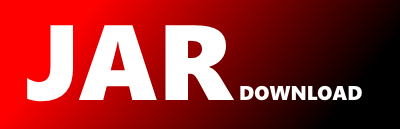
io.github.mike10004.vhs.StatefulHeuristicEntryMatcher Maven / Gradle / Ivy
package io.github.mike10004.vhs;
import com.google.common.collect.ImmutableMap;
import com.google.common.primitives.Ints;
import com.google.common.util.concurrent.AtomicLongMap;
import io.github.mike10004.vhs.harbridge.HttpMethod;
import io.github.mike10004.vhs.harbridge.ParsedRequest;
import org.apache.commons.lang3.tuple.ImmutablePair;
import org.apache.commons.lang3.tuple.Pair;
import javax.annotation.Nullable;
import java.io.IOException;
import java.net.URI;
import java.util.Collection;
import java.util.List;
import java.util.function.Function;
public class StatefulHeuristicEntryMatcher extends HeuristicEntryMatcher {
private static final int SEQUENCE_MATCH_BOOST = BasicHeuristic.DEFAULT_INCREMENT;
private final ImmutableMap entrySequencePositions;
public StatefulHeuristicEntryMatcher(Heuristic heuristic, int thresholdExclusive, Collection entries) {
super(heuristic, thresholdExclusive, entries);
this.entrySequencePositions = findSequencePositions(entries);
}
private static ImmutableMap findSequencePositions(Iterable entries) {
ImmutableMap.Builder b = ImmutableMap.builder();
AtomicLongMap> counter = AtomicLongMap.create();
for (ParsedEntry entry : entries) {
int sequencePosition = Ints.saturatedCast(counter.getAndIncrement(ImmutablePair.of(entry.request.method, entry.request.url)));
b.put(entry, sequencePosition);
}
return b.build();
}
@Override
protected Function createEntryToRatingFunction(ParsedRequest request, ReplaySessionState state) {
return entry -> {
int rating = heuristic.rate(entry.request, request);
int boost = 0;
if (rating > 0) {
int entrySequencePosition = entrySequencePositions.get(entry);
int requestSequencePosition = state.query(request);
boost = entrySequencePosition == requestSequencePosition ? SEQUENCE_MATCH_BOOST : 0;
}
return new RatedEntry(entry, rating + boost);
};
}
public static EntryMatcherFactory factory(Heuristic heuristic, int thresholdExclusive) {
return new MyFactory(heuristic, thresholdExclusive);
}
protected static class MyFactory extends Factory {
public MyFactory(Heuristic heuristic, int thresholdExclusive) {
super(heuristic, thresholdExclusive);
}
@Override
public EntryMatcher createEntryMatcher(List entries, EntryParser requestParser) throws IOException {
List parsedEntries = parseEntries(entries, requestParser);
return new StatefulHeuristicEntryMatcher(heuristic, thresholdExclusive, parsedEntries);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy