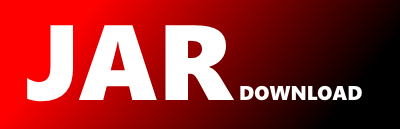
com.github.mrivanplays.acf.api.ACFCommand Maven / Gradle / Ivy
Show all versions of IvanACF Show documentation
/*
* *
* * Copyright 2018-2019 MrIvanPlays
* *
* * Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"),
* * to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense,
* * and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
* *
* * The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
* *
* * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
* * IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
* * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*
*/
package com.github.mrivanplays.acf.api;
import com.github.mrivanplays.acf.util.Comment;
import org.apache.commons.lang.Validate;
import org.bukkit.ChatColor;
import org.bukkit.command.*;
import org.bukkit.plugin.Plugin;
import java.util.List;
@Comment("Represents command")
/**
* Represents a command that
* does the things we have here.
*/
public abstract class ACFCommand extends Command implements CommandExecutor, PluginIdentifiableCommand {
/**
* The plugin's where
* command is assigned to
*/
private Plugin plugin;
/**
* The tab completer
* that is assigned to
* the specified command
*/
private TabCompleter completer;
/**
* Command's creation method
*
* @param plugin plugin assigned to
* @param name command name
*/
public ACFCommand(Plugin plugin, String name){
super(name);
this.plugin = plugin;
}
/**
* Gets the plugin where
* the command is assigned
* to
*
* @return plugin
*/
@Override
public Plugin getPlugin(){
return plugin;
}
/**
* Sets the specified command a tab completer
*
* @param tabCompleter set tab completer
*/
public void setTabCompleter(TabCompleter tabCompleter){
this.completer = tabCompleter;
}
/**
* Command's every command executor
*
* @param sender Command's sender
* @param s command label
* @param args command arguments
* @throws CommandException if the specified command
* haves any mistakes
*/
@Override
public boolean execute(CommandSender sender, String s, String[] args){
if(getPermission() != null){
if(!sender.hasPermission(getPermission())){
if(getPermissionMessage() == null){
sender.sendMessage(ChatColor.RED + "I'm sorry but you do not have permission to perform this command. If you believe this is an error contact an administrator");
}else{
sender.sendMessage(getPermissionMessage());
}
return true;
}else{
try{
onCommand(sender, this, s, args);
}catch(Throwable exc){
sender.sendMessage(ChatColor.RED + "An error occurred while performing this command.");
throw new CommandException("Unhandled exception executing command '" + s + "' in plugin '" + getPlugin().getDescription().getFullName() + "'", exc);
}
}
}else{
try{
onCommand(sender, this, s, args);
}catch(Throwable exc){
sender.sendMessage(ChatColor.RED + "An error occurred while performing this command.");
throw new CommandException("Unhandled exception executing command '" + s + "' in plugin '" + getPlugin().getDescription().getFullName() + "'", exc);
}
}
return true;
}
/**
* Method for tab completion
*
* @param sender tab completer
* @param alias command alias
* @param args command arguments
* @throws CommandException if something occurs when
* tab completing
*/
@Override
public List tabComplete(CommandSender sender, String alias, String[] args){
Validate.notNull(sender, "Sender cannot be null");
Validate.notNull(args, "Arguments cannot be null");
Validate.notNull(alias, "Alias cannot be null");
List completions = null;
try {
if(this.completer != null){
completions = this.completer.onTabComplete(sender, this, alias, args);
}
if(completions == null && this instanceof TabCompleter) {
completions = ((TabCompleter)this).onTabComplete(sender, this, alias, args);
}
}catch(Throwable exc) {
StringBuilder message = new StringBuilder();
message.append("Unhandled exception during tab completion for command '/").append(alias).append(' ');
for(String arg : args) {
message.append(arg).append(' ');
}
message.deleteCharAt(message.length() - 1).append("' in plugin ").append(getPlugin().getDescription().getFullName());
throw new CommandException(message.toString(), exc);
}
return completions == null ? super.tabComplete(sender, alias, args) : completions;
}
}