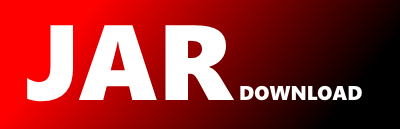
io.mstream.trader.commons.config.ConfigClient Maven / Gradle / Ivy
The newest version!
package io.mstream.trader.commons.config;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.mstream.trader.commons.config.schema.SchemaValidator;
import org.slf4j.Logger;
import javax.inject.Inject;
import java.util.Optional;
import static org.slf4j.LoggerFactory.getLogger;
public class ConfigClient {
private static final Logger LOGGER = getLogger(ConfigClient.class);
private final CuratorFrameworkSupplier curatorSupplier;
private final ObjectMapper mapper;
private final SchemaValidator schemaValidator;
@Inject
public ConfigClient(
CuratorFrameworkSupplier curatorSupplier,
ObjectMapper mapper,
SchemaValidator schemaValidator
) {
this.curatorSupplier = curatorSupplier;
this.mapper = mapper;
this.schemaValidator = schemaValidator;
}
public Optional read(Class configType) {
try {
byte[] configBytes = curatorSupplier
.get()
.getData()
.forPath("/");
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("fetched config: {}", new String(configBytes));
}
JsonNode configNode = mapper.readTree(configBytes);
boolean schemaCompliant = schemaValidator.test(configNode);
if (!schemaCompliant) {
throw new IllegalStateException(
"fetched config is not compliant with the config schema"
);
}
T config = mapper.treeToValue(configNode, configType);
return Optional.of(config);
} catch (Exception e) {
String configPath =
curatorSupplier
.get()
.getNamespace();
LOGGER.error(
"could not read the config from path: " + configPath,
e
);
return Optional.empty();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy