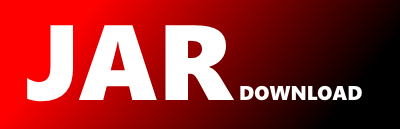
com.github.mathiewz.slick.opengl.CompositeImageData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of modernized-slick Show documentation
Show all versions of modernized-slick Show documentation
The main purpose of this libraryis to modernize and maintain the slick2D library.
The newest version!
package com.github.mathiewz.slick.opengl;
import java.io.BufferedInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import com.github.mathiewz.slick.SlickException;
import com.github.mathiewz.slick.util.Log;
/**
* A composite data source that checks multiple loaders in order of
* preference
*
* @author kevin
*/
public class CompositeImageData implements LoadableImageData {
/** The list of images sources in order of preference to try loading the data with */
private final ArrayList sources = new ArrayList<>();
/** The data source that worked and was used - or null if no luck */
private LoadableImageData picked;
/**
* Add a potentional source of image data
*
* @param data
* The data source to try
*/
public void add(LoadableImageData data) {
sources.add(data);
}
/**
* @see com.github.mathiewz.slick.opengl.LoadableImageData#loadImage(java.io.InputStream)
*/
@Override
public ByteBuffer loadImage(InputStream fis) throws IOException {
return loadImage(fis, false, null);
}
/**
* @see com.github.mathiewz.slick.opengl.LoadableImageData#loadImage(java.io.InputStream, boolean, int[])
*/
@Override
public ByteBuffer loadImage(InputStream fis, boolean flipped, int[] transparent) throws IOException {
return loadImage(fis, flipped, false, transparent);
}
/**
* @see com.github.mathiewz.slick.opengl.LoadableImageData#loadImage(java.io.InputStream, boolean, boolean, int[])
*/
@Override
public ByteBuffer loadImage(InputStream is, boolean flipped, boolean forceAlpha, int[] transparent) throws IOException {
CompositeIOException exception = new CompositeIOException();
ByteBuffer buffer = null;
BufferedInputStream in = new BufferedInputStream(is, is.available());
in.mark(is.available());
// cycle through our source until one of them works
for (int i = 0; i < sources.size(); i++) {
in.reset();
try {
LoadableImageData data = sources.get(i);
buffer = data.loadImage(in, flipped, forceAlpha, transparent);
picked = data;
break;
} catch (Exception e) {
Log.warn(sources.get(i).getClass() + " failed to read the data", e);
exception.addException(e);
}
}
if (picked == null) {
throw exception;
}
return buffer;
}
/**
* Check the state of the image data and throw a
* runtime exception if theres a problem
*/
private void checkPicked() {
if (picked == null) {
throw new SlickException("Attempt to make use of uninitialised or invalid composite image data");
}
}
/**
* @see com.github.mathiewz.slick.opengl.ImageData#getDepth()
*/
@Override
public int getDepth() {
checkPicked();
return picked.getDepth();
}
/**
* @see com.github.mathiewz.slick.opengl.ImageData#getHeight()
*/
@Override
public int getHeight() {
checkPicked();
return picked.getHeight();
}
/**
* @see com.github.mathiewz.slick.opengl.ImageData#getImageBufferData()
*/
@Override
public ByteBuffer getImageBufferData() {
checkPicked();
return picked.getImageBufferData();
}
/**
* @see com.github.mathiewz.slick.opengl.ImageData#getTexHeight()
*/
@Override
public int getTexHeight() {
checkPicked();
return picked.getTexHeight();
}
/**
* @see com.github.mathiewz.slick.opengl.ImageData#getTexWidth()
*/
@Override
public int getTexWidth() {
checkPicked();
return picked.getTexWidth();
}
/**
* @see com.github.mathiewz.slick.opengl.ImageData#getWidth()
*/
@Override
public int getWidth() {
checkPicked();
return picked.getWidth();
}
/**
* @see com.github.mathiewz.slick.opengl.LoadableImageData#configureEdging(boolean)
*/
@Override
public void configureEdging(boolean edging) {
for (int i = 0; i < sources.size(); i++) {
sources.get(i).configureEdging(edging);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy