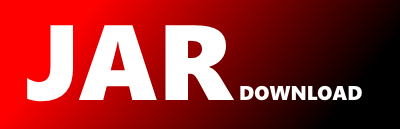
com.github.mathiewz.slick.geom.OverTriangulator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of modernized-slick Show documentation
Show all versions of modernized-slick Show documentation
The main purpose of this libraryis to modernize and maintain the slick2D library.
The newest version!
package com.github.mathiewz.slick.geom;
/**
* A triangulator implementation that splits the triangules of another, subdividing
* to give a higher tesselation - and hence smoother transitions.
*
* @author kevin
*/
public class OverTriangulator implements Triangulator {
/** The triangles data */
private final float[][] triangles;
/**
* Create a new triangulator
*
* @param tris
* The original set of triangles to be sub-dividied
*/
public OverTriangulator(Triangulator tris) {
triangles = new float[tris.getTriangleCount() * 6 * 3][2];
int tcount = 0;
for (int i = 0; i < tris.getTriangleCount(); i++) {
float cx = 0;
float cy = 0;
for (int p = 0; p < 3; p++) {
float[] pt = tris.getTrianglePoint(i, p);
cx += pt[0];
cy += pt[1];
}
cx /= 3;
cy /= 3;
for (int p = 0; p < 3; p++) {
int n = p + 1;
if (n > 2) {
n = 0;
}
float[] pt1 = tris.getTrianglePoint(i, p);
float[] pt2 = tris.getTrianglePoint(i, n);
pt1[0] = (pt1[0] + pt2[0]) / 2;
pt1[1] = (pt1[1] + pt2[1]) / 2;
triangles[tcount * 3 + 0][0] = cx;
triangles[tcount * 3 + 0][1] = cy;
triangles[tcount * 3 + 1][0] = pt1[0];
triangles[tcount * 3 + 1][1] = pt1[1];
triangles[tcount * 3 + 2][0] = pt2[0];
triangles[tcount * 3 + 2][1] = pt2[1];
tcount++;
}
for (int p = 0; p < 3; p++) {
int n = p + 1;
if (n > 2) {
n = 0;
}
float[] pt1 = tris.getTrianglePoint(i, p);
float[] pt2 = tris.getTrianglePoint(i, n);
pt2[0] = (pt1[0] + pt2[0]) / 2;
pt2[1] = (pt1[1] + pt2[1]) / 2;
triangles[tcount * 3 + 0][0] = cx;
triangles[tcount * 3 + 0][1] = cy;
triangles[tcount * 3 + 1][0] = pt1[0];
triangles[tcount * 3 + 1][1] = pt1[1];
triangles[tcount * 3 + 2][0] = pt2[0];
triangles[tcount * 3 + 2][1] = pt2[1];
tcount++;
}
}
}
/**
* @see com.github.mathiewz.slick.geom.Triangulator#addPolyPoint(float, float)
*/
@Override
public void addPolyPoint(float x, float y) {
}
/**
* @see com.github.mathiewz.slick.geom.Triangulator#getTriangleCount()
*/
@Override
public int getTriangleCount() {
return triangles.length / 3;
}
/**
* @see com.github.mathiewz.slick.geom.Triangulator#getTrianglePoint(int, int)
*/
@Override
public float[] getTrianglePoint(int tri, int i) {
float[] pt = triangles[tri * 3 + i];
return new float[] { pt[0], pt[1] };
}
/**
* @see com.github.mathiewz.slick.geom.Triangulator#startHole()
*/
@Override
public void startHole() {
}
/**
* @see com.github.mathiewz.slick.geom.Triangulator#triangulate()
*/
@Override
public boolean triangulate() {
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy