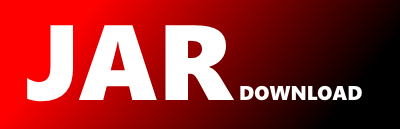
com.github.meazza.handler.RequestHandlerInterceptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-boot-request-copy Show documentation
Show all versions of spring-boot-request-copy Show documentation
Provide Spring MVC @RequestCopy annotation
package com.github.meazza.handler;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.github.meazza.annotation.RequestCopy;
import java.lang.reflect.Method;
import java.util.Optional;
import java.util.Random;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.web.client.RestTemplate;
import org.springframework.web.method.HandlerMethod;
import org.springframework.web.servlet.HandlerInterceptor;
public class RequestHandlerInterceptor implements HandlerInterceptor {
private RestTemplate restTemplate = new RestTemplate();
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler)
throws Exception {
HandlerMethod handlerMethod = (HandlerMethod) handler;
Method method = handlerMethod.getMethod();
RequestCopy requestCopy = method.getAnnotation(RequestCopy.class);
if (requestCopy != null) {
String url = requestCopy.url();
float ratio = requestCopy.ratio();
if (new Random().nextFloat() <= ratio) {
switch (request.getMethod()) {
case "GET": {
new Thread(() -> {
String result = restTemplate.getForObject(
url + "?" + Optional.ofNullable(request.getQueryString()).orElse(""),
String.class);
System.out.println(result);
}).run();
break;
}
case "POST": {
switch (request.getHeader("Content-Type")) {
case "application/json": {
RequestBodyWrapper requestWrapper = new RequestBodyWrapper(request);
JSONObject jsonObject = JSON.parseObject(requestWrapper.getBody());
if (jsonObject != null) {
new Thread(() -> {
String result = restTemplate.postForObject(
url + "?" + Optional.ofNullable(request.getQueryString()).orElse(""),
jsonObject, String.class);
System.out.println(result);
}).run();
}
break;
}
}
break;
}
}
}
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy