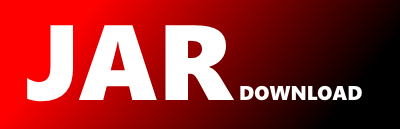
com.github.matheusesoft.alm.api.model.ReleaseCycle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alm-rest-api Show documentation
Show all versions of alm-rest-api Show documentation
A simple Java API client to connect to HP ALM using REST service
The newest version!
package com.github.matheusesoft.alm.api.model;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementWrapper;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "Entity")
@XmlAccessorType(XmlAccessType.FIELD)
public class ReleaseCycle
{
@XmlElement(name = "Field", required = true)
@XmlElementWrapper(name = "Fields")
private List fields;
@XmlAttribute(name = "Type", required = true)
private String type;
public ReleaseCycle(Entity entity)
{
this.fields = entity.fields();
this.type = entity.type();
}
public ReleaseCycle()
{
type("release-cycle");
}
public String id()
{
return fieldValue("id");
}
public void id(String value)
{
fieldValue("id", value);
}
public String name(){
return fieldValue("name");
}
public void name(String value){
fieldValue("name", value);
}
public String parentId(){
return fieldValue("parent-id");
}
public void parentId(String value){
fieldValue("parent-id", value);
}
public List fields()
{
if (fields == null)
{
fields = new ArrayList();
}
return fields;
}
public String type()
{
return type;
}
public void type(String value)
{
type = value;
}
public Field field(String name)
{
for (Field field: fields())
{
if (name.equals(field.name()))
{
return field;
}
}
return null;
}
public void removeField(String name)
{
Iterator it = fields().iterator();
while (it.hasNext())
{
Field field = it.next();
if (name.equals(field.name()))
{
it.remove();
}
}
}
public void clearBeforeUpdate()
{
removeField("id");
}
public String fieldValue(String name)
{
Field field = field(name);
return field != null ? field.value() : null;
}
public void fieldValue(String name, String value)
{
Field field = field(name);
if (field == null)
{
field = new Field(name);
fields().add(field);
}
field.value(value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy