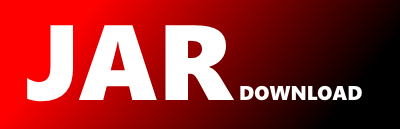
com.github.matheusesoft.alm.api.Dao Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alm-rest-api Show documentation
Show all versions of alm-rest-api Show documentation
A simple Java API client to connect to HP ALM using REST service
The newest version!
package com.github.matheusesoft.alm.api;
import java.net.URI;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.ws.rs.core.HttpHeaders;
import javax.ws.rs.core.MultivaluedHashMap;
import javax.ws.rs.core.MultivaluedMap;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.Status;
import org.apache.commons.lang.StringUtils;
import com.github.matheusesoft.alm.api.model.*;
import com.github.matheusesoft.alm.api.utils.ResponseException;
public final class Dao
{
private Dao()
{
}
public static String isAuthenticated() throws Exception
{
String isAuthenticatedUrl = "qcbin/rest/is-authenticated";
try
{
connector().get(isAuthenticatedUrl, Response.class, null, null);
return null;
}
catch (ResponseException ex)
{
Response response = ex.response();
if (response.getStatus() != Status.UNAUTHORIZED.getStatusCode())
{
throw ex;
}
String authPoint = response.getHeaderString(HttpHeaders.WWW_AUTHENTICATE);
if (StringUtils.isNotBlank(authPoint))
{
authPoint = authPoint.split("=")[1].replace("\"", "");
authPoint += "/authenticate";
return "qcbin/api/authentication/sign-in";
}
throw new Exception("Invalid authentication point");
}
}
public static void login(String authenticationPoint, String username, String password) throws Exception
{
connector().get(authenticationPoint, Response.class, RestConnector.createBasicAuthHeader(username, password), null);
}
public static void login(String username, String password) throws Exception
{
String authenticationPoint = isAuthenticated();
if (authenticationPoint != null)
{
URI uri = new URI(authenticationPoint);
login(uri.getPath(), username, password);
}
}
public static void logout() throws Exception
{
String logoutUrl = "qcbin/authentication-point/logout";
connector().get(logoutUrl, Response.class, null, null);
}
public static Test readTest(String id) throws Exception
{
String testUrl = connector().buildEntityUrl("test", id);
return connector().get(testUrl, Test.class, null, null);
}
public static TestSet readTestSet(String username, String password, String id) throws Exception
{
String testSetUrl = connector().buildEntityUrl("test-set", id);
return connector().get(testSetUrl, TestSet.class, RestConnector.createBasicAuthHeader(username, password), null);
}
public static TestSet readTestSet(String username, String password, String name, String assignRcyc) throws Exception
{
String testSetUrl = connector().buildEntityCollectionUrl("test-set");
Map criteria = new HashMap();
criteria.put("query", "{name[\"" + name + "*\"]; assign-rcyc["+assignRcyc+"]}");
return connector().get(testSetUrl, TestSets.class, RestConnector.createBasicAuthHeader(username, password), criteria).entities().get(0);
}
public static ReleaseCycle readReleaseCycles(String username, String password, String name, String parentId) throws Exception
{
String testSetUrl = connector().buildEntityCollectionUrl("release-cycle");
Map criteria = new HashMap();
criteria.put("query", "{name[\"" + name + "*\"]; parent-id["+parentId+"]}");
List cycles = connector().get(testSetUrl, ReleaseCycles.class, RestConnector.createBasicAuthHeader(username, password), criteria).entities();
for (ReleaseCycle releaseCycle : cycles) {
if (releaseCycle.name().trim().equals(name.trim())) {
return releaseCycle;
}
}
return null;
}
public static ReleaseCycle readReleaseCycles(String username, String password, String cycleId) throws Exception
{
String testSetUrl = connector().buildEntityCollectionUrl("release-cycle");
Map criteria = new HashMap();
criteria.put("query", "{id["+cycleId+"]}");
return connector().get(testSetUrl, ReleaseCycles.class, RestConnector.createBasicAuthHeader(username, password), criteria).entities().get(0);
}
public static Release readRelease(String username, String password, String name) throws Exception
{
String testSetUrl = connector().buildEntityCollectionUrl("release");
Map criteria = new HashMap();
criteria.put("query", "{name[\"" + name + "*\"]}");
return connector().get(testSetUrl, Releases.class, RestConnector.createBasicAuthHeader(username, password), criteria).entities().get(0);
}
public static TestInstance readTestInstance(String id) throws Exception
{
String testInstanceUrl = connector().buildEntityUrl("test-instance", id);
return connector().get(testInstanceUrl, TestInstance.class, null, null);
}
public static TestInstances readTestInstances(String testSetId) throws Exception
{
String testInstancesUrl = connector().buildEntityCollectionUrl("test-instance");
Map criteria = new HashMap();
criteria.put("query", "{cycle-id[" + testSetId + "]}");
return connector().get(testInstancesUrl, TestInstances.class, null, criteria);
}
public static Attachment createRunAttachment(String runId, String fileName, byte[] fileData) throws Exception
{
String attachmentsUrl = connector().buildEntityUrl("run", runId) + "/attachments";
return createAttachment(attachmentsUrl, fileName, fileData);
}
public static Attachment createRunStepAttachment(String runStepId, String fileName, byte[] fileData) throws Exception
{
String attachmentsUrl = connector().buildEntityUrl("run-step", runStepId) + "/attachments";
return createAttachment(attachmentsUrl, fileName, fileData);
}
public static Run createRun(Run run) throws Exception
{
String runsUrl = connector().buildEntityCollectionUrl("run");
return connector().post(runsUrl, Run.class, null, null, run);
}
public static Run loadRun(String runId) throws Exception
{
String runUrl = connector().buildEntityUrl("run", runId);
return connector().get(runUrl, Run.class, null, null);
}
public static Run updateRun(Run run) throws Exception
{
String runUrl = connector().buildEntityUrl("run", run.id());
run.clearBeforeUpdate();
return connector().put(runUrl, Run.class, null, null, run);
}
public static RunSteps readRunSteps(String runId) throws Exception
{
String runStepsUrl = connector().buildEntityUrl("run", runId) + "/run-steps";
return connector().get(runStepsUrl, RunSteps.class, null, null);
}
public static RunStep createRunStep(RunStep runStep) throws Exception
{
String runStepsUrl = connector().buildEntityUrl("run", runStep.runId()) + "/run-steps";
return connector().post(runStepsUrl, RunStep.class, null, null, runStep);
}
public static RunStep updateRunStep(RunStep runStep) throws Exception
{
String runStepUrl = connector().buildEntityUrl("run", runStep.runId()) + "/run-steps/" + runStep.id();
runStep.clearBeforeUpdate();
return connector().put(runStepUrl, RunStep.class, null, null, runStep);
}
private static RestConnector connector()
{
return RestConnector.instance();
}
private static Attachment createAttachment(String entityUrl, String fileName, byte[] fileData) throws Exception
{
MultivaluedMap headers = new MultivaluedHashMap();
headers.add("Slug", fileName);
return connector().post(entityUrl, Attachment.class, headers, null, fileData, "application/octet-stream");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy