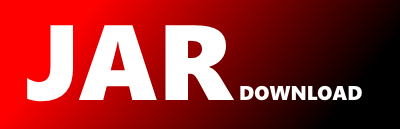
com.github.matheusesoft.alm.api.Client Maven / Gradle / Ivy
package com.github.matheusesoft.alm.api;
import static com.github.matheusesoft.alm.api.Util.readAlmProperties;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.net.InetAddress;
import java.net.UnknownHostException;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.github.matheusesoft.alm.api.model.*;
import com.github.matheusesoft.alm.api.utils.Config;
import com.github.matheusesoft.alm.api.utils.ZipHelper;
public class Client {
private final static Logger Log = LoggerFactory.getLogger(Client.class);
private final Config config;
public static Class> clazz;
private static Client instance = null;
private static Date inicioExec;
private Client() throws IOException, Exception {
config = new Config(readAlmProperties(clazz));
RestConnector.instance().init(config.host(), config.port(), config.domain(), config.project());
}
public static void start(Class> clazz) {
inicioExec = new Date();
Client.clazz = clazz;
}
public static Client get() {
if (clazz != null) {
if (instance == null) {
try {
instance = new Client();
} catch (Exception e) {
e.printStackTrace();
}
}
} else {
new Exception("Client.start(Class ?) - start Client Class = @AlmOptions class");
}
return instance;
}
public void login() throws Exception {
Log.info(String.format("Logging in as '%s' ...", config.username()));
Dao.login(config.username(), config.password());
Log.info(String.format("Successfully authenticated as '%s'", config.username()));
}
public void logout() throws Exception {
Dao.logout();
Log.info("Successfully logout");
}
public TestSet loadTestSet(String testSetId) throws Exception {
Log.info(String.format("Loading TestSet ... (test-set-id = %s)", testSetId));
TestSet testSet = Dao.readTestSet(config.username(), config.password(), testSetId);
Log.info(String.format("Loaded TestSet (test-set-id = %s, '%s')", testSet.id(), testSet.name()));
return testSet;
}
public ReleaseCycle loadReleaseCycle(String name, String parentId) throws Exception {
Log.info(String.format("Loading release-cycle ... (name = %s)", name));
ReleaseCycle releaseCycle = Dao.readReleaseCycles(config.username(), config.password(), name, parentId);
Log.info(String.format("Loaded TestSet (release-cycle-id = %s, '%s')", releaseCycle.id(), releaseCycle.name()));
return releaseCycle;
}
public ReleaseCycle loadReleaseCycle(String cycleId) throws Exception {
Log.info(String.format("Loading release-cycle ... (id = %s)", cycleId));
ReleaseCycle releaseCycle = Dao.readReleaseCycles(config.username(), config.password(), cycleId);
Log.info(String.format("Loaded TestSet (release-cycle-id = %s, '%s')", releaseCycle.id(), releaseCycle.name()));
return releaseCycle;
}
public Release loadRelease(String name) throws Exception {
Log.info(String.format("Loading release ... (name = %s)", name));
Release release = Dao.readRelease(config.username(), config.password(), name);
Log.info(String.format("Loaded TestSet (release-id = %s, '%s')", release.id(), release.name()));
return release;
}
public TestSet loadTestSet(String name, String assignRcyc) throws Exception {
Log.info(String.format("Loading TestSet ... (name = %s)", name));
TestSet testSet = Dao.readTestSet(config.username(), config.password(), name, assignRcyc);
Log.info(String.format("Loaded TestSet (test-set-id = %s, '%s')", testSet.id(), testSet.name()));
return testSet;
}
public TestInstances loadTestInstances(TestSet testSet) throws Exception {
Log.info(String.format("Loading TestInstances ... (test-set-id = %s)", testSet.id()));
TestInstances testInstances = Dao.readTestInstances(testSet.id());
Log.info(String.format("Loaded %d TestInstances", testInstances.entities().size()));
return testInstances;
}
public Test loadTest(TestInstance testInstance) throws Exception {
Log.info(String.format("Loading Test ... (test-id = %s)", testInstance.testId()));
Test test = Dao.readTest(testInstance.testId());
Log.info(String.format("Loaded Test (test-id = %s, '%s'", test.id(), test.name()));
return test;
}
public Run loadRun(String id) throws Exception {
Log.info(String.format("Loading Run ... "));
Run run = Dao.loadRun(id);
Log.info(String.format("Run has been Loaded (run-id = %s, '%s', %s)", run.id(), run.name(), run.status()));
return run;
}
public Run createRun(TestInstance testInstance, Test test) throws Exception {
final SimpleDateFormat dateFormat = new SimpleDateFormat("MM-dd_HH-mm-ss");
final String runName = "Run_" + dateFormat.format(this.inicioExec);
long duration = System.currentTimeMillis() - inicioExec.getTime();
Run prepRun = new Run();
prepRun.testInstanceId(testInstance.id());
prepRun.name(runName);
prepRun.testId(test.id());
prepRun.owner(config.username());
prepRun.testSetId(testInstance.testSetId());
prepRun.testType(Run.TEST_TYPE_LEANFT);
prepRun.status(Run.STATUS_NOT_COMPLETED);
prepRun.host(hostName().toUpperCase());
prepRun.comments(test.description());
prepRun.duration(Long.toString(duration/1000));
prepRun.userTemplate01("Automatizado");
Log.info(String.format("Creating Run ... ('%s', %s)", prepRun.name(), prepRun.status()));
Run run = Dao.createRun(prepRun);
Log.info(String.format("Run has been created (run-id = %s, '%s', %s)", run.id(), run.name(), run.status()));
return run;
}
public Run updateRun(Run run) throws Exception {
Log.info(String.format("Update Run ... ('%s', %s)", run.name(), run.status()));
long duration = System.currentTimeMillis() - inicioExec.getTime();
run.duration(Long.toString(duration/1000));
Run runUpdate = Dao.updateRun(run);
Log.info(String.format("Run has been update (run-id = %s, '%s', %s)", runUpdate.id(), runUpdate.name(), runUpdate.status()));
return runUpdate;
}
public void createRunSteps(Run run, RunSteps runSteps) throws Exception {
Log.info("Creating RunSteps ...");
for (RunStep runStep : runSteps.entities()) {
RunStep prepRunStep = new RunStep();
prepRunStep.runId(run.id());
prepRunStep.name(runStep.name());
prepRunStep.status(runStep.status());
Log.info(String.format("Creating RunStep ... ('%s', %s)", prepRunStep.name(), prepRunStep.status()));
Dao.createRunStep(prepRunStep);
Log.info(String.format("RunStep has been created (run-step-id = %s, '%s', %s)", prepRunStep.id(),
prepRunStep.name(), prepRunStep.status()));
}
}
public void createRunAttachment(Run run, String path) throws Exception {
Log.info("Creating Run Attachment ... "+path);
File outputFile = new File(System.getProperty("java.io.tmpdir") + "\\RunResults.zip");
File[] inputFiles = new File(path).listFiles();
new ZipHelper().zip(inputFiles, outputFile);
byte[] bFile = new byte[(int) outputFile.length()];
FileInputStream fileInputStream = null;
try {
fileInputStream = new FileInputStream(outputFile);
fileInputStream.read(bFile);
fileInputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
Dao.createRunAttachment(run.id(), "RunResults.zip", bFile);
}
public void createRunAttachmentCustom(Run run, String path) throws Exception {
if (getOptions().gravacao()) {
Log.info("Creating Run Attachment Custom ... "+path);
File outputFile = new File(System.getProperty("java.io.tmpdir") + "\\CustomRunResults.zip");
File[] inputFiles = new File(path).listFiles();
new ZipHelper().zip(inputFiles, outputFile);
byte[] bFile = new byte[(int) outputFile.length()];
FileInputStream fileInputStream = null;
try {
fileInputStream = new FileInputStream(outputFile);
fileInputStream.read(bFile);
fileInputStream.close();
} catch (Exception e) {
System.out.println(e);
}
Dao.createRunAttachment(run.id(), "CustomRunResults.zip", bFile);
}
}
private static String hostName() throws UnknownHostException {
try {
return InetAddress.getLocalHost().getHostName();
} catch (UnknownHostException e) {
return "Unknown hostname";
}
}
public AlmOptions getOptions() {
return (AlmOptions) Client.clazz.getAnnotation(AlmOptions.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy