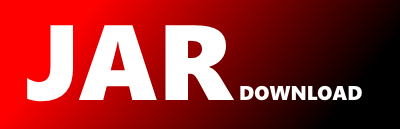
com.github.mvysny.fakeservlet.SessionAttributeMap.kt Maven / Gradle / Ivy
The newest version!
package com.github.mvysny.fakeservlet
import java.util.AbstractMap
import jakarta.servlet.http.HttpSession
/**
* A live map of all attributes in this session. Modifications to the map will be
* reflected to the session and vice versa.
*/
public val HttpSession.attributes: MutableMap
get() = SessionAttributeMap(this)
private class SessionAttributeEntrySetIterator(val session: HttpSession) : MutableIterator> {
/**
* Copy the attribute names, otherwise [remove] would throw [ConcurrentModificationException].
*/
private val attrNames: Iterator = session.attributeNames.toList().iterator()
private var lastAttributeName: String? = null
override fun hasNext(): Boolean = attrNames.hasNext()
override fun next(): MutableMap.MutableEntry {
if (!hasNext()) {
throw NoSuchElementException()
}
lastAttributeName = attrNames.next()
return AbstractMap.SimpleEntry(lastAttributeName!!, session.getAttribute(lastAttributeName!!))
}
override fun remove() {
check(lastAttributeName != null)
session.removeAttribute(lastAttributeName!!)
lastAttributeName = null
}
}
private class SessionAttributeEntrySet(val session: HttpSession) : AbstractMutableSet>() {
override val size: Int
get() = session.attributeNames.asSequence().count()
override fun isEmpty(): Boolean = !session.attributeNames.hasMoreElements()
override fun add(element: MutableMap.MutableEntry): Boolean {
val modified: Boolean = session.getAttribute(element.key) != element.value
if (modified) {
session.setAttribute(element.key, element.value)
}
return modified
}
override fun iterator(): MutableIterator> =
SessionAttributeEntrySetIterator(session)
override fun remove(element: MutableMap.MutableEntry): Boolean {
val contains: Boolean = contains(element)
if (contains) {
session.removeAttribute(element.key)
}
return contains
}
override fun contains(element: MutableMap.MutableEntry): Boolean =
session.getAttribute(element.key) == element.value
}
private class SessionAttributeMap(val session: HttpSession) : AbstractMutableMap() {
override val entries: MutableSet> =
SessionAttributeEntrySet(session)
override fun get(key: String): Any? = session.getAttribute(key)
override fun put(key: String, value: Any): Any? {
val old: Any? = session.getAttribute(key)
session.setAttribute(key, value)
return old
}
override fun remove(key: String): Any? {
val old: Any? = session.getAttribute(key)
session.removeAttribute(key)
return old
}
override fun remove(key: String, value: Any): Boolean = if (get(key) == value) {
remove(key)
true
} else {
false
}
override fun isEmpty(): Boolean = entries.isEmpty()
override fun containsKey(key: String): Boolean = session.getAttribute(key) != null
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy