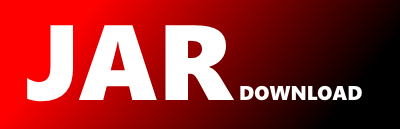
com.github.mike10004.xvfbmanager.ListTreeNode Maven / Gradle / Ivy
/*
* (c) 2016 Mike Chaberski
*
* Created by mike
*/
package com.github.mike10004.xvfbmanager;
import com.google.common.base.Function;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Iterables;
import com.google.common.collect.Iterators;
import javax.annotation.concurrent.NotThreadSafe;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.google.common.base.Preconditions.checkState;
/**
* Implementation of a tree node that uses an array list for its children.
* @param the label type
*/
@NotThreadSafe
public class ListTreeNode implements TreeNode {
private TreeNode parent;
private final T label;
private List> children;
public ListTreeNode(T label) {
this.label = checkNotNull(label, "label must be non-null");
children = ImmutableList.of();
}
@Override
public T getLabel() {
return label;
}
@Override
public Iterable> children() {
List> children_ = children;
if (children_ instanceof ImmutableList) {
return children_;
} else {
return Collections.unmodifiableList(children);
}
}
@Override
public int getLevel() {
int level = 0;
TreeNode node = parent;
while (node != null) {
level++;
node = node.getParent();
}
return level;
}
@Override
public int getChildCount() {
return children.size();
}
@Override
public TreeNode getParent() {
return parent;
}
@Override
public TreeNode setParent(TreeNode parent) {
TreeNode oldParent = this.parent;
this.parent = parent;
checkState(Iterables.contains(parent.children(), this), "setParent can only be called on child nodes");
return oldParent;
}
@Override
public ListTreeNode addChild(TreeNode child) {
List> children_ = children;
if (children_ instanceof ImmutableList) {
this.children = children_ = new ArrayList(children_);
}
children_.add(child);
child.setParent(this);
return this;
}
@Override
public boolean isRoot() {
return parent == null;
}
@Override
public Iterator iterator() {
Function, T> labelGetter = TreeNode.Utils.labelFunction();
return Iterators.transform(TreeNode.Utils.traverser().breadthFirstTraversal(this).iterator(), labelGetter);
}
@Override
public boolean isLeaf() {
return getChildCount() == 0;
}
@Override
public String toString() {
int childCount = getChildCount();
boolean leaf = childCount == 0;
return "ListTreeNode{" +
(isRoot() ? "root" : (leaf ? "leaf" : "node")) +
", label=" + label +
", children.size=" + childCount +
'}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy