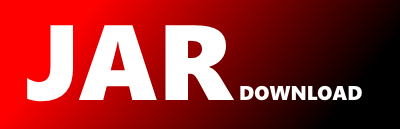
nats.codec.AbstractFrameDecoder Maven / Gradle / Ivy
/*
* Copyright (c) 2013 Mike Heath. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package nats.codec;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.MessageList;
import io.netty.handler.codec.ReplayingDecoder;
import io.netty.handler.codec.TooLongFrameException;
import io.netty.util.CharsetUtil;
import nats.Constants;
/**
* @author Mike Heath
*/
abstract class AbstractFrameDecoder extends ReplayingDecoder {
private final int maxMessageSize;
protected AbstractFrameDecoder() {
this(Constants.DEFAULT_MAX_FRAME_SIZE);
}
protected AbstractFrameDecoder(int maxMessageSize) {
this.maxMessageSize = maxMessageSize;
}
@Override
protected void decode(ChannelHandlerContext ctx, ByteBuf in, MessageList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy