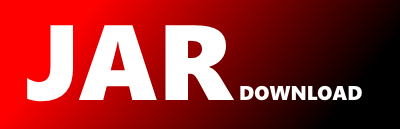
com.github.moaxcp.x11client.protocol.X11Output Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of x11-client Show documentation
Show all versions of x11-client Show documentation
An x11 client implemented in java
package com.github.moaxcp.x11client.protocol;
import java.io.IOException;
import java.util.List;
import java.util.stream.IntStream;
import static java.util.stream.Collectors.toList;
public interface X11Output {
void writeBool(boolean bool) throws IOException;
void writeBool(List bool) throws IOException;
void writeByte(byte b) throws IOException;
void writeInt8(byte int8) throws IOException;
void writeInt16(short int16) throws IOException;
void writeInt32(int int32) throws IOException;
void writeInt32(List int32) throws IOException;
void writeInt64(long int64) throws IOException;
void writeCard8(byte card8) throws IOException;
void writeCard8(List card8) throws IOException;
void writeCard16(short card16) throws IOException;
void writeCard16(List card16) throws IOException;
void writeCard32(int card32) throws IOException;
void writeCard32(List card32) throws IOException;
void writeCard64(long card64) throws IOException;
void writeCard64(List card64) throws IOException;
void writeFloat(float f) throws IOException;
void writeFloat(List f) throws IOException;
void writeDouble(double d) throws IOException;
void writeDouble(List d) throws IOException;
void writeChar(List string) throws IOException;
void writeString8(String string) throws IOException;
void writeByte(List bytes) throws IOException;
void writeVoid(List bytes) throws IOException;
void writeFd(int fd) throws IOException;
void writeFd(List fd) throws IOException;
default void writePad(int length) throws IOException {
writeByte(IntStream.range(0, length).mapToObj(i -> (byte) 0).collect(toList()));
}
default void writePadAlign(int forLength) throws IOException {
writePad(XObject.getSizeForPadAlign(forLength));
}
default void writePadAlign(int pad, int forLength) throws IOException {
writePad(XObject.getSizeForPadAlign(pad, forLength));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy