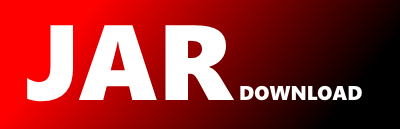
com.github.mrivanplays.bungee.messaging.api.BungeeMessagingAPI Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of BungeeMessagingAPI Show documentation
Show all versions of BungeeMessagingAPI Show documentation
BungeeCord Plugin Messaging API
The newest version!
/*
* Copyright 2019 Ivan Pekov
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NON INFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.github.mrivanplays.bungee.messaging.api;
import org.bukkit.Bukkit;
import org.bukkit.entity.Player;
import java.net.InetSocketAddress;
import java.util.List;
import java.util.concurrent.CompletableFuture;
/** Represents the API itself */
@SuppressWarnings("unused")
public interface BungeeMessagingAPI {
/**
* Sets a global listener for all 'forwarded' messages
*
* @param globalListener set listener
* @see Spgot
*/
void registerForwardListener(ForwardConsumer globalListener);
/**
* Sets a listener for all 'forwarded' messages in the specified subChannel
*
* @param subChannel the subChannel
* @param listener set listener
* @see Spigot
*/
void registerForwardListener(String subChannel, ForwardConsumer listener);
/**
* Gets the amount of players in the specified server. If the server name equals "ALL" or it will
* get the players on all servers. If the server name is null, the method will get the players on
* all servers.
*
* @param serverName specified server or "ALL"/"all" for all players on the servers.
* @return a {@link CompletableFuture} that when completed will return an {@link Integer}
* @throws NullPointerException if there are no players globally.
*/
CompletableFuture getPlayerCount(String serverName);
/**
* Gets the player names as {@link List} on the specified server name. If server name equals "ALL"
* it will get the player names on all servers. If the server name
*
* @param serverName specified server or "ALL"/"all" for all player names on the servers.
* @return a {@link CompletableFuture} that when completed will return an {@link List} of {@link
* String} (player names)
* @throws NullPointerException if there are no players globally.
*/
CompletableFuture> getPlayerList(String serverName);
/**
* Gets the server names as {@link List}, as defined in the BungeeCord config
*
* @return a {@link CompletableFuture} that when completed will return an {@link List} of {@link
* String} (server names)
* @throws NullPointerException if there are no players globally.
*/
CompletableFuture> getServerNames();
/**
* Connects the specified {@link Player} to the specified serverName. In this case the message
* will be sent to the {@link Player} unlike the {@link #connectOther(Player, String)}
*
* @param player connected player
* @param serverName server, connected to
* @throws NullPointerException if the player or the server is null
*/
void connect(Player player, String serverName);
/**
* Connects the specified {@link Player} to the specified server name
*
* @param player connected player
* @param serverName server, connected to
* @throws NullPointerException if the player or server is null
*/
void connectOther(Player player, String serverName);
/**
* Connects the specified player's name to the specified server name
*
* @param playerName connected player's name
* @param serverName server, connected to
* @throws NullPointerException if the player or server is null
*/
default void connectOther(String playerName, String serverName) {
connectOther(Bukkit.getPlayerExact(playerName), serverName);
}
/**
* Gets the specified {@link Player}'s (real) IP address
*
* @param player the player you wish to get the IP of
* @return a {@link CompletableFuture} that when completed will return an {@link
* InetSocketAddress} (the player IP address)
* @throws NullPointerException if the player is null
*/
CompletableFuture getIPAddress(Player player);
/**
* Gets the specified player's name's (real) IP address
*
* @param playerName the player's name you wish to get the IP of
* @return a {@link CompletableFuture} that when completed will return an {@link
* InetSocketAddress} (the player IP address)
* @throws NullPointerException if the player is null
*/
default CompletableFuture getIPAddress(String playerName) {
return getIPAddress(Bukkit.getPlayerExact(playerName));
}
/**
* Sends a message to the specified {@link Player}
*
* @param player the player you wish to message
* @param message sent message
* @throws NullPointerException if the player is null
*/
void sendMessage(Player player, String message);
/**
* Sends a message to the specified player name
*
* @param playerName the player's name you wish to message
* @param message sent message
* @throws NullPointerException if the player is null
*/
default void sendMessage(String playerName, String message) {
sendMessage(Bukkit.getPlayerExact(playerName), message);
}
/**
* Get this server's name, as defined in the BungeeCord config
*
* @return a {@link CompletableFuture} that when completed will return {@link String} (the server
* name)
* @throws NullPointerException if there are no players online
*/
CompletableFuture getServer();
/**
* Gets the specified {@link Player}'s Unique ID in {@link String}
*
* @param player the player you want to get the UUID
* @return a {@link CompletableFuture} that when completed will return {@link String} (the player
* {@link java.util.UUID} in {@link String})
*/
CompletableFuture getUUID(Player player);
/**
* Gets the specified player's name's Unique ID in {@link String}
*
* @param playerName the player's name you want to get the UUID
* @return a {@link CompletableFuture} that when completed will return {@link String} (the player
* {@link java.util.UUID} in {@link String})
*/
default CompletableFuture getUUID(String playerName) {
return getUUID(Bukkit.getPlayerExact(playerName));
}
/**
* Gets the specified {@link Player}'s Unique ID in {@link String}
*
* @param player the player you want to get the UUID
* @return a {@link CompletableFuture} that when completed will return {@link String} (the player
* {@link java.util.UUID} in {@link String})
* @throws NullPointerException if there are no players online
*/
CompletableFuture getUUIDOther(Player player);
/**
* Gets the specified player's name's Unique ID in {@link String}
*
* @param playerName the player's name you want to get the UUID
* @return a {@link CompletableFuture} that when completed will return {@link String} (the player
* {@link java.util.UUID} in {@link String})
* @throws NullPointerException if there are no players online
*/
default CompletableFuture getUUIDOther(String playerName) {
return getUUIDOther(Bukkit.getPlayerExact(playerName));
}
/**
* Gets the specified server's name's IP
*
* @param serverName the server's name you want to get the IP as specified in the BungeeCord
* config
* @return a {@link CompletableFuture} that when completed will return an {@link
* InetSocketAddress} (the server IP address)
* @throws NullPointerException if there are no players online in the specified server
*/
CompletableFuture getServerIP(String serverName);
/**
* Kicks the specified {@link Player} with the specified kick reason
*
* @param player the player you want to kick
* @param kickReason the kick reason
* @throws NullPointerException if the player is null (not online)
*/
void kickPlayer(Player player, String kickReason);
/**
* Kicks the specified player's name with the specified kick reason
*
* @param playerName the player's name you want to kick
* @param kickReason the kick reason
* @throws NullPointerException if the player is null (not online)
*/
default void kickPlayer(String playerName, String kickReason) {
kickPlayer(Bukkit.getPlayerExact(playerName), kickReason);
}
/**
* Sends a custom plugin message to the specified server. This is one of the most useful channels
* ever (not gonna lie). Remember, the sending and receiving server(s) need to have a {@link
* Player} online
*
* @param server the name of the server send to as specified in the BungeeCord config, "ALL" or
* "all" to send it on every server (except the one sending the message), or ONLINE to send to
* every server that's online (except the one sending the message)
* @param channelName the subchannel for plugin usage
* @param data sent data
* @throws NullPointerException if the specified server is null / if the data is null / if there
* are no players online.
*/
void forward(String server, String channelName, byte[] data);
/**
* Sends a custom plugin message to the specified {@link Player}. This is one of the most useful
* channels ever (not gonna lie).
*
* @param player the player send to
* @param channelName subchannel for plugin usage
* @param data sent data
* @throws NullPointerException if the data is null / if the player is null
*/
void forwardToPlayer(Player player, String channelName, byte[] data);
String toString();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy