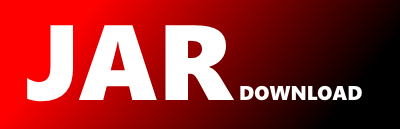
com.github.mrivanplays.acf.util.HookManager Maven / Gradle / Ivy
Show all versions of IvanACF Show documentation
/*
* *
* * Copyright 2018-2019 MrIvanPlays
* *
* * Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"),
* * to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense,
* * and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
* *
* * The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
* *
* * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
* * IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
* * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*
*/
package com.github.mrivanplays.acf.util;
import org.bukkit.plugin.Plugin;
import java.util.ArrayList;
import java.util.List;
/**
* Represents a hook manager
* which manages all hooks
*/
public class HookManager {
/**
* Hooked plugins are here
*/
private static List hooks = new ArrayList<>();
/**
* Checks if the specified {@link Plugin}
* is registered into the {@link List}
*
* @param plugin checked plugin
* @return true/false
*/
private static boolean isRegisteredHook(Plugin plugin){
return hooks.contains(plugin);
}
/**
* Registers a hook into the {@link List}
*
* @param plugin registered plugin
*/
public static void registerHook(Plugin plugin){
if(!isRegisteredHook(plugin)){
hooks.add(plugin);
}
}
/**
* Removes a hook from the {@link List}
*
* @param plugin removed plugin
*/
public static void removeHook(Plugin plugin){
if(isRegisteredHook(plugin)){
hooks.remove(plugin);
}
}
/**
* Gets the hooked plugins
*
* @return hooked plugins
*/
public static List getHookedPlugins(){
return hooks;
}
}