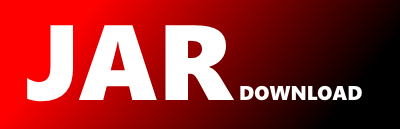
io.mstream.trader.commons.config.model.BaseUrl Maven / Gradle / Ivy
The newest version!
package io.mstream.trader.commons.config.model;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.mstream.trader.commons.utils.Preconditions;
import static java.lang.String.format;
public class BaseUrl {
private final String url;
private final boolean verifyCertificate;
@JsonCreator
public BaseUrl(
@JsonProperty(
value = "protocol",
required = true
)
String protocol,
@JsonProperty(
value = "host",
required = true
)
String host,
@JsonProperty(
value = "port"
)
Integer port,
@JsonProperty(
value = "basePath"
)
String basePath,
@JsonProperty(
value = "verifyCertificate"
)
Boolean verifyCertificate
) {
Preconditions.checkNotEmpty("protocol", protocol);
Preconditions.checkNotEmpty("host", host);
url = format(
"%s://%s:%d%s",
protocol,
host,
defaultPort(port, protocol),
defaultBasePath(basePath)
);
this.verifyCertificate =
defaultVerifyCertificate(
verifyCertificate,
protocol
);
}
private int defaultPort(Integer port, String protocol) {
if (port != null) {
return port;
}
switch (protocol) {
case "http":
return 80;
case "https":
return 443;
default:
throw new IllegalArgumentException(
format(
"can't guess the default port for %s protocol",
protocol
)
);
}
}
private String defaultBasePath(String basePath) {
return basePath == null ?
"" :
basePath;
}
private boolean defaultVerifyCertificate(
Boolean verifyCertificate,
String protocol
) {
if (verifyCertificate != null) {
return verifyCertificate;
}
switch (protocol) {
case "https":
return true;
default:
return false;
}
}
public String url() {
return url;
}
public boolean isVerifyCertificate() {
return verifyCertificate;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy