass = caller.getClassName();
// System.out.println("CALLER CLASS: '" + callerClass + "'");
Logger logger = getLogger(callerClass);
switch (level) {
case TRACE:
logger.trace(message);
break;
case INFO:
logger.info(message);
break;
case DEBUG:
logger.debug(message);
break;
case WARN:
logger.warn(message);
break;
case ERROR:
if (error == null) {
// no throwable given, only log the message
logger.error(message);
} else {
// throwable is given, log message and throwable
logger.error(message, error);
}
break;
default:
throw new IllegalArgumentException("Unknown LogLevel: " + level);
}
}
/**
* Logs a message to the console, based on the given log level.
*
* @param logLevel The log level to employ. Must not be null
, is a no-op if it is OFF.
* @param message The message to log.
*/
public static void log(final LogLevel logLevel, final String message) {
if (logLevel == LogLevel.OFF) {
return;
}
logInternal(logLevel, message == null ? "" : message, null);
}
/**
* Logs an information message to the console.
* Fully equivalent to:
*
*
*
* logInfo(message);
*
*
* @param message The message to log. If null
, the empty string will be used instead.
*/
public static void log(final String message) {
if (CCC.MIN_LOG_LEVEL.isGreaterThan(LogLevel.INFO)) {
// don't log
return;
}
String actualMessage = message;
if (actualMessage == null) {
actualMessage = "";
}
logInternal(LogLevel.INFO, actualMessage, null);
}
/**
* Logs a trace message to the console.
*
* @param message The message to log. If null
, the empty string will be used instead.
*/
public static void logTrace(final String message) {
if (CCC.MIN_LOG_LEVEL.isGreaterThan(LogLevel.TRACE)) {
// don't log
return;
}
String actualMessage = message;
if (actualMessage == null) {
actualMessage = "";
}
logInternal(LogLevel.TRACE, actualMessage, null);
}
/**
* Logs an information message to the console.
*
* @param message The message to log. If null
, the empty string will be used instead.
*/
public static void logInfo(final String message) {
if (CCC.MIN_LOG_LEVEL.isGreaterThan(LogLevel.INFO)) {
// don't log
return;
}
String actualMessage = message;
if (actualMessage == null) {
actualMessage = "";
}
logInternal(LogLevel.INFO, actualMessage, null);
}
/**
* Logs a debug message to the console.
*
* @param message The message to log. If null
, the empty string will be used instead.
*/
public static void logDebug(final String message) {
if (CCC.MIN_LOG_LEVEL.isGreaterThan(LogLevel.DEBUG)) {
// don't log
return;
}
String actualMessage = message;
if (actualMessage == null) {
actualMessage = "";
}
logInternal(LogLevel.DEBUG, actualMessage, null);
}
/**
* Logs a warning message to the console.
*
* @param message The message to log. If null
, the empty string will be used instead.
*/
public static void logWarning(final String message) {
if (CCC.MIN_LOG_LEVEL.isGreaterThan(LogLevel.WARN)) {
// don't log
return;
}
String actualMessage = message;
if (actualMessage == null) {
actualMessage = "";
}
logInternal(LogLevel.WARN, actualMessage, null);
}
/**
* Logs an error message to the console.
* NOTE: By default, messages classified as ERROR will appear on System.err
instead of
* System.out
.
*
* @param message The message to log. If null
, the empty string will be used instead.
*/
public static void logError(final String message) {
if (CCC.MIN_LOG_LEVEL.isGreaterThan(LogLevel.ERROR)) {
// don't log
return;
}
String actualMessage = message;
if (actualMessage == null) {
actualMessage = "";
}
logInternal(LogLevel.ERROR, actualMessage, null);
}
/**
* Logs an exception to the console.
* NOTE: By default, such messages will appear on System.err
instead of System.out
.
*
* @param message A textual message describing the error
* @param throwable The exception to log. May be null
.
*/
public static void logError(final String message, final Throwable throwable) {
if (CCC.MIN_LOG_LEVEL.isGreaterThan(LogLevel.ERROR)) {
// don't log
return;
}
if (throwable == null) {
return;
}
logInternal(LogLevel.ERROR, message, throwable);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy