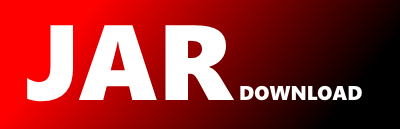
com.github.mathiewz.slick.geom.Point Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of modernized-slick Show documentation
Show all versions of modernized-slick Show documentation
The main purpose of this libraryis to modernize and maintain the slick2D library.
The newest version!
package com.github.mathiewz.slick.geom;
/**
* A single point shape
*
* @author Kova
*/
public class Point extends Shape {
/**
* Create a new point
*
* @param x
* The x coordinate of the point
* @param y
* The y coordinate of the point
*/
public Point(float x, float y) {
this.x = x;
this.y = y;
checkPoints();
}
/**
* @see com.github.mathiewz.slick.geom.Shape#transform(com.github.mathiewz.slick.geom.Transform)
*/
@Override
public Shape transform(Transform transform) {
Float[] result = new Float[points.length];
transform.transform(points, 0, result, 0, points.length / 2);
return new Point(points[0], points[1]);
}
/**
* @see com.github.mathiewz.slick.geom.Shape#createPoints()
*/
@Override
protected void createPoints() {
points = new Float[2];
points[0] = x;
points[1] = y;
maxX = x;
maxY = y;
minX = x;
minY = y;
findCenter();
calculateRadius();
}
/**
* @see com.github.mathiewz.slick.geom.Shape#findCenter()
*/
@Override
protected void findCenter() {
center = new float[2];
center[0] = points[0];
center[1] = points[1];
}
/**
* @see com.github.mathiewz.slick.geom.Shape#calculateRadius()
*/
@Override
protected void calculateRadius() {
boundingCircleRadius = 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy