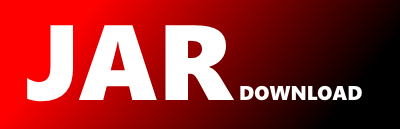
com.github.mathiewz.converter.EnumConverter Maven / Gradle / Ivy
package com.github.mathiewz.converter;
import java.util.Optional;
import java.util.function.Function;
import java.util.stream.Stream;
/**
* This class provides method to convert data to an enum entry.
* All conversions are available through static and instance methods.
* Static methods have an extra parmeter to specify which enum is targeted.
* @param The enum class to target
*/
public class EnumConverter> {
private Class clazz;
/**
* Create a new converter targeting the specified class.
* @param clazz the target class. must be an enum.
*/
public EnumConverter(Class clazz){
this.clazz = clazz;
}
/**
* Get enum entry corresponding to the specified name
* @param name the name of the entry (case sensitive)
* @return An optionnal with the entry if it exists, empty otherwise.
*/
public Optional getByName(String name){
return EnumConverter.getByName(clazz, name);
}
/**
* Get enum entry corresponding to the specified value.
* @param the type of the value to convert
* @param getterReference the method reference returning the value to check.
* @param value the value to convert.
* @return An optionnal with the entry if the result of the getterReference is equals to the value parameter.
*/
public Optional getByGetter(Function getterReference, E value){
return EnumConverter.getByGetter(clazz, getterReference, value);
}
/**
* Get enum entry corresponding to the specified name
* @param the type of the targeted enum.
* @param clazz the target class. must be an enum.
* @param name the name of the entry (case sensitive)
* @return An optionnal with the entry if it exists, empty otherwise.
*/
public static > Optional getByName(Class clazz, String name){
return getByGetter(clazz, Enum::name, name);
}
/**
* Get enum entry corresponding to the specified value.
* @param the type of the value to convert
* @param the type of the targeted enum.
* @param clazz the target class. must be an enum.
* @param getterReference the method reference returning the value to check.
* @param value the value to convert.
* @return An optionnal with the entry if the result of the getterReference is equals to the value parameter.
*/
public static > Optional getByGetter(Class clazz, Function getterReference, E value){
return Stream.of(clazz.getEnumConstants())
.filter(entry -> getterReference.apply(entry).equals(value))
.findFirst();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy