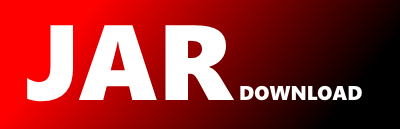
org.chronos.common.util.CacheUtils Maven / Gradle / Ivy
package org.chronos.common.util;
import static com.google.common.base.Preconditions.*;
import java.util.function.Function;
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
/**
* This is a very simple utility class that eases the syntactical handling of the Guava {@link CacheBuilder}.
*
*
* Please use one of the static
methods provided by this class. Do not instantiate it.
*
* @author [email protected] -- Initial Contribution and API
*
*/
public class CacheUtils {
/**
* Builds a new Least-Recently-Used cache with the given size and loading function.
*
* @param size
* The size of the cache, i.e. the maximum number of elements that can be present. Must be strictly larger than zero. Loading an element that would exceed the given limit will cause the least-recently-used element to be evicted from the cache.
* @param loadingFunction
* The function that loads the value for the given key. Must not be null
.
*
* @return The newly created cache. Never null
.
*/
public static LoadingCache buildLRU(final int size, final Function loadingFunction) {
checkArgument(size > 0, "Precondition violation - argument 'size' must be greater than zero!");
checkNotNull(loadingFunction, "Precondition violation - argument 'loadingFunction' must not be NULL!");
return CacheBuilder.newBuilder().maximumSize(size).build(new CacheLoader() {
@Override
public V load(final K key) throws Exception {
V value = loadingFunction.apply(key);
if(value == null){
throw new Exception("No element with ID '" + key + "'!");
}
return value;
}
});
}
/**
* Builds a new cache with weakly referenced values.
*
* @param loadingFunction The function that loads the value for a given key in case of a cache miss. Must not be null
.
* @param The type of the keys
* @param The type of the values
* @return The newly created cache instance. Never null
.
*/
public static LoadingCache buildWeak(final Function loadingFunction) {
checkNotNull(loadingFunction, "Precondition violation - argument 'loadingFunction' must not be NULL!");
return CacheBuilder.newBuilder().weakValues().build(new CacheLoader() {
@Override
public V load(final K key) throws Exception {
V value = loadingFunction.apply(key);
if(value == null){
throw new Exception("No element with ID '" + key + "'!");
}
return value;
}
});
}
}