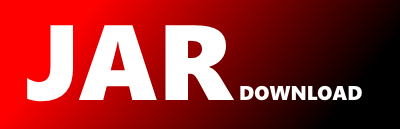
com.github.mhendred.face4j.response.ResponseHelper Maven / Gradle / Ivy
package com.github.mhendred.face4j.response;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import com.github.mhendred.face4j.model.Group;
import com.github.mhendred.face4j.model.Namespace;
import com.github.mhendred.face4j.model.Photo;
import com.github.mhendred.face4j.model.RemovedTag;
import com.github.mhendred.face4j.model.SavedTag;
import com.github.mhendred.face4j.model.UserStatus;
/**
* This class contains static methods to convert JSON
* responses into model classes. This could be done using
* reflection but isn't for performance reasons.
*
* @author mhendred
*
*/
final class ResponseHelper
{
// No Instances
private ResponseHelper ()
{
throw new AssertionError();
}
static List toSavedTagList (final JSONArray jArr) throws JSONException
{
final List savedTags = new ArrayList();
if (jArr != null)
{
for (int i = 0; i < jArr.length(); i++)
{
savedTags.add(new SavedTag(jArr.getJSONObject(i)));
}
}
return savedTags;
}
static List toPhotoList (JSONArray jArr) throws JSONException
{
final List photos = new ArrayList();
for (int i = 0; i < jArr.length(); i++)
{
photos.add(new Photo(jArr.getJSONObject(i)));
}
return photos;
}
static List toUserStatusList (JSONArray jArr) throws JSONException
{
final List status = new LinkedList();
if (jArr != null)
{
for (int i = 0; i < jArr.length(); i++)
{
status.add(new UserStatus(jArr.getJSONObject(i)));
}
}
return status;
}
static List toRemovedTagList (JSONArray jArr) throws JSONException
{
final List removedTags = new LinkedList();
for (int i = 0; i < jArr.length(); i++)
{
removedTags.add(new RemovedTag(jArr.getJSONObject(i)));
}
return removedTags;
}
static List toGroupList (JSONArray jArr) throws JSONException
{
final List groups = new ArrayList();
for (int i = 0; i < jArr.length(); i++)
{
groups.add(new Group(jArr.getJSONObject(i)));
}
return groups;
}
static List toStringList (JSONArray jArr) throws JSONException
{
final List strings = new ArrayList();
for (int i = 0; i < jArr.length(); i++)
{
strings.add(jArr.getString(i));
}
return strings;
}
static List toNamespaceList (JSONArray jArr) throws JSONException
{
final List namespaces = new ArrayList();
for (int i = 0; i < jArr.length(); i++)
{
namespaces.add(new Namespace(jArr.getJSONObject(i)));
}
return namespaces;
}
static int optInt (JSONObject jObj, String name) throws JSONException
{
return jObj.isNull(name) ? -1 : jObj.getInt(name);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy