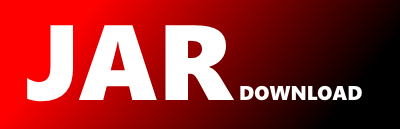
com.github.mkopylec.charon.configuration.RequestMappingConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of charon-spring-webflux Show documentation
Show all versions of charon-spring-webflux Show documentation
Reverse proxy implementation in form of Spring Boot starter
The newest version!
package com.github.mkopylec.charon.configuration;
import com.github.mkopylec.charon.forwarding.WebClientConfiguration;
import com.github.mkopylec.charon.forwarding.interceptors.RequestForwardingInterceptor;
import com.github.mkopylec.charon.forwarding.interceptors.RequestForwardingInterceptorType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Pattern;
import static java.util.Collections.sort;
import static java.util.Collections.unmodifiableList;
import static java.util.regex.Pattern.compile;
import static java.util.stream.Collectors.toList;
import static org.springframework.util.Assert.hasText;
public class RequestMappingConfiguration implements Valid {
private String name;
private Pattern pathRegex;
private WebClientConfiguration webClientConfiguration;
private List requestForwardingInterceptors;
private List unsetRequestForwardingInterceptors;
private RequestMappingConfigurer requestMappingConfigurer;
RequestMappingConfiguration(String name) {
this.name = name;
pathRegex = compile("/.*");
requestForwardingInterceptors = new ArrayList<>();
unsetRequestForwardingInterceptors = new ArrayList<>();
}
@Override
public void validate() {
hasText(name, "No request forwarding name set");
}
public String getName() {
return name;
}
public Pattern getPathRegex() {
return pathRegex;
}
void setPathRegex(String pathRegex) {
this.pathRegex = compile(pathRegex);
}
public WebClientConfiguration getWebClientConfiguration() {
return webClientConfiguration;
}
void setWebClientConfiguration(WebClientConfiguration webClientConfiguration) {
this.webClientConfiguration = webClientConfiguration;
}
void mergeWebClientConfiguration(WebClientConfiguration webClientConfiguration) {
if (this.webClientConfiguration == null) {
this.webClientConfiguration = webClientConfiguration;
}
}
public List getRequestForwardingInterceptors() {
return unmodifiableList(requestForwardingInterceptors);
}
void addRequestForwardingInterceptor(RequestForwardingInterceptor requestForwardingInterceptor) {
removeRequestForwardingInterceptor(requestForwardingInterceptors, requestForwardingInterceptor.getType());
unsetRequestForwardingInterceptors.remove(requestForwardingInterceptor.getType());
requestForwardingInterceptors.add(requestForwardingInterceptor);
}
void removeRequestForwardingInterceptor(RequestForwardingInterceptorType requestForwardingInterceptorType) {
removeRequestForwardingInterceptor(requestForwardingInterceptors, requestForwardingInterceptorType);
unsetRequestForwardingInterceptors.add(requestForwardingInterceptorType);
}
void mergeRequestForwardingInterceptors(List requestForwardingInterceptors) {
List additionalInterceptors = requestForwardingInterceptors.stream()
.filter(interceptor -> this.requestForwardingInterceptors.stream().noneMatch(i -> i.getType().equals(interceptor.getType())))
.filter(interceptor -> unsetRequestForwardingInterceptors.stream().noneMatch(i -> i.equals(interceptor.getType())))
.collect(toList());
this.requestForwardingInterceptors.addAll(additionalInterceptors);
sort(this.requestForwardingInterceptors);
}
RequestMappingConfigurer getRequestMappingConfigurer() {
return requestMappingConfigurer;
}
void setRequestMappingConfigurer(RequestMappingConfigurer requestMappingConfigurer) {
this.requestMappingConfigurer = requestMappingConfigurer;
}
private void removeRequestForwardingInterceptor(List requestForwardingInterceptors, RequestForwardingInterceptorType requestForwardingInterceptorType) {
requestForwardingInterceptors.removeIf(interceptor -> interceptor.getType().equals(requestForwardingInterceptorType));
}
@Override
public String toString() {
return "'" + name + "'";
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj.getClass() != getClass()) {
return false;
}
RequestMappingConfiguration rhs = (RequestMappingConfiguration) obj;
return new EqualsBuilder()
.append(this.name, rhs.name)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder()
.append(name)
.toHashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy