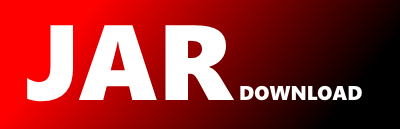
br.com.fluentvalidator.rule.RuleBuilderCollectionImpl Maven / Gradle / Ivy
Show all versions of java-fluent-validator Show documentation
package br.com.fluentvalidator.rule;
import java.util.Collection;
import java.util.LinkedList;
import java.util.Objects;
import java.util.function.Function;
import java.util.function.Predicate;
import br.com.fluentvalidator.Validator;
import br.com.fluentvalidator.annotation.CleanValidationContextException;
import br.com.fluentvalidator.builder.AttemptedValue;
import br.com.fluentvalidator.builder.Code;
import br.com.fluentvalidator.builder.Critical;
import br.com.fluentvalidator.builder.FieldName;
import br.com.fluentvalidator.builder.HandleInvalidField;
import br.com.fluentvalidator.builder.Message;
import br.com.fluentvalidator.builder.Must;
import br.com.fluentvalidator.builder.RuleBuilderCollection;
import br.com.fluentvalidator.builder.WhenCollection;
import br.com.fluentvalidator.builder.WheneverCollection;
import br.com.fluentvalidator.builder.WithValidator;
import br.com.fluentvalidator.context.ValidationContext;
import br.com.fluentvalidator.exception.ValidationException;
import br.com.fluentvalidator.handler.HandlerInvalidField;
public class RuleBuilderCollectionImpl extends AbstractRuleBuilder, WhenCollection, WheneverCollection> implements RuleBuilderCollection, WhenCollection, WheneverCollection {
private final Collection>> rules = new LinkedList<>();
private final RuleProcessorStrategy ruleProcessor = RuleProcessorStrategy.getFailFast();
private ValidationRule> currentValidation;
public RuleBuilderCollectionImpl(final String fieldName, final Function> function) {
super(fieldName, function);
}
public RuleBuilderCollectionImpl(final Function> function) {
super(function);
}
@Override
public boolean apply(final T instance) {
final Collection value = Objects.nonNull(instance) ? function.apply(instance) : null;
return ruleProcessor.process(instance, value, rules);
}
@Override
public WheneverCollection whenever(final Predicate> whenever) {
this.currentValidation = new ValidatorRuleInternal(fieldName, whenever);
this.rules.add(this.currentValidation);
return this;
}
@Override
public Must, WhenCollection, WheneverCollection> must(final Predicate> must) {
this.currentValidation = new ValidationRuleInternal(fieldName, must);
this.rules.add(this.currentValidation);
return this;
}
@Override
public Message, WhenCollection, WheneverCollection> withMessage(final String message) {
this.currentValidation.withMessage(obj -> message);
return this;
}
@Override
public Message, WhenCollection, WheneverCollection> withMessage(final Function message) {
this.currentValidation.withMessage(message);
return this;
}
@Override
public Code, WhenCollection, WheneverCollection> withCode(final String code) {
this.currentValidation.withCode(obj -> code);
return this;
}
@Override
public Code, WhenCollection, WheneverCollection> withCode(final Function code) {
this.currentValidation.withCode(code);
return this;
}
@Override
public FieldName, WhenCollection, WheneverCollection> withFieldName(final String fieldName) {
this.currentValidation.withFieldName(obj -> fieldName);
return this;
}
@Override
public FieldName, WhenCollection, WheneverCollection> withFieldName(final Function fieldName) {
this.currentValidation.withFieldName(fieldName);
return this;
}
@Override
public AttemptedValue, WhenCollection, WheneverCollection> withAttempedValue(final Object attemptedValue) {
this.currentValidation.withAttemptedValue(obj -> attemptedValue);
return this;
}
@Override
public AttemptedValue, WhenCollection, WheneverCollection> withAttempedValue(final Function attemptedValue) {
this.currentValidation.withAttemptedValue(attemptedValue);
return this;
}
@Override
public HandleInvalidField, WhenCollection, WheneverCollection> handlerInvalidField(final HandlerInvalidField> handlerInvalidField) {
this.currentValidation.withHandlerInvalidField(handlerInvalidField);
return this;
}
@Override
public Critical, WhenCollection, WheneverCollection> critical() {
this.currentValidation.critical();
return this;
}
@Override
public Critical, WhenCollection, WheneverCollection> critical(final Class extends ValidationException> clazz) {
this.currentValidation.critical(clazz);
return this;
}
@Override
public WithValidator, WhenCollection, WheneverCollection> withValidator(final Validator validator) {
this.currentValidation.withValidator(validator);
return this;
}
@Override
public WhenCollection when(final Predicate> when) {
this.currentValidation.when(when);
return this;
}
class ValidationRuleInternal extends AbstractValidationRule> {
ValidationRuleInternal(final Function fieldName, final Predicate> must) {
super.must(must);
super.withFieldName(fieldName);
}
@Override
public boolean support(final Collection instance) {
return Boolean.TRUE.equals(getWhen().test(instance));
}
@Override
@CleanValidationContextException
public boolean apply(final Object obj, final Collection
instance) {
final boolean apply = getMust().test(instance);
if (Boolean.FALSE.equals(apply)) {
ValidationContext.get().addErrors(getHandlerInvalid().handle(obj, instance));
}
if (Objects.nonNull(getCriticalException()) && Boolean.FALSE.equals(apply)) {
throw ValidationException.create(getCriticalException());
}
return !(Boolean.TRUE.equals(isCritical()) && Boolean.FALSE.equals(apply));
}
}
class ValidatorRuleInternal extends AbstractValidationRule
> {
ValidatorRuleInternal(final Function fieldName, final Predicate> whenever) {
super.whenever(whenever);
super.withFieldName(fieldName);
}
@Override
public boolean support(final Collection instance) {
return Boolean.TRUE.equals(getWhenever().test(instance));
}
@Override
@CleanValidationContextException
public boolean apply(final Object obj, final Collection
instance) {
final boolean apply = ruleProcessor.process(obj, instance, getValidator());
if (Objects.nonNull(getCriticalException()) && Boolean.FALSE.equals(apply)) {
throw ValidationException.create(getCriticalException());
}
return !(Boolean.TRUE.equals(isCritical()) && Boolean.FALSE.equals(apply));
}
}
}