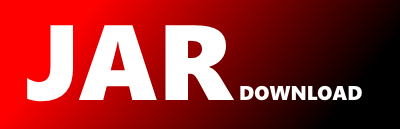
com.thebuzzmedia.exiftool.commons.iterables.Collections Maven / Gradle / Ivy
The newest version!
/**
* Copyright 2011 The Buzz Media, LLC
* Copyright 2015-2019 Mickael Jeanroy
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.thebuzzmedia.exiftool.commons.iterables;
import java.util.ArrayList;
import java.util.Collection;
import java.util.stream.Collectors;
import java.util.stream.StreamSupport;
/**
* Static Collection Utilities.
*/
public final class Collections {
// Ensure non instantiation.
private Collections() {
}
/**
* Check if collection is empty ({@code null} or empty).
*
* @param values Collection of values.
* @param Type of element in collection.
* @return {@code true} if collection is empty ({@code null} or empty), {@code false} otherwise.
*/
public static boolean isEmpty(Collection values) {
return values == null || values.isEmpty();
}
/**
* Check if collection is not empty (not {@code null} and not empty).
*
* @param values Collection of values.
* @param Type of element in collection.
* @return {@code true} if collection is not empty (not {@code null} and not empty), {@code false} otherwise.
*/
public static boolean isNotEmpty(Collection values) {
return !isEmpty(values);
}
/**
* Get size of collection.
* If collection is empty (null or empty), zero will be returned.
*
* @param values Collection of values.
* @param Type of element in collection.
* @return Size of collection.
*/
public static int size(Collection values) {
return values == null ? 0 : values.size();
}
/**
* Create in memory collection from given iterable elements.
*
*
* - If {@code iterables} is null, an empty array list is returned.
* - If {@code iterables} is already an instance of {@link Collection}, it is returned.
* - Otherwise, iterable structure is iterated and loaded into an {@link ArrayList}.
*
*
* @param iterables Given iterable.
* @param Type of element in iterable structure.
* @return The collection result.
*/
public static Collection toCollection(Iterable iterables) {
if (iterables == null) {
return new ArrayList<>(0);
}
if (iterables instanceof Collection) {
return (Collection) iterables;
}
return StreamSupport.stream(iterables.spliterator(), false).collect(Collectors.toList());
}
/**
* Add all elements of given iterable structure to given collection.
*
* @param collection The collection.
* @param iterable The iterable structure.
* @param Type of elements.
*/
public static void addAll(Collection collection, Iterable iterable) {
if (iterable == null || collection == null) {
return;
}
for (T value : iterable) {
collection.add(value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy