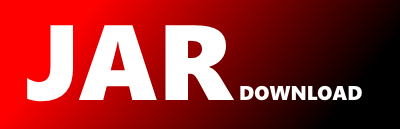
com.github.mike10004.nativehelper.subprocess.BasicProcessMonitor Maven / Gradle / Ivy
package com.github.mike10004.nativehelper.subprocess;
import com.github.mike10004.nativehelper.subprocess.Subprocess.ProcessExecutionException;
import com.google.common.util.concurrent.ListenableFuture;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import static java.util.Objects.requireNonNull;
public class BasicProcessMonitor implements ProcessMonitor {
private final Process process;
private final ListenableFuture> future;
private final ProcessTracker processTracker;
BasicProcessMonitor(Process process, ListenableFuture> future, ProcessTracker processTracker) {
this.future = requireNonNull(future);
this.process = requireNonNull(process);
this.processTracker = requireNonNull(processTracker);
}
public ListenableFuture> future() {
return future;
}
public ProcessDestructor destructor() {
return new BasicProcessDestructor(process, processTracker);
}
public Process process() {
return process;
}
public ProcessTracker tracker() {
return processTracker;
}
public static class ProcessExecutionInnerException extends ProcessExecutionException {
public ProcessExecutionInnerException(Throwable cause) {
super(cause);
}
}
public ProcessResult await(long timeout, TimeUnit unit) throws TimeoutException, InterruptedException, ProcessExecutionInnerException {
try {
boolean ok = process.waitFor(timeout, unit);
if (!ok) {
throw new TimeoutException("process.waitFor timeout elapsed");
}
return future().get();
} catch (ExecutionException e) {
throw new ProcessExecutionInnerException(e.getCause());
}
}
public ProcessResult await() throws ProcessException, InterruptedException {
try {
return future().get();
} catch (ExecutionException e) {
throw new ProcessExecutionInnerException(e.getCause());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy