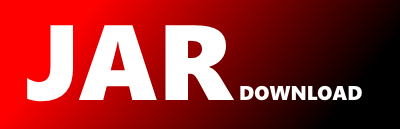
com.easydiameter.util.ProtocolDefinitions Maven / Gradle / Ivy
package com.easydiameter.util;
public interface ProtocolDefinitions {
/* Diameter Message Header Flags */
public static final byte HEADER_FLAG_NONE = (byte) 0x00;
public static final byte HEADER_FLAG_E = (byte) 0x20;
public static final byte HEADER_FLAG_P = (byte) 0x40;
public static final byte HEADER_FLAG_R = (byte) 0x80;
public static final byte HEADER_FLAG_P_E = (byte) 0x60;
public static final byte HEADER_FLAG_R_T = (byte) 0x90;
public static final byte HEADER_FLAG_R_P = (byte) 0xC0;
public static final byte HEADER_FLAG_R_P_T = (byte) 0xD0;
/* Diameter Message Header Flag Bits Masks */
public static final int HEADER_MASK_BIT_R = 0x80;
public static final int HEADER_MASK_BIT_P = 0x40;
public static final int HEADER_MASK_BIT_E = 0x20;
public static final int HEADER_MASK_BIT_T = 0x10;
public static final int HEADER_MASK_RESERVED = 0x0F;
/* AVP Flags */
public static final byte AVP_FLAG_V = (byte) 0x80;
public static final byte AVP_FLAG_M = (byte) 0x40;
public static final byte AVP_FLAG_V_M = (byte) 0xC0;
public static final byte AVP_FLAG_NONE = (byte) 0x00;
/* Diameter AVP Flag Bits Masks */
public static final int AVP_MASK_BIT_V = 0x80;
public static final int AVP_MASK_BIT_M = 0x40;
public static final int AVP_MASK_BIT_P = 0x20;
public static final int AVP_MASK_RESERVED = 0x1F;
/* Diameter Message Header Length Definitions */
public static final int DIAMETER_MSG_HDR_LEN = 20;
/* Diameter AVP Header Length Definitions */
public static final int AVP_HDR_LEN_WITH_VENDOR = 12;
public static final int AVP_HDR_LEN_WITHOUT_VENDOR = 8;
/* Available Diameter Versions */
public static final byte DIAMETER_VERSION = 1;
/* InetAddress Types */
public static final int ADDRESS_TYPE_IPv4 = 1;
public static final int ADDRESS_TYPE_IPv6 = 2;
/* AVP Data Types - DT stands for "Data Type" */
public static final int DT_UNKNOWN = -1;
public static final int DT_OCTET_STRING = 0;
public static final int DT_INTEGER_32 = 1;
public static final int DT_INTEGER_64 = 2;
public static final int DT_UNSIGNED_32 = 3;
public static final int DT_UNSIGNED_64 = 4;
public static final int DT_FLOAT_32 = 5;
public static final int DT_FLOAT_64 = 6;
public static final int DT_GROUPED = 7;
public static final int DT_ADDRESS = 8;
public static final int DT_TIME = 9;
public static final int DT_UTF8STRING = 10;
public static final int DT_DIAMETER_IDENTITY = 11;
public static final int DT_DIAMETER_URI = 12;
public static final int DT_ENUMERATED = 13;
public static final int DT_IP_FILTER_RULE = 14;
public static final int DT_QoS_FILTER_RULE = 15;
/* Command Codes */
public static final int COMMAND_CER_CEA = 257; /* [RFC6733] */
public static final int COMMAND_RAR_RAA = 258; /* [RFC6733] */
public static final int COMMAND_AMR_AMA = 260; /* [RFC4004] */
public static final int COMMAND_HAR_HAA = 262; /* [RFC4004] */
public static final int COMMAND_AAR_AAA = 265; /* [RFC7155] */
public static final int COMMAND_DER_DEA = 268; /* [RFC4072] */
public static final int COMMAND_ACR_ACA = 271; /* [RFC6733] */
public static final int COMMAND_CCR_CCA = 272; /* [RFC4006] */
public static final int COMMAND_ASR_ASA = 274; /* [RFC6733] */
public static final int COMMAND_STR_STA = 275; /* [RFC6733] */
public static final int COMMAND_DWR_DWA = 280; /* [RFC6733] */
public static final int COMMAND_DPR_DPA = 282; /* [RFC6733] */
public static final int COMMAND_UAR_UAA = 283; /* [RFC4740] */
public static final int COMMAND_SAR_SAA = 284; /* [RFC4740] */
public static final int COMMAND_LIR_LIA = 285; /* [RFC4740] */
public static final int COMMAND_MAR_MAA = 286; /* [RFC4740] */
public static final int COMMAND_RTR_RTA = 287; /* [RFC4740] */
public static final int COMMAND_PPR_PPA = 288; /* [RFC4740] */
public static final int COMMAND_PDR_PDA = 314; /* [RFC5224] */
/* AVP Codes - AC stands for "AVP Code" */
public static final int AC_MIP_FA_TO_HA_SPI = 318; /* [RFC4004] */
public static final int AC_MIP_FA_TO_MN_SPI = 319; /* [RFC4004] */
public static final int AC_MIP_REG_REQUEST = 320; /* [RFC4004] */
public static final int AC_MIP_REG_REPLY = 321; /* [RFC4004] */
public static final int AC_MIP_MN_AAA_AUTH = 322; /* [RFC4004] */
public static final int AC_MIP_HA_TO_FA_SPI = 323; /* [RFC4004] */
public static final int AC_MIP_MN_TO_FA_MSA = 325; /* [RFC4004] */
public static final int AC_MIP_FA_TO_MN_MSA = 326; /* [RFC4004] */
public static final int AC_MIP_FA_TO_HA_MSA = 328; /* [RFC4004] */
public static final int AC_MIP_HA_TO_FA_MSA = 329; /* [RFC4004] */
public static final int AC_MIP_MN_TO_HA_MSA = 331; /* [RFC4004] */
public static final int AC_MIP_HA_TO_MN_MSA = 332; /* [RFC4004] */
public static final int AC_MIP_MOBILE_NODE_ADRESS = 333; /* [RFC4004] */
public static final int AC_MIP_NONCE = 335; /* [RFC4004] */
public static final int AC_MIP_CANDIDATE_HOME_AGENT_HOST = 336; /* [RFC4004] */
public static final int AC_MIP_FEATURE_VECTOR = 337; /* [RFC4004] */
public static final int AC_MIP_AUTH_INPUT_DATA_LENGTH = 338; /* [RFC4004] */
public static final int AC_MIP_AUTHENTICATOR_LENGTH = 339; /* [RFC4004] */
public static final int AC_MIP_AUTHENTICATOR_OFFSET = 340; /* [RFC4004] */
public static final int AC_MIP_MN_AAA_SPI = 341; /* [RFC4004] */
public static final int AC_MIP_FILTER_RULE = 342; /* [RFC4004] */
public static final int AC_MIP_SESSION_KEY = 343; /* [RFC4004] */
public static final int AC_MIP_FA_CHALLENGE = 344; /* [RFC4004] */
public static final int AC_MIP_ALGORITHM_TYPE = 345; /* [RFC4004] */
public static final int AC_MIP_REPLAY_MODE = 346; /* [RFC4004] */
public static final int AC_MIP_ORIGINATING_FOREIGN_AAA = 347; /* [RFC4004] */
public static final int AC_MIP_MSA_LIFETIME = 367; /* [RFC4004] */
public static final int AC_SIP_ACCOUNTING_INFORMATION = 368; /* [RFC4740] */
public static final int AC_SIP_ACCOUNTING_SERVER_URI = 369; /* [RFC4740] */
public static final int AC_SIP_CREDIT_CONTROL_SERVER_URI = 370; /* [RFC4740] */
public static final int AC_SIP_SERVER_URI = 371; /* [RFC4740] */
public static final int AC_SIP_SERVER_CAPABILITIES = 372; /* [RFC4740] */
public static final int AC_SIP_MANDATORY_CAPABILITY = 373; /* [RFC4740] */
public static final int AC_SIP_OPTIONAL_CAPABILITY = 374; /* [RFC4740] */
public static final int AC_SIP_SERVER_ASSIGNMENT_TYPE = 375; /* [RFC4740] */
public static final int AC_SIP_AUTHENTICATION_INFO = 381; /* [RFC4740] */
public static final int AC_SIP_DEREGISTRATION_REASON = 383; /* [RFC4740] */
public static final int AC_SIP_REASON_CODE = 384; /* [RFC4740] */
public static final int AC_SIP_REASON_INFO = 385; /* [RFC4740] */
public static final int AC_SIP_VISITED_NETWORK_ID = 386; /* [RFC4740] */
public static final int AC_SIP_USER_AUTHORIZATION_TYPE = 387; /* [RFC4740] */
public static final int AC_SIP_SUPPORTED_USER_DATA_TYPE = 388; /* [RFC4740] */
public static final int AC_SIP_USER_DATA = 389; /* [RFC4740] */
public static final int AC_SIP_USER_DATA_TYPE = 390; /* [RFC4740] */
public static final int AC_SIP_USER_DATA_CONTENTS = 391; /* [RFC4740] */
public static final int AC_SIP_USER_DATA_ALREADY_AVAILABLE = 392; /* [RFC4740] */
public static final int AC_CHAP_ALGORTIHM = 403; /* [RFC7155] */
public static final int AC_QoS_FILTER_RULE = 407; /* [RFC7155] */
public static final int AC_REQUEST_ACTION = 436; /* [RFC4006] */
public static final int AC_REQUEST_SERVICE_UNIT = 437; /* [RFC4006] */
public static final int AC_MIP_AUTHENTICATOR = 488; /* [RFC5778] */
public static final int AC_MIP_MAC_MOBILITY_DATA = 489; /* [RFC5778] */
public static final int AC_MIP_TIMESTAMP = 490; /* [RFC5778] */
public static final int AC_MIP_MN_HA_SPI = 491; /* [RFC5778] */
public static final int AC_MIP_MN_HA_MSA = 492; /* [RFC5778] */
public static final int AC_MIP6_AUTH_MODE = 494; /* [RFC5778] */
public static final int AC_TMOD_1 = 495; /* [RFC5624] */
public static final int AC_TOKEN_RATE = 496; /* [RFC5624] */
public static final int AC_BUCKET_DEPTH = 497; /* [RFC5624] */
public static final int AC_PEAK_TRAFFIC_RATE = 498; /* [RFC5624] */
public static final int AC_MINIMUM_POLICED_UNIT = 499; /* [RFC5624] */
public static final int AC_MAXIMUM_PACKET_SIZE = 500; /* [RFC5624] */
public static final int AC_TMOD_2 = 501; /* [RFC5624] */
public static final int AC_BANDWITH = 502; /* [RFC5624] */
public static final int AC_PHB_CLASS = 503; /* [RFC5624] */
public static final int AC_PMIP6_DHCP_SERVER_ADDRESS = 504; /* [RFC5779] */
public static final int AC_PMIP6_IPV4_HOME_ADDRESS = 505; /* [RFC5779] */
public static final int AC_SERVICE_CONFIGURATION = 507; /* [RFC5779] */
public static final int AC_QoS_RESOURCES = 508; /* [RFC5777] */
public static final int AC_IP_MASK_BIT_MASK_WIDTH = 523; /* [RFC5777] */
public static final int AC_QoS_PROFILE_ID = 573; /* [RFC5777] */
public static final int AC_QoS_PROFILE_TEMPLATE = 574; /* [RFC5777] */
public static final int AC_QoS_SEMANTICS = 575; /* [RFC5777] */
public static final int AC_QoS_PARAMETERS = 576; /* [RFC5777] */
public static final int AC_QoS_CAPABILITY = 578; /* [RFC5777] */
public static final int AC_QoS_AUTHORIZATION_DATA = 579; /* [RFC5866] */
public static final int AC_BOUND_AUTH_SESSION_ID = 580; /* [RFC5866] */
public static final int AC_IKEv2_NONCES = 587; /* [RFC6738] */
public static final int AC_NI = 588; /* [RFC6738] */
public static final int AC_NR = 589; /* [RFC6738] */
public static final int AC_IKEv2_IDENTITY = 590; /* [RFC6738] */
public static final int AC_INITIATOR_IDENTITY = 591; /* [RFC6738] */
public static final int AC_ID_TYPE = 592; /* [RFC6738] */
public static final int AC_IDENTIFICATION_DATA = 593; /* [RFC6738] */
public static final int AC_RESPONDER_IDENTITY = 594; /* [RFC6738] */
public static final int AC_NC_REQUEST_TYPE = 595; /* [RFC6736] */
public static final int AC_NAT_CONTROL_INSTALL = 596; /* [RFC6736] */
public static final int AC_NAT_CONTROL_REMOVE = 597; /* [RFC6736] */
public static final int AC_NAT_CONTROL_DEFINITION = 598; /* [RFC6736] */
public static final int AC_NAT_INTERNAL_ADDRESS = 599; /* [RFC6736] */
public static final int AC_NAT_EXTERNAL_ADDRESS = 600; /* [RFC6736] */
public static final int AC_MAX_NAT_BINDINGS = 601; /* [RFC6736] */
public static final int AC_NAT_CONTROL_BINDING_TEMPLATE = 602; /* [RFC6736] */
public static final int AC_DUPLICATE_SESSION_ID = 603; /* [RFC6736] */
public static final int AC_NAT_EXTERNAL_PORT_STYLE = 604; /* [RFC6736] */
public static final int AC_NAT_CONTROL_RECORD = 605; /* [RFC6736] */
public static final int AC_NAT_CONTROL_BINDING_STATUS = 606; /* [RFC6736] */
public static final int AC_CURRENT_NAT_BINDINGS = 607; /* [RFC6736] */
public static final int AC_DUAL_PRIORITY = 608; /* [RFC6735] */
public static final int AC_PREEMPTION_PRIORITY = 609; /* [RFC6735] */
public static final int AC_DEFENDING_PRIORITY = 610; /* [RFC6735] */
public static final int AC_ADMISSION_PRIORITY = 611; /* [RFC6735] */
public static final int AC_SIP_RESOURCE_PRIORITY = 612; /* [RFC6735] */
public static final int AC_SIP_RESOURCE_PRIORITY_NAMESPACE = 613; /* [RFC6735] */
public static final int AC_SIP_RESOURCE_PRIORITY_VALUE = 614; /* [RFC6735] */
public static final int AC_APPLICATION_LEVEL_RESOURCE_PRIORITY = 615; /* [RFC6735] */
public static final int AC_ALRP_NAMESPACE = 616; /* [RFC6735] */
public static final int AC_ALRP_VALUE = 617; /* [RFC6735] */
public static final int AC_REDIRECT_REALM = 620; /* [RFC7075] */
public static final int AC_ECN_IP_CODEPOINT = 628; /* [RFC7660] */
public static final int AC_CONGESTION_TREATMENT = 629; /* [RFC7660] */
public static final int AC_FLOW_COUNT = 630; /* [RFC7660] */
public static final int AC_PACKET_COUNT = 631; /* [RFC7660] */
public static final int AC_IP_PREFIX_LENGTH = 632; /* [RFC7678] */
public static final int AC_BORDER_ROUTER_NAME = 633; /* [RFC7678] */
public static final int AC_64_MULTICAST_ATTRIBUTES = 634; /* [RFC7678] */
public static final int AC_ASM_MPREFIX64 = 635; /* [RFC7678] */
public static final int AC_SSM_MPREFIX64 = 636; /* [RFC7678] */
public static final int AC_TUNNEL_SOURCE_PREF_OR_ADDR = 637; /* [RFC7678] */
public static final int AC_TUNNEL_SOURCE_IPv6_ADDRESS = 638; /* [RFC7678] */
public static final int AC_PORT_SET_IDENTIFIER = 639; /* [RFC7678] */
public static final int AC_LW4O6_BINDING = 640; /* [RFC7678] */
public static final int AC_LW4O6_EXTERNAL_IPv4_ADDR = 641; /* [RFC7678] */
public static final int AC_MAP_E_ATTRIBUTES = 642; /* [RFC7678] */
public static final int AC_MAP_MESH_MODE = 643; /* [RFC7678] */
public static final int AC_MAP_MAPPING_RULE = 644; /* [RFC7678] */
public static final int AC_RULE_IPv4_ADDR_OR_PREFIX = 645; /* [RFC7678] */
public static final int AC_RULE_IPv6_PREFIX = 646; /* [RFC7678] */
public static final int AC_EA_FIELD_LENGTH = 647; /* [RFC7678] */
public static final int AC_OC_PEER_ALGO = 648; /* [DimeAgentOverload11] */
public static final int AC_SOURCEID = 649; /* [DimeAgentOverload11] */
public static final int AC_LOAD = 650; /* [RFCIetfDimeLoad09] */
public static final int AC_LOAD_TYPE = 651; /* [RFCIetfDimeLoad09] */
public static final int AC_LOAD_VALUE = 652; /* [RFCIetfDimeLoad09] */
public static final int AC_1XRTT_RCID = 2554; /* [29.172-d10] */
public static final int AC_3GPP_AAA_SERVER_NAME = 318; /* [29.234-b20] */
public static final int AC_3GPP_ALLOCATE_IP_TYPE = 27; /* [29.061-f10] */
public static final int AC_3GPP_CAMEL_CHARGING_INFO = 24; /* [29.061-f10] */
public static final int AC_3GPP_CHARGING_CHARACTERISTICS = 13; /* [29.061-f10] */
public static final int AC_3GPP_CHARGING_DNS_SERVERS = 17; /* [29.061-f10] */
public static final int AC_3GPP_CHARGING_GATEWAY_ADDRESS = 4; /* [29.061-f10] */
public static final int AC_3GPP_CHARGING_GATEWAY_IPV6_ADDRESS = 14; /* [29.061-f10] */
public static final int AC_3GPP_CHARGING_ID = 2; /* [29.061-f10] */
public static final int AC_3GPP_GGSN_ADDRESS = 7; /* [29.061-f10] */
public static final int AC_3GPP_GGSN_IPV6_ADDRESS = 16; /* [29.061-f10] */
public static final int AC_3GPP_GGSN_MCC_MNC = 9; /* [29.061-f10] */
public static final int AC_3GPP_GPRS_NEGOTIATED_QOS_PROFILE = 5; /* [29.061-f10] */
public static final int AC_3GPP_IMEISV = 20; /* [29.061-f10] */
public static final int AC_3GPP_IMSI = 1; /* [29.061-f10] */
public static final int AC_3GPP_IMSI_MCC_MNC = 8; /* [29.061-f10] */
public static final int AC_3GPP_MS_TIMEZONE = 23; /* [29.061-f10] */
public static final int AC_3GPP_NEGOTIATED_DSCP = 26; /* [29.061-f10] */
public static final int AC_3GPP_NSAPI = 10; /* [29.061-f10] */
public static final int AC_3GPP_PACKET_FILTER = 25; /* [29.061-f10] */
public static final int AC_3GPP_PDP_TYPE = 3; /* [29.061-f10] */
public static final int AC_3GPP_PS_DATA_OFF_STATUS = 2847; /* [29.212-f10] */
public static final int AC_3GPP_RAT_TYPE = 21; /* [29.061-f10] */
public static final int AC_3GPP_SELECTION_MODE = 12; /* [29.061-f10] */
public static final int AC_3GPP_SESSION_STOP_INDICATOR = 11; /* [29.061-f10] */
public static final int AC_3GPP_SGSN_ADDRESS = 6; /* [29.061-f10] */
public static final int AC_3GPP_SGSN_IPV6_ADDRESS = 15; /* [29.061-f10] */
public static final int AC_3GPP_SGSN_MCC_MNC = 18; /* [29.061-f10] */
public static final int AC_3GPP_SGSN_MCC_MNC_GX = 18; /* [29.061] */
public static final int AC_3GPP_TEARDOWN_INDICATOR = 19; /* [29.061-f10] */
public static final int AC_3GPP_USER_LOCATION_INFO = 22; /* [29.061-f10] */
public static final int AC_3GPP_USER_LOCATION_INFO_TIME = 30; /* [29.061-f10] */
public static final int AC_3GPP_WLAN_APN_ID = 100; /* [29.003] */
public static final int AC_3GPP2_BSID = 9010; /* [3GPP2 X.S0057-0 v3.0] */
public static final int AC_3GPP2_MEID = 1471; /* [29.272-f10] */
public static final int AC_A_MSISDN = 1643; /* [29.272-f10] */
public static final int AC_AAA_FAILURE_INDICATION = 1518; /* [29.273-f10] */
public static final int AC_AAR_FLAGS = 1539; /* [29.273-f10] */
public static final int AC_ABORT_CAUSE = 500; /* [29.214-f10] */
public static final int AC_ABSENT_SUBSCRIBER_DIAGNOSTIC_T4 = 3201; /* [29.337-e10] */
public static final int AC_ABSENT_USER_DIAGNOSTIC_SM = 3322; /* [29.338-f00] */
public static final int AC_ABSOLUTE_END_FRACTIONAL_SECONDS = 569; /* [RFC 5777] */
public static final int AC_ABSOLUTE_END_TIME = 568; /* [RFC 5777] */
public static final int AC_ABSOLUTE_START_FRACTIONAL_SECONDS = 567; /* [RFC 5777] */
public static final int AC_ABSOLUTE_START_TIME = 566; /* [RFC 5777] */
public static final int AC_ACCEPTABLE_SERVICE_INFO = 526; /* [29.214-f10] */
public static final int AC_ACCESS_AUTHORIZATION_FLAGS = 1511; /* [29.273-f10] */
public static final int AC_ACCESS_AVAILABILITY_CHANGE_REASON = 2833; /* [29.212-f10] */
public static final int AC_ACCESS_NETWORK_CHARGING_ADDRESS = 501; /* [29.214-f10] */
public static final int AC_ACCESS_NETWORK_CHARGING_IDENTIFIER = 502; /* [29.214-f10] */
public static final int AC_ACCESS_NETWORK_CHARGING_IDENTIFIER_GX = 1022; /* [29.212-f10] */
public static final int AC_ACCESS_NETWORK_CHARGING_IDENTIFIER_VALUE = 503; /* [29.214-f10] */
public static final int AC_ACCESS_NETWORK_INFO = 1526; /* [29.273-f10] */
public static final int AC_ACCESS_NETWORK_INFORMATION = 1263; /* [32-299-f10] */
public static final int AC_ACCESS_RESTRICTION_DATA = 1426; /* [29.272-f10] */
public static final int AC_ACCOUNT_EXPIRATION = 2309; /* [32-299-f10] */
public static final int AC_ACCOUNTING_AUTH_METHOD = 406; /* [RFC 7155] */
public static final int AC_ACCOUNTING_EAP_AUTH_METHOD = 465; /* [no_reference] */
public static final int AC_ACCOUNTING_INPUT_OCTETS = 363; /* [RFC 7155] */
public static final int AC_ACCOUNTING_INPUT_PACKETS = 365; /* [RFC 7155] */
public static final int AC_ACCOUNTING_OUTPUT_OCTETS = 364; /* [RFC 7155] */
public static final int AC_ACCOUNTING_OUTPUT_PACKETS = 366; /* [RFC 7155] */
public static final int AC_ACCOUNTING_REALTIME_REQUIRED = 483; /* [RFC 6733] */
public static final int AC_ACCOUNTING_RECORD_NUMBER = 485; /* [RFC 6733] */
public static final int AC_ACCOUNTING_RECORD_TYPE = 480; /* [RFC 6733] */
public static final int AC_ACCOUNTING_SUB_SESSION_ID = 287; /* [RFC 6733] */
public static final int AC_ACCT_APPLICATION_ID = 259; /* [RFC 6733] */
public static final int AC_ACCT_AUTHENTIC = 45; /* [RFC 7155] */
public static final int AC_ACCT_DELAY_TIME = 41; /* [RFC 7155] */
public static final int AC_ACCT_INTERIM_INTERVAL = 85; /* [RFC 6733] */
public static final int AC_ACCT_LINK_COUNT = 51; /* [RFC 7155] */
public static final int AC_ACCT_MULTI_SESSION_ID = 50; /* [RFC 6733] */
public static final int AC_ACCT_SESSION_ID = 44; /* [RFC 6733] */
public static final int AC_ACCT_SESSION_TIME = 46; /* [RFC 7155] */
public static final int AC_ACCT_TUNNEL_CONNECTION = 68; /* [RFC 7155] */
public static final int AC_ACCT_TUNNEL_PACKETS_LOST = 86; /* [RFC 7155] */
public static final int AC_ACCUMULATED_COST = 2052; /* [32-299-f10] */
public static final int AC_ACCURACY = 3137; /* [29.336-f10] */
public static final int AC_ACCURACY_FULFILMENT_INDICATOR = 2513; /* [29.172-d10] */
public static final int AC_ACTION_TYPE = 3005; /* [29.368-e20] */
public static final int AC_ACTIVE_APN = 1612; /* [29.272-f10] */
public static final int AC_ACTIVE_TIME = 4324; /* [29.128-f00] */
public static final int AC_ADAPTATIONS = 1217; /* [32-299-f10] */
public static final int AC_ADC_REVALIDATION_TIME = 2801; /* [29.212-f10] */
public static final int AC_ADC_RULE_BASE_NAME = 1095; /* [29.212-f10] */
public static final int AC_ADC_RULE_DEFINITION = 1094; /* [29.212-f10] */
public static final int AC_ADC_RULE_INSTALL = 1092; /* [29.212-f10] */
public static final int AC_ADC_RULE_NAME = 1096; /* [29.212-f10] */
public static final int AC_ADC_RULE_REMOVE = 1093; /* [29.212-f10] */
public static final int AC_ADC_RULE_REPORT = 1097; /* [29.212-f10] */
public static final int AC_ADDITIONAL_CONTENT_INFORMATION = 1207; /* [32-299-f10] */
public static final int AC_ADDITIONAL_CONTEXT_IDENTIFIER = 1683; /* [29.272-f10] */
public static final int AC_ADDITIONAL_EXCEPTION_REPORTS = 3936; /* [32-299-f10] */
public static final int AC_ADDITIONAL_SERVING_NODE = 2406; /* [29.173-e00] */
public static final int AC_ADDITIONAL_SERVING_NODE_T4 = 2406; /* [29.336-f10] */
public static final int AC_ADDITIONAL_TYPE_INFORMATION = 1205; /* [32-299-f10] */
public static final int AC_ADDRESS_DATA = 897; /* [32-299-f10] */
public static final int AC_ADDRESS_DOMAIN = 898; /* [32-299-f10] */
public static final int AC_ADDRESS_TYPE = 899; /* [32-299-f10] */
public static final int AC_ADDRESSEE_TYPE = 1208; /* [32-299-f10] */
public static final int AC_ADJACENT_ACCESS_RESTRICTION_DATA = 1673; /* [29.272-f10] */
public static final int AC_ADJACENT_PLMNS = 1672; /* [29.272-f10] */
public static final int AC_AESE_COMMUNICATION_PATTERN = 3113; /* [29.336-f10] */
public static final int AC_AESE_COMMUNICATION_PATTERN_CONFIG_STATUS = 3120; /* [29.336-f10] */
public static final int AC_AESE_COMMUNICATION_PATTERN_S6 = 3113; /* [29.272-f10] */
public static final int AC_AESE_ERROR_REPORT = 3121; /* [29.336-f10] */
public static final int AC_AF_APPLICATION_IDENTIFIER = 504; /* [29.214-f10] */
public static final int AC_AF_CHARGING_IDENTIFIER = 505; /* [29.214-f10] */
public static final int AC_AF_CORRELATION_INFORMATION = 1276; /* [32-299-f10] */
public static final int AC_AF_REQUESTED_DATA = 551; /* [29.214-f10] */
public static final int AC_AF_SIGNALLING_PROTOCOL = 529; /* [29.214-f10] */
public static final int AC_AGE_OF_LOCATION_ESTIMATE = 2514; /* [29.172-d10] */
public static final int AC_AGE_OF_LOCATION_INFORMATION = 1611; /* [29.272-f10] */
public static final int AC_AGGREGATED_CONGESTION_INFO = 4000; /* [29.217-e20] */
public static final int AC_AGGREGATED_RUCI_REPORT = 4001; /* [29.217-e20] */
public static final int AC_AIR_FLAGS = 1679; /* [29.272-f10] */
public static final int AC_ALERT_REASON = 1434; /* [29.272-f10] */
public static final int AC_ALL_APN_CONFIGURATIONS_INCLUDED_INDICATOR = 1428; /* [29.272-f10] */
public static final int AC_ALLOCATION_RETENTION_PRIORITY = 1034; /* [29.212-f10] */
public static final int AC_ALLOWED_PLMN_LIST = 3158; /* [29.336-f10] */
public static final int AC_ALLOWED_WAF_WWSF_IDENTITIES = 656; /* [29.229-e20] */
public static final int AC_ALTERNATE_CHARGED_PARTY_ADDRESS = 1280; /* [32-299-f10] */
public static final int AC_AMBR = 1435; /* [29.272-f10] */
public static final int AC_AN_GW_ADDRESS = 1050; /* [29.212-f10] */
public static final int AC_AN_GW_STATUS = 2811; /* [29.212-f10] */
public static final int AC_AN_TRUSTED = 1503; /* [29.273-f10] */
public static final int AC_ANID = 1504; /* [29.273-f10] */
public static final int AC_AOC_COST_INFORMATION = 2053; /* [32-299-f10] */
public static final int AC_AOC_FORMAT = 2310; /* [32-299-f10] */
public static final int AC_AOC_INFORMATION = 2054; /* [32-299-f10] */
public static final int AC_AOC_REQUEST_TYPE = 2055; /* [32-299-f10] */
public static final int AC_AOC_SERVICE = 2311; /* [32-299-f10] */
public static final int AC_AOC_SERVICE_OBLIGATORY_TYPE = 2312; /* [32-299-f10] */
public static final int AC_AOC_SERVICE_TYPE = 2313; /* [32-299-f10] */
public static final int AC_AOC_SUBSCRIPTION_INFORMATION = 2314; /* [32-299-f10] */
public static final int AC_APN_AGGREGATE_MAX_BITRATE_DL = 1040; /* [29.212-f10] */
public static final int AC_APN_AGGREGATE_MAX_BITRATE_UL = 1041; /* [29.212-f10] */
public static final int AC_APN_AUTHORIZED = 307; /* [29.234-b20] */
public static final int AC_APN_BARRING_TYPE = 309; /* [29.234-b20] */
public static final int AC_APN_CONFIGURATION = 1430; /* [29.272-f10] */
public static final int AC_APN_CONFIGURATION_PROFILE = 1429; /* [29.272-f10] */
public static final int AC_APN_CONFIGURATION_S6 = 1430; /* [29.272-f10] */
public static final int AC_APN_OI_REPLACEMENT = 1427; /* [29.272-f10] */
public static final int AC_APN_RATE_CONTROL = 3933; /* [32-299-f10] */
public static final int AC_APN_RATE_CONTROL_DOWNLINK = 3934; /* [32-299-f10] */
public static final int AC_APN_RATE_CONTROL_UPLINK = 3935; /* [32-299-f10] */
public static final int AC_APN_VALIDITY_TIME = 3169; /* [29.336-f10] */
public static final int AC_APPLIC_ID = 1218; /* [32-299-f10] */
public static final int AC_APPLICATION_DETECTION_INFORMATION = 1098; /* [29.212-f10] */
public static final int AC_APPLICATION_PORT_IDENTIFIER = 3010; /* [29.368-e20] */
public static final int AC_APPLICATION_PROVIDED_CALLED_PARTY_ADDRESS = 837; /* [32-299-f10] */
public static final int AC_APPLICATION_SERVER = 836; /* [32-299-f10] */
public static final int AC_APPLICATION_SERVER_ID = 2101; /* [OMADDSChargingData] */
public static final int AC_APPLICATION_SERVER_INFORMATION = 850; /* [32-299-f10] */
public static final int AC_APPLICATION_SERVICE_PROVIDER_IDENTITY = 532; /* [29.214-f10] */
public static final int AC_APPLICATION_SERVICE_TYPE = 2102; /* [OMADDSChargingData] */
public static final int AC_APPLICATION_SESSION_ID = 2103; /* [OMADDSChargingData] */
public static final int AC_ARAP_CHALLENGE_RESPONSE = 84; /* [RFC 7155] */
public static final int AC_ARAP_FEATURES = 71; /* [RFC 7155] */
public static final int AC_ARAP_PASSWORD = 70; /* [RFC 7155] */
public static final int AC_ARAP_SECURITY = 73; /* [RFC 7155] */
public static final int AC_ARAP_SECURITY_DATA = 74; /* [RFC 7155] */
public static final int AC_ARAP_ZONE_ACCESS = 72; /* [RFC 7155] */
public static final int AC_AREA = 2535; /* [29.172-d10] */
public static final int AC_AREA_DEFINITION = 2534; /* [29.172-d10] */
public static final int AC_AREA_EVENT_INFO = 2533; /* [29.172-d10] */
public static final int AC_AREA_IDENTIFICATION = 2537; /* [29.172-d10] */
public static final int AC_AREA_SCOPE = 1624; /* [29.272-f10] */
public static final int AC_AREA_TYPE = 2536; /* [29.172-d10] */
public static final int AC_AS_NUMBER = 722; /* [29.329-f00] */
public static final int AC_ASSOCIATED_IDENTITIES = 632; /* [29.229-e20] */
public static final int AC_ASSOCIATED_PARTY_ADDRESS = 2035; /* [32-299-f10] */
public static final int AC_ASSOCIATED_REGISTERED_IDENTITIES = 647; /* [29.229-e20] */
public static final int AC_ASSOCIATED_URI = 856; /* [32-299-f10] */
public static final int AC_ASSOCIATION_TYPE = 3138; /* [29.336-f10] */
public static final int AC_AUTH_APPLICATION_ID = 258; /* [RFC 6733] */
public static final int AC_AUTH_GRACE_PERIOD = 276; /* [RFC 6733] */
public static final int AC_AUTH_REQUEST_TYPE = 274; /* [RFC 6733] */
public static final int AC_AUTH_SESSION_STATE = 277; /* [RFC 6733] */
public static final int AC_AUTHENTICATION_INFO = 1413; /* [29.272-f10] */
public static final int AC_AUTHENTICATION_INFORMATION_SIM = 301; /* [29.234-b20] */
public static final int AC_AUTHENTICATION_METHOD = 300; /* [29.234-b20] */
public static final int AC_AUTHORIZATION_INFORMATION_SIM = 302; /* [29.234-b20] */
public static final int AC_AUTHORIZATION_LIFETIME = 291; /* [RFC 6733] */
public static final int AC_AUTN = 1449; /* [29.272-f10] */
public static final int AC_AUX_APPLIC_INFO = 1219; /* [32-299-f10] */
public static final int AC_BAROMETRIC_PRESSURE = 2557; /* [29.172-d10] */
public static final int AC_BASE_TIME_INTERVAL = 1265; /* [32-299-f10] */
public static final int AC_BEARER_CONTROL_MODE = 1023; /* [29.212-f10] */
public static final int AC_BEARER_IDENTIFIER = 1020; /* [29.212-f10] */
public static final int AC_BEARER_OPERATION = 1021; /* [29.212-f10] */
public static final int AC_BEARER_SERVICE = 854; /* [32-299-f10] */
public static final int AC_BEARER_USAGE = 1000; /* [29.212-f10] */
public static final int AC_BSSGP_CAUSE = 64309; /* [29.128-f10] */
public static final int AC_BSSID = 2716; /* [32-299-f10] */
public static final int AC_C_VID_END = 556; /* [RFC 5777] */
public static final int AC_C_VID_START = 555; /* [RFC 5777] */
public static final int AC_CALL_BARRING_INFO = 1488; /* [29.272-f10] */
public static final int AC_CALL_ID_SIP_HEADER = 643; /* [29.229-e20] */
public static final int AC_CALL_REFERENCE_INFO = 720; /* [29.329-f00] */
public static final int AC_CALL_REFERENCE_NUMBER = 721; /* [29.329-f00] */
public static final int AC_CALLBACK_ID = 20; /* [RFC 7155] */
public static final int AC_CALLBACK_NUMBER = 19; /* [RFC 7155] */
public static final int AC_CALLED_ASSERTED_IDENTITY = 1250; /* [32-299-f10] */
public static final int AC_CALLED_IDENTITY = 3916; /* [32-299-f10] */
public static final int AC_CALLED_IDENTITY_CHANGE = 3917; /* [32-299-f10] */
public static final int AC_CALLED_PARTY_ADDRESS = 832; /* [32-299-f10] */
public static final int AC_CALLED_STATION_ID = 30; /* [RFC 7155] */
public static final int AC_CALLING_PARTY_ADDRESS = 831; /* [32-299-f10] */
public static final int AC_CALLING_STATION_ID = 31; /* [RFC 7155] */
public static final int AC_CANCELLATION_TYPE = 1420; /* [29.272-f10] */
public static final int AC_CARRIER_FREQUENCY = 1696; /* [29.272-f10] */
public static final int AC_CARRIER_SELECT_ROUTING_INFORMATION = 2023; /* [32-299-f10] */
public static final int AC_CAUSE_CODE = 861; /* [32-299-f10] */
public static final int AC_CAUSE_TYPE = 4301; /* [29.128-f10] */
public static final int AC_CC_CORRELATION_ID = 411; /* [RFC 4006] */
public static final int AC_CC_INPUT_OCTETS = 412; /* [RFC 4006] */
public static final int AC_CC_MONEY = 413; /* [RFC 4006] */
public static final int AC_CC_OUTPUT_OCTETS = 414; /* [RFC 4006] */
public static final int AC_CC_REQUEST_NUMBER = 415; /* [RFC 4006] */
public static final int AC_CC_REQUEST_TYPE = 416; /* [RFC 4006] */
public static final int AC_CC_SERVICE_SPECIFIC_UNITS = 417; /* [RFC 4006] */
public static final int AC_CC_SESSION_FAILOVER = 418; /* [RFC 4006] */
public static final int AC_CC_SUB_SESSION_ID = 419; /* [RFC 4006] */
public static final int AC_CC_TIME = 420; /* [RFC 4006] */
public static final int AC_CC_TOTAL_OCTETS = 421; /* [RFC 4006] */
public static final int AC_CC_UNIT_TYPE = 454; /* [RFC 4006] */
public static final int AC_CELL_GLOBAL_IDENTITY = 1604; /* [29.272-f10] */
public static final int AC_CELL_PORTION_ID = 2553; /* [29.172-d10] */
public static final int AC_CG_ADDRESS = 846; /* [32-299-f10] */
public static final int AC_CHANGE_CONDITION = 2037; /* [32-299-f10] */
public static final int AC_CHANGE_TIME = 2038; /* [32-299-f10] */
public static final int AC_CHAP_ALGORITHM = 403; /* [RFC 7155] */
public static final int AC_CHAP_AUTH = 402; /* [RFC 7155] */
public static final int AC_CHAP_CHALLENGE = 60; /* [RFC 7155] */
public static final int AC_CHAP_IDENT = 404; /* [RFC 7155] */
public static final int AC_CHAP_RESPONSE = 405; /* [RFC 7155] */
public static final int AC_CHARGE_REASON_CODE = 2118; /* [32-299-f10] */
public static final int AC_CHARGED_PARTY = 857; /* [32-299-f10] */
public static final int AC_CHARGED_PARTY_S6 = 857; /* [29.272-f10] */
public static final int AC_CHARGING_CHARACTERISTICS = 314; /* [29.234-b20] */
public static final int AC_CHARGING_CHARACTERISTICS_SELECTION_MODE = 2066; /* [32-299-f10] */
public static final int AC_CHARGING_CORRELATION_INDICATOR = 1073; /* [29.212-f10] */
public static final int AC_CHARGING_DATA = 304; /* [29.234-b20] */
public static final int AC_CHARGING_INFORMATION = 618; /* [29.229-e20] */
public static final int AC_CHARGING_NODES = 315; /* [29.234-b20] */
public static final int AC_CHARGING_PER_IP_CAN_SESSION_INDICATOR = 4400; /* [32-299-f10] */
public static final int AC_CHARGING_RULE_BASE_NAME = 1004; /* [29.212-f10] */
public static final int AC_CHARGING_RULE_DEFINITION = 1003; /* [29.212-f10] */
public static final int AC_CHARGING_RULE_INSTALL = 1001; /* [29.212-f10] */
public static final int AC_CHARGING_RULE_NAME = 1005; /* [29.212-f10] */
public static final int AC_CHARGING_RULE_REMOVE = 1002; /* [29.212-f10] */
public static final int AC_CHARGING_RULE_REPORT = 1018; /* [29.212-f10] */
public static final int AC_CHECK_BALANCE_RESULT = 422; /* [RFC 4006] */
public static final int AC_CIA_FLAGS = 3164; /* [29.336-f10] */
public static final int AC_CIR_FLAGS = 3145; /* [29.336-f10] */
public static final int AC_CIVIC_ADDRESS = 2556; /* [29.172-d10] */
public static final int AC_CLASS = 25; /* [RFC 6733] */
public static final int AC_CLASS_IDENTIFIER = 1214; /* [32-299-f10] */
public static final int AC_CLASSIFIER = 511; /* [RFC 5777] */
public static final int AC_CLASSIFIER_ID = 512; /* [RFC 5777] */
public static final int AC_CLIENT_ADDRESS = 2018; /* [32-299-f10] */
public static final int AC_CLIENT_IDENTITY = 1480; /* [29.272-f10] */
public static final int AC_CLR_FLAGS = 1638; /* [29.272-f10] */
public static final int AC_CMR_FLAGS = 4317; /* [29.128-f10] */
public static final int AC_CN_IP_MULTICAST_DISTRIBUTION = 921; /* [29.061-f10] */
public static final int AC_CN_OPERATOR_SELECTION_ENTITY = 3421; /* [32-299-f10] */
public static final int AC_COA_INFORMATION = 1039; /* [29.212-f10] */
public static final int AC_COA_IP_ADDRESS = 1035; /* [29.212-f10] */
public static final int AC_CODEC_DATA = 524; /* [29.214-f10] */
public static final int AC_COLLECTION_PERIOD_RRM_LTE = 1657; /* [29.272-f10] */
public static final int AC_COLLECTION_PERIOD_RRM_UMTS = 1658; /* [29.272-f10] */
public static final int AC_COMMUNICATION_DURATION_TIME = 3116; /* [29.336-f10] */
public static final int AC_COMMUNICATION_FAILURE_INFORMATION = 4300; /* [29.128-f10] */
public static final int AC_COMMUNICATION_PATTERN_SET = 3114; /* [29.336-f10] */
public static final int AC_COMMUNICATION_PATTERN_SET_S6 = 3114; /* [29.272-f10] */
public static final int AC_COMPLETE_DATA_LIST_INCLUDED_INDICATOR = 1468; /* [29.272-f10] */
public static final int AC_CONDITIONAL_APN_AGGREGATE_MAX_BITRATE = 2818; /* [29.212-f10] */
public static final int AC_CONDITIONAL_POLICY_INFORMATION = 2840; /* [29.212-f10] */
public static final int AC_CONDITIONAL_RESTRICTION = 4007; /* [29.217-e20] */
public static final int AC_CONFIDENTIALITY_KEY = 625; /* [29.229-e20] */
public static final int AC_CONFIGURATION_TOKEN = 78; /* [RFC 7155] */
public static final int AC_CONGESTION_LEVEL_DEFINITION = 4002; /* [29.217-e20] */
public static final int AC_CONGESTION_LEVEL_RANGE = 4003; /* [29.217-e20] */
public static final int AC_CONGESTION_LEVEL_SET_ID = 4004; /* [29.217-e20] */
public static final int AC_CONGESTION_LEVEL_VALUE = 4005; /* [29.217-e20] */
public static final int AC_CONGESTION_LOCATION_ID = 4006; /* [29.217-e20] */
public static final int AC_CONNECT_INFO = 77; /* [RFC 7155] */
public static final int AC_CONNECTION_ACTION = 4314; /* [29.128-f10] */
public static final int AC_CONNECTIVITY_FLAGS = 1529; /* [29.273-f10] */
public static final int AC_CONTACT = 641; /* [29.229-e20] */
public static final int AC_CONTENT_CLASS = 1220; /* [32-299-f10] */
public static final int AC_CONTENT_DISPOSITION = 828; /* [32-299-f10] */
public static final int AC_CONTENT_ID = 2116; /* [OMADDSChargingData] */
public static final int AC_CONTENT_LENGTH = 827; /* [32-299-f10] */
public static final int AC_CONTENT_PROVIDER_ID = 2117; /* [OMADDSChargingData] */
public static final int AC_CONTENT_SIZE = 1206; /* [32-299-f10] */
public static final int AC_CONTENT_TYPE = 826; /* [32-299-f10] */
public static final int AC_CONTENT_VERSION = 552; /* [29.214-f10] */
public static final int AC_CONTEXT_IDENTIFIER = 1423; /* [29.272-f10] */
public static final int AC_COST_INFORMATION = 423; /* [RFC 4006] */
public static final int AC_COST_UNIT = 424; /* [RFC 4006] */
public static final int AC_COUNTER_VALUE = 4319; /* [29.128-f10] */
public static final int AC_COUPLED_NODE_DIAMETER_ID = 1666; /* [29.272-f10] */
public static final int AC_CP_CIOT_EPS_OPTIMISATION_INDICATOR = 3930; /* [32-299-f10] */
public static final int AC_CREDIT_CONTROL = 426; /* [RFC 4006] */
public static final int AC_CREDIT_CONTROL_FAILURE_HANDLING = 427; /* [RFC 4006] */
public static final int AC_CREDIT_MANAGEMENT_STATUS = 1082; /* [29.212-f10] */
public static final int AC_CS_SERVICE_QOS_REQUEST_IDENTIFIER = 2807; /* [29.212-f10] */
public static final int AC_CS_SERVICE_QOS_REQUEST_OPERATION = 2808; /* [29.212-f10] */
public static final int AC_CS_SERVICE_RESOURCE_FAILURE_CAUSE = 2814; /* [29.212-f10] */
public static final int AC_CS_SERVICE_RESOURCE_REPORT = 2813; /* [29.212-f10] */
public static final int AC_CS_SERVICE_RESOURCE_RESULT_OPERATION = 2815; /* [29.212-f10] */
public static final int AC_CSG_ACCESS_MODE = 2317; /* [32-299-f10] */
public static final int AC_CSG_ID = 1437; /* [29.272-f10] */
public static final int AC_CSG_INFORMATION_REPORTING = 1071; /* [29.212-f10] */
public static final int AC_CSG_MEMBERSHIP_INDICATION = 2318; /* [32-299-f10] */
public static final int AC_CSG_SUBSCRIPTION_DATA = 1436; /* [29.272-f10] */
public static final int AC_CUG_INFORMATION = 2304; /* [32-299-f10] */
public static final int AC_CURRENCY_CODE = 425; /* [RFC 4006] */
public static final int AC_CURRENT_LOCATION = 707; /* [29.329-f00] */
public static final int AC_CURRENT_LOCATION_RETRIEVED = 1610; /* [29.272-f10] */
public static final int AC_CURRENT_TARIFF = 2056; /* [32-299-f10] */
public static final int AC_DATA_CODING_SCHEME = 2001; /* [32-299-f10] */
public static final int AC_DATA_REFERENCE = 703; /* [29.329-f00] */
public static final int AC_DAY_OF_MONTH_MASK = 564; /* [RFC 5777] */
public static final int AC_DAY_OF_WEEK_MASK = 563; /* [RFC 5777] */
public static final int AC_DAYLIGHT_SAVING_TIME = 1650; /* [29.272-f10] */
public static final int AC_DCD_INFORMATION = 2115; /* [OMADDSChargingData] */
public static final int AC_DEA_FLAGS = 1521; /* [29.273-f10] */
public static final int AC_DEFAULT_ACCESS = 2829; /* [29.212-f10] */
public static final int AC_DEFAULT_BEARER_INDICATION = 2844; /* [29.212-f10] */
public static final int AC_DEFAULT_EPS_BEARER_QOS = 1049; /* [29.212-f10] */
public static final int AC_DEFAULT_QOS_INFORMATION = 2816; /* [29.212-f10] */
public static final int AC_DEFAULT_QOS_NAME = 2817; /* [29.212-f10] */
public static final int AC_DEFERRED_LOCATION_EVENT_TYPE = 1230; /* [32-299-f10] */
public static final int AC_DEFERRED_LOCATION_TYPE = 2532; /* [29.172-d10] */
public static final int AC_DEFERRED_MT_LR_DATA = 2547; /* [29.172-d10] */
public static final int AC_DELAYED_LOCATION_REPORTING_DATA = 2555; /* [29.172-d10] */
public static final int AC_DELIVERY_OUTCOME = 3009; /* [29.368-e20] */
public static final int AC_DELIVERY_REPORT_REQUESTED = 1216; /* [32-299-f10] */
public static final int AC_DELIVERY_STATUS = 2104; /* [OMADDSChargingData] */
public static final int AC_DER_FLAGS = 1520; /* [29.273-f10] */
public static final int AC_DER_S6B_FLAGS = 1523; /* [29.273-f10] */
public static final int AC_DEREGISTRATION_REASON = 615; /* [29.229-e20] */
public static final int AC_DESTINATION_HOST = 293; /* [RFC 6733] */
public static final int AC_DESTINATION_INTERFACE = 2002; /* [32-299-f10] */
public static final int AC_DESTINATION_REALM = 283; /* [RFC 6733] */
public static final int AC_DESTINATION_SIP_URI = 3327; /* [29.338-f00] */
public static final int AC_DEVICE_ACTION = 3001; /* [29.368-e20] */
public static final int AC_DEVICE_NOTIFICATION = 3002; /* [29.368-e20] */
public static final int AC_DIAGNOSTICS = 2039; /* [32-299-f10] */
public static final int AC_DIFFSERV_CODE_POINT = 535; /* [RFC 5777] */
public static final int AC_DIGEST_ALGORITHM = 111; /* [29.229-e20] */
public static final int AC_DIGEST_HA1 = 121; /* [29.229-e20] */
public static final int AC_DIGEST_QOP = 110; /* [29.229-e20] */
public static final int AC_DIGEST_REALM = 104; /* [29.229-e20] */
public static final int AC_DIRECT_DEBITING_FAILURE_HANDLING = 428; /* [RFC 4006] */
public static final int AC_DIRECTION = 514; /* [RFC 5777] */
public static final int AC_DISCONNECT_CAUSE = 273; /* [RFC 6733] */
public static final int AC_DL_BUFFERING_SUGGESTED_PACKET_COUNT = 1674; /* [29.272-f10] */
public static final int AC_DOMAIN_NAME = 1200; /* [32-299-f10] */
public static final int AC_DOWNLINK_RATE_LIMIT = 4312; /* [29.128-f10] */
public static final int AC_DRA_BINDING = 2208; /* [29.215-f00] */
public static final int AC_DRA_DEPLOYMENT = 2206; /* [29.215-f00] */
public static final int AC_DRM_CONTENT = 1221; /* [32-299-f10] */
public static final int AC_DRMP = 301; /* [RFC 7944] */
public static final int AC_DSA_FLAGS = 1422; /* [29.272-f10] */
public static final int AC_DSAI_TAG = 711; /* [29.329-f00] */
public static final int AC_DSR_FLAGS = 1421; /* [29.272-f10] */
public static final int AC_DYNAMIC_ADDRESS_FLAG = 2051; /* [32-299-f10] */
public static final int AC_DYNAMIC_ADDRESS_FLAG_EXTENSION = 2068; /* [32-299-f10] */
public static final int AC_E_UTRAN_CELL_GLOBAL_IDENTITY = 1602; /* [29.272-f10] */
public static final int AC_E_UTRAN_VECTOR = 1414; /* [29.272-f10] */
public static final int AC_E2E_SEQUENCE = 300; /* [RFC 6733] */
public static final int AC_EAP_KEY_NAME = 102; /* [no_reference] */
public static final int AC_EAP_MASTER_SESSION_KEY = 464; /* [no_reference] */
public static final int AC_EAP_PAYLOAD = 462; /* [no_reference] */
public static final int AC_EAP_REISSUED_PAYLOAD = 463; /* [no_reference] */
public static final int AC_EARLY_MEDIA_DESCRIPTION = 1272; /* [32-299-f10] */
public static final int AC_ECGI = 2517; /* [29.172-d10] */
public static final int AC_EDRX_CYCLE_LENGTH = 1691; /* [29.272-f10] */
public static final int AC_EDRX_CYCLE_LENGTH_VALUE = 1692; /* [29.272-f10] */
public static final int AC_EMERGENCY_INFO = 1687; /* [29.272-f10] */
public static final int AC_EMERGENCY_SERVICES = 1538; /* [29.273-f10] */
public static final int AC_ENHANCED_COVERAGE_RESTRICTION = 3155; /* [29.336-f10] */
public static final int AC_ENHANCED_COVERAGE_RESTRICTION_DATA = 3156; /* [29.336-f10] */
public static final int AC_ENODEB_ID = 4008; /* [29.217-e20] */
public static final int AC_ENODEB_ID_S6 = 4008; /* [29.272-f10] */
public static final int AC_ENVELOPE = 1266; /* [32-299-f10] */
public static final int AC_ENVELOPE_END_TIME = 1267; /* [32-299-f10] */
public static final int AC_ENVELOPE_REPORTING = 1268; /* [32-299-f10] */
public static final int AC_ENVELOPE_START_TIME = 1269; /* [32-299-f10] */
public static final int AC_EPDG_ADDRESS = 3425; /* [32-299-f10] */
public static final int AC_EPS_LOCATION_INFORMATION = 1496; /* [29.272-f10] */
public static final int AC_EPS_SUBSCRIBED_QOS_PROFILE = 1431; /* [29.272-f10] */
public static final int AC_EPS_USER_STATE = 1495; /* [29.272-f10] */
public static final int AC_EQUIPMENT_STATUS = 1445; /* [29.272-f10] */
public static final int AC_EQUIVALENT_PLMN_LIST = 1637; /* [29.272-f10] */
public static final int AC_ERP_AUTHORIZATION = 1541; /* [29.273-f10] */
public static final int AC_ERP_REALM = 619; /* [RFC 6942] */
public static final int AC_ERP_RK_REQUEST = 618; /* [RFC 6942] */
public static final int AC_ERROR_DIAGNOSTIC = 1614; /* [29.272-f10] */
public static final int AC_ERROR_MESSAGE = 281; /* [RFC 6733] */
public static final int AC_ERROR_REPORTING_HOST = 294; /* [RFC 6733] */
public static final int AC_ESMLC_CELL_INFO = 2552; /* [29.172-d10] */
public static final int AC_ETH_ETHER_TYPE = 550; /* [RFC 5777] */
public static final int AC_ETH_OPTION = 548; /* [RFC 5777] */
public static final int AC_ETH_PROTO_TYPE = 549; /* [RFC 5777] */
public static final int AC_ETH_SAP = 551; /* [RFC 5777] */
public static final int AC_EUI64_ADDRESS = 527; /* [RFC 5777] */
public static final int AC_EUI64_ADDRESS_MASK = 528; /* [RFC 5777] */
public static final int AC_EUI64_ADDRESS_MASK_PATTERN = 529; /* [RFC 5777] */
public static final int AC_EUTRAN_POSITIONING_DATA = 2516; /* [29.172-d10] */
public static final int AC_EVENT = 825; /* [32-299-f10] */
public static final int AC_EVENT_CHARGING_TIMESTAMP = 1258; /* [32-299-f10] */
public static final int AC_EVENT_HANDLING = 3149; /* [29.336-f10] */
public static final int AC_EVENT_REPORT_INDICATION = 1033; /* [29.212-f10] */
public static final int AC_EVENT_THRESHOLD_EVENT_1F = 1661; /* [29.272-f10] */
public static final int AC_EVENT_THRESHOLD_EVENT_1I = 1662; /* [29.272-f10] */
public static final int AC_EVENT_THRESHOLD_RSRP = 1629; /* [29.272-a60] */
public static final int AC_EVENT_THRESHOLD_RSRQ = 1630; /* [29.272-a60] */
public static final int AC_EVENT_TIMESTAMP = 55; /* [RFC 6733] */
public static final int AC_EVENT_TRIGGER = 1006; /* [29.212-f10] */
public static final int AC_EVENT_TRIGGER_R11 = 1006; /* [29.212-f10] */
public static final int AC_EVENT_TYPE = 823; /* [32-299-f10] */
public static final int AC_EXCESS_TREATMENT = 577; /* [RFC 5777] */
public static final int AC_EXECUTION_TIME = 2839; /* [29.212-f10] */
public static final int AC_EXPERIMENTAL_RESULT = 297; /* [RFC 6733] */
public static final int AC_EXPERIMENTAL_RESULT_CODE = 298; /* [RFC 6733] */
public static final int AC_EXPIRATION_DATE = 1439; /* [29.272-f10] */
public static final int AC_EXPIRES = 888; /* [32-299-f10] */
public static final int AC_EXPIRY_TIME = 709; /* [29.329-f00] */
public static final int AC_EXPONENT = 429; /* [RFC 4006] */
public static final int AC_EXT_PDP_ADDRESS = 1621; /* [29.272-f10] */
public static final int AC_EXT_PDP_TYPE = 1620; /* [29.272-f10] */
public static final int AC_EXTENDED_APN_AMBR_DL = 2848; /* [29.212-f10] */
public static final int AC_EXTENDED_APN_AMBR_UL = 2849; /* [29.212-f10] */
public static final int AC_EXTENDED_ENODEB_ID = 4013; /* [29.217-e20] */
public static final int AC_EXTENDED_GBR_DL = 2850; /* [29.212-f10] */
public static final int AC_EXTENDED_GBR_UL = 2851; /* [29.212-f10] */
public static final int AC_EXTENDED_MAX_REQUESTED_BW_DL = 554; /* [29.214-f10] */
public static final int AC_EXTENDED_MAX_REQUESTED_BW_UL = 555; /* [29.214-f10] */
public static final int AC_EXTENDED_MAX_SUPPORTED_BW_DL = 556; /* [29.214-f10] */
public static final int AC_EXTENDED_MAX_SUPPORTED_BW_UL = 557; /* [29.214-f10] */
public static final int AC_EXTENDED_MIN_DESIRED_BW_DL = 558; /* [29.214-f10] */
public static final int AC_EXTENDED_MIN_DESIRED_BW_UL = 559; /* [29.214-f10] */
public static final int AC_EXTENDED_MIN_REQUESTED_BW_DL = 560; /* [29.214-f10] */
public static final int AC_EXTENDED_MIN_REQUESTED_BW_UL = 561; /* [29.214-f10] */
public static final int AC_EXTENDED_PCO = 4313; /* [29.128-f10] */
public static final int AC_EXTERNAL_CLIENT = 1479; /* [29.272-f10] */
public static final int AC_EXTERNAL_IDENTIFIER = 3111; /* [29.336-f10] */
public static final int AC_EXTERNAL_IDENTIFIER_S6 = 3111; /* [29.272-f10] */
public static final int AC_FAILED_AVP = 279; /* [RFC 6733] */
public static final int AC_FEATURE_LIST = 630; /* [29.229-e20] */
public static final int AC_FEATURE_LIST_ID = 629; /* [29.229-e20] */
public static final int AC_FEATURE_LIST_S6T = 630; /* [29.336-f10] */
public static final int AC_FEATURE_LIST_T6 = 630; /* [29.128-f10] */
public static final int AC_FEATURE_SUPPORTED_IN_FINAL_TARGET = 3012; /* [29.368-e20] */
public static final int AC_FILE_REPAIR_SUPPORTED = 1224; /* [32-299-f10] */
public static final int AC_FILTER_ID = 11; /* [RFC 7155] */
public static final int AC_FILTER_Id = 11; /* [RFC 2865] */
public static final int AC_FILTER_RULE = 509; /* [RFC 5777] */
public static final int AC_FILTER_RULE_PRECEDENCE = 510; /* [RFC 5777] */
public static final int AC_FINAL_UNIT_ACTION = 449; /* [RFC 4006] */
public static final int AC_FINAL_UNIT_INDICATION = 430; /* [RFC 4006] */
public static final int AC_FIRMWARE_REVISION = 267; /* [RFC 6733] */
public static final int AC_FIXED_USER_LOCATION_INFO = 2825; /* [29.212-f10] */
public static final int AC_FLOW_DESCRIPTION = 507; /* [29.214-f10] */
public static final int AC_FLOW_DIRECTION = 1080; /* [29.212-f10] */
public static final int AC_FLOW_INFORMATION = 1058; /* [29.212-f10] */
public static final int AC_FLOW_LABEL = 1057; /* [29.212-f10] */
public static final int AC_FLOW_NUMBER = 509; /* [29.214-f10] */
public static final int AC_FLOW_STATUS = 511; /* [29.214-f10] */
public static final int AC_FLOW_USAGE = 512; /* [29.214-f10] */
public static final int AC_FLOWS = 510; /* [29.214-f10] */
public static final int AC_FRAGMENTATION_FLAG = 536; /* [RFC 5777] */
public static final int AC_FRAMED_APPLETALK_LINK = 37; /* [RFC 7155] */
public static final int AC_FRAMED_APPLETALK_NETWORK = 38; /* [RFC 7155] */
public static final int AC_FRAMED_APPLETALK_ZONE = 39; /* [RFC 7155] */
public static final int AC_FRAMED_COMPRESSION = 13; /* [RFC 7155] */
public static final int AC_FRAMED_INTERFACE_ID = 96; /* [RFC 7155] */
public static final int AC_FRAMED_IP_ADDRESS = 8; /* [RFC 7155] */
public static final int AC_FRAMED_IP_NETMASK = 9; /* [RFC 7155] */
public static final int AC_FRAMED_IPV6_POOL = 100; /* [RFC 7155] */
public static final int AC_FRAMED_IPV6_PREFIX = 97; /* [RFC 7155] */
public static final int AC_FRAMED_IPV6_ROUTE = 99; /* [RFC 7155] */
public static final int AC_FRAMED_IPX_NETWORK = 23; /* [RFC 7155] */
public static final int AC_FRAMED_MTU = 12; /* [RFC 7155] */
public static final int AC_FRAMED_POOL = 88; /* [RFC 7155] */
public static final int AC_FRAMED_PROTOCOL = 7; /* [RFC 7155] */
public static final int AC_FRAMED_ROUTE = 22; /* [RFC 7155] */
public static final int AC_FRAMED_ROUTING = 10; /* [RFC 7155] */
public static final int AC_FROM_SIP_HEADER = 644; /* [29.229-e20] */
public static final int AC_FROM_SPEC = 515; /* [RFC 5777] */
public static final int AC_FULL_NETWORK_NAME = 1516; /* [29.273-f10] */
public static final int AC_G_S_U_POOL_IDENTIFIER = 453; /* [RFC 4006] */
public static final int AC_G_S_U_POOL_REFERENCE = 457; /* [RFC 4006] */
public static final int AC_GCS_IDENTIFIER = 538; /* [29.214-f10] */
public static final int AC_GEODETIC_INFORMATION = 1609; /* [29.272-f10] */
public static final int AC_GEOGRAPHICAL_INFORMATION = 1608; /* [29.272-f10] */
public static final int AC_GERAN_GANSS_POSTIONING_DATA = 2526; /* [29.172-d10] */
public static final int AC_GERAN_POSITIONING_DATA = 2525; /* [29.172-d10] */
public static final int AC_GERAN_POSITIONING_INFO = 2524; /* [29.172-d10] */
public static final int AC_GERAN_VECTOR = 1416; /* [29.272-f10] */
public static final int AC_GGSN_ADDRESS = 847; /* [32-299-f10] */
public static final int AC_GMLC_ADDRESS = 2405; /* [29.173-e00] */
public static final int AC_GMLC_ADDRESS_S6 = 2405; /* [29.173] */
public static final int AC_GMLC_NUMBER = 1474; /* [29.272-f10] */
public static final int AC_GMLC_RESTRICTION = 1481; /* [29.272-f10] */
public static final int AC_GMM_CAUSE = 4304; /* [29.128-f10] */
public static final int AC_GPRS_SUBSCRIPTION_DATA = 1467; /* [29.272-f10] */
public static final int AC_GRANTED_SERVICE_UNIT = 431; /* [RFC 4006] */
public static final int AC_GRANTED_VALIDITY_TIME = 3160; /* [29.336-f10] */
public static final int AC_GROUP_MONITORING_EVENT_REPORT = 3165; /* [29.336-f10] */
public static final int AC_GROUP_MONITORING_EVENT_REPORT_ITEM = 3166; /* [29.336-f10] */
public static final int AC_GROUP_PLMN_ID = 1677; /* [29.272-f10] */
public static final int AC_GROUP_REPORTING_GUARD_TIMER = 3163; /* [29.336-f10] */
public static final int AC_GROUP_SERVICE_ID = 1676; /* [29.272-f10] */
public static final int AC_GUARANTEED_BITRATE_DL = 1025; /* [29.212-f10] */
public static final int AC_GUARANTEED_BITRATE_UL = 1026; /* [29.212-f10] */
public static final int AC_HENB_BBF_FQDN = 2803; /* [29.212-f10] */
public static final int AC_HENB_LOCAL_IP_ADDRESS = 2804; /* [29.212-f10] */
public static final int AC_HESSID = 1525; /* [29.273-f10] */
public static final int AC_HIGH_USER_PRIORITY = 559; /* [RFC 5777] */
public static final int AC_HOMOGENEOUS_SUPPORT_OF_IMS_VOICE_OVER_PS_SESSIONS = 1493; /* [29.272-f10] */
public static final int AC_HORIZONTAL_ACCURACY = 2505; /* [29.172-d10] */
public static final int AC_HOST_IP_ADDRESS = 257; /* [RFC 6733] */
public static final int AC_HPLMN_ODB = 1418; /* [29.272-f10] */
public static final int AC_HSS_CAUSE = 3109; /* [29.336-f10] */
public static final int AC_HSS_ID = 3325; /* [29.338-f00] */
public static final int AC_ICMP_CODE = 547; /* [RFC 5777] */
public static final int AC_ICMP_TYPE = 545; /* [RFC 5777] */
public static final int AC_ICMP_TYPE_NUMBER = 546; /* [RFC 5777] */
public static final int AC_ICS_INDICATOR = 1491; /* [29.272-f10] */
public static final int AC_IDA_FLAGS = 1441; /* [29.272-f10] */
public static final int AC_IDENTITY_SET = 708; /* [29.329-f00] */
public static final int AC_IDENTITY_WITH_EMERGENCY_REGISTRATION = 651; /* [29.229-e20] */
public static final int AC_IDLE_STATUS_INDICATION = 4322; /* [29.128-f00] */
public static final int AC_IDLE_STATUS_INDICATION_S6T = 4322; /* [29.336-f10] */
public static final int AC_IDLE_STATUS_TIMESTAMP = 4323; /* [29.128-f00] */
public static final int AC_IDLE_TIMEOUT = 28; /* [RFC 7155] */
public static final int AC_IDR_FLAGS = 1490; /* [29.272-f10] */
public static final int AC_IM_INFORMATION = 2110; /* [OMADDSChargingData] */
public static final int AC_IMEI = 1402; /* [29.272-f10] */
public static final int AC_IMEI_CHANGE = 3141; /* [29.336-f10] */
public static final int AC_IMMEDIATE_RESPONSE_PREFERRED = 1412; /* [29.272-f10] */
public static final int AC_IMS_APPLICATION_REFERENCE_IDENTIFIER = 2601; /* [32-299-f10] */
public static final int AC_IMS_CHARGING_IDENTIFIER = 841; /* [32-299-f10] */
public static final int AC_IMS_COMMUNICATION_SERVICE_IDENTIFIER = 1281; /* [32-299-f10] */
public static final int AC_IMS_INFORMATION = 876; /* [32-299-f10] */
public static final int AC_IMS_VOICE_OVER_PS_SESSIONS_SUPPORTED = 1492; /* [29.272-f10] */
public static final int AC_IMSI_GROUP_ID = 1675; /* [29.272-f10] */
public static final int AC_IMSI_LIST = 4009; /* [29.217-e20] */
public static final int AC_IMSI_UNAUTHENTICATED_FLAG = 2308; /* [32-299-f10] */
public static final int AC_INBAND_SECURITY_ID = 299; /* [RFC 6733] */
public static final int AC_INCOMING_TRUNK_GROUP_ID = 852; /* [32-299-f10] */
public static final int AC_INCREMENTAL_COST = 2062; /* [32-299-f10] */
public static final int AC_INITIAL_CSEQ_SEQUENCE_NUMBER = 654; /* [29.229-e20] */
public static final int AC_INITIAL_IMS_CHARGING_IDENTIFIER = 2321; /* [32-299-f10] */
public static final int AC_INTEGRITY_KEY = 626; /* [29.229-e20] */
public static final int AC_INTER_OPERATOR_IDENTIFIER = 838; /* [32-299-f10] */
public static final int AC_INTERFACE_ID = 2003; /* [32-299-f10] */
public static final int AC_INTERFACE_PORT = 2004; /* [32-299-f10] */
public static final int AC_INTERFACE_TEXT = 2005; /* [32-299-f10] */
public static final int AC_INTERFACE_TYPE = 2006; /* [32-299-f10] */
public static final int AC_INTERVAL_TIME = 2539; /* [29.172-d10] */
public static final int AC_IP_ADDRESS = 518; /* [RFC 5777] */
public static final int AC_IP_ADDRESS_END = 521; /* [RFC 5777] */
public static final int AC_IP_ADDRESS_MASK = 522; /* [RFC 5777] */
public static final int AC_IP_ADDRESS_RANGE = 519; /* [RFC 5777] */
public static final int AC_IP_ADDRESS_START = 520; /* [RFC 5777] */
public static final int AC_IP_BIT_MASK_WIDTH = 523; /* [RFC 5777] */
public static final int AC_IP_CAN_SESSION_CHARGING_SCOPE = 2827; /* [29.212-f10] */
public static final int AC_IP_CAN_TYPE = 1027; /* [29.212-f10] */
public static final int AC_IP_DOMAIN_ID = 537; /* [29.214-f10] */
public static final int AC_IP_OPTION = 537; /* [RFC 5777] */
public static final int AC_IP_OPTION_TYPE = 538; /* [RFC 5777] */
public static final int AC_IP_OPTION_VALUE = 539; /* [RFC 5777] */
public static final int AC_IP_REALM_DEFAULT_INDICATION = 2603; /* [32-299-f10] */
public static final int AC_IP_SM_GW_NAME = 3101; /* [29.336-f10] */
public static final int AC_IP_SM_GW_NUMBER = 3100; /* [29.336-f10] */
public static final int AC_IP_SM_GW_REALM = 3112; /* [29.336-f10] */
public static final int AC_IP_SM_GW_SM_DELIVERY_OUTCOME = 3320; /* [29.338-f00] */
public static final int AC_ITEM_NUMBER = 1419; /* [29.272-f10] */
public static final int AC_JOB_TYPE = 1623; /* [29.272-f10] */
public static final int AC_KASME = 1450; /* [29.272-f10] */
public static final int AC_KC = 1453; /* [29.272-f10] */
public static final int AC_KEY = 581; /* [RFC 6734] */
public static final int AC_KEY_LIFETIME = 584; /* [RFC 6734] */
public static final int AC_KEY_NAME = 586; /* [RFC 6734] */
public static final int AC_KEY_SPI = 585; /* [RFC 6734] */
public static final int AC_KEY_TYPE = 582; /* [RFC 6734] */
public static final int AC_KEYING_MATERIAL = 583; /* [RFC 6734] */
public static final int AC_LAST_UE_ACTIVITY_TIME = 1494; /* [29.272-f10] */
public static final int AC_LCS_APN = 1231; /* [32-299-f10] */
public static final int AC_LCS_CAPABILITIES_SETS = 2404; /* [29.173-e00] */
public static final int AC_LCS_CLIENT_DIALED_BY_MS = 1233; /* [32-299-f10] */
public static final int AC_LCS_CLIENT_EXTERNAL_ID = 1234; /* [32-299-f10] */
public static final int AC_LCS_CLIENT_ID = 1232; /* [32-299-f10] */
public static final int AC_LCS_CLIENT_NAME = 1235; /* [32-299-f10] */
public static final int AC_LCS_CLIENT_TYPE = 1241; /* [32-299-f10] */
public static final int AC_LCS_CODEWORD = 2511; /* [29.172-d10] */
public static final int AC_LCS_DATA_CODING_SCHEME = 1236; /* [32-299-f10] */
public static final int AC_LCS_EPS_CLIENT_NAME = 2501; /* [29.172-d10] */
public static final int AC_LCS_FORMAT_INDICATOR = 1237; /* [32-299-f10] */
public static final int AC_LCS_INFO = 1473; /* [29.272-f10] */
public static final int AC_LCS_INFORMATION = 878; /* [32-299-f10] */
public static final int AC_LCS_NAME_STRING = 1238; /* [32-299-f10] */
public static final int AC_LCS_PRIORITY = 2503; /* [29.172-d10] */
public static final int AC_LCS_PRIVACY_CHECK = 2512; /* [29.172-d10] */
public static final int AC_LCS_PRIVACY_CHECK_NON_SESSION = 2521; /* [29.172-d10] */
public static final int AC_LCS_PRIVACY_CHECK_SESSION = 2522; /* [29.172-d10] */
public static final int AC_LCS_PRIVACYEXCEPTION = 1475; /* [29.272-f10] */
public static final int AC_LCS_QOS = 2504; /* [29.172-d10] */
public static final int AC_LCS_QOS_CLASS = 2523; /* [29.172-d10] */
public static final int AC_LCS_REFERENCE_NUMBER = 2531; /* [29.172-d10] */
public static final int AC_LCS_REQUESTOR_ID = 1239; /* [32-299-f10] */
public static final int AC_LCS_REQUESTOR_ID_STRING = 1240; /* [32-299-f10] */
public static final int AC_LCS_REQUESTOR_NAME = 2502; /* [29.172-d10] */
public static final int AC_LCS_SERVICE_TYPE_ID = 2520; /* [29.172-d10] */
public static final int AC_LCS_SUPPORTED_GAD_SHAPES = 2510; /* [29.172-d10] */
public static final int AC_LIA_FLAGS = 653; /* [29.229-e20] */
public static final int AC_LINE_IDENTIFIER = 500; /* [29.229-e20] */
public static final int AC_LIPA_PERMISSION = 1618; /* [29.272-f10] */
public static final int AC_LIST_OF_MEASUREMENTS = 1625; /* [29.272-f10] */
public static final int AC_LMSI = 2400; /* [29.173-e00] */
public static final int AC_LOCAL_GROUP_ID = 1678; /* [29.272-f10] */
public static final int AC_LOCAL_GW_INSERTED_INDICATION = 2604; /* [32-299-f10] */
public static final int AC_LOCAL_SEQUENCE_NUMBER = 2063; /* [32-299-f10] */
public static final int AC_LOCAL_TIME_ZONE = 1649; /* [29.272-f10] */
public static final int AC_LOCAL_TIME_ZONE_INDICATION = 718; /* [29.329-f00] */
public static final int AC_LOCATION_AREA_IDENTITY = 1606; /* [29.272-f10] */
public static final int AC_LOCATION_ESTIMATE = 1242; /* [32-299-f10] */
public static final int AC_LOCATION_ESTIMATE_TYPE = 1243; /* [32-299-f10] */
public static final int AC_LOCATION_EVENT = 2518; /* [29.172-d10] */
public static final int AC_LOCATION_INFORMATION = 127; /* [RFC 5580] */
public static final int AC_LOCATION_INFORMATION_CONFIGURATION = 3135; /* [29.336-f10] */
public static final int AC_LOCATION_INFORMATION_CONFIGURATION_S6 = 3135; /* [29.272-f10] */
public static final int AC_LOCATION_TYPE = 1244; /* [32-299-f10] */
public static final int AC_LOGGING_DURATION = 1632; /* [29.272-a60] */
public static final int AC_LOGGING_INTERVAL = 1631; /* [29.272-a60] */
public static final int AC_LOGICAL_ACCESS_ID = 302; /* [ETSI ES 283 034] */
public static final int AC_LOGIN_IP_HOST = 14; /* [RFC 7155] */
public static final int AC_LOGIN_IPV6_HOST = 98; /* [RFC 7155] */
public static final int AC_LOGIN_LAT_GROUP = 36; /* [RFC 7155] */
public static final int AC_LOGIN_LAT_NODE = 35; /* [RFC 7155] */
public static final int AC_LOGIN_LAT_PORT = 63; /* [RFC 7155] */
public static final int AC_LOGIN_LAT_SERVICE = 34; /* [RFC 7155] */
public static final int AC_LOGIN_SERVICE = 15; /* [RFC 7155] */
public static final int AC_LOGIN_TCP_PORT = 16; /* [RFC 7155] */
public static final int AC_LOOSE_ROUTE_INDICATION = 638; /* [29.229-e20] */
public static final int AC_LOSS_OF_CONNECTIVITY_REASON = 3162; /* [29.336-f10] */
public static final int AC_LOW_BALANCE_INDICATION = 2020; /* [32-299-f10] */
public static final int AC_LOW_PRIORITY_INDICATOR = 2602; /* [32-299-f10] */
public static final int AC_LOW_USER_PRIORITY = 558; /* [RFC 5777] */
public static final int AC_LRA_FLAGS = 2549; /* [29.172-d10] */
public static final int AC_LRR_FLAGS = 2530; /* [29.172-d10] */
public static final int AC_MAC_ADDRESS = 524; /* [RFC 5777] */
public static final int AC_MAC_ADDRESS_MASK = 525; /* [RFC 5777] */
public static final int AC_MAC_ADDRESS_MASK_PATTERN = 526; /* [RFC 5777] */
public static final int AC_MANDATORY_CAPABILITY = 604; /* [29.229-e20] */
public static final int AC_MAX_REQUESTED_BANDWIDTH = 313; /* [29.234-b20] */
public static final int AC_MAX_REQUESTED_BANDWIDTH_DL = 515; /* [29.214-f10] */
public static final int AC_MAX_REQUESTED_BANDWIDTH_UL = 516; /* [29.214-f10] */
public static final int AC_MAX_SUPPORTED_BANDWIDTH_DL = 1083; /* [29.212-f10] */
public static final int AC_MAX_SUPPORTED_BANDWIDTH_UL = 1084; /* [29.212-f10] */
public static final int AC_MAXIMUM_BANDWIDTH = 1082; /* [29.212-f10] */
public static final int AC_MAXIMUM_DETECTION_TIME = 3131; /* [29.336-f10] */
public static final int AC_MAXIMUM_LATENCY = 3133; /* [29.336-f10] */
public static final int AC_MAXIMUM_NUMBER_ACCESSES = 319; /* [29.234-b20] */
public static final int AC_MAXIMUM_NUMBER_OF_REPORTS = 3128; /* [29.336-f10] */
public static final int AC_MAXIMUM_NUMBER_OF_REPORTS_S6 = 3128; /* [29.272-f10] */
public static final int AC_MAXIMUM_RESPONSE_TIME = 3134; /* [29.336-f10] */
public static final int AC_MAXIMUM_RETRANSMISSION_TIME = 3330; /* [29.338-f00] */
public static final int AC_MAXIMUM_UE_AVAILABILITY_TIME = 3329; /* [29.338-f00] */
public static final int AC_MAXIMUM_WAIT_TIME = 1537; /* [29.273-f10] */
public static final int AC_MBMS_GW_ADDRESS = 2307; /* [32-299-f10] */
public static final int AC_MBMS_2G_3G_INDICATOR = 907; /* [29.061-f10] */
public static final int AC_MBMS_INFORMATION = 880; /* [32-299-f10] */
public static final int AC_MBMS_SERVICE_AREA = 903; /* [29.061-f10] */
public static final int AC_MBMS_SERVICE_TYPE = 906; /* [29.061-f10] */
public static final int AC_MBMS_SESSION_IDENTITY = 908; /* [29.061-f10] */
public static final int AC_MBMS_USER_SERVICE_TYPE = 1225; /* [32-299-f10] */
public static final int AC_MBSFN_AREA = 1694; /* [29.272-f10] */
public static final int AC_MBSFN_AREA_ID = 1695; /* [29.272-f10] */
public static final int AC_MCPTT_IDENTIFIER = 547; /* [29.214-f10] */
public static final int AC_MDT_ALLOWED_PLMN_ID = 1671; /* [29.272-f10] */
public static final int AC_MDT_CONFIGURATION = 1622; /* [29.272-f10] */
public static final int AC_MDT_USER_CONSENT = 1634; /* [29.272-a60] */
public static final int AC_MEASUREMENT_PERIOD_LTE = 1655; /* [29.272-f10] */
public static final int AC_MEASUREMENT_PERIOD_UMTS = 1656; /* [29.272-f10] */
public static final int AC_MEASUREMENT_QUANTITY = 1660; /* [29.272-f10] */
public static final int AC_MEDIA_COMPONENT_DESCRIPTION = 517; /* [29.214-f10] */
public static final int AC_MEDIA_COMPONENT_NUMBER = 518; /* [29.214-f10] */
public static final int AC_MEDIA_COMPONENT_STATUS = 549; /* [29.214-f10] */
public static final int AC_MEDIA_INITIATOR_FLAG = 882; /* [32-299-f10] */
public static final int AC_MEDIA_INITIATOR_PARTY = 1288; /* [32-299-f10] */
public static final int AC_MEDIA_SUB_COMPONENT = 519; /* [29.214-f10] */
public static final int AC_MEDIA_TYPE = 520; /* [29.214-f10] */
public static final int AC_MESSAGE_BODY = 889; /* [32-299-f10] */
public static final int AC_MESSAGE_CLASS = 1213; /* [32-299-f10] */
public static final int AC_MESSAGE_ID = 1210; /* [32-299-f10] */
public static final int AC_MESSAGE_SIZE = 1212; /* [32-299-f10] */
public static final int AC_MESSAGE_TYPE = 1211; /* [32-299-f10] */
public static final int AC_METERING_METHOD = 1007; /* [29.212-f10] */
public static final int AC_MIN_REQUESTED_BANDWIDTH_DL = 534; /* [29.214-f10] */
public static final int AC_MIN_REQUESTED_BANDWIDTH_UL = 535; /* [29.214-f10] */
public static final int AC_MIP_CAREOF_ADDRESS = 487; /* [RFC 5778] */
public static final int AC_MIP_FA_RK = 1506; /* [29.273-f10] */
public static final int AC_MIP_FA_RK_SPI = 1507; /* [29.273-f10] */
public static final int AC_MIP_HOME_AGENT_ADDRESS = 334; /* [RFC 4004] */
public static final int AC_MIP_HOME_AGENT_HOST = 348; /* [RFC 4004] */
public static final int AC_MIP6_AGENT_INFO = 486; /* [RFC 5447] */
public static final int AC_MIP6_FEATURE_VECTOR = 124; /* [RFC 5447] */
public static final int AC_MIP6_HOME_LINK_PREFIX = 125; /* [RFC 5447] */
public static final int AC_MM_CONTENT_TYPE = 1203; /* [32-299-f10] */
public static final int AC_MMBOX_STORAGE_REQUESTED = 1248; /* [32-299-f10] */
public static final int AC_MME_ABSENT_USER_DIAGNOSTIC_SM = 3313; /* [29.338-f00] */
public static final int AC_MME_LOCATION_INFORMATION = 1600; /* [29.272-f10] */
public static final int AC_MME_LOCATION_INFORMATION_S6T = 1600; /* [29.336-f10] */
public static final int AC_MME_NAME = 2402; /* [29.173-e00] */
public static final int AC_MME_NUMBER_FOR_MT_SMS = 1645; /* [29.272-f10] */
public static final int AC_MME_REALM = 2408; /* [29.173-e00] */
public static final int AC_MME_SM_DELIVERY_OUTCOME = 3317; /* [29.338-f00] */
public static final int AC_MME_USER_STATE = 1497; /* [29.272-f10] */
public static final int AC_MMS_INFORMATION = 877; /* [32-299-f10] */
public static final int AC_MMTEL_INFORMATION = 2030; /* [32-299-f10] */
public static final int AC_MMTEL_SSERVICE_TYPE = 2031; /* [32-299-f10] */
public static final int AC_MO_LR = 1485; /* [29.272-f10] */
public static final int AC_MOBILE_NODE_IDENTIFIER = 506; /* [29.273-f10] */
public static final int AC_MONITORING_DURATION = 3130; /* [29.336-f10] */
public static final int AC_MONITORING_DURATION_S6 = 3130; /* [29.272-f10] */
public static final int AC_MONITORING_EVENT_CONFIG_STATUS = 3142; /* [29.336-f10] */
public static final int AC_MONITORING_EVENT_CONFIG_STATUS_S6 = 3142; /* [29.272-f10] */
public static final int AC_MONITORING_EVENT_CONFIGURATION = 3122; /* [29.336-f10] */
public static final int AC_MONITORING_EVENT_CONFIGURATION_T6 = 3122; /* [29.128-f10] */
public static final int AC_MONITORING_EVENT_CONFIGURATION_S6 = 3122; /* [29.272-f10] */
public static final int AC_MONITORING_EVENT_REPORT = 3123; /* [29.336-f10] */
public static final int AC_MONITORING_EVENT_REPORT_STATUS = 3171; /* [29.336-f10] */
public static final int AC_MONITORING_EVENT_REPORT_T6 = 3123; /* [29.128-f10] */
public static final int AC_MONITORING_EVENT_REPORT_S6 = 3123; /* [29.272-f10] */
public static final int AC_MONITORING_FLAGS = 2828; /* [29.212-f10] */
public static final int AC_MONITORING_KEY = 1066; /* [29.212-f10] */
public static final int AC_MONITORING_TIME = 2810; /* [29.212-f10] */
public static final int AC_MONITORING_TYPE = 3127; /* [29.336-f10] */
public static final int AC_MONITORING_TYPE_S6 = 3127; /* [29.272-f10] */
public static final int AC_MONTE_LOCATION_TYPE = 3136; /* [29.336-f10] */
public static final int AC_MONTH_OF_YEAR_MASK = 565; /* [RFC 5777] */
public static final int AC_MPS_IDENTIFIER = 528; /* [29.214-f10] */
public static final int AC_MPS_PRIORITY = 1616; /* [29.272-f10] */
public static final int AC_MSC_ABSENT_USER_DIAGNOSTIC_SM = 3314; /* [29.338-f00] */
public static final int AC_MSC_NUMBER = 2403; /* [29.173-e00] */
public static final int AC_MSC_SM_DELIVERY_OUTCOME = 3318; /* [29.338-f00] */
public static final int AC_MSISDN = 701; /* [29.329-f00] */
public static final int AC_MTC_ERROR_DIAGNOSTIC = 3203; /* [29.337-e10] */
public static final int AC_MULTI_ROUND_TIME_OUT = 272; /* [RFC 6733] */
public static final int AC_MULTIPLE_BBERF_ACTION = 2204; /* [29.215-f00] */
public static final int AC_MULTIPLE_REGISTRATION_INDICATION = 648; /* [29.229-e20] */
public static final int AC_MULTIPLE_SERVICES_CREDIT_CONTROL = 456; /* [RFC 4006] */
public static final int AC_MULTIPLE_SERVICES_CREDIT_CONTROL_32299 = 456; /* [32-299-f10] */
public static final int AC_MULTIPLE_SERVICES_INDICATOR = 455; /* [RFC 4006] */
public static final int AC_MUTE_NOTIFICATION = 2809; /* [29.212-f10] */
public static final int AC_MWD_STATUS = 3312; /* [29.338-f00] */
public static final int AC_NAS_FILTER_RULE = 400; /* [RFC 7155] */
public static final int AC_NAS_IDENTIFIER = 32; /* [RFC 7155] */
public static final int AC_NAS_IP_ADDRESS = 4; /* [RFC 7155] */
public static final int AC_NAS_IPV6_ADDRESS = 95; /* [RFC 7155] */
public static final int AC_NAS_PORT = 5; /* [RFC 7155] */
public static final int AC_NAS_PORT_ID = 87; /* [RFC 7155] */
public static final int AC_NAS_PORT_TYPE = 61; /* [RFC 7155] */
public static final int AC_NBIFOM_MODE = 2830; /* [29.212-f10] */
public static final int AC_NBIFOM_SUPPORT = 2831; /* [29.212-f10] */
public static final int AC_NEGATED = 517; /* [RFC 5777] */
public static final int AC_NetLoc_ACCESS_SUPPORT = 2824; /* [29.212-f10] */
public static final int AC_NETLOC_ACCESS_SUPPORT = 2824; /* [29.212-f10] */
public static final int AC_NETWORK_ACCESS_MODE = 1417; /* [29.272-f10] */
public static final int AC_NETWORK_AREA_INFO_LIST = 4201; /* [29.154-f00] */
public static final int AC_NETWORK_CONGESTION_AREA_REPORT = 4101; /* [29.153-e00] */
public static final int AC_NETWORK_REQUEST_SUPPORT = 1024; /* [29.212-f10] */
public static final int AC_NEXT_TARIFF = 2057; /* [32-299-f10] */
public static final int AC_NIDD_AUTHORIZATION_REQUEST = 3150; /* [29.336-f10] */
public static final int AC_NIDD_AUTHORIZATION_RESPONSE = 3151; /* [29.336-f10] */
public static final int AC_NIDD_AUTHORIZATION_UPDATE = 3161; /* [29.336-f10] */
public static final int AC_NODE_FUNCTIONALITY = 862; /* [32-299-f10] */
public static final int AC_NODE_ID = 2064; /* [32-299-f10] */
public static final int AC_NODE_TYPE = 3153; /* [29.336-f10] */
public static final int AC_NON_3GPP_IP_ACCESS = 1501; /* [29.273-f10] */
public static final int AC_NON_3GPP_IP_ACCESS_APN = 1502; /* [29.273-f10] */
public static final int AC_NON_3GPP_USER_DATA = 1500; /* [29.273-f10] */
public static final int AC_NON_IP_DATA = 4315; /* [29.128-f10] */
public static final int AC_NON_IP_DATA_DELIVERY_MECHANISM = 1682; /* [29.272-f10] */
public static final int AC_NON_IP_PDN_TYPE_INDICATOR = 1681; /* [29.272-f10] */
public static final int AC_NOR_FLAGS = 1443; /* [29.272-f10] */
public static final int AC_NOTIFICATION_TO_UE_USER = 1478; /* [29.272-f10] */
public static final int AC_NS_REQUEST_TYPE = 4102; /* [29.153-e00] */
public static final int AC_NUMBER_OF_DIVERSIONS = 2034; /* [32-299-f10] */
public static final int AC_NUMBER_OF_MESSAGES_SENT = 2019; /* [32-299-f10] */
public static final int AC_NUMBER_OF_MESSAGES_SUCCESSFULLY_EXPLODED = 2111; /* [OMADDSChargingData] */
public static final int AC_NUMBER_OF_MESSAGES_SUCCESSFULLY_SENT = 2112; /* [OMADDSChargingData] */
public static final int AC_NUMBER_OF_PARTICIPANTS = 885; /* [32-299-f10] */
public static final int AC_NUMBER_OF_RECEIVED_TALK_BURSTS = 1282; /* [32-299-f10] */
public static final int AC_NUMBER_OF_REQUESTED_VECTORS = 1410; /* [29.272-f10] */
public static final int AC_NUMBER_OF_TALK_BURSTS = 1283; /* [32-299-f10] */
public static final int AC_NUMBER_OF_UE_PER_LOCATION_CONFIGURATION = 4306; /* [29.128-f10] */
public static final int AC_NUMBER_OF_UE_PER_LOCATION_REPORT = 4307; /* [29.128-f10] */
public static final int AC_NUMBER_OF_UES = 4209; /* [29.154-f00] */
public static final int AC_NUMBER_PORTABILITY_ROUTING_INFORMATION = 2024; /* [32-299-f10] */
public static final int AC_OC_FEATURE_VECTOR = 622; /* [RFC 7683] */
public static final int AC_OC_OLR = 623; /* [RFC 7683] */
public static final int AC_OC_OLR_S6 = 623; /* [29.272-f10] */
public static final int AC_OC_REDUCTION_PERCENTAGE = 627; /* [RFC 7683] */
public static final int AC_OC_REPORT_TYPE = 626; /* [RFC 7683] */
public static final int AC_OC_SEQUENCE_NUMBER = 624; /* [RFC 7683] */
public static final int AC_OC_SUPPORTED_FEATURES = 621; /* [RFC 7683] */
public static final int AC_OC_SUPPORTED_FEATURES_S6 = 621; /* [29.272-f10] */
public static final int AC_OC_VALIDITY_DURATION = 625; /* [RFC 7683] */
public static final int AC_OCCURRENCE_INFO = 2538; /* [29.172-d10] */
public static final int AC_OFFLINE = 1008; /* [29.212-f10] */
public static final int AC_OFFLINE_CHARGING = 1278; /* [32-299-f10] */
public static final int AC_OFR_FLAGS = 3328; /* [29.338-f00] */
public static final int AC_OLD_REFERENCE_NUMBER = 3011; /* [29.368-e20] */
public static final int AC_OMC_ID = 1466; /* [29.272-f10] */
public static final int AC_ONE_TIME_NOTIFICATION = 712; /* [29.329-f00] */
public static final int AC_ONLINE = 1009; /* [29.212-f10] */
public static final int AC_ONLINE_CHARGING_FLAG = 2303; /* [32-299-f10] */
public static final int AC_OPERATOR_DETERMINED_BARRING = 1425; /* [29.272-f10] */
public static final int AC_OPERATOR_NAME = 126; /* [no_reference] */
public static final int AC_OPTIONAL_CAPABILITY = 605; /* [29.229-e20] */
public static final int AC_ORIGIN_AAA_PROTOCOL = 408; /* [RFC 7155] */
public static final int AC_ORIGIN_HOST = 264; /* [RFC 6733] */
public static final int AC_ORIGIN_REALM = 296; /* [RFC 6733] */
public static final int AC_ORIGIN_STATE_ID = 278; /* [RFC 6733] */
public static final int AC_ORIGINATING_IOI = 839; /* [32-299-f10] */
public static final int AC_ORIGINATING_LINE_INFO = 94; /* [RFC 7155] */
public static final int AC_ORIGINATING_REQUEST = 633; /* [29.229-e20] */
public static final int AC_ORIGINATING_SIP_URI = 3326; /* [29.338-f00] */
public static final int AC_ORIGINATION_TIME_STAMP = 1536; /* [29.273-f10] */
public static final int AC_ORIGINATOR = 864; /* [32-299-f10] */
public static final int AC_ORIGINATOR_ADDRESS = 886; /* [32-299-f10] */
public static final int AC_ORIGINATOR_INTERFACE = 2009; /* [32-299-f10] */
public static final int AC_ORIGINATOR_RECEIVED_ADDRESS = 2027; /* [32-299-f10] */
public static final int AC_ORIGINATOR_SCCP_ADDRESS = 2008; /* [32-299-f10] */
public static final int AC_OUTGOING_SESSION_ID = 2320; /* [32-299-f10] */
public static final int AC_OUTGOING_TRUNK_GROUP_ID = 853; /* [32-299-f10] */
public static final int AC_PACKET_FILTER_CONTENT = 1059; /* [29.212-f10] */
public static final int AC_PACKET_FILTER_IDENTIFIER = 1060; /* [29.212-f10] */
public static final int AC_PACKET_FILTER_INFORMATION = 1061; /* [29.212-f10] */
public static final int AC_PACKET_FILTER_OPERATION = 1062; /* [29.212-f10] */
public static final int AC_PACKET_FILTER_USAGE = 1072; /* [29.212-f10] */
public static final int AC_PARTICIPANT_ACCESS_PRIORITY = 1259; /* [32-299-f10] */
public static final int AC_PARTICIPANT_ACTION_TYPE = 2049; /* [32-299-f10] */
public static final int AC_PARTICIPANT_GROUP = 1260; /* [32-299-f10] */
public static final int AC_PARTICIPANTS_INVOLVED = 887; /* [32-299-f10] */
public static final int AC_PASSWORD_RETRY = 75; /* [RFC 7155] */
public static final int AC_PATH = 640; /* [29.229-e20] */
public static final int AC_PAYLOAD = 3004; /* [29.368-e20] */
public static final int AC_PCC_RULE_STATUS = 1019; /* [29.212-f10] */
public static final int AC_PCRF_ADDRESS = 2207; /* [29.215-f00] */
public static final int AC_PCSCF_RESTORATION_INDICATION = 2826; /* [29.212-f10] */
public static final int AC_PDG_ADDRESS = 895; /* [32-299-f10] */
public static final int AC_PDG_CHARGING_ID = 896; /* [32-299-f10] */
public static final int AC_PDN_CONNECTION_CHARGING_ID = 2050; /* [32-299-f10] */
public static final int AC_PDN_CONNECTION_CONTINUITY = 1690; /* [29.272-f10] */
public static final int AC_PDN_CONNECTION_ID = 1065; /* [29.212-f10] */
public static final int AC_PDN_GW_ALLOCATION_TYPE = 1438; /* [29.272-f10] */
public static final int AC_PDN_TYPE = 1456; /* [29.272-f10] */
public static final int AC_PDP_ADDRESS = 1227; /* [32-299-f10] */
public static final int AC_PDP_ADDRESS_PREFIX_LENGTH = 2606; /* [32-299-f10] */
public static final int AC_PDP_CONTEXT = 1469; /* [29.272-f10] */
public static final int AC_PDP_CONTEXT_TYPE = 1247; /* [32-299-f10] */
public static final int AC_PDP_TYPE = 1470; /* [29.272-f10] */
public static final int AC_PENDING_POLICY_COUNTER_CHANGE_TIME = 2906; /* [29.219-f10] */
public static final int AC_PENDING_POLICY_COUNTER_INFORMATION = 2905; /* [29.219-f10] */
public static final int AC_PERIODIC_COMMUNICATION_INDICATOR = 3115; /* [29.336-f10] */
public static final int AC_PERIODIC_LDR_INFORMATION = 2540; /* [29.172-d10] */
public static final int AC_PERIODIC_LOCATION_SUPPORT_INDICATOR = 2550; /* [29.172-d10] */
public static final int AC_PERIODIC_TIME = 3117; /* [29.336-f10] */
public static final int AC_PHYSICAL_ACCESS_ID = 313; /* [ETSI ES 283 034] */
public static final int AC_PLA_FLAGS = 2546; /* [29.172-d10] */
public static final int AC_PLMN_CLIENT = 1482; /* [29.272-f10] */
public static final int AC_PLMN_ID_LIST = 2544; /* [29.172-d10] */
public static final int AC_PLMN_ID_REQUESTED = 3172; /* [29.336-f10] */
public static final int AC_PLR_FLAGS = 2545; /* [29.172-d10] */
public static final int AC_POC_CHANGE_CONDITION = 1261; /* [32-299-f10] */
public static final int AC_POC_CHANGE_TIME = 1262; /* [32-299-f10] */
public static final int AC_POC_CONTROLLING_ADDRESS = 858; /* [32-299-f10] */
public static final int AC_POC_EVENT_TYPE = 2025; /* [32-299-f10] */
public static final int AC_POC_GROUP_NAME = 859; /* [32-299-f10] */
public static final int AC_POC_INFORMATION = 879; /* [32-299-f10] */
public static final int AC_POC_SERVER_ROLE = 883; /* [32-299-f10] */
public static final int AC_POC_SESSION_ID = 1229; /* [32-299-f10] */
public static final int AC_POC_SESSION_INITIATION_TYPE = 1277; /* [32-299-f10] */
public static final int AC_POC_SESSION_TYPE = 884; /* [32-299-f10] */
public static final int AC_POC_USER_ROLE = 1252; /* [32-299-f10] */
public static final int AC_POC_USER_ROLE_IDS = 1253; /* [32-299-f10] */
public static final int AC_POC_USER_ROLE_INFO_UNITS = 1254; /* [32-299-f10] */
public static final int AC_POLICY_COUNTER_IDENTIFIER = 2901; /* [29.219-f10] */
public static final int AC_POLICY_COUNTER_STATUS = 2902; /* [29.219-f10] */
public static final int AC_POLICY_COUNTER_STATUS_REPORT = 2903; /* [29.219-f10] */
public static final int AC_PORT = 530; /* [RFC 5777] */
public static final int AC_PORT_END = 533; /* [RFC 5777] */
public static final int AC_PORT_LIMIT = 62; /* [RFC 7155] */
public static final int AC_PORT_RANGE = 531; /* [RFC 5777] */
public static final int AC_PORT_START = 532; /* [RFC 5777] */
public static final int AC_POSITIONING_DATA = 1245; /* [32-299-f10] */
public static final int AC_POSITIONING_METHOD = 1659; /* [29.272-f10] */
public static final int AC_PPR_ADDRESS = 2407; /* [29.173-e00] */
public static final int AC_PPR_FLAGS = 1508; /* [29.273-f10] */
public static final int AC_PRA_INSTALL = 2845; /* [29.212-f10] */
public static final int AC_PRA_REMOVE = 2846; /* [29.212-f10] */
public static final int AC_PRE_EMPTION_CAPABILITY = 1047; /* [29.212-f10] */
public static final int AC_PRE_EMPTION_CONTROL_INFO = 553; /* [29.214-f10] */
public static final int AC_PRE_EMPTION_VULNERABILITY = 1048; /* [29.212-f10] */
public static final int AC_PRE_PAGING_SUPPORTED = 717; /* [29.329-f00] */
public static final int AC_PRECEDENCE = 1010; /* [29.212-f10] */
public static final int AC_PREFERRED_AOC_CURRENCY = 2315; /* [32-299-f10] */
public static final int AC_PREFERRED_DATA_MODE = 1686; /* [29.272-f10] */
public static final int AC_PRESENCE_REPORTING_AREA_ELEMENTS_LIST = 2820; /* [29.212-f10] */
public static final int AC_PRESENCE_REPORTING_AREA_IDENTIFIER = 2821; /* [29.212-f10] */
public static final int AC_PRESENCE_REPORTING_AREA_INFORMATION = 2822; /* [29.212-f10] */
public static final int AC_PRESENCE_REPORTING_AREA_STATUS = 2823; /* [29.212-f10] */
public static final int AC_PRIMARY_CHARGING_COLLECTION_FUNCTION_NAME = 621; /* [29.229-e20] */
public static final int AC_PRIMARY_EVENT_CHARGING_FUNCTION_NAME = 619; /* [29.229-e20] */
public static final int AC_PRIMARY_OCS_CHARGING_FUNCTION_NAME = 316; /* [29.234-b20] */
public static final int AC_PRIORITIZED_LIST_INDICATOR = 2551; /* [29.172-d10] */
public static final int AC_PRIORITY = 1209; /* [32-299-f10] */
public static final int AC_PRIORITY_INDICATION = 3006; /* [29.368-e20] */
public static final int AC_PRIORITY_LEVEL = 1046; /* [29.212-f10] */
public static final int AC_PRIORITY_SHARING_INDICATOR = 550; /* [29.214-f10] */
public static final int AC_PRIVILEDGED_SENDER_INDICATION = 652; /* [29.229-e20] */
public static final int AC_PRODUCT_NAME = 269; /* [RFC 6733] */
public static final int AC_PROMPT = 76; /* [RFC 7155] */
public static final int AC_PROSE_PERMISSION = 3702; /* [29.344] */
public static final int AC_PROSE_SUBSCRIPTION_DATA_S6 = 3701; /* [29.272-f10] */
public static final int AC_PROTOCOL = 513; /* [RFC 5777] */
public static final int AC_PROXY_HOST = 280; /* [RFC 6733] */
public static final int AC_PROXY_INFO = 284; /* [RFC 6733] */
public static final int AC_PROXY_STATE = 33; /* [RFC 6733] */
public static final int AC_PS_APPEND_FREE_FORMAT_DATA = 867; /* [32-299-f10] */
public static final int AC_PS_FREE_FORMAT_DATA = 866; /* [32-299-f10] */
public static final int AC_PS_FURNISH_CHARGING_INFORMATION = 865; /* [32-299-f10] */
public static final int AC_PS_INFORMATION = 874; /* [32-299-f10] */
public static final int AC_PS_TO_CS_SESSION_CONTINUITY = 1099; /* [29.212-f10] */
public static final int AC_PSEUDONYM_INDICATOR = 2519; /* [29.172-d10] */
public static final int AC_PUA_FLAGS = 1442; /* [29.272-f10] */
public static final int AC_PUBLIC_IDENTITY = 601; /* [29.229-e20] */
public static final int AC_PUR_FLAGS = 1635; /* [29.272-a60] */
public static final int AC_QOS_CAPABILITY = 578; /* [RFC 5777] */
public static final int AC_QOS_CLASS_IDENTIFIER = 1028; /* [29.212-f10] */
public static final int AC_QOS_FILTER_RULE = 407; /* [RFC 7155] */
public static final int AC_QOS_INFORMATION = 1016; /* [29.212-f10] */
public static final int AC_QOS_NEGOTIATION = 1029; /* [29.212-f10] */
public static final int AC_QOS_PARAMETERS = 576; /* [RFC 5777] */
public static final int AC_QOS_PROFILE_ID = 573; /* [RFC 5777] */
public static final int AC_QOS_PROFILE_TEMPLATE = 574; /* [RFC 5777] */
public static final int AC_QOS_RESOURCES = 508; /* [RFC 5777] */
public static final int AC_QOS_RULE_BASE_NAME = 1074; /* [29.212-f10] */
public static final int AC_QOS_RULE_DEFINITION = 1053; /* [29.212-f10] */
public static final int AC_QOS_RULE_INSTALL = 1051; /* [29.212-f10] */
public static final int AC_QOS_RULE_NAME = 1054; /* [29.212-f10] */
public static final int AC_QOS_RULE_REMOVE = 1052; /* [29.212-f10] */
public static final int AC_QOS_RULE_REPORT = 1055; /* [29.212-f10] */
public static final int AC_QOS_SEMANTICS = 575; /* [RFC 5777] */
public static final int AC_QOS_SUBSCRIBED = 1404; /* [29.272-f10] */
public static final int AC_QOS_UPGRADE = 1030; /* [29.212-f10] */
public static final int AC_QUOTA_CONSUMPTION_TIME = 881; /* [32-299-f10] */
public static final int AC_QUOTA_HOLDING_TIME = 871; /* [32-299-f10] */
public static final int AC_RAI = 909; /* [29.061-f10] */
public static final int AC_RAN_NAS_RELEASE_CAUSE = 2819; /* [29.212-f10] */
public static final int AC_RAN_RULE_SUPPORT = 2832; /* [29.212-f10] */
public static final int AC_RANAP_CAUSE = 4303; /* [29.128-f10] */
public static final int AC_RAND = 1447; /* [29.272-f10] */
public static final int AC_RAR_FLAGS = 1522; /* [29.273-f10] */
public static final int AC_RAT_FREQUENCY_SELECTION_PRIORITY_ID = 1440; /* [29.272-f10] */
public static final int AC_RAT_TYPE = 1032; /* [29.212-f10] */
public static final int AC_RAT_TYPE_850 = 1032; /* [29.212-850] */
public static final int AC_RAT_TYPE_S6 = 1032; /* [29.272-f20] */
public static final int AC_RATE_CONTROL_MAX_MESSAGE_SIZE = 3937; /* [32-299-f10] */
public static final int AC_RATE_CONTROL_MAX_RATE = 3938; /* [32-299-f10] */
public static final int AC_RATE_CONTROL_TIME_UNIT = 3939; /* [32-299-f10] */
public static final int AC_RATE_ELEMENT = 2058; /* [32-299-f10] */
public static final int AC_RATING_GROUP = 432; /* [RFC 4006] */
public static final int AC_RCAF_ID = 4010; /* [29.217-e20] */
public static final int AC_RDR_FLAGS = 3323; /* [29.338-f00] */
public static final int AC_RDS_INDICATOR = 1697; /* [29.272-f10] */
public static final int AC_RE_AUTH_REQUEST_TYPE = 285; /* [RFC 6733] */
public static final int AC_RE_SYNCHRONIZATION_INFO = 1411; /* [29.272-f10] */
public static final int AC_REACHABILITY_INFORMATION = 3140; /* [29.336-f10] */
public static final int AC_REACHABILITY_INFORMATION_S6 = 3140; /* [29.272-f10] */
public static final int AC_REACHABILITY_TYPE = 3132; /* [29.336-f10] */
public static final int AC_REACHABILITY_TYPE_S6 = 3132; /* [29.272-f10] */
public static final int AC_READ_REPLY_REPORT_REQUESTED = 1222; /* [32-299-f10] */
public static final int AC_REAL_TIME_TARIFF_INFORMATION = 2305; /* [32-299-f10] */
public static final int AC_REASON_CODE = 616; /* [29.229-e20] */
public static final int AC_REASON_INFO = 617; /* [29.229-e20] */
public static final int AC_RECEIVED_TALK_BURST_TIME = 1284; /* [32-299-f10] */
public static final int AC_RECEIVED_TALK_BURST_VOLUME = 1285; /* [32-299-f10] */
public static final int AC_RECIPIENT_ADDRESS = 1201; /* [32-299-f10] */
public static final int AC_RECIPIENT_INFO = 2026; /* [32-299-f10] */
public static final int AC_RECIPIENT_RECEIVED_ADDRESS = 2028; /* [32-299-f10] */
public static final int AC_RECIPIENT_SCCP_ADDRESS = 2010; /* [32-299-f10] */
public static final int AC_RECORD_ROUTE = 646; /* [29.229-e20] */
public static final int AC_REDIRECT_ADDRESS_TYPE = 433; /* [RFC 4006] */
public static final int AC_REDIRECT_HOST = 292; /* [RFC 6733] */
public static final int AC_REDIRECT_HOST_USAGE = 261; /* [RFC 6733] */
public static final int AC_REDIRECT_INFORMATION = 1085; /* [29.212-f10] */
public static final int AC_REDIRECT_MAX_CACHE_TIME = 262; /* [RFC 6733] */
public static final int AC_REDIRECT_SERVER = 434; /* [RFC 4006] */
public static final int AC_REDIRECT_SERVER_ADDRESS = 435; /* [RFC 4006] */
public static final int AC_REDIRECT_SUPPORT = 1086; /* [29.212-f10] */
public static final int AC_REFERENCE_ID = 4202; /* [29.154-f00] */
public static final int AC_REFERENCE_ID_VALIDITY_TIME = 3148; /* [29.336-f10] */
public static final int AC_REFERENCE_ID_VALIDITY_TIME_S6 = 3148; /* [29.272-f10] */
public static final int AC_REFERENCE_NUMBER = 3007; /* [29.368-e20] */
public static final int AC_REFUND_INFORMATION = 2022; /* [32-299-f10] */
public static final int AC_REGIONAL_SUBSCRIPTION_ZONE_CODE = 1446; /* [29.272-f10] */
public static final int AC_RELAY_NODE_INDICATOR = 1633; /* [29.272-a60] */
public static final int AC_REMAINING_BALANCE = 2021; /* [32-299-f10] */
public static final int AC_REMOVAL_OF_ACCESS = 2842; /* [29.212-f10] */
public static final int AC_REPLY_APPLIC_ID = 1223; /* [32-299-f10] */
public static final int AC_REPLY_MESSAGE = 18; /* [RFC 7155] */
public static final int AC_REPLY_PATH_REQUESTED = 2011; /* [32-299-f10] */
public static final int AC_REPORT_AMOUNT = 1628; /* [29.272-a60] */
public static final int AC_REPORT_INTERVAL = 1627; /* [29.272-a60] */
public static final int AC_REPORTING_AMOUNT = 2541; /* [29.172-d10] */
public static final int AC_REPORTING_INTERVAL = 2542; /* [29.172-d10] */
public static final int AC_REPORTING_LEVEL = 1011; /* [29.212-f10] */
public static final int AC_REPORTING_PLMN_LIST = 2543; /* [29.172-d10] */
public static final int AC_REPORTING_REASON = 872; /* [32.299-e50] */
public static final int AC_REPORTING_RESTRICTION = 4011; /* [29.217-e20] */
public static final int AC_REPORTING_TRIGGER = 1626; /* [29.272-f10] */
public static final int AC_REPOSITORY_DATA_ID = 715; /* [29.329-f00] */
public static final int AC_REQUEST_STATUS = 3008; /* [29.368-e20] */
public static final int AC_REQUEST_TYPE = 2838; /* [29.212-f10] */
public static final int AC_REQUESTED_ACTION = 436; /* [RFC 4006] */
public static final int AC_REQUESTED_DOMAIN = 706; /* [29.329-f00] */
public static final int AC_REQUESTED_EUTRAN_AUTHENTICATION_INFO = 1408; /* [29.272-f10] */
public static final int AC_REQUESTED_NODES = 713; /* [29.329-f00] */
public static final int AC_REQUESTED_PARTY_ADDRESS = 1251; /* [32-299-f10] */
public static final int AC_REQUESTED_RETRANSMISSION_TIME = 3331; /* [29.338-f00] */
public static final int AC_REQUESTED_SERVICE_UNIT = 437; /* [RFC 4006] */
public static final int AC_REQUESTED_UTRAN_GERAN_AUTHENTICATION_INFO = 1409; /* [29.272-f10] */
public static final int AC_REQUESTED_VALIDITY_TIME = 3159; /* [29.336-f10] */
public static final int AC_REQUIRED_ACCESS_INFO = 536; /* [29.214-f10] */
public static final int AC_REQUIRED_MBMS_BEARER_CAPABILITIES = 901; /* [29.061-f10] */
public static final int AC_RESERVATION_PRIORITY = 458; /* [ETSI-TS-183-017] */
public static final int AC_RESET_ID = 1670; /* [29.272-f10] */
public static final int AC_RESOURCE_ALLOCATION_NOTIFICATION = 1063; /* [29.212-f10] */
public static final int AC_RESOURCE_RELEASE_NOTIFICATION = 2841; /* [29.212-f10] */
public static final int AC_RESPONSE_TIME = 2509; /* [29.172-d10] */
public static final int AC_RESTORATION_INFO = 649; /* [29.229-e20] */
public static final int AC_RESTORATION_PRIORITY = 1663; /* [29.272-f10] */
public static final int AC_RESTRICTED_PLMN_LIST = 3157; /* [29.336-f10] */
public static final int AC_RESTRICTION_FILTER_RULE = 438; /* [RFC 4006] */
public static final int AC_RESULT_CODE = 268; /* [RFC 6733] */
public static final int AC_RETRY_INTERVAL = 541; /* [29.214-f10] */
public static final int AC_REVALIDATION_TIME = 1042; /* [29.212-f10] */
public static final int AC_RIA_FLAGS = 2411; /* [29.173-e00] */
public static final int AC_RIR_FLAGS = 3167; /* [29.336-f10] */
public static final int AC_ROAMING_INFORMATION = 3139; /* [29.336-f10] */
public static final int AC_ROAMING_RESTRICTED_DUE_TO_UNSUPPORTED_FEATURE = 1457; /* [29.272-f10] */
public static final int AC_ROLE_OF_NODE = 829; /* [32-299-f10] */
public static final int AC_ROUTE_RECORD = 282; /* [RFC 6733] */
public static final int AC_ROUTING_AREA_IDENTITY = 1605; /* [29.272-f10] */
public static final int AC_ROUTING_FILTER = 1078; /* [29.212-f10] */
public static final int AC_ROUTING_IP_ADDRESS = 1079; /* [29.212-f10] */
public static final int AC_ROUTING_POLICY = 312; /* [29.234-b20] */
public static final int AC_ROUTING_RULE_DEFINITION = 1076; /* [29.212-f10] */
public static final int AC_ROUTING_RULE_FAILURE_CODE = 2834; /* [29.212-f10] */
public static final int AC_ROUTING_RULE_IDENTIFIER = 1077; /* [29.212-f10] */
public static final int AC_ROUTING_RULE_INSTALL = 1081; /* [29.212-f10] */
public static final int AC_ROUTING_RULE_REMOVE = 1075; /* [29.212-f10] */
public static final int AC_ROUTING_RULE_REPORT = 2835; /* [29.212-f10] */
public static final int AC_RR_BANDWIDTH = 521; /* [29.214-f10] */
public static final int AC_RRC_CAUSE_COUNTER = 4318; /* [29.128-f10] */
public static final int AC_RRC_COUNTER_TIMESTAMP = 4320; /* [29.128-f10] */
public static final int AC_RS_BANDWIDTH = 522; /* [29.214-f10] */
public static final int AC_RUCI_ACTION = 4012; /* [29.217-e20] */
public static final int AC_RULE_ACTIVATION_TIME = 1043; /* [29.212-f10] */
public static final int AC_RULE_DEACTIVATION_TIME = 1044; /* [29.212-f10] */
public static final int AC_RULE_FAILURE_CODE = 1031; /* [29.212-f10] */
public static final int AC_RX_REQUEST_TYPE = 533; /* [29.214-f10] */
public static final int AC_S_VID_END = 554; /* [RFC 5777] */
public static final int AC_S_VID_START = 553; /* [RFC 5777] */
public static final int AC_S1AP_CAUSE = 4302; /* [29.128-f10] */
public static final int AC_S6T_HSS_CAUSE = 3154; /* [29.336-f10] */
public static final int AC_SAR_FLAGS = 655; /* [29.229-e20] */
public static final int AC_SC_ADDRESS = 3300; /* [29.338-f00] */
public static final int AC_SCALE_FACTOR = 2059; /* [32-299-f10] */
public static final int AC_SCEF_ID = 3125; /* [29.336-f10] */
public static final int AC_SCEF_ID_S6 = 3125; /* [29.272-f10] */
public static final int AC_SCEF_REALM = 1684; /* [29.272-f10] */
public static final int AC_SCEF_REFERENCE_ID = 3124; /* [29.336-f10] */
public static final int AC_SCEF_REFERENCE_ID_FOR_DELETION = 3126; /* [29.336-f10] */
public static final int AC_SCEF_REFERENCE_ID_FOR_DELETION_S6 = 3126; /* [29.272-f10] */
public static final int AC_SCEF_REFERENCE_ID_S6 = 3124; /* [29.272-f10] */
public static final int AC_SCEF_WAIT_TIME = 4316; /* [29.128-f10] */
public static final int AC_SCHEDULED_COMMUNICATION_TIME = 3118; /* [29.336-f10] */
public static final int AC_SCS_ADDRESS = 3941; /* [32-299-f10] */
public static final int AC_SCS_AS_ADDRESS = 3940; /* [32-299-f10] */
public static final int AC_SCS_IDENTITY = 3104; /* [29.336-f10] */
public static final int AC_SCS_REALM = 3942; /* [32-299-f10] */
public static final int AC_SCSCF_RESTORATION_INFO = 639; /* [29.229-e20] */
public static final int AC_SDP_ANSWER_TIMESTAMP = 1275; /* [32-299-f10] */
public static final int AC_SDP_MEDIA_COMPONENT = 843; /* [32-299-f10] */
public static final int AC_SDP_MEDIA_DESCRIPTION = 845; /* [32-299-f10] */
public static final int AC_SDP_MEDIA_NAME = 844; /* [32-299-f10] */
public static final int AC_SDP_OFFER_TIMESTAMP = 1274; /* [32-299-f10] */
public static final int AC_SDP_SESSION_DESCRIPTION = 842; /* [32-299-f10] */
public static final int AC_SDP_TIMESTAMPS = 1273; /* [32-299-f10] */
public static final int AC_SDP_TYPE = 2036; /* [32-299-f10] */
public static final int AC_SECONDARY_CHARGING_COLLECTION_FUNCTION_NAME = 622; /* [29.229-e20] */
public static final int AC_SECONDARY_EVENT_CHARGING_FUNCTION_NAME = 620; /* [29.229-e20] */
public static final int AC_SECONDARY_OCS_CHARGING_FUNCTION_NAME = 317; /* [29.234-b20] */
public static final int AC_SECURITY_PARAMETER_INDEX = 1056; /* [29.212-f10] */
public static final int AC_SEND_DATA_INDICATION = 710; /* [29.329-f00] */
public static final int AC_SEQUENCE_NUMBER = 716; /* [29.329-f00] */
public static final int AC_SERVED_PARTY_IP_ADDRESS = 848; /* [32-299-f10] */
public static final int AC_SERVER_ASSIGNMENT_TYPE = 614; /* [29.229-e20] */
public static final int AC_SERVER_CAPABILITIES = 603; /* [29.229-e20] */
public static final int AC_SERVER_NAME = 602; /* [29.229-e20] */
public static final int AC_SERVICE_AREA_IDENTITY = 1607; /* [29.272-f10] */
public static final int AC_SERVICE_AUTHORIZATION_INFO = 548; /* [29.214-f10] */
public static final int AC_SERVICE_CONTEXT_ID = 461; /* [RFC 4006] */
public static final int AC_SERVICE_DATA = 3107; /* [29.336-f10] */
public static final int AC_SERVICE_DATA_CONTAINER = 2040; /* [32-299-f10] */
public static final int AC_SERVICE_GENERIC_INFORMATION = 1256; /* [OMADDSChargingData] */
public static final int AC_SERVICE_ID = 855; /* [32-299-f10] */
public static final int AC_SERVICE_ID_S6M = 3103; /* [29.336-f10] */
public static final int AC_SERVICE_IDENTIFIER = 439; /* [RFC 4006] */
public static final int AC_SERVICE_INDICATION = 704; /* [29.329-f00] */
public static final int AC_SERVICE_INFO_STATUS = 527; /* [29.214-f10] */
public static final int AC_SERVICE_INFORMATION = 873; /* [32-299-f10] */
public static final int AC_SERVICE_MODE = 2032; /* [32-299-f10] */
public static final int AC_SERVICE_PARAMETER_INFO = 440; /* [RFC 4006] */
public static final int AC_SERVICE_PARAMETER_TYPE = 441; /* [RFC 4006] */
public static final int AC_SERVICE_PARAMETER_VALUE = 442; /* [RFC 4006] */
public static final int AC_SERVICE_PARAMETERS = 3105; /* [29.336-f10] */
public static final int AC_SERVICE_REPORT = 3152; /* [29.336-f10] */
public static final int AC_SERVICE_RESULT = 3146; /* [29.336-f10] */
public static final int AC_SERVICE_RESULT_CODE = 3147; /* [29.336-f10] */
public static final int AC_SERVICE_SELECTION = 493; /* [RFC 5778] */
public static final int AC_SERVICE_SPECIFIC_DATA = 863; /* [32-299-f10] */
public static final int AC_SERVICE_SPECIFIC_INFO = 1249; /* [32-299-f10] */
public static final int AC_SERVICE_SPECIFIC_TYPE = 1257; /* [32-299-f10] */
public static final int AC_SERVICE_TYPE = 6; /* [RFC 7155] */
public static final int AC_SERVICE_TYPE_32_299 = 2031; /* [32-299-f10] */
public static final int AC_SERVICE_TYPE_S6 = 1483; /* [29.272-f10] */
public static final int AC_SERVICE_URN = 525; /* [29.214-f10] */
public static final int AC_SERVICETYPEIDENTITY = 1484; /* [29.272-f10] */
public static final int AC_SERVING_NODE = 2401; /* [29.173-e00] */
public static final int AC_SERVING_NODE_INDICATION = 714; /* [29.329-f00] */
public static final int AC_SERVING_NODE_T4 = 2401; /* [29.336-f10] */
public static final int AC_SERVING_NODE_TYPE = 2047; /* [32-299-f10] */
public static final int AC_SERVING_PLMN_RATE_CONTROL = 4310; /* [29.128-f10] */
public static final int AC_SESSION_BINDING = 270; /* [RFC 6733] */
public static final int AC_SESSION_ID = 263; /* [RFC 6733] */
public static final int AC_SESSION_LINKING_INDICATOR = 1064; /* [29.212-f10] */
public static final int AC_SESSION_PRIORITY = 650; /* [29.229-e20] */
public static final int AC_SESSION_RELEASE_CAUSE = 1045; /* [29.212-f10] */
public static final int AC_SESSION_REQUEST_TYPE = 311; /* [29.234-b20] */
public static final int AC_SESSION_SERVER_FAILOVER = 271; /* [RFC 6733] */
public static final int AC_SESSION_TIMEOUT = 27; /* [RFC 6733] */
public static final int AC_SGI_PTP_TUNNELLING_METHOD = 3931; /* [32-299-f10] */
public static final int AC_SGS_MME_IDENTITY = 1664; /* [29.272-f10] */
public static final int AC_SGSN_ABSENT_USER_DIAGNOSTIC_SM = 3315; /* [29.338-f00] */
public static final int AC_SGSN_ADDRESS = 1228; /* [32-299-f10] */
public static final int AC_SGSN_LOCATION_INFORMATION = 1601; /* [29.272-f10] */
public static final int AC_SGSN_LOCATION_INFORMATION_S6T = 1601; /* [29.336-f10] */
public static final int AC_SGSN_NAME = 2409; /* [29.173-c30] */
public static final int AC_SGSN_NUMBER = 1489; /* [29.272-f10] */
public static final int AC_SGSN_REALM = 2410; /* [29.173-c30] */
public static final int AC_SGSN_SM_DELIVERY_OUTCOME = 3319; /* [29.338-f00] */
public static final int AC_SGSN_USER_STATE = 1498; /* [29.272-f10] */
public static final int AC_SGW_ADDRESS = 2067; /* [32-299-f10] */
public static final int AC_SGW_CHANGE = 2065; /* [32-299-f10] */
public static final int AC_SHARING_KEY_DL = 539; /* [29.214-f10] */
public static final int AC_SHARING_KEY_UL = 540; /* [29.214-f10] */
public static final int AC_SHORT_NETWORK_NAME = 1517; /* [29.273-f10] */
public static final int AC_SIP_AUTH_DATA_ITEM = 612; /* [29.229-e20] */
public static final int AC_SIP_AUTH_DATA_ITEM_WX = 612; /* [29.234-b20] */
public static final int AC_SIP_AUTHENTICATE = 609; /* [29.229-e20] */
public static final int AC_SIP_AUTHENTICATION_CONTEXT = 611; /* [29.229-e20] */
public static final int AC_SIP_AUTHENTICATION_SCHEME = 608; /* [29.229-e20] */
public static final int AC_SIP_AUTHORIZATION = 610; /* [29.229-e20] */
public static final int AC_SIP_DIGEST_AUTHENTICATE = 635; /* [29.229-e20] */
public static final int AC_SIP_FORKING_INDICATION = 523; /* [29.214-f10] */
public static final int AC_SIP_ITEM_NUMBER = 613; /* [29.229-e20] */
public static final int AC_SIP_METHOD = 824; /* [32-299-f10] */
public static final int AC_SIP_NUMBER_AUTH_ITEMS = 607; /* [29.229-e20] */
public static final int AC_SIP_REQUEST_TIMESTAMP = 834; /* [32-299-f10] */
public static final int AC_SIP_REQUEST_TIMESTAMP_FRACTION = 2301; /* [32-299-f10] */
public static final int AC_SIP_RESPONSE_TIMESTAMP = 835; /* [32-299-f10] */
public static final int AC_SIP_RESPONSE_TIMESTAMP_FRACTION = 2302; /* [32-299-f10] */
public static final int AC_SIPTO_LOCAL_NETWORK_PERMISSION = 1665; /* [29.272-f10] */
public static final int AC_SIPTO_PERMISSION = 1613; /* [29.272-f10] */
public static final int AC_SIR_FLAGS = 3110; /* [29.336-f10] */
public static final int AC_SL_REQUEST_TYPE = 2904; /* [29.219-f10] */
public static final int AC_SLG_LOCATION_TYPE = 2500; /* [29.172-d10] */
public static final int AC_SM_BACK_OFF_TIMER = 1534; /* [29.273-f10] */
public static final int AC_SM_CAUSE = 4305; /* [29.128-f10] */
public static final int AC_SM_DELIVERY_CAUSE = 3321; /* [29.338-f00] */
public static final int AC_SM_DELIVERY_FAILURE_CAUSE = 3303; /* [29.338-f00] */
public static final int AC_SM_DELIVERY_NOT_INTENDED = 3311; /* [29.338-f00] */
public static final int AC_SM_DELIVERY_OUTCOME = 3316; /* [29.338-f00] */
public static final int AC_SM_DELIVERY_OUTCOME_T4 = 3200; /* [29.337-e10] */
public static final int AC_SM_DELIVERY_START_TIME = 3307; /* [29.338-f00] */
public static final int AC_SM_DELIVERY_TIMER = 3306; /* [29.338-f00] */
public static final int AC_SM_DIAGNOSTIC_INFO = 3305; /* [29.338-f00] */
public static final int AC_SM_DISCHARGE_TIME = 2012; /* [32-299-f10] */
public static final int AC_SM_ENUMERATED_DELIVERY_FAILURE_CAUSE = 3304; /* [29.338-f00] */
public static final int AC_SM_MESSAGE_TYPE = 2007; /* [32-299-f10] */
public static final int AC_SM_PROTOCOL_ID = 2013; /* [32-299-f10] */
public static final int AC_SM_RP_MTI = 3308; /* [29.338-f00] */
public static final int AC_SM_RP_SMEA = 3309; /* [29.338-f00] */
public static final int AC_SM_RP_UI = 3301; /* [29.338-f00] */
public static final int AC_SM_SERVICE_TYPE = 2029; /* [32-299-f10] */
public static final int AC_SM_STATUS = 2014; /* [32-299-f10] */
public static final int AC_SM_USER_DATA_HEADER = 2015; /* [32-299-f10] */
public static final int AC_SMS_APPLICATION_PORT_ID = 3010; /* [29.368-e20] */
public static final int AC_SMS_GMSC_ADDRESS = 3332; /* [29.338-f00] */
public static final int AC_SMS_GMSC_ALERT_EVENT = 3333; /* [29.338-f00] */
public static final int AC_SMS_INFORMATION = 2000; /* [32-299-f10] */
public static final int AC_SMS_NODE = 2016; /* [32-299-f10] */
public static final int AC_SMS_REGISTER_REQUEST = 1648; /* [29.272-f10] */
public static final int AC_SMSC_ADDRESS = 2017; /* [32-299-f10] */
public static final int AC_SMSMI_CORRELATION_ID = 3324; /* [29.338-f00] */
public static final int AC_SN_REQUEST_TYPE = 2907; /* [29.219-f10] */
public static final int AC_SOFTWARE_VERSION = 1403; /* [29.272-f10] */
public static final int AC_SPECIFIC_ACTION = 513; /* [29.214-f10] */
public static final int AC_SPECIFIC_APN_INFO = 1472; /* [29.272-f10] */
public static final int AC_SPONSOR_IDENTITY = 531; /* [29.214-f10] */
public static final int AC_SPONSORED_CONNECTIVITY_DATA = 530; /* [29.214-f10] */
public static final int AC_SPONSORING_ACTION = 542; /* [29.214-f10] */
public static final int AC_SRES = 1454; /* [29.272-f10] */
public static final int AC_SRR_FLAGS = 3310; /* [29.338-f00] */
public static final int AC_SS_CODE = 1476; /* [29.272-f10] */
public static final int AC_SS_STATUS = 1477; /* [29.272-f10] */
public static final int AC_SSID = 1524; /* [29.273-f10] */
public static final int AC_START_TIME = 2041; /* [32-299-f10] */
public static final int AC_STATE = 24; /* [RFC 7155] */
public static final int AC_STATIONARY_INDICATION = 3119; /* [29.336-f10] */
public static final int AC_STN_SR = 1433; /* [29.272-f10] */
public static final int AC_STOP_TIME = 2042; /* [32-299-f10] */
public static final int AC_SUBMISSION_TIME = 1202; /* [32-299-f10] */
public static final int AC_SUBS_REQ_TYPE = 705; /* [29.329-f00] */
public static final int AC_SUBSCRIBED_PERIODIC_RAU_TAU_TIMER = 1619; /* [29.272-f10] */
public static final int AC_SUBSCRIBED_VSRVCC = 1636; /* [29.272-f10] */
public static final int AC_SUBSCRIBER_ROLE = 2033; /* [32-299-f10] */
public static final int AC_SUBSCRIBER_STATUS = 1424; /* [29.272-f10] */
public static final int AC_SUBSCRIPTION_DATA = 1400; /* [29.272-f10] */
public static final int AC_SUBSCRIPTION_DATA_DELETION = 1685; /* [29.272-f10] */
public static final int AC_SUBSCRIPTION_DATA_FLAGS = 16454; /* [29.272-f10] */
public static final int AC_SUBSCRIPTION_ID = 443; /* [RFC 4006] */
public static final int AC_SUBSCRIPTION_ID_DATA = 444; /* [RFC 4006] */
public static final int AC_SUBSCRIPTION_ID_TYPE = 450; /* [RFC 4006] */
public static final int AC_SUBSCRIPTION_INFO = 642; /* [29.229-e20] */
public static final int AC_SUBSESSION_DECISION_INFO = 2200; /* [29.215-f00] */
public static final int AC_SUBSESSION_ENFORCEMENT_INFO = 2201; /* [29.215-f00] */
public static final int AC_SUBSESSION_ID = 2202; /* [29.215-f00] */
public static final int AC_SUBSESSION_OPERATION = 2203; /* [29.215-f00] */
public static final int AC_SUGGESTED_NETWORK_CONFIGURATION = 3170; /* [29.336-f10] */
public static final int AC_SUPPLEMENTARY_SERVICE = 2048; /* [32-299-f10] */
public static final int AC_SUPPORTED_APPLICATIONS = 631; /* [29.229-e20] */
public static final int AC_SUPPORTED_FEATURES = 628; /* [29.229-e20] */
public static final int AC_SUPPORTED_FEATURES_S6A = 628; /* [29.272-f10] */
public static final int AC_SUPPORTED_MONITORING_EVENTS = 3144; /* [29.336-f10] */
public static final int AC_SUPPORTED_MONITORING_EVENTS_S6 = 3144; /* [29.272-f10] */
public static final int AC_SUPPORTED_SERVICES = 3143; /* [29.336-f10] */
public static final int AC_SUPPORTED_SERVICES_S6 = 3143; /* [29.272-f10] */
public static final int AC_SUPPORTED_VENDOR_ID = 265; /* [RFC 6733] */
public static final int AC_T4_DATA = 3108; /* [29.336-f10] */
public static final int AC_T4_PARAMETERS = 3106; /* [29.336-f10] */
public static final int AC_TALK_BURST_EXCHANGE = 1255; /* [32-299-f10] */
public static final int AC_TALK_BURST_TIME = 1286; /* [32-299-f10] */
public static final int AC_TALK_BURST_VOLUME = 1287; /* [32-299-f10] */
public static final int AC_TARIFF_CHANGE_USAGE = 452; /* [RFC 4006] */
public static final int AC_TARIFF_INFORMATION = 2060; /* [32-299-f10] */
public static final int AC_TARIFF_TIME_CHANGE = 451; /* [RFC 4006] */
public static final int AC_TARIFF_XML = 2306; /* [32-299-f10] */
public static final int AC_TCP_FLAG_TYPE = 544; /* [RFC 5777] */
public static final int AC_TCP_FLAGS = 543; /* [RFC 5777] */
public static final int AC_TCP_OPTION = 540; /* [RFC 5777] */
public static final int AC_TCP_OPTION_TYPE = 541; /* [RFC 5777] */
public static final int AC_TCP_OPTION_VALUE = 542; /* [RFC 5777] */
public static final int AC_TCP_SOURCE_PORT = 2843; /* [29.212-f10] */
public static final int AC_TDA_FLAGS = 4321; /* [29.128-f00] */
public static final int AC_TDF_APPLICATION_IDENTIFIER = 1088; /* [29.212-f10] */
public static final int AC_TDF_APPLICATION_INSTANCE_IDENTIFIER = 2802; /* [29.212-f10] */
public static final int AC_TDF_DESTINATION_HOST = 1089; /* [29.212-f10] */
public static final int AC_TDF_DESTINATION_REALM = 1090; /* [29.212-f10] */
public static final int AC_TDF_INFORMATION = 1087; /* [29.212-f10] */
public static final int AC_TDF_IP_ADDRESS = 1091; /* [29.212-f10] */
public static final int AC_TELESERVICE_LIST = 1486; /* [29.272-f10] */
public static final int AC_TERMINAL_INFORMATION = 1401; /* [29.272-f10] */
public static final int AC_TERMINAL_INFORMATION_T6 = 1401; /* [29.128-f00] */
public static final int AC_TERMINATING_IOI = 840; /* [32-299-f10] */
public static final int AC_TERMINATION_CAUSE = 295; /* [RFC 6733] */
public static final int AC_TERMINATION_CAUSE_SLG = 2548; /* [29.172-d10] */
public static final int AC_TFR_FLAGS = 3302; /* [29.338-f00] */
public static final int AC_TFT_FILTER = 1012; /* [29.212-f10] */
public static final int AC_TFT_PACKET_FILTER_INFORMATION = 1013; /* [29.212-f10] */
public static final int AC_TIME_FIRST_USAGE = 2043; /* [32-299-f10] */
public static final int AC_TIME_LAST_USAGE = 2044; /* [32-299-f10] */
public static final int AC_TIME_OF_DAY_CONDITION = 560; /* [RFC 5777] */
public static final int AC_TIME_OF_DAY_END = 562; /* [RFC 5777] */
public static final int AC_TIME_OF_DAY_START = 561; /* [RFC 5777] */
public static final int AC_TIME_QUOTA_MECHANISM = 1270; /* [32-299-f10] */
public static final int AC_TIME_QUOTA_THRESHOLD = 868; /* [32-299-f10] */
public static final int AC_TIME_QUOTA_TYPE = 1271; /* [32-299-f10] */
public static final int AC_TIME_STAMPS = 833; /* [32-299-f10] */
public static final int AC_TIME_USAGE = 2045; /* [32-299-f10] */
public static final int AC_TIME_WINDOW = 4204; /* [29.154-f00] */
public static final int AC_TIME_ZONE = 1642; /* [29.272-f10] */
public static final int AC_TIMEZONE_FLAG = 570; /* [RFC 5777] */
public static final int AC_TIMEZONE_OFFSET = 571; /* [RFC 5777] */
public static final int AC_TMGI = 900; /* [29.061-f10] */
public static final int AC_TO_SIP_HEADER = 645; /* [29.229-e20] */
public static final int AC_TO_SPEC = 516; /* [RFC 5777] */
public static final int AC_TOKEN_TEXT = 1215; /* [32-299-f10] */
public static final int AC_TOS_TRAFFIC_CLASS = 1014; /* [29.212-f10] */
public static final int AC_TOTAL_NUMBER_OF_MESSAGES_EXPLODED = 2113; /* [OMADDSChargingData] */
public static final int AC_TOTAL_NUMBER_OF_MESSAGES_SENT = 2114; /* [OMADDSChargingData] */
public static final int AC_TRACE_COLLECTION_ENTITY = 1452; /* [29.272-f10] */
public static final int AC_TRACE_DATA = 1458; /* [29.272-f10] */
public static final int AC_TRACE_DEPTH = 1462; /* [32.422] */
public static final int AC_TRACE_EVENT_LIST = 1465; /* [32.422] */
public static final int AC_TRACE_INFO = 1505; /* [29.273-f10] */
public static final int AC_TRACE_INTERFACE_LIST = 1464; /* [32.422] */
public static final int AC_TRACE_NE_TYPE_LIST = 1463; /* [32.422] */
public static final int AC_TRACE_REFERENCE = 1459; /* [29.272-f10] */
public static final int AC_TRACKING_AREA_IDENTITY = 1603; /* [29.272-f10] */
public static final int AC_TRAFFIC_DATA_VOLUMES = 2046; /* [32-299-f10] */
public static final int AC_TRAFFIC_STEERING_POLICY_IDENTIFIER_DL = 2836; /* [29.212-f10] */
public static final int AC_TRAFFIC_STEERING_POLICY_IDENTIFIER_UL = 2837; /* [29.212-f10] */
public static final int AC_TRANSCODER_INSERTED_INDICATION = 2605; /* [32-299-f10] */
public static final int AC_TRANSFER_END_TIME = 4205; /* [29.154-f00] */
public static final int AC_TRANSFER_POLICY = 4207; /* [29.154-f00] */
public static final int AC_TRANSFER_POLICY_ID = 4208; /* [29.154-f00] */
public static final int AC_TRANSFER_REQUEST_TYPE = 4203; /* [29.154-f00] */
public static final int AC_TRANSFER_START_TIME = 4206; /* [29.154-f00] */
public static final int AC_TRANSPORT_ACCESS_TYPE = 1519; /* [29.273-f10] */
public static final int AC_TREATMENT_ACTION = 572; /* [RFC 5777] */
public static final int AC_TRIGGER = 1264; /* [32-299-f10] */
public static final int AC_TRIGGER_ACTION = 3202; /* [29.337-e10] */
public static final int AC_TRIGGER_DATA = 3003; /* [29.368-e20] */
public static final int AC_TRIGGER_TYPE = 870; /* [32-299-f10] */
public static final int AC_TRUNK_GROUP_ID = 851; /* [32-299-f10] */
public static final int AC_TS_CODE = 1487; /* [29.272-f10] */
public static final int AC_TUNNEL_ASSIGNMENT_ID = 82; /* [RFC 7155] */
public static final int AC_TUNNEL_CLIENT_AUTH_ID = 90; /* [RFC 7155] */
public static final int AC_TUNNEL_CLIENT_ENDPOINT = 66; /* [RFC 7155] */
public static final int AC_TUNNEL_HEADER_FILTER = 1036; /* [29.212-f10] */
public static final int AC_TUNNEL_HEADER_LENGTH = 1037; /* [29.212-f10] */
public static final int AC_TUNNEL_INFORMATION = 1038; /* [29.212-f10] */
public static final int AC_TUNNEL_MEDIUM_TYPE = 65; /* [RFC 7155] */
public static final int AC_TUNNEL_PASSWORD = 69; /* [RFC 7155] */
public static final int AC_TUNNEL_PREFERENCE = 83; /* [RFC 7155] */
public static final int AC_TUNNEL_PRIVATE_GROUP_ID = 81; /* [RFC 7155] */
public static final int AC_TUNNEL_SERVER_AUTH_ID = 91; /* [RFC 7155] */
public static final int AC_TUNNEL_SERVER_ENDPOINT = 67; /* [RFC 7155] */
public static final int AC_TUNNEL_TYPE = 64; /* [RFC 7155] */
public static final int AC_TUNNELING = 401; /* [RFC 7155] */
public static final int AC_TWAG_ADDRESS = 3903; /* [32-299-f10] */
public static final int AC_TWAG_CP_ADDRESS = 1531; /* [29.273-f10] */
public static final int AC_TWAG_UP_ADDRESS = 1532; /* [29.273-f10] */
public static final int AC_TWAN_ACCESS_INFO = 1510; /* [29.273-f10] */
public static final int AC_TWAN_CONNECTION_MODE = 1527; /* [29.273-f10] */
public static final int AC_TWAN_CONNECTIVITY_PARAMETERS = 1528; /* [29.273-f10] */
public static final int AC_TWAN_DEFAULT_APN_CONTEXT_ID = 1512; /* [29.273-f10] */
public static final int AC_TWAN_IDENTIFIER = 29; /* [29.061-f10] */
public static final int AC_TWAN_PCO = 1530; /* [29.273-f10] */
public static final int AC_TWAN_S2A_FAILURE_CAUSE = 1533; /* [29.273-f10] */
public static final int AC_TWAN_USER_LOCATION_INFO = 2714; /* [32-299-f10] */
public static final int AC_TYPE_NUMBER = 1204; /* [32-299-f10] */
public static final int AC_TYPE_OF_EXTERNAL_IDENTIFIER = 3168; /* [29.336-f10] */
public static final int AC_UAR_FLAGS = 637; /* [29.229-e20] */
public static final int AC_UDP_SOURCE_PORT = 2806; /* [29.212-f10] */
public static final int AC_UDR_FLAGS = 719; /* [29.329-f00] */
public static final int AC_UE_COUNT = 4308; /* [29.128-f10] */
public static final int AC_UE_LOCAL_IP_ADDRESS = 2805; /* [29.212-f10] */
public static final int AC_UE_PC5_AMBR = 1693; /* [29.272-f10] */
public static final int AC_UE_REACHABILITY_CONFIGURATION = 3129; /* [29.336-f10] */
public static final int AC_UE_REACHABILITY_CONFIGURATION_S6 = 3129; /* [29.272-f10] */
public static final int AC_UE_SRVCC_CAPABILITY = 1615; /* [29.272-f10] */
public static final int AC_UE_USAGE_TYPE = 1680; /* [29.272-f10] */
public static final int AC_ULA_FLAGS = 1406; /* [29.272-f10] */
public static final int AC_ULR_FLAGS = 1405; /* [29.272-f10] */
public static final int AC_UNI_PDU_CP_ONLY_FLAG = 3932; /* [32-299-f10] */
public static final int AC_UNIT_COST = 2061; /* [32-299-f10] */
public static final int AC_UNIT_QUOTA_THRESHOLD = 1226; /* [32-299-f10] */
public static final int AC_UNIT_VALUE = 445; /* [RFC 4006] */
public static final int AC_UNUSED_QUOTA_TIMER = 4407; /* [32-299-f10] */
public static final int AC_UPLINK_RATE_LIMIT = 4311; /* [29.128-f10] */
public static final int AC_USAGE_MONITORING_INFORMATION = 1067; /* [29.212-f10] */
public static final int AC_USAGE_MONITORING_LEVEL = 1068; /* [29.212-f10] */
public static final int AC_USAGE_MONITORING_REPORT = 1069; /* [29.212-f10] */
public static final int AC_USAGE_MONITORING_SUPPORT = 1070; /* [29.212-f10] */
public static final int AC_USE_ASSIGNED_ADDRESS = 534; /* [RFC 5777] */
public static final int AC_USED_SERVICE_UNIT = 446; /* [RFC 4006] */
public static final int AC_USED_SERVICE_UNIT_32299 = 446; /* [32-299-f10] */
public static final int AC_USER_AUTHORIZATION_TYPE = 623; /* [29.229-e20] */
public static final int AC_USER_CSG_INFORMATION = 2319; /* [32-299-f10] */
public static final int AC_USER_CSG_INFORMATION_S6 = 2319; /* [29.272-f10] */
public static final int AC_USER_DATA = 606; /* [29.229-e20] */
public static final int AC_USER_DATA_ALREADY_AVAILABLE = 624; /* [29.229-e20] */
public static final int AC_USER_DATA_SH = 702; /* [29.329-f00] */
public static final int AC_USER_EQUIPMENT_INFO = 458; /* [RFC 4006] */
public static final int AC_USER_EQUIPMENT_INFO_TYPE = 459; /* [RFC 4006] */
public static final int AC_USER_EQUIPMENT_INFO_VALUE = 460; /* [RFC 4006] */
public static final int AC_USER_ID = 1444; /* [29.272-f10] */
public static final int AC_USER_IDENTIFIER = 3102; /* [29.336-f10] */
public static final int AC_USER_IDENTIFIER_S6T = 3102; /* [29.336-f10] */
public static final int AC_USER_IDENTIFIER_T6 = 3102; /* [29.128-f10] */
public static final int AC_USER_IDENTITY = 700; /* [29.329-f00] */
public static final int AC_USER_LOCATION_INFO_TIME = 2812; /* [29.212-f10] */
public static final int AC_USER_NAME = 1; /* [RFC 6733] */
public static final int AC_USER_PARTICIPATING_TYPE = 1279; /* [32-299-f10] */
public static final int AC_USER_PASSWORD = 2; /* [RFC 7155] */
public static final int AC_USER_PRIORITY_RANGE = 557; /* [RFC 5777] */
public static final int AC_USER_SESSION_ID = 830; /* [32-299-f10] */
public static final int AC_USER_STATE = 1499; /* [29.272-f10] */
public static final int AC_UTRAN_ADDITIONAL_POSITIONING_DATA = 2558; /* [29.172-d10] */
public static final int AC_UTRAN_GANSS_POSTIONING_DATA = 2529; /* [29.172-d10] */
public static final int AC_UTRAN_POSITIONING_INFO = 2527; /* [29.172-d10] */
public static final int AC_UTRAN_POSTIONING_DATA = 2528; /* [29.172-d10] */
public static final int AC_UTRAN_VECTOR = 1415; /* [29.272-f10] */
public static final int AC_UVA_FLAGS = 1640; /* [29.272-f10] */
public static final int AC_UVR_FLAGS = 1639; /* [29.272-f10] */
public static final int AC_UWAN_USER_LOCATION_INFO = 3918; /* [32-299-f10] */
public static final int AC_V2X_PERMISSION = 1689; /* [29.272-f10] */
public static final int AC_V2X_SUBSCRIPTION_DATA = 1688; /* [29.272-f10] */
public static final int AC_VALIDITY_TIME = 448; /* [RFC 4006] */
public static final int AC_VALUE_DIGITS = 447; /* [RFC 4006] */
public static final int AC_VAS_ID = 1102; /* [29.140-700] */
public static final int AC_VASP_ID = 1101; /* [29.140-700] */
public static final int AC_VELOCITY_ESTIMATE = 2515; /* [29.172-d10] */
public static final int AC_VELOCITY_REQUESTED = 2508; /* [29.172-d10] */
public static final int AC_VENDOR_ID = 266; /* [RFC 6733] */
public static final int AC_VENDOR_SPECIFIC_APPLICATION_ID = 260; /* [RFC 6733] */
public static final int AC_VERTICAL_ACCURACY = 2506; /* [29.172-d10] */
public static final int AC_VERTICAL_REQUESTED = 2507; /* [29.172-d10] */
public static final int AC_VISITED_NETWORK_IDENTIFIER = 600; /* [29.229-e20] */
public static final int AC_VISITED_NETWORK_IDENTIFIER_S6 = 600; /* [29.272-f10] */
public static final int AC_VISITED_PLMN_ID = 1407; /* [29.272-f10] */
public static final int AC_VLAN_ID_RANGE = 552; /* [RFC 5777] */
public static final int AC_VOLUME_QUOTA_THRESHOLD = 869; /* [32-299-f10] */
public static final int AC_VPLMN_CSG_SUBSCRIPTION_DATA = 1641; /* [29.272-f10] */
public static final int AC_VPLMN_DYNAMIC_ADDRESS_ALLOWED = 1432; /* [29.272-f10] */
public static final int AC_VPLMN_LIPA_ALLOWED = 1617; /* [29.272-f10] */
public static final int AC_WAG_ADDRESS = 890; /* [32-299-f10] */
public static final int AC_WAG_PLMN_ID = 891; /* [32-299-f10] */
public static final int AC_WEBRTC_AUTHENTICATION_FUNCTION_NAME = 657; /* [29.229-e20] */
public static final int AC_WEBRTC_WEB_SERVER_FUNCTION_NAME = 658; /* [29.229-e20] */
public static final int AC_WILDCARDED_IMPU = 636; /* [29.329-f00] */
public static final int AC_WILDCARDED_PUBLIC_IDENTITY = 634; /* [29.229-e20] */
public static final int AC_WLAN_3GPP_IP_ACCESS = 306; /* [29.234-b20] */
public static final int AC_WLAN_ACCESS = 305; /* [29.234-b20] */
public static final int AC_WLAN_DIRECT_IP_ACCESS = 310; /* [29.234-b20] */
public static final int AC_WLAN_IDENTIFIER = 1509; /* [29.273-f10] */
public static final int AC_WLAN_INFORMATION = 875; /* [32-299-f10] */
public static final int AC_WLAN_OFFLOADABILITY = 1667; /* [29.272-f10] */
public static final int AC_WLAN_OFFLOADABILITY_EUTRAN = 1668; /* [29.272-f10] */
public static final int AC_WLAN_OFFLOADABILITY_UTRAN = 1669; /* [29.272-f10] */
public static final int AC_WLAN_RADIO_CONTAINER = 892; /* [32-299-f10] */
public static final int AC_WLAN_SESSION_ID = 1246; /* [32-299-f10] */
public static final int AC_WLAN_TECHNOLOGY = 893; /* [32-299-f10] */
public static final int AC_WLAN_UE_LOCAL_IPADDRESS = 894; /* [32-299-f10] */
public static final int AC_WLAN_USER_DATA = 303; /* [29.234-b20] */
public static final int AC_WLCP_KEY = 1535; /* [29.273-f10] */
public static final int AC_XRES = 1448; /* [29.272-f10] */
/* Redirect-Host-Usage AVP Values (code 261) */
public static final int RHU_DONT_CACHE = 0; /* [RFC6733] */
public static final int RHU_ALL_SESSION = 1; /* [RFC6733] */
public static final int RHU_ALL_REALM = 2; /* [RFC6733] */
public static final int RHU_REALM_AND_APPLICATION = 3; /* [RFC6733] */
public static final int RHU_ALL_APPLICATION = 4; /* [RFC6733] */
public static final int RHU_ALL_HOST = 5; /* [RFC6733] */
public static final int RHU_ALL_USER = 6; /* [RFC6733] */
/* Result-Code AVP Values (code 268) - Informational */
public static final int RESULT_DIAMETER_MULTI_ROUND_AUTH = 1001; /* [RFC6733] */
/* Result-Code AVP Values (code 268) - Success */
public static final int RC_DIAMETER_SUCCESS = 2001; /* [RFC6733] */
public static final int RC_DIAMETER_LIMITED_SUCCESS = 2002; /* [RFC6733] */
public static final int RC_DIAMETER_FIRST_REGISTRATION = 2003; /* [RFC4740] */
public static final int RC_DIAMETER_SUBSEQUENT_REGISTRATION = 2004; /* [RFC4740] */
public static final int RC_DIAMETER_UNREGISTERED_SERVICE = 2005; /* [RFC4740] */
public static final int RC_DIAMETER_SUCCESS_SERVER_NAME_NOT_STORED = 2006; /* [RFC4740] */
public static final int RC_DIAMETER_SERVER_SELECTION = 2007; /* [RFC4740] */
public static final int RC_DIAMETER_SUCCESS_AUTH_SENT_SERVER_NOT_STORED = 2008; /* [RFC4740] */
public static final int RC_DIAMETER_SUCCESS_RELOCATE_HA = 2009; /* [RFC5778] */
/* Result-Code AVP Values (code 268) - Protocol Errors */
public static final int RC_DIAMETER_COMMAND_UNSUPPORTED = 3001; /* [RFC6733] */
public static final int RC_DIAMETER_UNABLE_TO_DELIVER = 3002; /* [RFC6733] */
public static final int RC_DIAMETER_REALM_NOT_SERVED = 3003; /* [RFC6733] */
public static final int RC_DIAMETER_DIAMETER_TOO_BUSY = 3004; /* [RFC6733] */
public static final int RC_DIAMETER_LOOP_DETECTED = 3005; /* [RFC6733] */
public static final int RC_DIAMETER_REDIRECT_INDICATION = 3006; /* [RFC6733] */
public static final int RC_DIAMETER_APPLICATION_UNSUPPORTED = 3007; /* [RFC6733] */
public static final int RC_DIAMETER_INVALID_HDR_BITS = 3008; /* [RFC6733] */
public static final int RC_DIAMETER_INVALID_AVP_BITS = 3009; /* [RFC6733] */
public static final int RC_DIAMETER_UNKNOWN_PEER = 3010; /* [RFC6733] */
public static final int RC_DIAMETER_REALM_REDIRECT_INDICATION = 3011; /* [RFC7075] */
/* Result-Code AVP Values (code 268) - Transient Failures */
public static final int RC_DIAMETER_AUTHENTICATION_REJECTED = 4001; /* [RFC6733] */
public static final int RC_DIAMETER_OUT_OF_SPACE = 4002; /* [RFC6733] */
public static final int RC_ELECTION_LOST = 4003; /* [RFC6733] */
public static final int RC_DIAMETER_ERROR_MIP_REPLY_FAILURE = 4005; /* [RFC4004] */
public static final int RC_DIAMETER_ERROR_HA_NOT_AVAILABLE = 4006; /* [RFC4004] */
public static final int RC_DIAMETER_ERROR_BAD_KEY = 4007; /* [RFC4004] */
public static final int RC_DIAMETER_ERROR_MIP_FILTER_NOT_SUPPORTED = 4008; /* [RFC4004] */
public static final int RC_DIAMETER_END_USER_SERVICE_DENIED = 4010; /* [RFC4006] */
public static final int RC_DIAMETER_CREDIT_CONTROL_NOT_APPLICABLE = 4011; /* [RFC4006] */
public static final int RC_DIAMETER_CREDIT_LIMIT_REACHED = 4012; /* [RFC4006] */
public static final int RC_DIAMETER_USER_NAME_REQUIRED = 4013; /* [RFC4740] */
public static final int RC_DIAMETER_RESOURCE_FAILURE = 4014; /* [RFC6736] */
/* Result-Code AVP Values (code 268) - Permanent Failure */
public static final int RC_DIAMETER_AVP_UNSUPPORTED = 5001; /* [RFC6733] */
public static final int RC_DIAMETER_UNKNOWN_SESSION_ID = 5002; /* [RFC6733] */
public static final int RC_DIAMETER_AUTHORIZATION_REJECTED = 5003; /* [RFC6733] */
public static final int RC_DIAMETER_INVALID_AVP_VALUE = 5004; /* [RFC6733] */
public static final int RC_DIAMETER_MISSING_AVP = 5005; /* [RFC6733] */
public static final int RC_DIAMETER_RESOURCES_EXCEEDED = 5006; /* [RFC6733] */
public static final int RC_DIAMETER_CONTRADICTING_AVPS = 5007; /* [RFC6733] */
public static final int RC_DIAMETER_AVP_NOT_ALLOWED = 5008; /* [RFC6733] */
public static final int RC_DIAMETER_AVP_OCCURS_TOO_MANY_TIMES = 5009; /* [RFC6733] */
public static final int RC_DIAMETER_NO_COMMON_APPLICATION = 5010; /* [RFC6733] */
public static final int RC_DIAMETER_UNSUPPORTED_VERSION = 5011; /* [RFC6733] */
public static final int RC_DIAMETER_UNABLE_TO_COMPLY = 5012; /* [RFC6733] */
public static final int RC_DIAMETER_INVALID_BIT_IN_HEADER = 5013; /* [RFC6733] */
public static final int RC_DIAMETER_INVALID_AVP_LENGTH = 5014; /* [RFC6733] */
public static final int RC_DIAMETER_INVALID_MESSAGE_LENGTH = 5015; /* [RFC6733] */
public static final int RC_DIAMETER_INVALID_AVP_BIT_COMBO = 5016; /* [RFC6733] */
public static final int RC_DIAMETER_NO_COMMON_SECURITY = 5017; /* [RFC6733] */
public static final int RC_DIAMETER_RADIUS_AVP_UNTRANSLATABLE = 5018; /* [RFC4849] */
public static final int RC_DIAMETER_ERROR_NO_FOREIGN_HA_SERVICE = 5024; /* [RFC4004] */
public static final int RC_DIAMETER_ERROR_END_TO_END_MIP_KEY_ENCRYPTION = 5025; /* [RFC4004] */
public static final int RC_DIAMETER_USER_UNKNOWN = 5030; /* [RFC4006] */
public static final int RC_DIAMETER_RATING_FAILED = 5031; /* [RFC4006] */
public static final int RC_DIAMETER_ERROR_USER_UNKNOWN = 5032; /* [RFC4740] */
public static final int RC_DIAMETER_ERROR_IDENTITIES_DONT_MATCH = 5033; /* [RFC4740] */
public static final int RC_DIAMETER_ERROR_IDENTITY_NOT_REGISTERED = 5034; /* [RFC4740] */
public static final int RC_DIAMETER_ERROR_ROAMING_NOT_ALLOWED = 5035; /* [RFC4740] */
public static final int RC_DIAMETER_ERROR_IDENTITY_ALREADY_REGISTERED = 5036; /* [RFC4740] */
public static final int RC_DIAMETER_ERROR_AUTH_SCHEME_NOT_SUPPORTED = 5037; /* [RFC4740] */
public static final int RC_DIAMETER_ERROR_IN_ASSIGNMENT_TYPE = 5038; /* [RFC4740] */
public static final int RC_DIAMETER_ERROR_TOO_MUCH_DATA = 5039; /* [RFC4740] */
public static final int RC_DIAMETER_ERROR_NOT_SUPPORTED_USER_DATA = 5040; /* [RFC4740] */
public static final int RC_DIAMETER_ERROR_MIP6_AUTH_MODE = 5041; /* [RFC5778] */
public static final int RC_DIAMETER_UNKNOWN_BINDING_TEMPLATE_NAME = 5042; /* [RFC6736] */
public static final int RC_DIAMETER_BINDING_FAILURE = 5043; /* [RFC6736] */
public static final int RC_DIAMETER_MAX_BINDING_SET_FAILURE = 5044; /* [RFC6736] */
public static final int RC_DIAMETER_MAXIMUM_BINDINGS_REACHED_FOR_ENDPOINT = 5045; /* [RFC6736] */
public static final int RC_DIAMETER_SESSION_EXISTS = 5046; /* [RFC6736] */
public static final int RC_DIAMETER_INSUFFICIENT_CLASSIFIERS = 5047; /* [RFC6736] */
public static final int RC_DIAMETER_ERROR_EAP_CODE_UNKNOWN = 5048; /* [RFC6942] */
/* Session-Binding AVP Values (code 270) */
public static final int SESSION_BINDING_RE_AUTH = 1; /* [RFC6733] */
public static final int SESSION_BINDING_STR = 2; /* [RFC6733] */
public static final int SESSION_BINDING_ACCOUNTING = 4; /* [RFC6733] */
/* Session-Server-Failover AVP Values (code 271) */
public static final int SSF_REFUSE_SERVICE = 0; /* [RFC6733] */
public static final int SSF_TRY_AGAIN = 1; /* [RFC6733] */
public static final int SSF_ALLOW_SERVICE = 2; /* [RFC6733] */
public static final int SSF_TRY_AGAIN_ALLOW_SERVICE = 3; /* [RFC6733] */
/* Disconnect-Cause AVP Values (code 273) */
public static final int DC_REBOOTING = 0; /* [RFC6733] */
public static final int DC_BUSY = 1; /* [RFC6733] */
public static final int DC_DO_NOT_WANT_TO_TALK_TO_YOU = 2; /* [RFC6733] */
/* Auth-Request-Type AVP Values (code 274) */
public static final int ART_AUTHENTICATE_ONLY = 1; /* [RFC6733] */
public static final int ART_AUTHORIZE_ONLY = 2; /* [RFC6733] */
public static final int ART_AUTHORIZE_AUTHENTICATE = 3; /* [RFC6733] */
/* Auth-Session-State AVP Values (code 277) */
public static final int ASS_STATE_MAINTAINED = 0; /* [RFC6733] */
public static final int ASS_NO_STATE_MAINTAINED = 1; /* [RFC6733] */
/* Re-Auth-Request-Type AVP Values (code 285) */
public static final int RART_AUTHORIZE_ONLY = 0; /* [RFC6733] */
public static final int RART_AUTHORIZE_AUTHENTICATE = 1; /* [RFC6733] */
/* Termination-Cause AVP Values (code 295) */
public static final int TC_DIAMETER_LOGOUT = 1; /* [RFC3588andRFC6733] */
public static final int TC_DIAMETER_SERVICE_NOT_PROVIDED = 2; /* [RFC3588andRFC6733] */
public static final int TC_DIAMETER_BAD_ANSWER = 3; /* [RFC3588andRFC6733] */
public static final int TC_DIAMETER_ADMINISTRATIVE = 4; /* [RFC3588andRFC6733] */
public static final int TC_DIAMETER_LINK_BROKEN = 5; /* [RFC3588andRFC6733] */
public static final int TC_DIAMETER_AUTH_EXPIRED = 6; /* [RFC3588andRFC6733] */
public static final int TC_DIAMETER_USER_MOVED = 7; /* [RFC3588andRFC6733] */
public static final int TC_DIAMETER_SESSION_TIMEOUT = 8; /* [RFC3588andRFC6733] */
public static final int TC_USER_REQUEST = 11; /* [RFC2866andRFC7155] */
public static final int TC_LOST_CARRIER = 12; /* [RFC2866andRFC7155] */
public static final int TC_LOST_SERVICE = 13; /* [RFC2866andRFC7155] */
public static final int TC_IDLE_TIMEOUT = 14; /* [RFC2866andRFC7155] */
public static final int TC_SESSION_TIMEOUT = 15; /* [RFC2866andRFC7155] */
public static final int TC_ADMIN_RESET = 16; /* [RFC2866andRFC7155] */
public static final int TC_ADMIN_REBOOT = 17; /* [RFC2866andRFC7155] */
public static final int TC_PORT_ERROR = 18; /* [RFC2866andRFC7155] */
public static final int TC_NAS_ERROR = 19; /* [RFC2866andRFC7155] */
public static final int TC_NAS_REQUEST = 20; /* [RFC2866andRFC7155] */
public static final int TC_NAS_REBOOT = 21; /* [RFC2866andRFC7155] */
public static final int TC_PORT_UNNEEDED = 22; /* [RFC2866andRFC7155] */
public static final int TC_PORT_PREEMPTED = 23; /* [RFC2866andRFC7155] */
public static final int TC_PORT_SUSPENDED = 24; /* [RFC2866andRFC7155] */
public static final int TC_SERVICE_UNAVAILABLE = 25; /* [RFC2866andRFC7155] */
public static final int TC_CALLBACK = 26; /* [RFC2866andRFC7155] */
public static final int TC_USER_ERROR = 27; /* [RFC2866andRFC7155] */
public static final int TC_HOST_REQUEST = 28; /* [RFC2866andRFC7155] */
public static final int TC_SUPPLICANT_RESTART = 29; /* [RFC3580andRFC7155] */
public static final int TC_REAUTHENTICATION_FAILURE = 30; /* [RFC3580andRFC7155] */
public static final int TC_PORT_REINITIALIZED = 31; /* [RFC3580andRFC7155] */
public static final int TC_PORT_ADMINISTRATIVELY_DISABLED = 32; /* [RFC3580andRFC7155] */
/* Inband-Security-Id AVP (code 299) */
public static final int ISI_NO_INBAND_SECURITY = 0; /* [RFC6733] */
public static final int ISI_TLS = 1; /* [RFC6733] */
/* MIP-Feature-Vector AVP (code 337) */
public static final int MIP_MOBILE_NODE_HOME_ADDRESS_REQUESTED = 1; /* [RFC4004] */
public static final int MIP_HOME_ADDRESS_ALLOCATABLE_ONLY_IN_HOME_REALM = 2; /* [RFC4004] */
public static final int MIP_HOME_AGENT_REQUESTED = 4; /* [RFC4004] */
public static final int MIP_FOREIGN_HOME_AGENT_AVAILABLE = 8; /* [RFC4004] */
public static final int MIP_MN_HA_KEY_REQUESTED = 16; /* [RFC4004] */
public static final int MIP_MN_FA_KEY_REQUESTED = 32; /* [RFC4004] */
public static final int MIP_FA_HA_KEY_REQUESTED = 64; /* [RFC4004] */
public static final int MIP_HOME_AGENT_IN_FOREIGN_NETWORK = 128; /* [RFC4004] */
public static final int MIP_CO_LOCATED_MOBILE_NODE = 256; /* [RFC4004] */
/* MIP-Algorithm-Type AVP Values (code 345) */
public static final int MIP_HMAC_SHA_1 = 2; /* [RFC4004] */
/* MIP-Replay-Mode AVP Values (code 346) */
public static final int MIP_NONE = 1; /* [RFC4004] */
public static final int MIP_TIMESTAMPS = 2; /* [RFC4004] */
public static final int MIP_NONCES = 3; /* [RFC4004] */
/* SIP-Server-Assignment-Type AVP Values (375) */
public static final int SIP_SA_NO_ASSIGNMENT = 0; /* [RFC4740] */
public static final int SIP_SA_REGISTRATION = 1; /* [RFC4740] */
public static final int SIP_SA_RE_REGISTRATION = 2; /* [RFC4740] */
public static final int SIP_SA_UNREGISTERED_USER = 3; /* [RFC4740] */
public static final int SIP_SA_TIMEOUT_DEREGISTRATION = 4; /* [RFC4740] */
public static final int SIP_SA_USER_DEREGISTRATION = 5; /* [RFC4740] */
public static final int SIP_SA_TIMEOUT_DEREGISTRATION_STORE_SERVER_NAME = 6; /* [RFC4740] */
public static final int SIP_SA_USER_DEREGISTRATION_STORE_SERVER_NAME = 7; /* [RFC4740] */
public static final int SIP_SA_ADMINISTRATIVE_DEREGISTRATION = 8; /* [RFC4740] */
public static final int SIP_SA_AUTHENTICATION_FAILURE = 9; /* [RFC4740] */
public static final int SIP_SA_AUTHENTICATION_TIMEOUT = 10; /* [RFC4740] */
public static final int SIP_SA_DEREGISTRATION_TOO_MUCH_DATA = 11; /* [RFC4740] */
/* SIP-Authentication-Scheme AVP Values (377) */
public static final int SIP_DIGEST = 0; /* [RFC4740] */
/* SIP-Reason-Code AVP Values (384) */
public static final int SIP_PERMANENT_TERMINATION = 0; /* [RFC4740] */
public static final int SIP_NEW_SIP_SERVER_ASSIGNED = 1; /* [RFC4740] */
public static final int SIP_SERVER_CHANGE = 2; /* [RFC4740] */
public static final int SIP_REMOVE_SIP_SERVER = 3; /* [RFC4740] */
/* SIP-User-Authorization-Type AVP Values (387) */
public static final int SIP_UA_REGISTRATION = 0; /* [RFC4740] */
public static final int SIP_UA_DEREGISTRATION = 1; /* [RFC4740] */
public static final int SIP_UA_REGISTRATION_AND_CAPABILITIES = 2; /* [RFC4740] */
/* SIP-User-Data-Already-Available AVP Values (392) */
public static final int SIP_USER_DATA_NOT_AVAILABLE = 0; /* [RFC4740] */
public static final int SIP_USER_DATA_ALREADY_AVAILABLE = 1; /* [RFC4740] */
/* Accounting-Auth-Method AVP Values (code 406) */
public static final int AAM_PAP = 1; /* [RFC7155] */
public static final int AAM_CHAP = 2; /* [RFC7155] */
public static final int AAM_MS_CHAP_1 = 3; /* [RFC7155] */
public static final int AAM_MS_CHAP_2 = 4; /* [RFC7155] */
public static final int AAM_EAP = 5; /* [RFC7155] */
public static final int AAM_NONE = 7; /* [RFC7155] */
/* Origin-AAA-Protocol AVP Values (code 408) */
public static final int ORIGIN_AAA_PROTOCOL_RADIUS = 1; /* [RFC7155] */
/* CC-Request-Type AVP Values (code 416) */
public static final int CC_INITIAL_REQUEST = 1; /* [RFC4006] */
public static final int CC_UPDATE_REQUEST = 2; /* [RFC4006] */
public static final int CC_TERMINATION_REQUEST = 3; /* [RFC4006] */
public static final int CC_EVENT_REQUEST = 4; /* [RFC4006] */
/* CC-Session-Failover AVP Values (code 418) */
public static final int CC_FAILOVER_NOT_SUPPORTED = 0; /* [RFC4006] */
public static final int CC_FAILOVER_SUPPORTED = 1; /* [RFC4006] */
/* Check-Balance-Result AVP Values (code 422) */
public static final int CHECK_BALANCE_ENOUGH_CREDIT = 0; /* [RFC4006] */
public static final int CHECK_BALANCE_NO_CREDIT = 1; /* [RFC4006] */
/* Credit-Control AVP Values (code 426) */
public static final int CC_CREDIT_AUTHORIZATION = 0; /* [RFC4006] */
public static final int CC_RE_AUTHORIZATION = 1; /* [RFC4006] */
/* Credit-Control-Failure-Handling AVP Values (code 427) */
public static final int CC_TERMINATE = 0; /* [RFC4006] */
public static final int CC_CONTINUE = 1; /* [RFC4006] */
public static final int CC_RETRY_AND_TERMINATE = 2; /* [RFC4006] */
/* Direct-Debiting-Failure-Handling AVP Values (code 428) */
public static final int DD_TERMINATE_OR_BUFFER = 0; /* [RFC4006] */
public static final int DD_CONTINUE = 1; /* [RFC4006] */
/* Redirect-Address-Type AVP Values (code 433) */
public static final int RAT_IPV4_ADDRESS = 0; /* [RFC4006] */
public static final int RAT_IPV6_ADDRESS = 1; /* [RFC4006] */
public static final int RAT_URL = 2; /* [RFC4006] */
public static final int RAT_SIP_URI = 3; /* [RFC4006] */
/* Requested-Action AVP Values (436) */
public static final int RA_DIRECT_DEBITING = 0; /* [RFC4006] */
public static final int RA_REFUND_ACCOUNT = 1; /* [RFC4006] */
public static final int RA_CHECK_BALANCE = 2; /* [RFC4006] */
public static final int RA_PRICE_ENQUIRY = 3; /* [RFC4006] */
/* Final-Unit-Action AVP Values (code 449) */
public static final int FUA_TERMINATE = 0; /* [RFC4006] */
public static final int FUA_REDIRECT = 1; /* [RFC4006] */
public static final int FUA_RESTRICT_ACCESS = 2; /* [RFC4006] */
/* Subscription-Id-Type AVP Values (code 450) */
public static final int SIT_END_USER_EI64 = 0; /* [RFC4006] */
public static final int SIT_END_USER_IMSI = 1; /* [RFC4006] */
public static final int SIT_END_USER_SIP_URI = 2; /* [RFC4006] */
public static final int SIT_END_USER_NAI = 3; /* [RFC4006] */
public static final int SIT_END_USER_PRIVATE = 4; /* [RFC4006] */
/* CC-Unit-Type AVP Values (code 454) */
public static final int CC_TIME = 0; /* [RFC4006] */
public static final int CC_MONEY = 1; /* [RFC4006] */
public static final int CC_TOTAL_OCTETS = 2; /* [RFC4006] */
public static final int CC_INPUT_OCTETS = 3; /* [RFC4006] */
public static final int CC_OUTPUT_OCTETS = 4; /* [RFC4006] */
public static final int CC_SERVICE_SPECIFIC_UNITS = 5; /* [RFC4006] */
/* Multiple-Services-Indicator AVP Values (code 455) */
public static final int MULTIPLE_SERVICES_NOT_SUPPORTED = 0; /* [RFC4006] */
public static final int MULTIPLE_SERVICES_SUPPORTED = 1; /* [RFC4006] */
/* Tariff-Change-Usage AVP Values (code 452) */
public static final int TCU_UNIT_BEFORE_TARIFF_CHANGE = 0; /* [RFC4006] */
public static final int TCU_UNIT_AFTER_TARIFF_CHANGE = 1; /* [RFC4006] */
public static final int TCU_UNIT_INDETERMINATE = 2; /* [RFC4006] */
/* User-Equipment-Info-Type AVP Values (code 459) */
public static final int UEIT_IMEISV = 0; /* [RFC4006] */
public static final int UEIT_MAC = 1; /* [RFC4006] */
public static final int UEIT_EUI64 = 2; /* [RFC4006] */
public static final int UEIT_MODIFIED_EUI64 = 3; /* [RFC4006] */
/* Accounting-Record-Type AVP Values (code 480) */
public static final int ACCT_EVENT_RECORD = 1; /* [RFC6733] */
public static final int ACCT_START_RECORD = 2; /* [RFC6733] */
public static final int ACCT_INTERIM_RECORD = 3; /* [RFC6733] */
public static final int ACCT_STOP_RECORD = 4; /* [RFC6733] */
/* Accounting-Realtime-Required AVP Values (code 483) */
public static final int ACCT_DELIVER_AND_GRANT = 1; /* [RFC6733] */
public static final int ACCT_GRANT_AND_STORE = 2; /* [RFC6733] */
public static final int ACCT_GRANT_AND_LOSE = 3; /* [RFC6733] */
/* Treatment-Action AVP Values (code 572) */
public static final int TREATMENT_ACTION_DROP = 0; /* [RFC5777] */
public static final int TREATMENT_ACTION_SHAPE = 1; /* [RFC5777] */
public static final int TREATMENT_ACTION_MARK = 2; /* [RFC5777] */
public static final int TREATMENT_ACTION_PERMIT = 3; /* [RFC5777] */
/* QoS-Semantics AVP Values (code 575) */
public static final int QoS_DESIRED = 0; /* [RFC5777] */
public static final int QoS_AVAILABLE = 1; /* [RFC5777] */
public static final int QoS_DELIVERED = 2; /* [RFC5777] */
public static final int QoS_MINIMUM_QOS = 3; /* [RFC5777] */
public static final int QoS_AUTHORIZED = 4; /* [RFC5777] */
/* Key-Type AVP Values (code 582) */
public static final int DSRK = 0; /* [RFC6734] */
public static final int RRK = 1; /* [RFC6734] */
public static final int RMSK = 2; /* [RFC6734] */
public static final int IKEv2_SK = 3; /* [RFC6738] */
/* NC-Request-Type AVP Values (code 595) */
public static final int NC_INITIAL_REQUEST = 1; /* [RFC6736] */
public static final int NC_UPDATE_REQUEST = 2; /* [RFC6736] */
public static final int NC_QUERY_REQUEST = 3; /* [RFC6736] */
/* NAT-External-Port-Style AVP Values (code 604) */
public static final int NAT_FOLLOW_INTERNAL_PORT_STYLE = 1; /* [RFC6736] */
/* NAT-Control-Binding-Status (code 606) */
public static final int NAT_INITIAL_REQUEST = 1; /* [RFC6736] */
public static final int NAT_UPDATE_REQUEST = 2; /* [RFC6736] */
public static final int NAT_QUERY_REQUEST = 3; /* [RFC6736] */
/* OC-Feature-Vector AVP Values (code 622) */
public static final int OC_OLR_DEFAULT_ALGO = 0x0000000000000001; /* [RFC7683] */
/* OC-Report-Type AVP Values (code 626) */
public static final int OC_HOST_REPORT = 0; /* [RFC7683] */
public static final int OC_REALM_REPORT = 1; /* [RFC7683] */
/* Known Vendor IDs */
public static final int VENDOR_ID_NONE = 0;
public static final int VENDOR_ID_HP = 11; /* Hewlett Packard */
public static final int VENDOR_ID_SUN = 42; /* Sun Microsystems, Inc. */
public static final int VENDOR_ID_MERIT = 61; /* Merit Networks */
public static final int VENDOR_ID_NOKIA = 94; /* Nokia */
public static final int VENDOR_ID_ERICSSON = 193; /* Ericsson */
public static final int VENDOR_ID_USR = 429; /* US Robotics Corp. */
public static final int VENDOR_ID_ALU = 637; /* ALU Network */
public static final int VENDOR_ID_LUCENT = 1751; /* Lucent Technologies */
public static final int VENDOR_ID_HUAWEI = 2011; /* Huawei */
public static final int VENDOR_ID_3GPP2 = 5535; /* 3GPP2 */
public static final int VENDOR_ID_3GPP = 10415; /* 3GPP */
public static final int VENDOR_ID_VODAFONE = 12645; /* Vodafone */
public static final int VENDOR_ID_ETSI = 13019; /* ETSI */
/* Known Application IDs */
public static final int APP_ID_DIAMETER_COMMON_MESSAGE = 0; /* [RFC6733] */
public static final int APP_ID_NASREQ = 1; /* [RFC7155] */
public static final int APP_ID_MOBILE_IPv4 = 2; /* [RFC4004] */
public static final int APP_ID_DIAMETER_BASE_ACCOUNTING = 3; /* [RFC6733] */
public static final int APP_ID_DIAMETER_CREDIT_CONTROL = 4; /* [RFC4006] */
public static final int APP_ID_DIAMETER_EAP = 5; /* [RFC4072] */
public static final int APP_ID_DAMETER_SIP_APPLICATION = 6; /* [RFC4740] */
public static final int APP_ID_DIAMETER_MOBILE_IPv6_IKE = 7; /* [RFC5778] */
public static final int APP_ID_DIAMETER_MOBILE_IPv6_AUTH = 8; /* [RFC5778] */
public static final int APP_ID_DIAMETER_QOS_APPLICATION = 9; /* [RFC5866] */
public static final int APP_ID_DIAMETER_CAPABILITIES_UPDATE = 10; /* [RFC6737] */
public static final int APP_ID_DIAMETER_IKE_SK = 11; /* [RFC6738] */
public static final int APP_ID_DIAMETER_NAT_CONTROL_APPLICATION = 12; /* [RFC6736] */
public static final int APP_ID_DIAMETER_ERP = 13; /* [RFC6942] */
public static final int APP_ID_3GPP_Cx = 16777216;
public static final int APP_ID_3GPP_Sh = 16777217;
public static final int APP_ID_3GPP_Re = 16777218;
public static final int APP_ID_3GPP_Wx = 16777219;
public static final int APP_ID_3GPP_Zn = 16777220;
public static final int APP_ID_3GPP_Zh = 16777221;
public static final int APP_ID_3GPP_Gq = 16777222;
public static final int APP_ID_3GPP_Gmb = 16777223;
public static final int APP_ID_3GPP_Gx = 16777224;
public static final int APP_ID_3GPP_Gx_OVER_Gy = 16777225;
public static final int APP_ID_3GPP_MM10 = 16777226;
public static final int APP_ID_3GPP_Sta = 16777250;
public static final int APP_ID_3GPP_S6a = 16777251;
public static final int APP_ID_3GPP_SWm = 16777264;
public static final int APP_ID_3GPP_SWx = 16777265;
public static final int APP_ID_3GPP_Gxx = 16777266;
public static final int APP_ID_3GPP_S9 = 16777267;
public static final int APP_ID_3GPP_Zpn = 16777268;
public static final int APP_ID_3GPP_S6b = 16777272;
public static final int APP_ID_3GPP_SLh = 16777291;
public static final int APP_ID_3GPP_SGmb = 16777292;
public static final int APP_ID_3GPP_Sy = 16777302;
public static final int APP_ID_3GPP_Sd = 16777303;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy