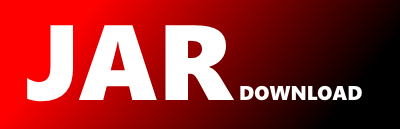
com.github.mvysny.karibudsl.v8.TreeIterator.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of karibu-dsl-v8 Show documentation
Show all versions of karibu-dsl-v8 Show documentation
Karibu-DSL, Kotlin extensions/DSL for Vaadin
The newest version!
package com.github.mvysny.karibudsl.v8
import com.vaadin.ui.Component
import com.vaadin.ui.HasComponents
import java.util.*
/**
* Given a tree root and a closure which computes children, iterates recursively over a tree of objects.
* Iterator works breadth-first: initially the root itself is offered, then its children, then the child's children etc.
* @property root the root object of the tree
* @property children for given node, returns a list of its children.
*/
public class TreeIterator(private val root: T, private val children: (T) -> Iterable) : Iterator {
/**
* The items to iterate over. Gradually filled with children, until there are no more items to iterate over.
*/
private val queue: Queue = LinkedList(listOf(root))
override fun hasNext(): Boolean = !queue.isEmpty()
override fun next(): T {
if (!hasNext()) throw NoSuchElementException()
val result = queue.remove()
queue.addAll(children(result))
return result
}
}
/**
* Walks over this component and all descendants of this component, breadth-first.
* @return iterable which iteratively walks over this component and all of its descendants.
*/
public fun (@VaadinDsl Component).walk(): Iterable = Iterable {
TreeIterator(this) { component -> component as? HasComponents ?: listOf() }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy