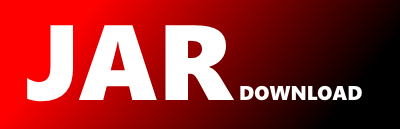
com.github.mfathi91.time.PersianDateTime Maven / Gradle / Ivy
Show all versions of persian-date-time Show documentation
package com.github.mfathi91.time;
import java.time.*;
import java.time.chrono.ChronoLocalDateTime;
import java.time.chrono.ChronoZonedDateTime;
import java.time.temporal.*;
import java.util.Objects;
import static java.time.temporal.ChronoField.*;
/**
*
*/
public final class PersianDateTime implements ChronoLocalDateTime {
/**
* The minimum supported {@code PersianDateTime}, '01-01-01T00:00:00'.
* This is the local date-time of midnight at the start of the minimum date.
* This combines {@link PersianDate#MIN} and {@link LocalTime#MIN}.
*/
public static final PersianDateTime MIN = PersianDateTime.of(PersianDate.MIN, LocalTime.MIN);
/**
* The maximum supported {@code PersianDateTime}, '1999-12-29T23:59:59'.
* This is the persian date-time just before midnight at the end of the maximum date.
* This combines {@link PersianDate#MAX} and {@link LocalTime#MAX}.
*/
public static final PersianDateTime MAX = PersianDateTime.of(PersianDate.MAX, LocalTime.MAX);
/**
* The date part.
*/
private final PersianDate date;
/**
* The time part.
*/
private final LocalTime time;
//-----------------------------------------------------------------------
/**
* @return
*/
public static PersianDateTime now() {
return new PersianDateTime(PersianDate.ofGregorian(LocalDate.now()), LocalTime.now());
}
/**
* @param year
* @param month
* @param dayOfMonth
* @param hour
* @param minute
* @param secodn
* @param nanoSecond
* @return
*/
public static PersianDateTime of(int year, PersianMonth month, int dayOfMonth, int hour,
int minute, int secodn, int nanoSecond) {
PersianDate persianDate = PersianDate.of(year, month, dayOfMonth);
LocalTime localTime = LocalTime.of(hour, minute, secodn, nanoSecond);
return new PersianDateTime(persianDate, localTime);
}
/**
* @param year
* @param month
* @param dayOfMonth
* @param hour
* @param minute
* @param second
* @return
*/
public static PersianDateTime of(int year, PersianMonth month, int dayOfMonth,
int hour, int minute, int second) {
return of(year, month, dayOfMonth, hour, minute, second, 0);
}
/**
* @param year
* @param month
* @param dayOfMonth
* @param hour
* @param minute
* @return
*/
public static PersianDateTime of(int year, PersianMonth month, int dayOfMonth,
int hour, int minute) {
return of(year, month, dayOfMonth, hour, minute, 0, 0);
}
/**
* @param year
* @param month
* @param dayOfMonth
* @param hour
* @param minute
* @param second
* @param nanoSecond
* @return
*/
public static PersianDateTime of(int year, int month, int dayOfMonth,
int hour, int minute, int second, int nanoSecond) {
return of(year, PersianMonth.of(month), dayOfMonth, hour, minute, second, nanoSecond);
}
/**
* @param year
* @param month
* @param dayOfMonth
* @param hour
* @param minute
* @param second
* @return
*/
public static PersianDateTime of(int year, int month, int dayOfMonth,
int hour, int minute, int second) {
return of(year, PersianMonth.of(month), dayOfMonth, hour, minute, second, 0);
}
public static PersianDateTime of(int year, int month, int dayOfMonth, int hour, int minute) {
return of(year, month, dayOfMonth, hour, minute, 0, 0);
}
/**
* @param persianDate
* @param localTime
* @return
*/
public static PersianDateTime of(PersianDate persianDate, LocalTime localTime) {
return new PersianDateTime(persianDate, localTime);
}
/**
* @param persianDate
* @param localTime
*/
private PersianDateTime(PersianDate persianDate, LocalTime localTime) {
this.date = Objects.requireNonNull(persianDate, "persianDate");
this.time = Objects.requireNonNull(localTime, "localTime");
}
//-----------------------------------------------------------------------
public static PersianDateTime ofGregorian(LocalDateTime gregDateTime) {
Objects.requireNonNull(gregDateTime, "gregDateTime");
return new PersianDateTime(PersianDate.ofGregorian(gregDateTime.toLocalDate()),
gregDateTime.toLocalTime());
}
public LocalDateTime toGregorian() {
return LocalDateTime.from(this);
}
//-----------------------------------------------------------------------
/**
* Gets the {@code PersianDate} part of this date-time.
*
* This returns a {@code PersianDate} with the same year, month and day
* as this date-time.
*
* @return the date part of this date-time, not null
*/
@Override
public PersianDate toLocalDate() {
return date;
}
/**
* @return the year
*/
public int getYear() {
return date.getYear();
}
/**
* Gets month-of-year field using {@link PersianMonth} enum. To get the
* numerical value of month, one should call {@link #getMonthValue()}.
*
* @return month-of-year, not null
* @see #getMonthValue()
*/
public PersianMonth getMonth() {
return date.getMonth();
}
/**
* Gets month-of-year from 1 to 12. Number 1 represents Farvardin and
* number 12 represents Esfand. One may use {@link #getMonth()} for more
* readability.
*
* @return month-of-year, from 1 to 12
* @see #getMonth()
*/
public int getMonthValue() {
return date.getMonthValue();
}
/**
* @return day-of-month, from 1 to 31
*/
public int getDayOfMonth() {
return date.getDayOfMonth();
}
/**
* @return day-of-year, from 1 to 365 or 366 in a leap year
*/
public int getDayOfYear() {
return date.getDayOfYear();
}
/**
* Returns day-of-week as an enum {@link DayOfWeek}. This avoids confusion as to what
* {@code int} means. If you need access to the primitive {@code int} value then the
* enum provides the {@link DayOfWeek#getValue() int value}.
*
* @return day-of-week, which is an enum {@link DayOfWeek}
*/
public DayOfWeek getDayOfWeek() {
return date.getDayOfWeek();
}
//-----------------------------------------------------------------------
/**
* Gets the {@code LocalTime} part of this date-time.
*
* This returns a {@code LocalTime} with the same hour, minute, second and
* nanosecond as this date-time.
*
* @return the time part of this date-time, not null
*/
@Override
public LocalTime toLocalTime() {
return time;
}
/**
* Gets the hour-of-day field.
*
* @return the hour-of-day, from 0 to 23
*/
public int getHour() {
return time.getHour();
}
/**
* Gets the minute-of-hour field.
*
* @return the minute-of-hour, from 0 to 59
*/
public int getMinute() {
return time.getMinute();
}
/**
* Gets the second-of-minute field.
*
* @return the second-of-minute, from 0 to 59
*/
public int getSecond() {
return time.getSecond();
}
/**
* Gets the nano-of-second field.
*
* @return the nano-of-second, from 0 to 999,999,999
*/
public int getNano() {
return time.getNano();
}
//-----------------------------------------------------------------------
@Override
public boolean isSupported(TemporalField field) {
if (field instanceof ChronoField) {
ChronoField f = (ChronoField) field;
return f.isDateBased() || f.isTimeBased();
}
return field != null && field.isSupportedBy(this);
}
@Override
public long getLong(TemporalField field) {
if (field instanceof ChronoField) {
ChronoField f = (ChronoField) field;
return (f.isTimeBased() ? time.getLong(field) : date.getLong(field));
}
return field.getFrom(this);
}
@Override
public ChronoLocalDateTime with(TemporalField field, long newValue) {
return null;
}
@Override
public ChronoLocalDateTime plus(long amountToAdd, TemporalUnit unit) {
return null;
}
@Override
public long until(Temporal endExclusive, TemporalUnit unit) {
return 0;
}
@Override
public ChronoZonedDateTime atZone(ZoneId zone) {
return null;
}
//-----------------------------------------------------------------------
/**
* Checks if this date-time is equal to another persian-date-time.
*
* Compares this {@code PersianDateTime} with another ensuring that the date-time is the same.
* Only objects of type {@code PersianDateTime} are compared, other types return false.
*
* @param obj the object to check, null returns false
* @return true if this is equal to the other persian-date-time
*/
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj instanceof PersianDateTime) {
PersianDateTime other = (PersianDateTime) obj;
return date.equals(other.date) && time.equals(other.time);
}
return false;
}
/**
* A hash code for this date-time.
*
* @return a suitable hash code
*/
@Override
public int hashCode() {
return date.hashCode() ^ time.hashCode();
}
//-----------------------------------------------------------------------
/**
* Outputs this date-time as a {@code String}, such as {@code 1396-12-03T10:15:30}.
*
* The output will be one of the following ISO-8601 formats:
*
* - {@code uuuu-MM-dd'T'HH:mm}
* - {@code uuuu-MM-dd'T'HH:mm:ss}
* - {@code uuuu-MM-dd'T'HH:mm:ss.SSS}
* - {@code uuuu-MM-dd'T'HH:mm:ss.SSSSSS}
* - {@code uuuu-MM-dd'T'HH:mm:ss.SSSSSSSSS}
*
* The format used will be the shortest that outputs the full value of
* the time where the omitted parts are implied to be zero.
*
* @return a string representation of this date-time, not null
*/
@Override
public String toString() {
return date.toString() + 'T' + time.toString();
}
}